- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Advanced Go: Working with Reflection & Interfaces
Learn practical examples, best practices, and performance considerations for implementing reflection in Go.

Advanced Go: Working with Reflection and Interfaces
Go’s reflection and interface system provides powerful tools for creating flexible and dynamic code. In this deep dive, we’ll explore advanced techniques for working with reflection and interfaces that can elevate your Go programming skills to the next level.
Understanding Go’s Reflection Package
At its core, reflection allows programs to examine, modify, and create variables, functions, and types at runtime. While it should be used judiciously, reflection opens up possibilities for creating more generic and adaptable code.
Let’s start with a practical example that demonstrates the power of reflection:
type Customer struct { Name string Age int Address string}
func inspectStruct(v interface{}) { val := reflect.ValueOf(v) typ := val.Type()
fmt.Printf("Type: %v\n", typ) for i := 0; i < val.NumField(); i++ { field := typ.Field(i) value := val.Field(i) fmt.Printf("Field: %s\tType: %v\tValue: %v\n", field.Name, field.Type, value) }}
Working with Interface Types
Interfaces in Go provide a powerful way to define behavior and create abstraction. When combined with reflection, they become even more versatile. Here’s how we can use reflection to work with interfaces dynamically:
type Processor interface { Process(data string) string}
func callProcess(v interface{}) { // Check if v implements Processor typ := reflect.TypeOf((*Processor)(nil)).Elem() val := reflect.ValueOf(v)
if val.Type().Implements(typ) { result := val.MethodByName("Process").Call([]reflect.Value{ reflect.ValueOf("test data"), }) fmt.Println("Process result:", result[0].String()) }}
Advanced Reflection Patterns
One powerful application of reflection is creating generic data validators or mappers. Here’s an example of a function that can validate struct fields based on tags:
type User struct { Name string `validate:"required,min=3"` Email string `validate:"required,email"` Age int `validate:"required,min=18"`}
func validateStruct(v interface{}) []string { var errors []string val := reflect.ValueOf(v) typ := val.Type()
for i := 0; i < val.NumField(); i++ { field := typ.Field(i) value := val.Field(i)
if validateTag := field.Tag.Get("validate"); validateTag != "" { rules := strings.Split(validateTag, ",") for _, rule := range rules { if err := validateField(field.Name, value, rule); err != nil { errors = append(errors, err.Error()) } } } } return errors}
Performance Considerations
While reflection is powerful, it comes with performance overhead. Here are some tips for using reflection efficiently:
- Cache reflect.Type information when possible
- Avoid reflection in hot paths
- Use interface assertions when type information is known
- Consider code generation alternatives for critical paths
Best Practices and Common Pitfalls
When working with reflection and interfaces, keep these guidelines in mind:
- Use reflection only when simpler alternatives don’t exist
- Always validate interface implementations at runtime
- Handle nil pointer cases carefully
- Test edge cases thoroughly
- Document why reflection is necessary
Remember that while reflection is powerful, it should be used sparingly and thoughtfully in your Go code.



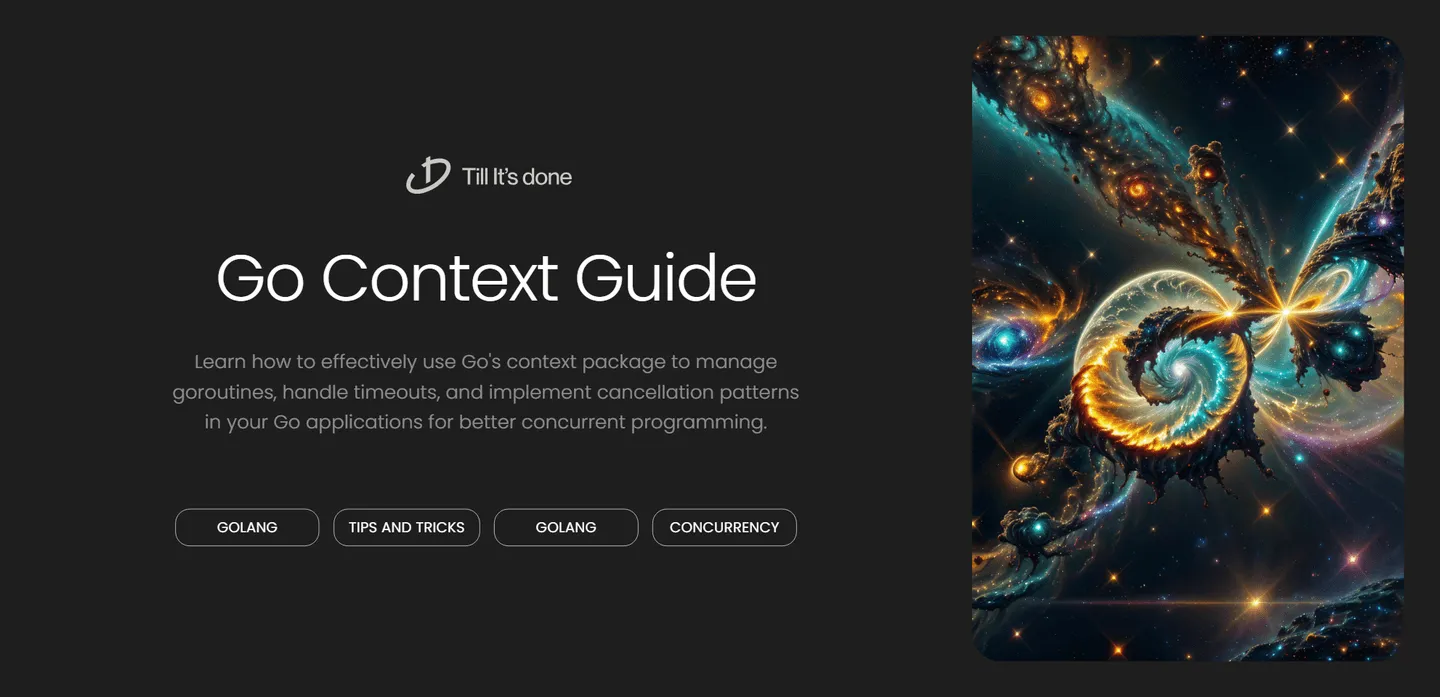


Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.