- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master Go's Context Package for Goroutines
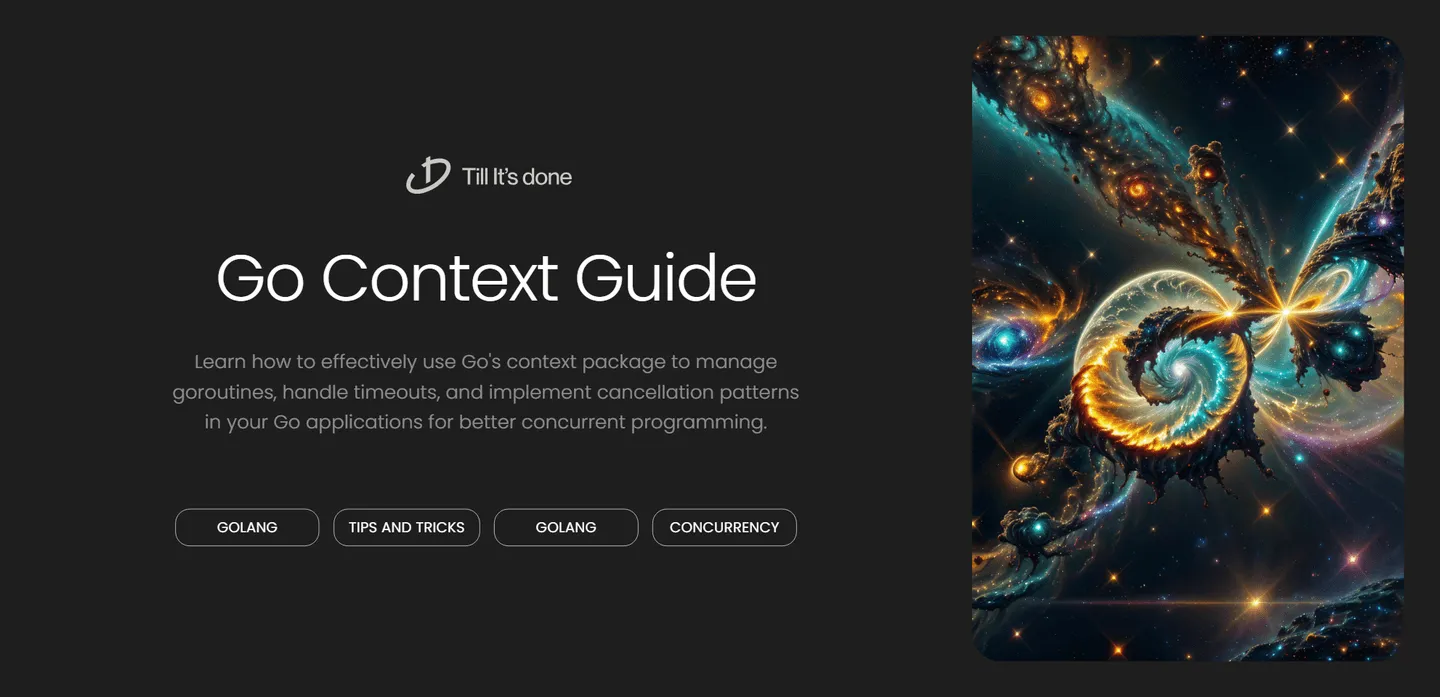
Using Go’s context package for better control of goroutines
Have you ever found yourself in a situation where your Go application has multiple goroutines running wild, and you’re not quite sure how to gracefully manage them? Maybe you’ve got a service that needs to handle timeouts properly, or you’re building a web server that should cancel ongoing operations when a client disconnects. Enter Go’s context package – your trusty companion for bringing order to concurrent chaos.
Understanding the Context Package
Think of context as a tool for carrying deadlines, cancellation signals, and request-scoped values across API boundaries and between processes. It’s like a smart contract between your goroutines, ensuring they behave well and clean up after themselves when their time is up.
Let’s dive into some practical examples that’ll make your life easier:
func main() { // Create a context with a timeout of 2 seconds ctx, cancel := context.WithTimeout(context.Background(), 2*time.Second) defer cancel() // Don't forget to release resources!
go worker(ctx) time.Sleep(3 * time.Second)}
func worker(ctx context.Context) { for { select { case <-ctx.Done(): fmt.Println("Time to clean up!") return default: // Do some work fmt.Println("Working...") time.Sleep(500 * time.Millisecond) } }}
Advanced Context Patterns
One of the most powerful aspects of context is its ability to carry request-scoped values. But remember – with great power comes great responsibility! Here’s a pattern I’ve found particularly useful:
type key int
const userIDKey key = 0
func enrichContext(ctx context.Context, userID string) context.Context { return context.WithValue(ctx, userIDKey, userID)}
func processRequest(ctx context.Context) { if userID, ok := ctx.Value(userIDKey).(string); ok { fmt.Printf("Processing request for user: %s\n", userID) }}
Best Practices and Tips
- Always pass context as the first parameter to your functions
- Don’t store contexts in structs; pass them explicitly
- Use context values sparingly – they’re not a replacement for proper parameter passing
- Remember to call cancel() when you’re done with a context
When building production services, proper context management can mean the difference between a graceful shutdown and a resource leak nightmare. Here’s a real-world example I often use in HTTP servers:
func handler(w http.ResponseWriter, r *http.Request) { ctx, cancel := context.WithTimeout(r.Context(), 5*time.Second) defer cancel()
select { case result := <-doExpensiveOperation(ctx): fmt.Fprintf(w, "Result: %v", result) case <-ctx.Done(): http.Error(w, "Request timed out", http.StatusGatewayTimeout) }}
Conclusion
The context package might seem like a small piece of Go’s standard library, but it’s one of those tools that, once mastered, becomes indispensable. Whether you’re building microservices, CLI applications, or complex distributed systems, proper context management will make your code more reliable, maintainable, and production-ready.






Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.