- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Understanding Go's defer Statement Guide
Learn best practices, common pitfalls, and practical examples to write more maintainable Go programs.

Understanding Go’s defer statement and best practices
Go’s defer statement is one of those features that, once mastered, becomes an indispensable tool in your programming arsenal. Think of it as your code’s cleanup crew, always ready to tidy up after your function finishes its work. Let’s dive deep into understanding how defer works and how to use it effectively.
What is defer?
The defer statement is like leaving a sticky note for your future self. When you defer a function call, you’re essentially saying, “Hey, make sure to run this before you leave the room.” The deferred function calls are executed in a Last-In-First-Out (LIFO) order, right before the surrounding function returns.
Common Use Cases
The most common and practical uses of defer include:
- File Operations
func readFile(filename string) error { file, err := os.Open(filename) if err != nil { return err } defer file.Close() // Rest of the file operations}
- Mutex Locks
func updateCache() { mu.Lock() defer mu.Unlock() // Protected code here}
- Database Connections
func queryDB() { conn := db.Connect() defer conn.Close() // Database operations}
Best Practices and Tips
1. Defer Right After Acquisition
Always place your defer statement right after acquiring a resource. This ensures you don’t forget about cleanup, even if you add more code later.
2. Watch Out for Loop Usage
Be careful when using defer inside loops. Remember that deferred functions won’t execute until the function returns, not when the loop iteration ends.
// Don't do thisfunc processFiles(filenames []string) error { for _, filename := range filenames { file, err := os.Open(filename) if err != nil { return err } defer file.Close() // This will stack up! } return nil}
// Do this insteadfunc processFiles(filenames []string) error { for _, filename := range filenames { if err := processFile(filename); err != nil { return err } } return nil}
func processFile(filename string) error { file, err := os.Open(filename) if err != nil { return err } defer file.Close() return nil}
3. Mind the Order
Remember that deferred functions are executed in LIFO order. This can be particularly important when dealing with dependent resources:
func process() { defer fmt.Println("First") defer fmt.Println("Second") defer fmt.Println("Third") // This will print: // Third // Second // First}
4. Be Careful with Named Return Values
When using named return values, defer can modify them:
func example() (result int) { defer func() { result *= 2 }() return 5 // Will return 10, not 5}
Conclusion
The defer statement is a powerful feature that helps write cleaner, more maintainable code. By following these best practices, you can avoid common pitfalls and make the most of this elegant Go feature. Remember: defer is not just about cleanup – it’s about writing code that’s both robust and readable.




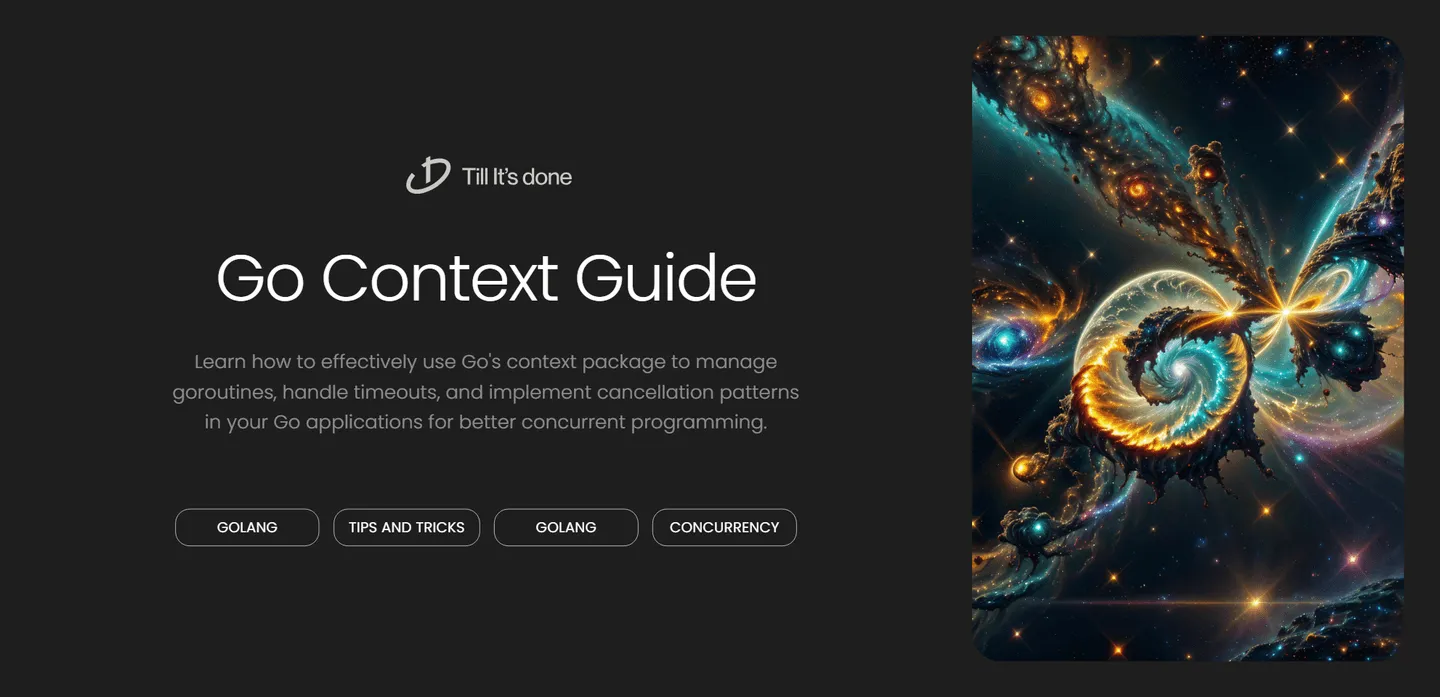

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.