- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master Go Concurrency: Goroutines & Channels
Discover practical patterns, best practices, and tips for writing efficient concurrent programs in Go.

Mastering Go Concurrency with Goroutines and Channels
Concurrency is one of Go’s most powerful features, setting it apart from many other programming languages. Today, let’s dive deep into how you can leverage goroutines and channels to write efficient, concurrent programs that can handle multiple tasks simultaneously.
Understanding Goroutines: Your Lightweight Threading Companion
Think of goroutines as tiny, efficient workers in your program. Unlike traditional threads that might consume megabytes of memory, goroutines start with just a few kilobytes. They’re like nimble ninjas that can quickly switch between tasks without breaking a sweat.
Here’s how simple it is to create a goroutine:
func main() { go sayHello("World") // Do other things time.Sleep(1 * time.Second)}
func sayHello(name string) { fmt.Printf("Hello, %s!\n", name)}
Channels: The Communication Superhighway
Channels are the magic that makes Go’s concurrency truly shine. They’re like secure pipelines where goroutines can safely pass data back and forth. No more worrying about race conditions or complex locking mechanisms.
func main() { messages := make(chan string)
go func() { messages <- "Ping!" }()
msg := <-messages fmt.Println(msg)}
Practical Patterns and Best Practices
The Worker Pool Pattern
One of the most powerful patterns in Go concurrency is the worker pool. Imagine having a team of workers efficiently processing tasks from a queue:
func worker(id int, jobs <-chan int, results chan<- int) { for job := range jobs { results <- job * 2 }}
func main() { jobs := make(chan int, 100) results := make(chan int, 100)
// Start 3 workers for w := 1; w <= 3; w++ { go worker(w, jobs, results) }
// Send jobs for j := 1; j <= 9; j++ { jobs <- j } close(jobs)
// Collect results for a := 1; a <= 9; a++ { <-results }}
Select Statement: The Traffic Controller
The select statement is your friend when dealing with multiple channels. It’s like a smart traffic controller, managing different data streams efficiently:
func main() { ch1 := make(chan string) ch2 := make(chan string)
go func() { time.Sleep(1 * time.Second) ch1 <- "One" }()
go func() { time.Sleep(2 * time.Second) ch2 <- "Two" }()
for i := 0; i < 2; i++ { select { case msg1 := <-ch1: fmt.Println("Received", msg1) case msg2 := <-ch2: fmt.Println("Received", msg2) } }}
Tips for Success
- Always close your channels when you’re done with them
- Use buffered channels when you need to prevent goroutine blocking
- Remember that goroutines need to be synchronized before your program exits
- Profile your application to find the right number of goroutines for your use case
Conclusion
Mastering Go’s concurrency features takes time and practice, but the payoff is worth it. With goroutines and channels, you can write elegant, efficient, and scalable concurrent programs that make the most of modern hardware. Keep experimenting, and you’ll discover even more powerful patterns and techniques to level up your Go programming skills.
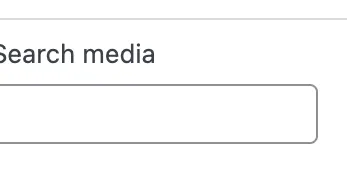





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.