- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using GoMock with Concurrent Code Guide
Discover how to handle race conditions, timeouts, and error scenarios with real-world examples and best practices.
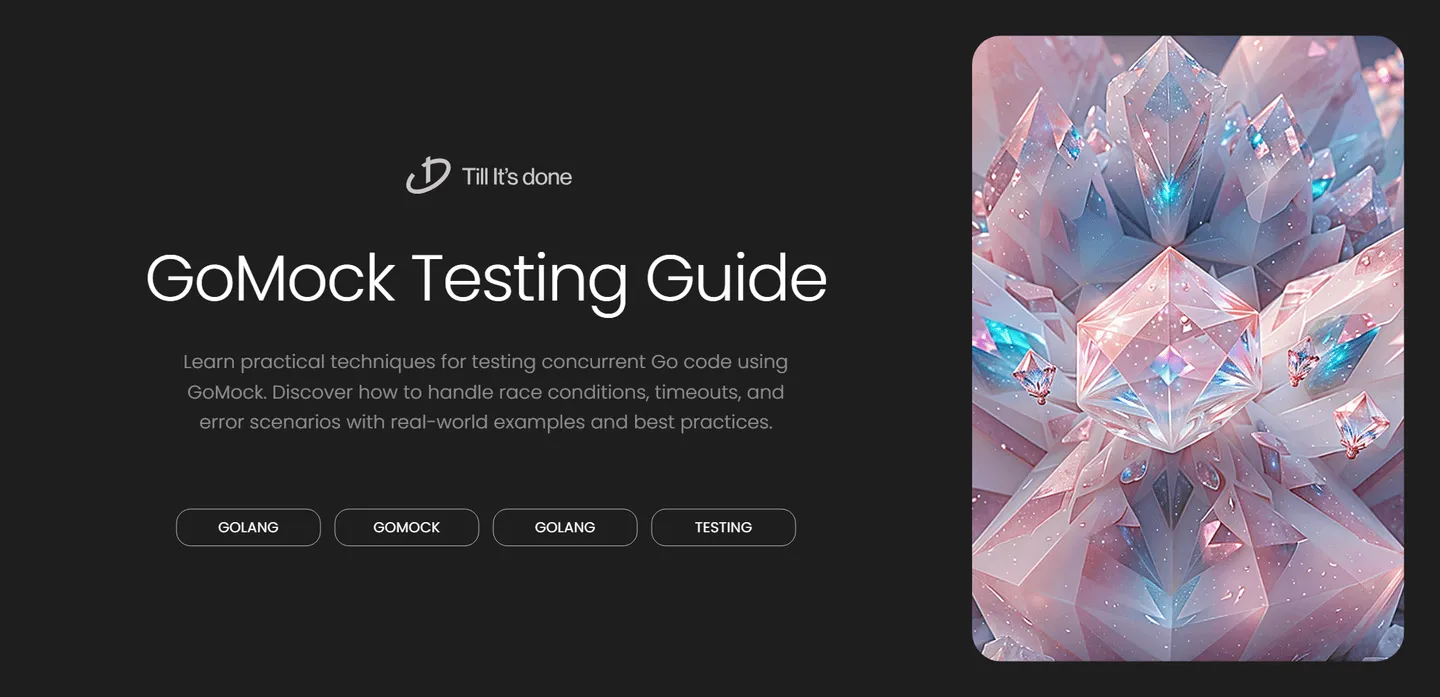
Using GoMock with Concurrent Code: Techniques and Examples
Testing concurrent code can be tricky, but with GoMock, we can make it manageable and reliable. In this post, we’ll explore practical techniques for testing concurrent Go code using GoMock, with real-world examples that you can apply to your projects.
Understanding the Challenge
When working with concurrent code, we often face challenges like race conditions, deadlocks, and timing issues. These challenges become even more complex when we try to test such code. GoMock helps us tackle these challenges by providing precise control over mock behaviors in concurrent scenarios.
Setting Up GoMock for Concurrent Testing
First, let’s set up a simple example. Imagine we have a service that processes user data concurrently:
type DataProcessor interface { ProcessUserData(userData string) (string, error)}
type UserService struct { processor DataProcessor}
func (s *UserService) ProcessMultipleUsers(users []string) []string { results := make([]string, len(users)) var wg sync.WaitGroup
for i, user := range users { wg.Add(1) go func(index int, userData string) { defer wg.Done() result, _ := s.processor.ProcessUserData(userData) results[index] = result }(i, user) }
wg.Wait() return results}
Mocking Concurrent Calls
Here’s where GoMock shines. We can use it to verify concurrent calls and control their behavior:
func TestProcessMultipleUsers(t *testing.T) { ctrl := gomock.NewController(t) defer ctrl.Finish()
mockProcessor := NewMockDataProcessor(ctrl)
// Handle concurrent calls mockProcessor.EXPECT(). ProcessUserData(gomock.Any()). Times(3). // Expect 3 concurrent calls DoAndReturn(func(userData string) (string, error) { time.Sleep(10 * time.Millisecond) // Simulate work return "processed_" + userData, nil })
service := &UserService{processor: mockProcessor} results := service.ProcessMultipleUsers([]string{"user1", "user2", "user3"})
// Verify results for i, result := range results { if !strings.HasPrefix(result, "processed_user") { t.Errorf("Unexpected result for index %d: %s", i, result) } }}
Advanced Techniques
1. Testing Timeouts
We can test timeout scenarios by controlling mock response times:
mockProcessor.EXPECT(). ProcessUserData(gomock.Any()). DoAndReturn(func(userData string) (string, error) { time.Sleep(2 * time.Second) // Simulate slow processing return "", errors.New("timeout") })
2. Testing Race Conditions
GoMock helps us test race conditions by controlling the order of concurrent operations:
var mu sync.Mutexvar callOrder []string
mockProcessor.EXPECT(). ProcessUserData(gomock.Any()). AnyTimes(). DoAndReturn(func(userData string) (string, error) { mu.Lock() callOrder = append(callOrder, userData) mu.Unlock() return "processed", nil })
3. Testing Error Scenarios
We can simulate various error scenarios in concurrent code:
mockProcessor.EXPECT(). ProcessUserData(gomock.Any()). AnyTimes(). Return("", errors.New("simulated error"))
Best Practices
- Always use
gomock.NewController(t)
with proper cleanup - Be careful with
.Times()
expectations in concurrent code - Use
DoAndReturn
for complex mock behaviors - Don’t forget to handle timeouts in your tests
- Consider using channels for synchronization in tests
Conclusion
Testing concurrent code doesn’t have to be intimidating. With GoMock, we can create reliable tests that verify our concurrent code behaves correctly under various conditions. Remember to always consider race conditions, timeouts, and error scenarios in your tests.
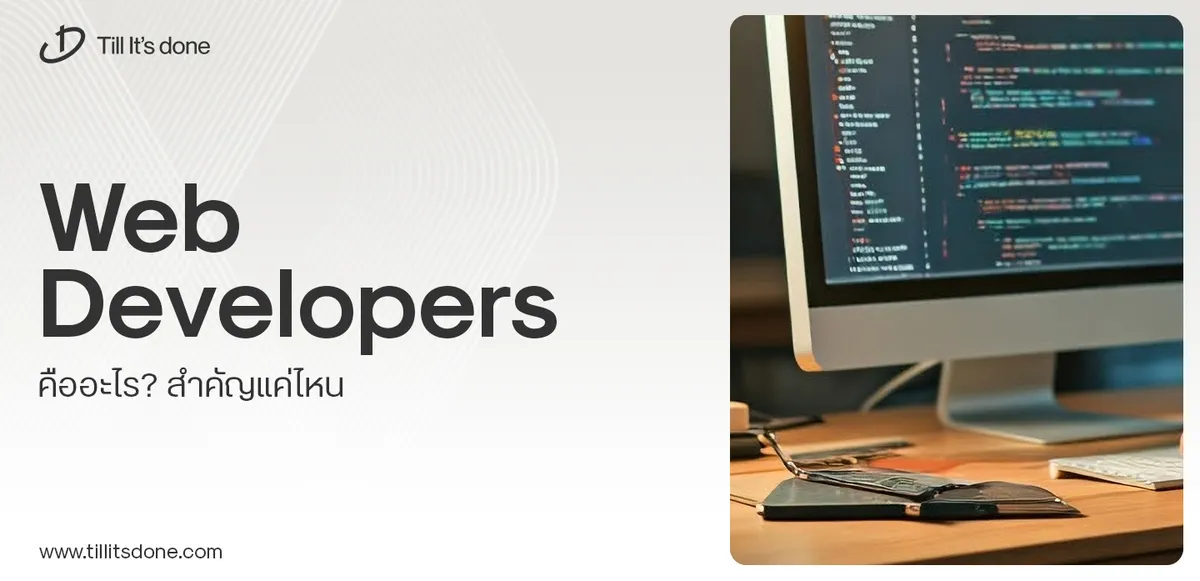
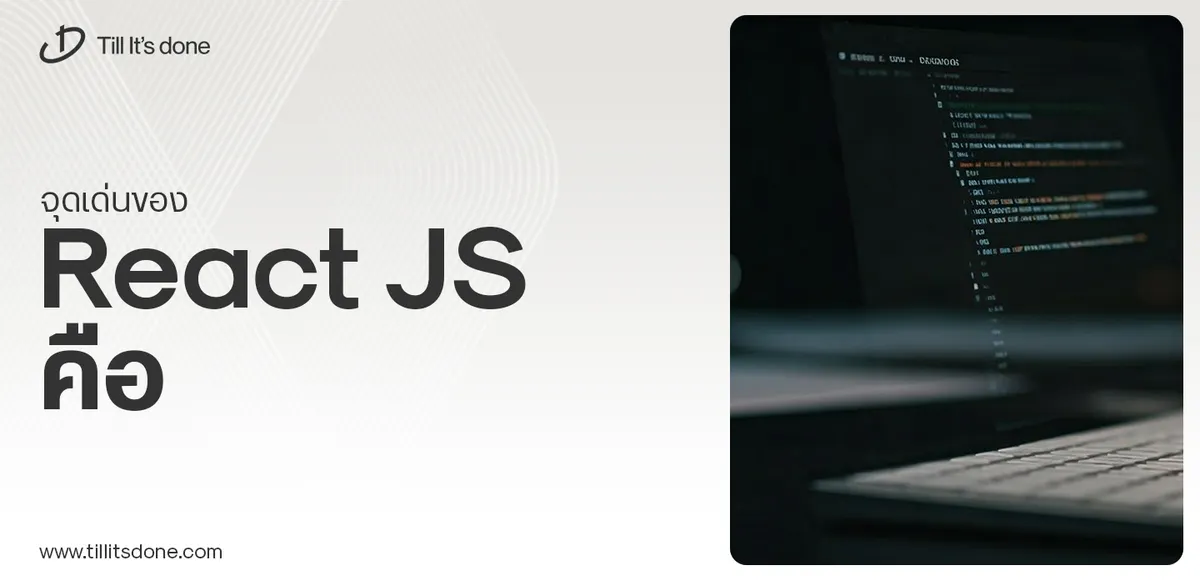
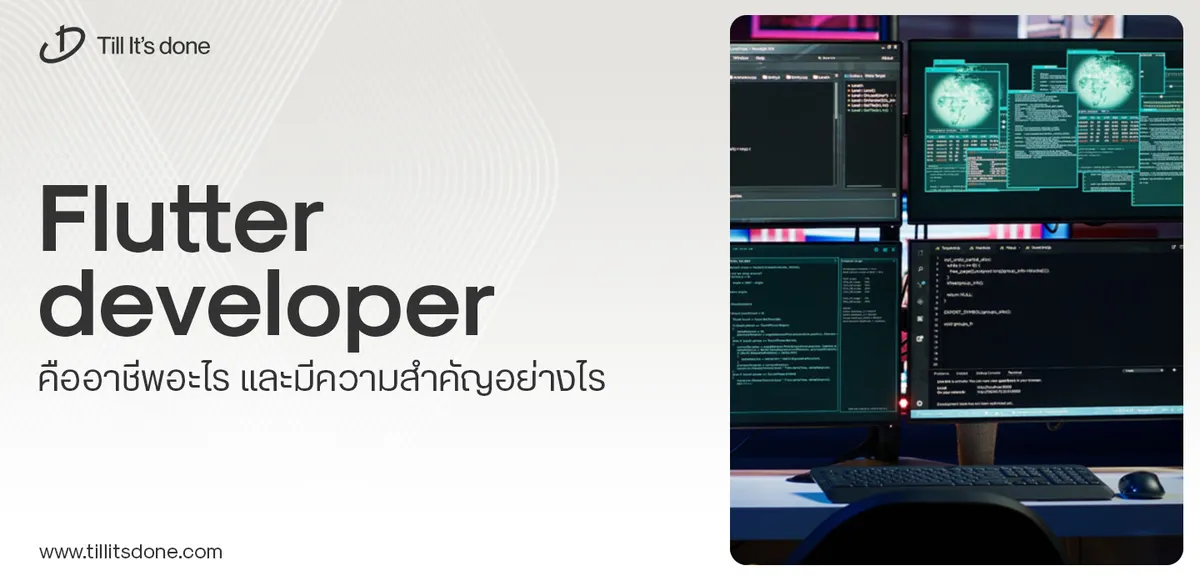
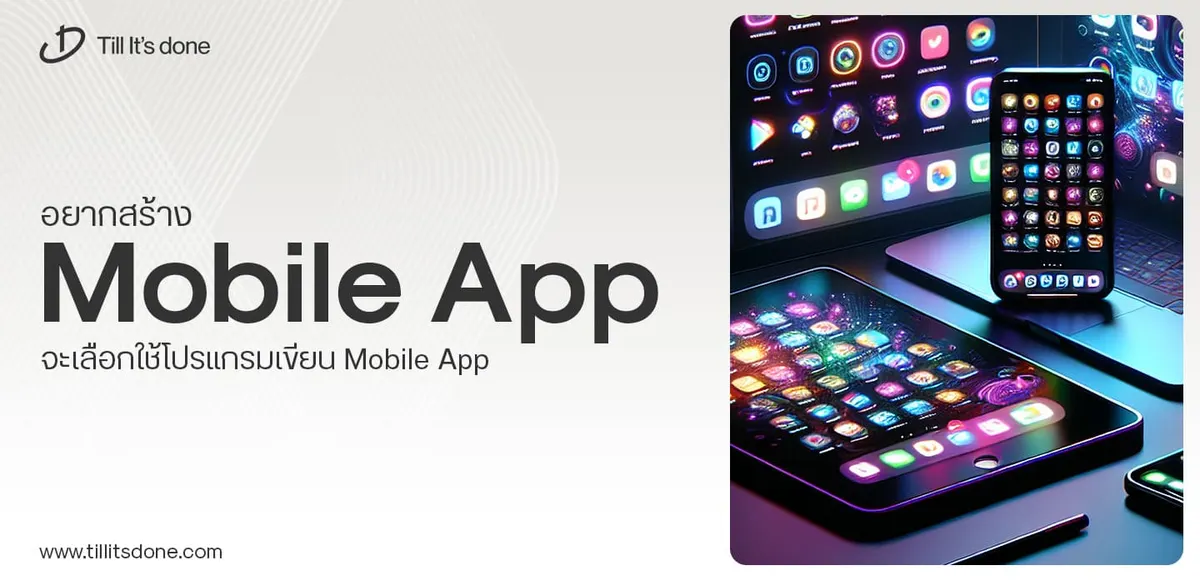
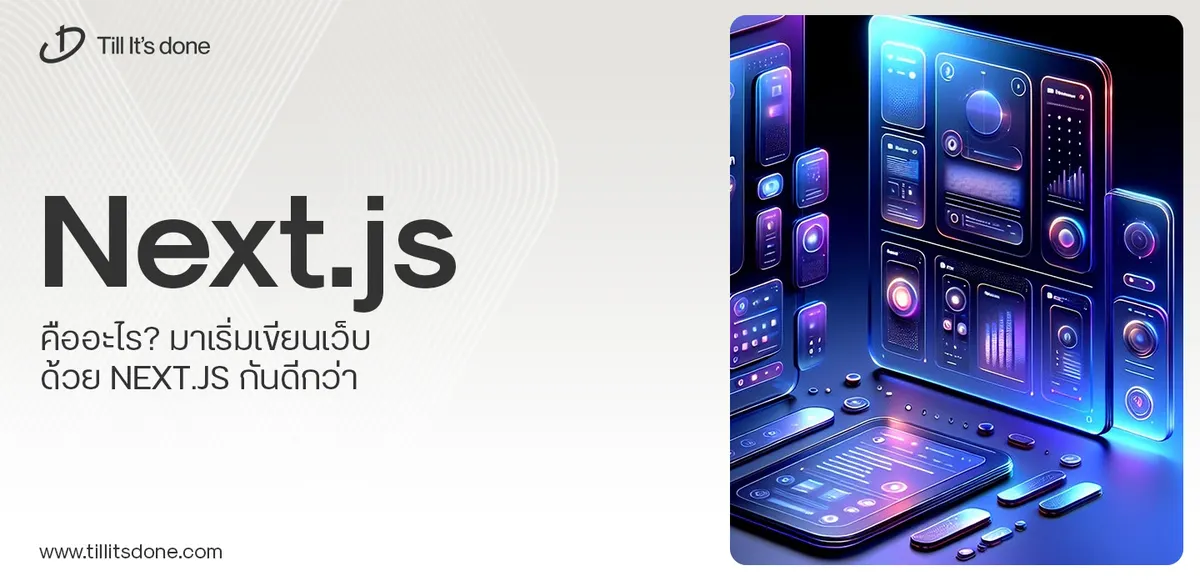
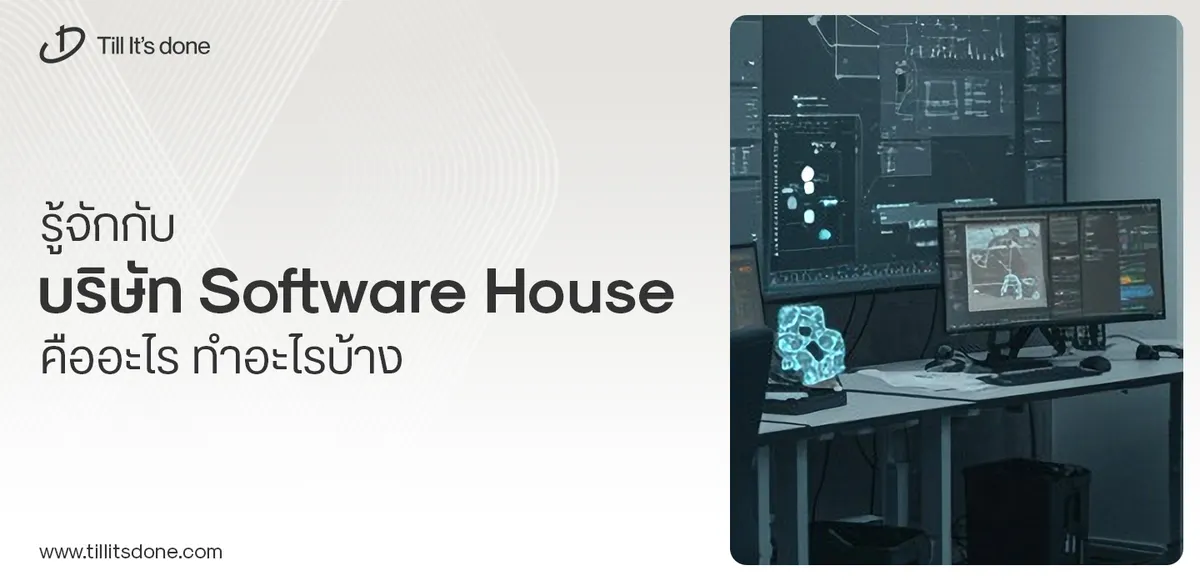
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.