- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Optimize Memory Usage in Go Applications
Discover techniques for efficient memory allocation, data structure optimization, and performance monitoring.
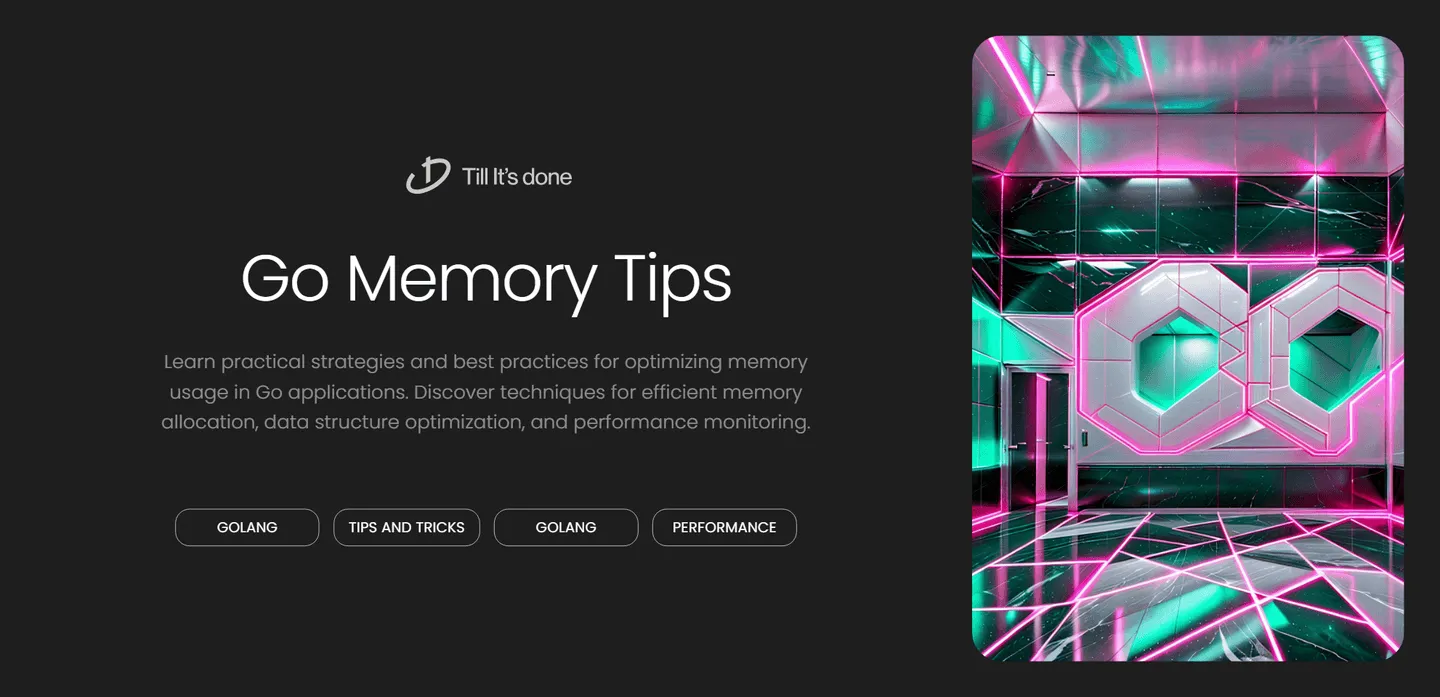
Optimizing Memory Usage in Go Applications
Memory management is crucial for building efficient Go applications. While Go’s garbage collector handles most memory-related tasks automatically, understanding and implementing proper memory optimization techniques can significantly improve your application’s performance. Let’s explore some practical strategies to optimize memory usage in your Go applications.
Understanding Memory Allocation in Go
When writing Go applications, every allocation counts. The way we handle data structures and manage resources directly impacts our application’s memory footprint. Memory optimization isn’t just about reducing usage—it’s about using memory more efficiently.
Use Sync.Pool for Temporary Objects
One of the most effective ways to reduce memory allocations is using sync.Pool
. This mechanism allows you to reuse temporary objects, reducing the pressure on the garbage collector:
var bufferPool = sync.Pool{ New: func() interface{} { return new(bytes.Buffer) },}
func processData(data []byte) { buffer := bufferPool.Get().(*bytes.Buffer) defer func() { buffer.Reset() bufferPool.Put(buffer) }()
// Use buffer for processing}
Pre-allocate Slices When Possible
If you know the size of your slice beforehand, pre-allocating it can prevent multiple resize operations:
// Instead ofdata := make([]int, 0)for i := 0; i < 10000; i++ { data = append(data, i)}
// Do thisdata := make([]int, 0, 10000)for i := 0; i < 10000; i++ { data = append(data, i)}
Advanced Memory Optimization Techniques
Optimize String Operations
String concatenation can be memory-intensive. Use strings.Builder for efficient string operations:
var builder strings.Builderbuilder.Grow(100) // Pre-allocate if you know the approximate sizefor i := 0; i < 100; i++ { builder.WriteString("item")}result := builder.String()
Use Proper Data Structures
Choose appropriate data structures based on your use case. For instance, use maps instead of slices for lookups, and consider using struct fields instead of map[string]interface{} when the structure is known:
// Instead oftype Config map[string]interface{}
// Usetype Config struct { Name string Version int Feature bool}
Monitoring and Profiling
Remember to regularly monitor your application’s memory usage using Go’s built-in tools:
import _ "net/http/pprof"
func main() { go func() { http.ListenAndServe(":6060", nil) }() // Your application code}
Final Thoughts
Memory optimization is an ongoing process that requires careful consideration and monitoring. By implementing these techniques and regularly profiling your application, you can maintain efficient memory usage and improve overall performance.
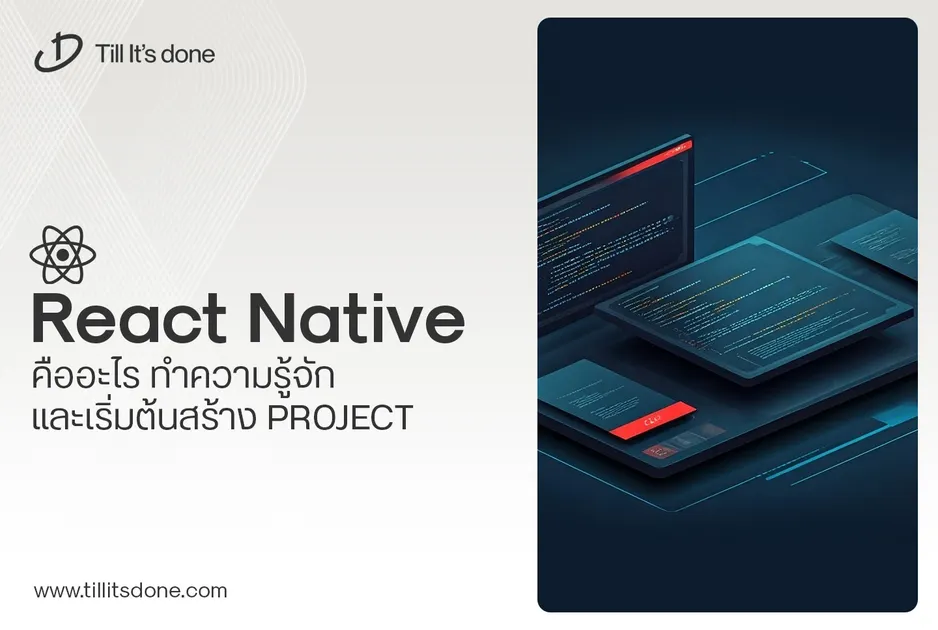
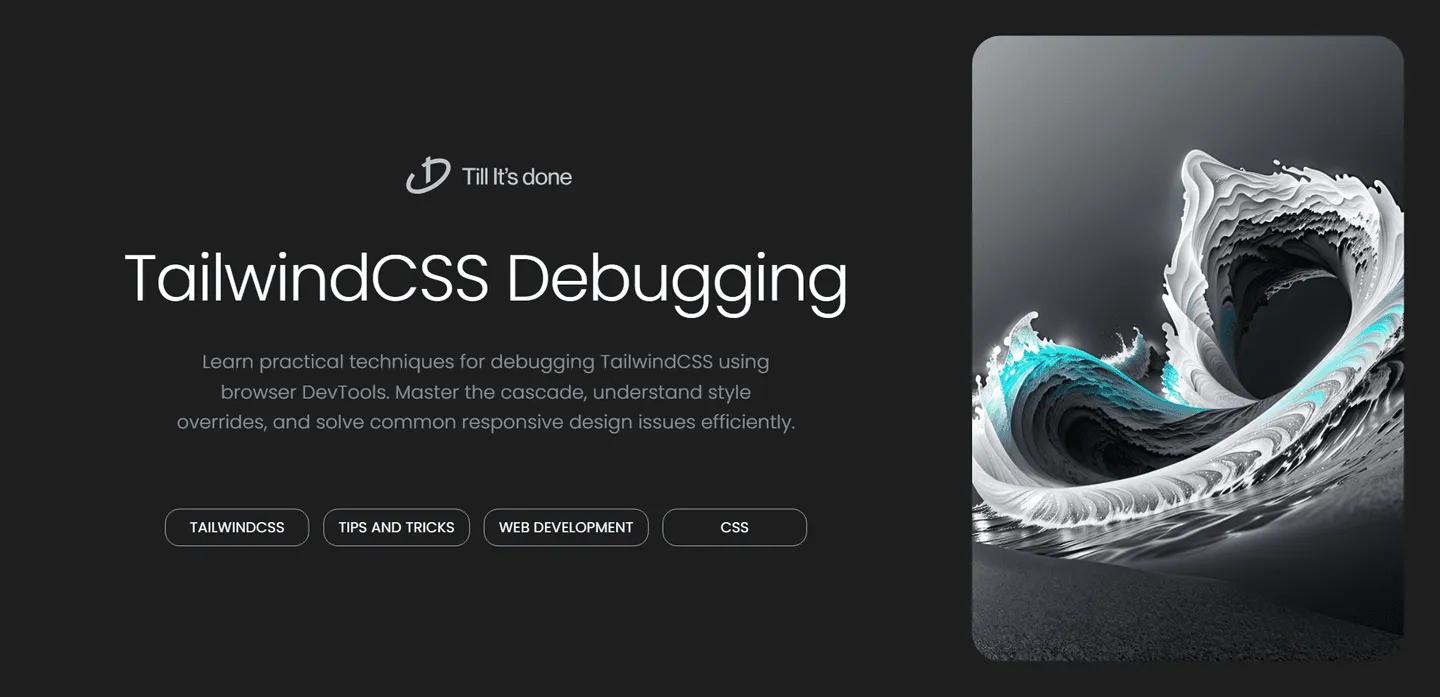
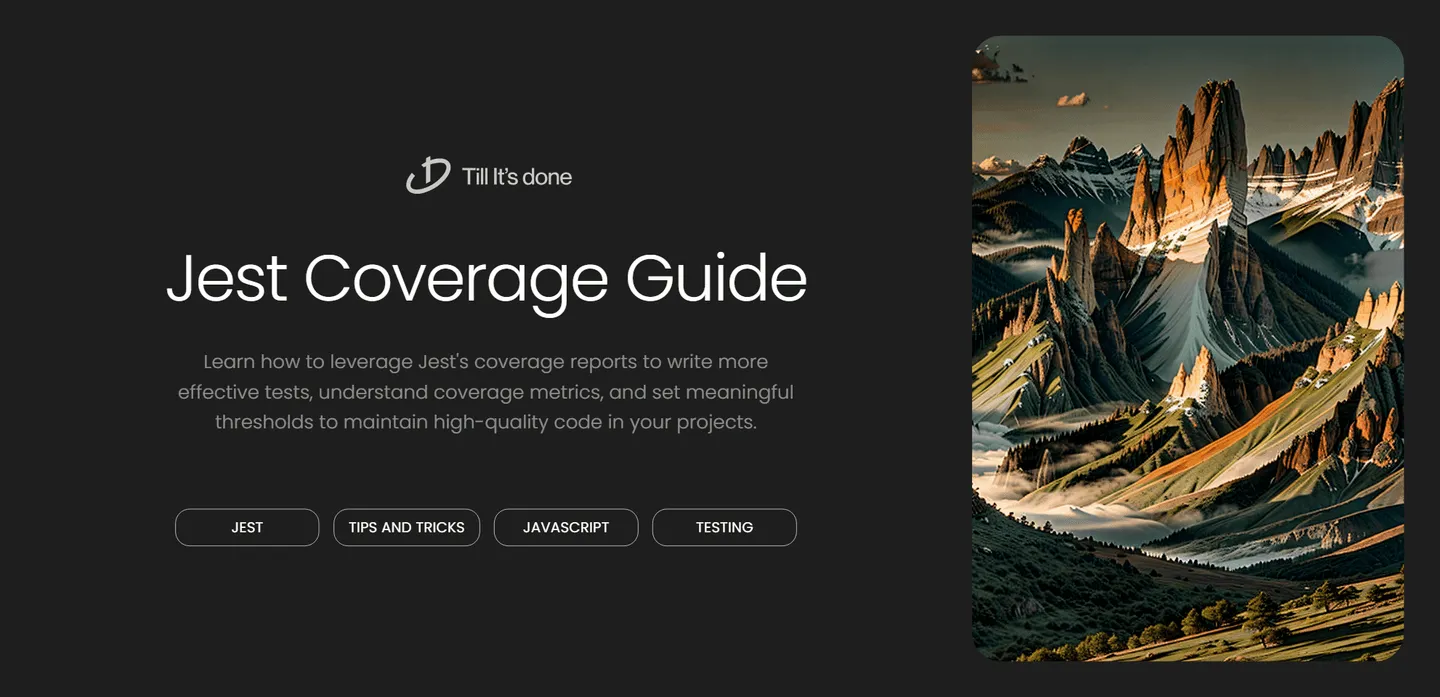
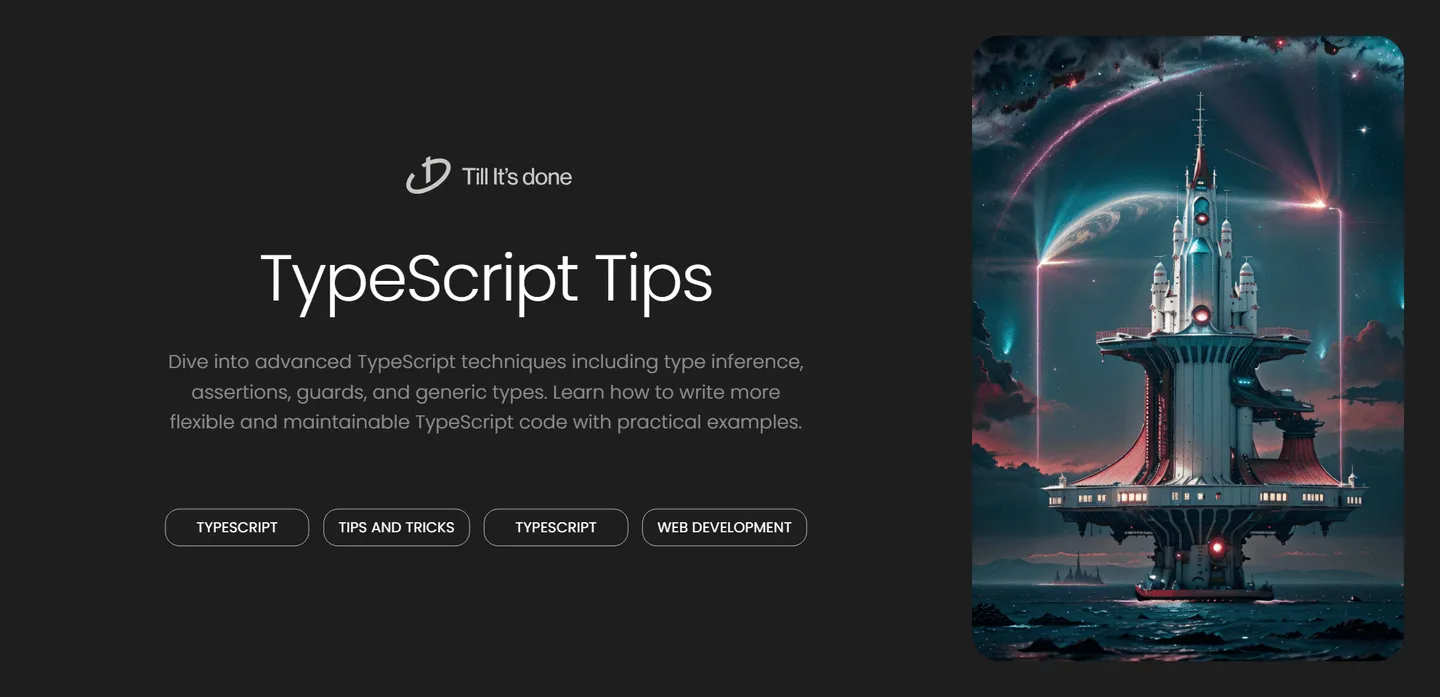
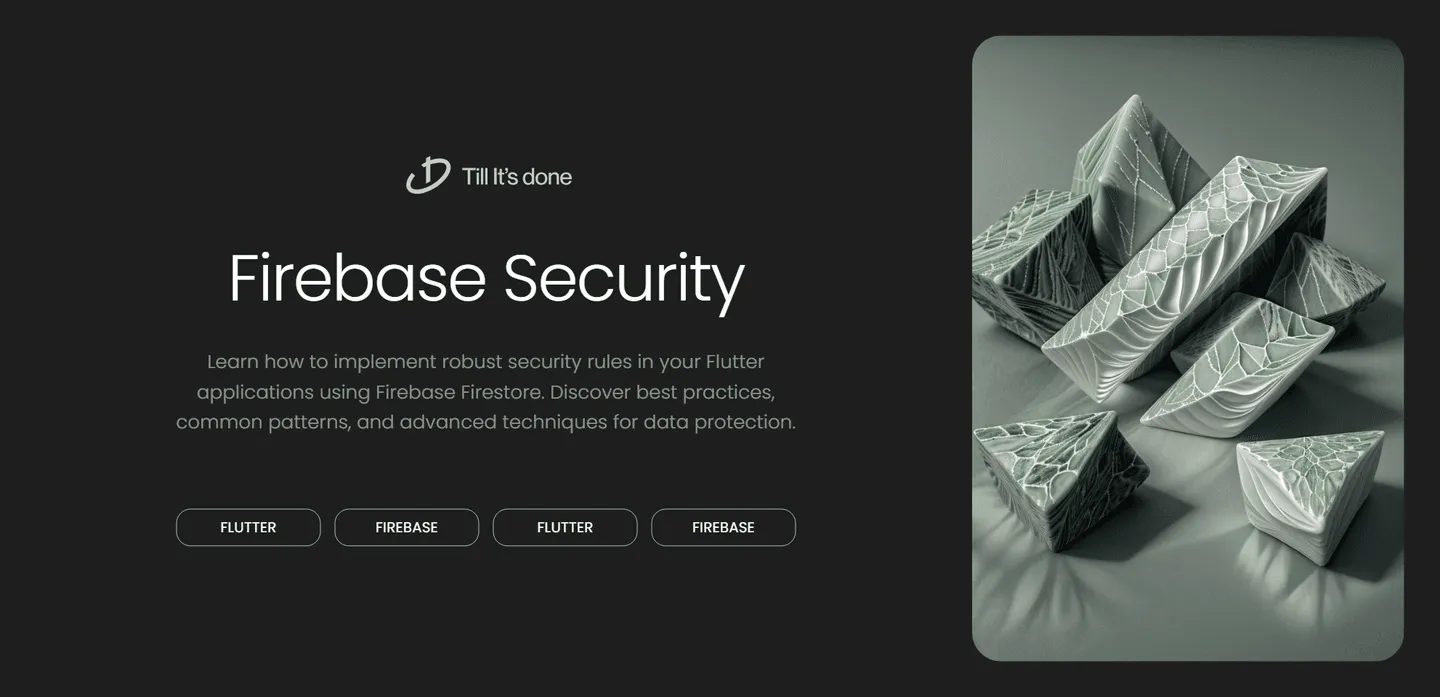
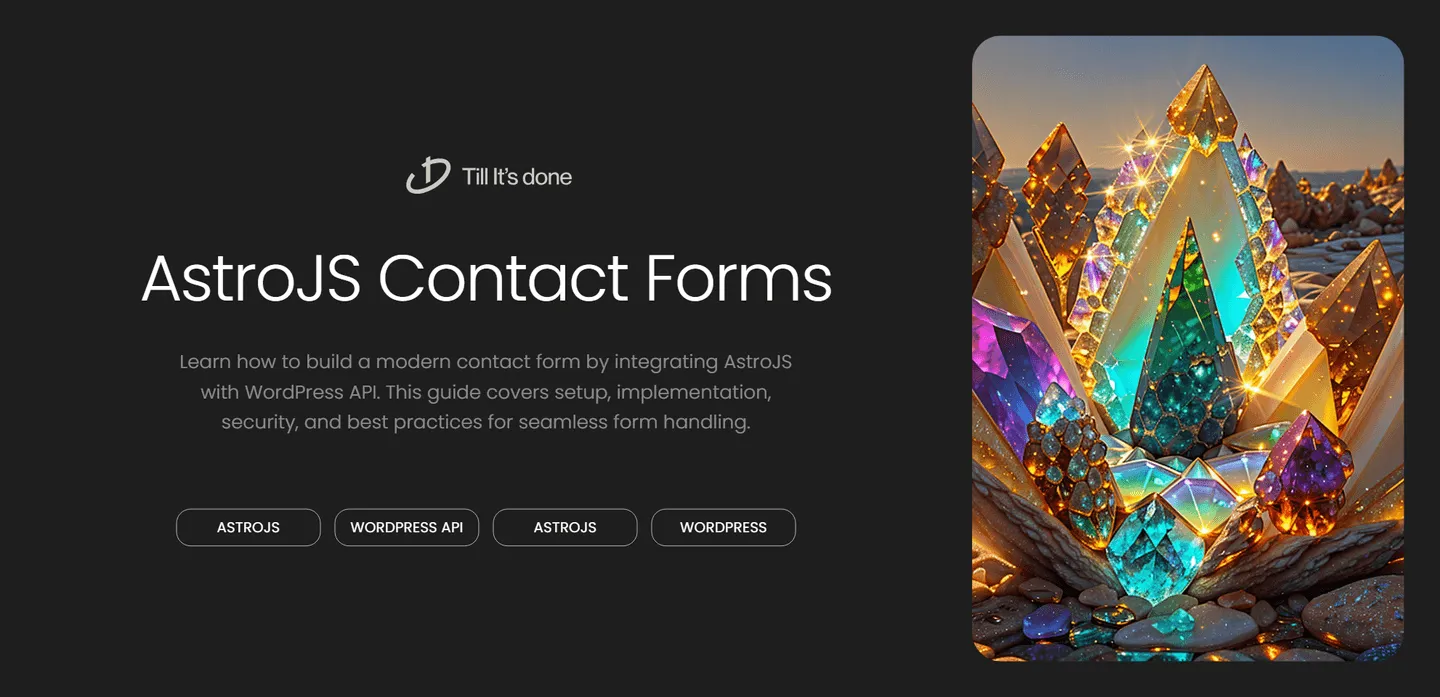
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.