- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Go Idioms: Writing Go Code Like a Pro
Learn about multiple return values, interfaces, error handling, and struct embedding.
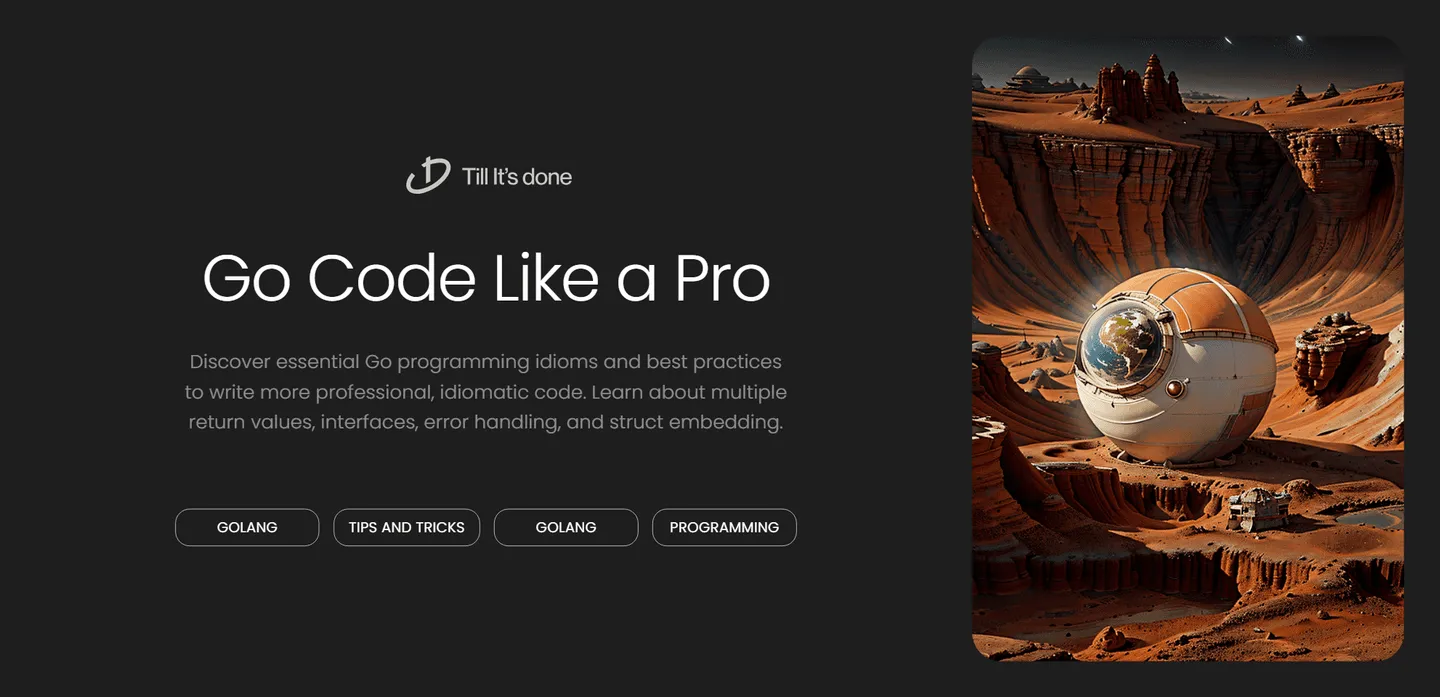
Go Idioms: Writing Go Code Like a Pro
Every programming language has its own unique way of doing things, and Go is no exception. Today, let’s dive into some powerful Go idioms that will help you write more idiomatic and professional Go code.
Multiple Return Values - The Go Way
One of Go’s most distinctive features is its ability to return multiple values from a function. Instead of using complex objects or pointer parameters, Go encourages explicit error handling through multiple returns.
func divide(x, y float64) (float64, error) { if y == 0 { return 0, errors.New("division by zero") } return x / y, nil}
Embracing Interfaces
Go’s interface system is beautifully simple yet powerful. Instead of large, complex interfaces, Go prefers small, focused ones. This approach, known as interface segregation, leads to more flexible and maintainable code.
type Reader interface { Read(p []byte) (n int, err error)}
type Writer interface { Write(p []byte) (n int, err error)}
The Empty Interface and Type Assertions
While Go is statically typed, the empty interface interface{}
(or any
in modern Go) allows us to handle values of unknown types. However, use this judiciously - it’s not a substitute for proper type design.
func printAny(v interface{}) { switch v := v.(type) { case string: fmt.Printf("String: %s\n", v) case int: fmt.Printf("Integer: %d\n", v) default: fmt.Printf("Unknown type: %T\n", v) }}
Struct Embedding for Composition
Go doesn’t have inheritance, but it does have composition through embedding. This leads to cleaner, more maintainable code that avoids the complexity of deep inheritance hierarchies.
type Logger struct { Level string}
type Server struct { Logger // Embedded struct Address string Port int}
The init() Function
While not always necessary, the init()
function can be useful for setting up package-level state. Remember that each package can have multiple init()
functions, and they’re called in the order they’re defined.
var logger *log.Logger
func init() { logger = log.New(os.Stdout, "app: ", log.LstdFlags)}
Error Handling Patterns
Error handling in Go is explicit and straightforward. Embrace it rather than fighting it. Use custom errors when needed, and always provide context with your errors.
type ValidationError struct { Field string Error string}
func (v *ValidationError) Error() string { return fmt.Sprintf("validation failed on %s: %s", v.Field, v.Error)}
Closing Thoughts
Writing idiomatic Go code isn’t just about following rules - it’s about embracing the language’s philosophy of simplicity and explicitness. The more you work with Go, the more these patterns will become second nature.
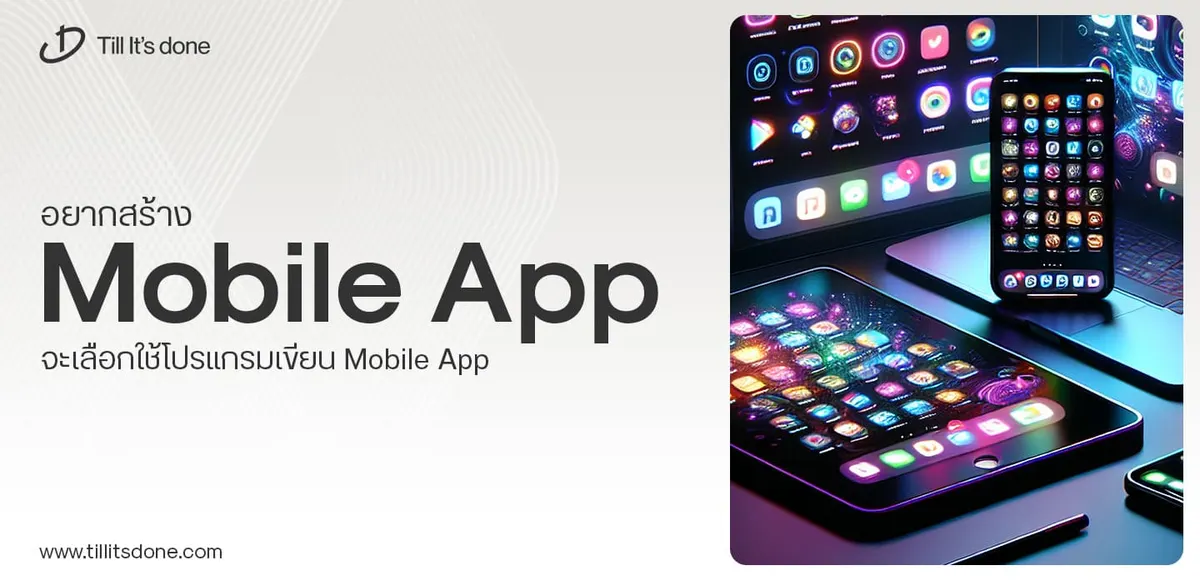
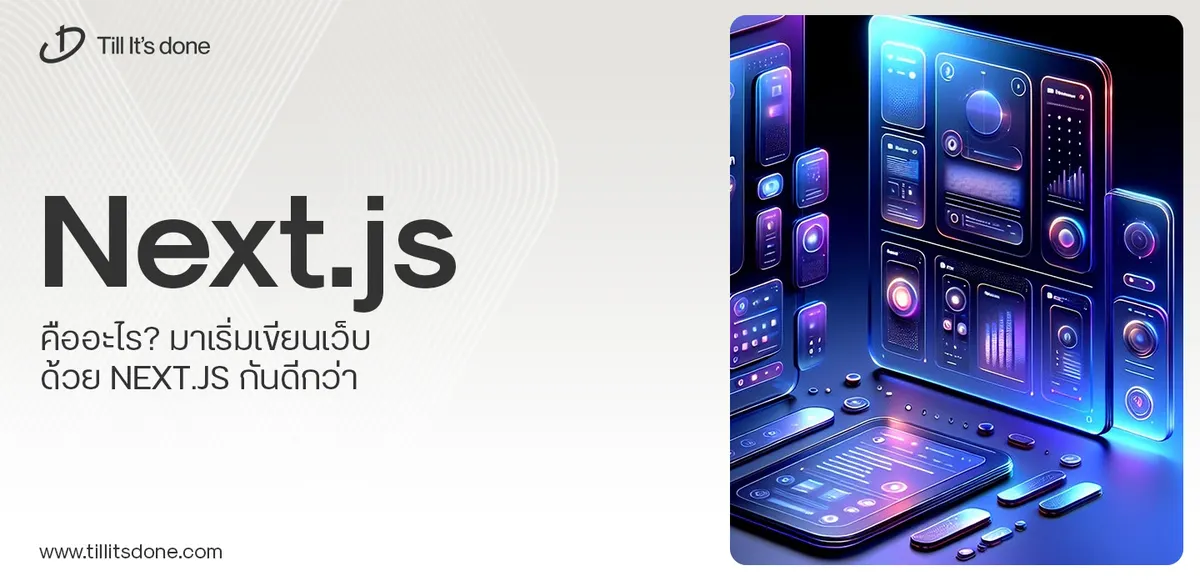
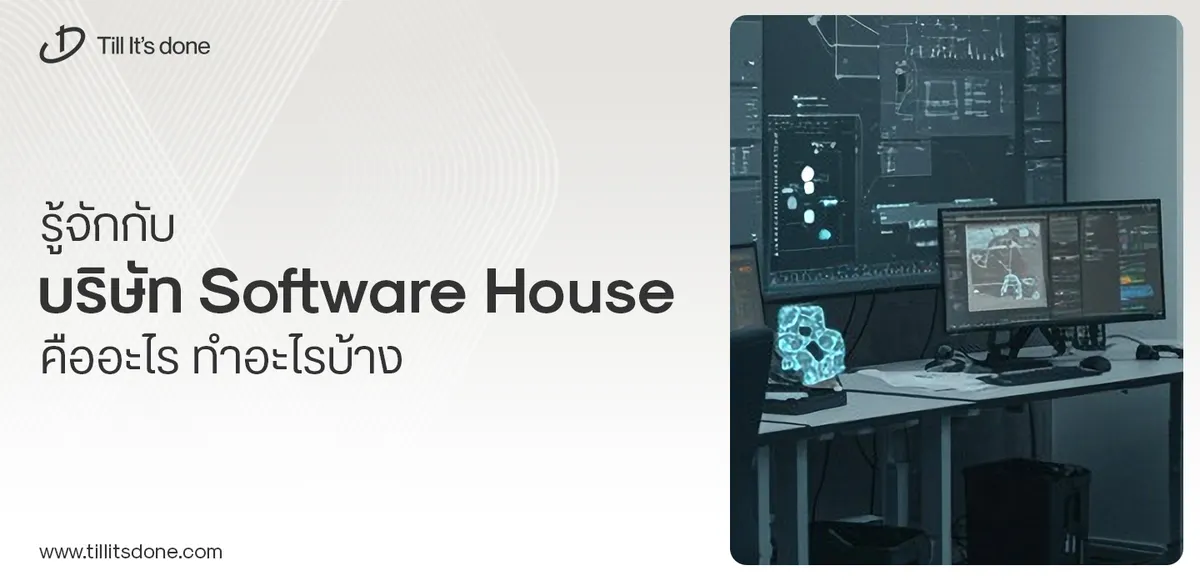
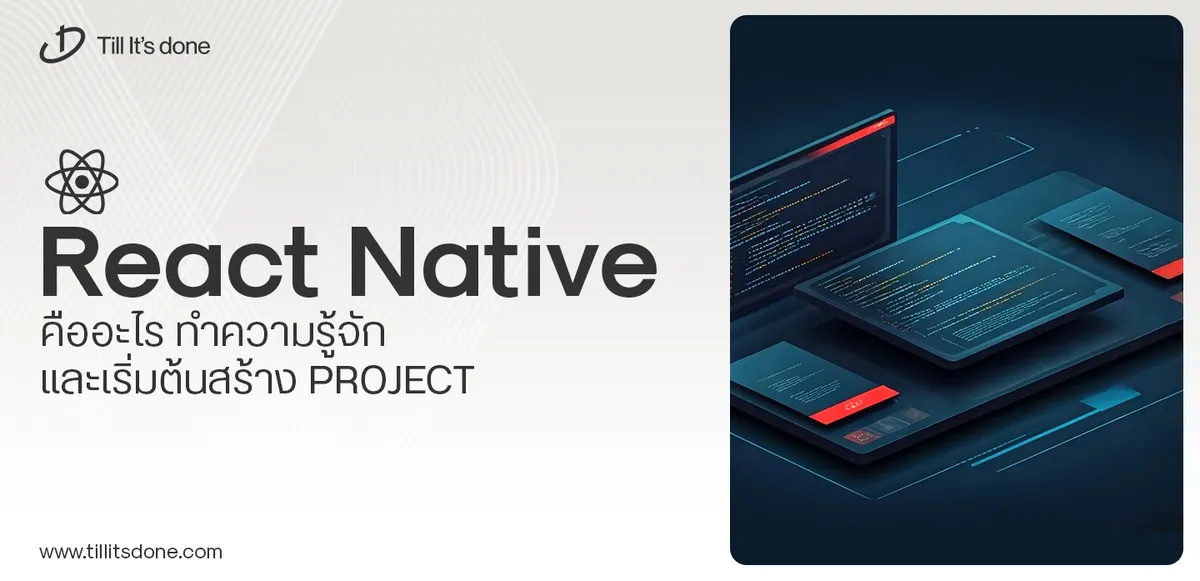
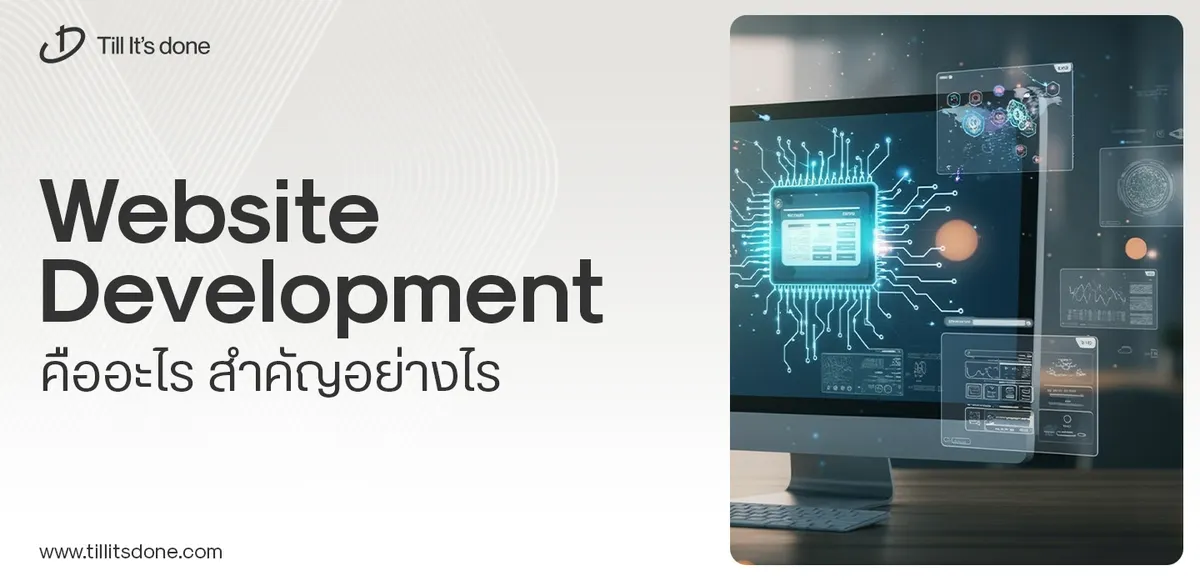
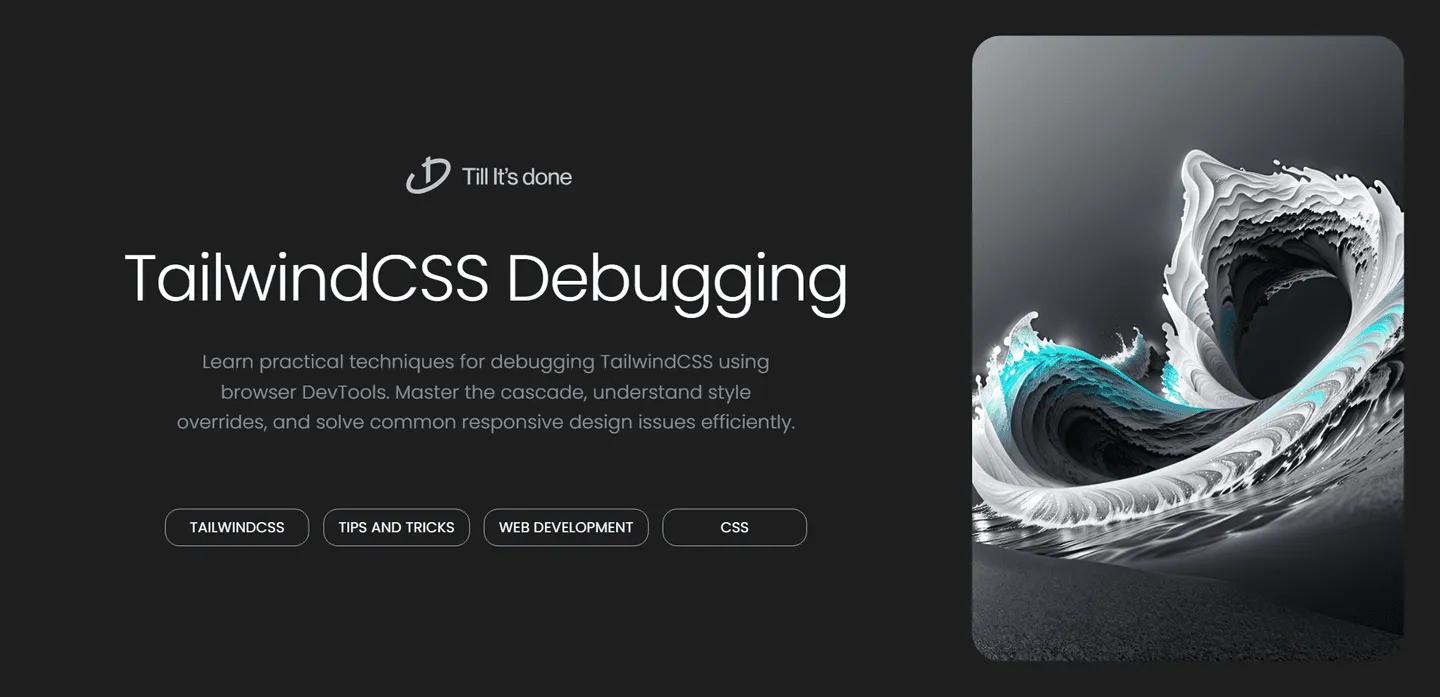
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.