- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Introduction to GoMock: Guide to Mocking in Go
This beginner-friendly guide covers installation, mock generation, test writing, and best practices for effective testing.
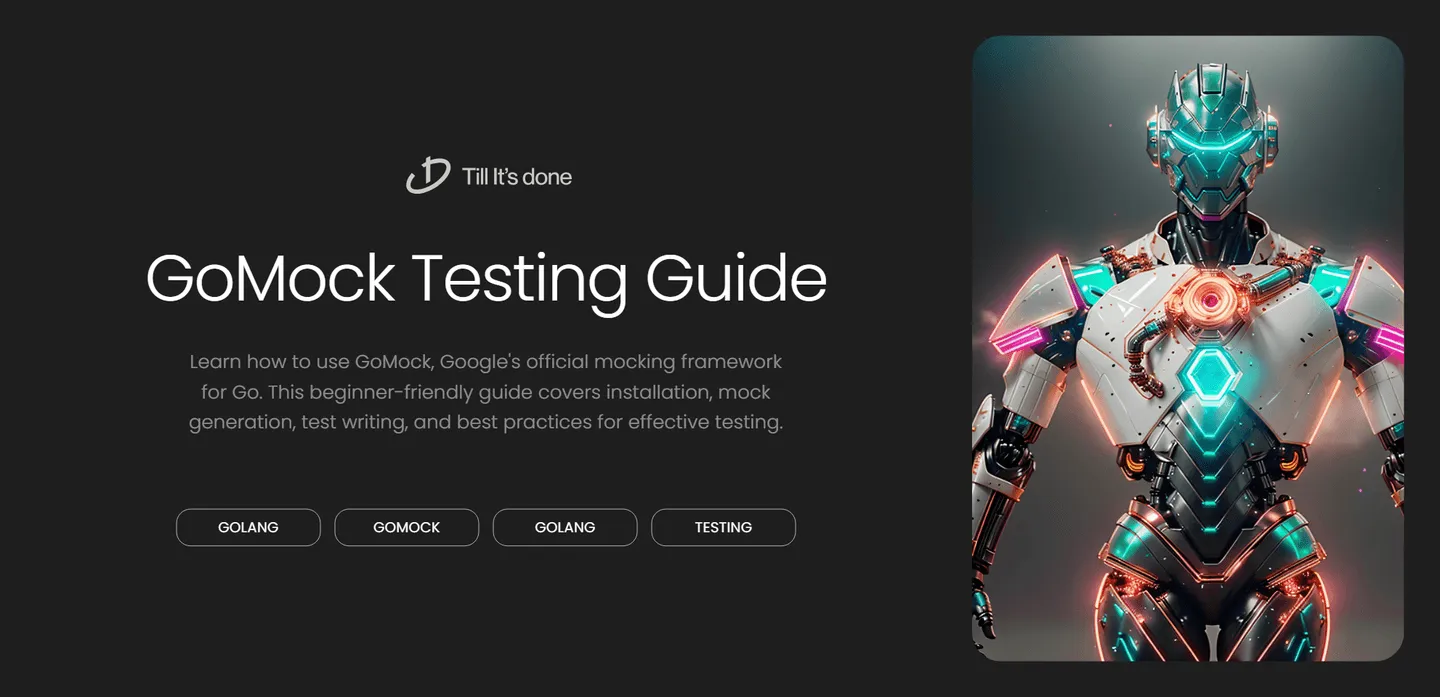
Introduction to GoMock: A Beginner’s Guide to Mocking in Go
Testing is a crucial part of software development, and in Go, it’s no different. When writing unit tests, we often need to isolate the component we’re testing from its dependencies. This is where mocking comes into play, and GoMock is one of the most powerful mocking frameworks available for Go developers.
What is GoMock?
GoMock is an official mocking framework developed by Google for the Go programming language. It provides a flexible way to create mock objects for interfaces, making it easier to write unit tests for your Go applications. By using GoMock, you can simulate the behavior of complex dependencies and focus on testing your actual code.
Getting Started with GoMock
Let’s start by setting up GoMock in your project. First, you’ll need to install two essential tools:
go install github.com/golang/mock/mockgen@v1.6.0
The mockgen
tool will help us generate mock implementations of our interfaces. Here’s a simple example to demonstrate how it works:
Let’s say we have a user service interface:
type UserService interface { GetUser(id string) (*User, error) CreateUser(user *User) error}
Generating and Using Mocks
One of the best features of GoMock is its ability to generate mock implementations automatically. After defining your interface, you can generate a mock using the mockgen
command:
mockgen -source=user_service.go -destination=mocks/mock_user_service.go
Writing Tests with GoMock
Now, let’s look at how to use the generated mock in your tests:
func TestUserHandler(t *testing.T) { ctrl := gomock.NewController(t) defer ctrl.Finish()
mockService := mocks.NewMockUserService(ctrl)
// Set up expectations mockService.EXPECT(). GetUser("123"). Return(&User{ID: "123", Name: "John"}, nil)
handler := NewUserHandler(mockService) user, err := handler.GetUserById("123")
assert.NoError(t, err) assert.Equal(t, "John", user.Name)}
Best Practices and Tips
- Always use
defer ctrl.Finish()
to ensure proper cleanup - Keep your mocks focused and specific to each test case
- Use the
gomock.Any()
matcher when the exact input doesn’t matter - Consider using
.Times(n)
to specify how many times a method should be called - Take advantage of GoMock’s ordering capabilities with
.After()
and.Before()
Conclusion
GoMock is a powerful tool that makes testing Go applications more manageable and reliable. By following the practices outlined in this guide, you’ll be well on your way to writing better tests and more maintainable code.
Remember, effective mocking is about finding the right balance between thorough testing and maintainable test code. GoMock provides the tools you need to achieve this balance in your Go projects.
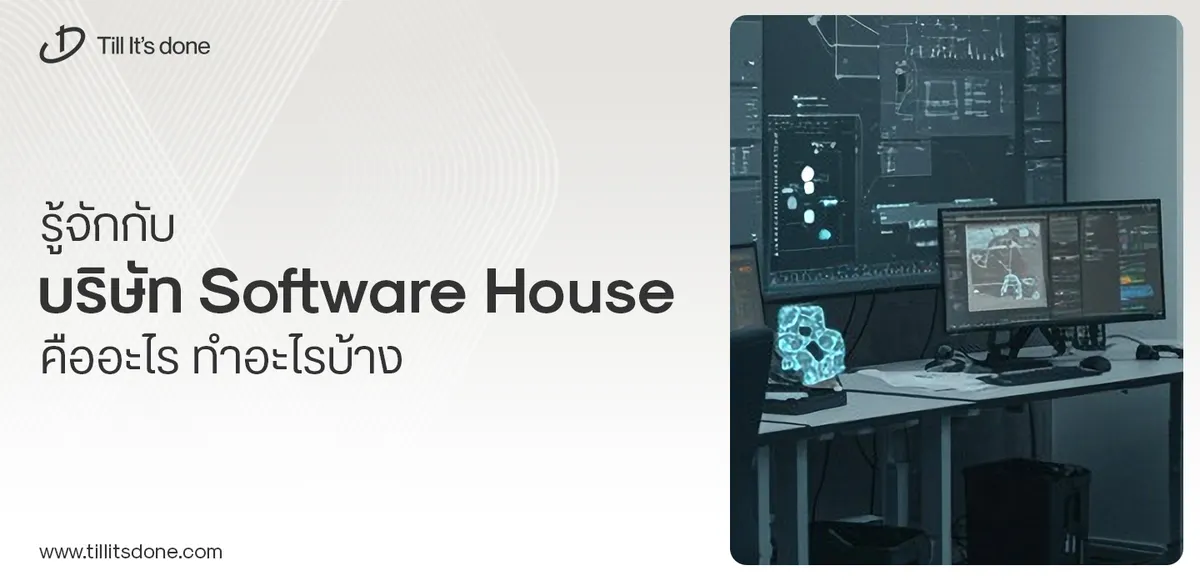
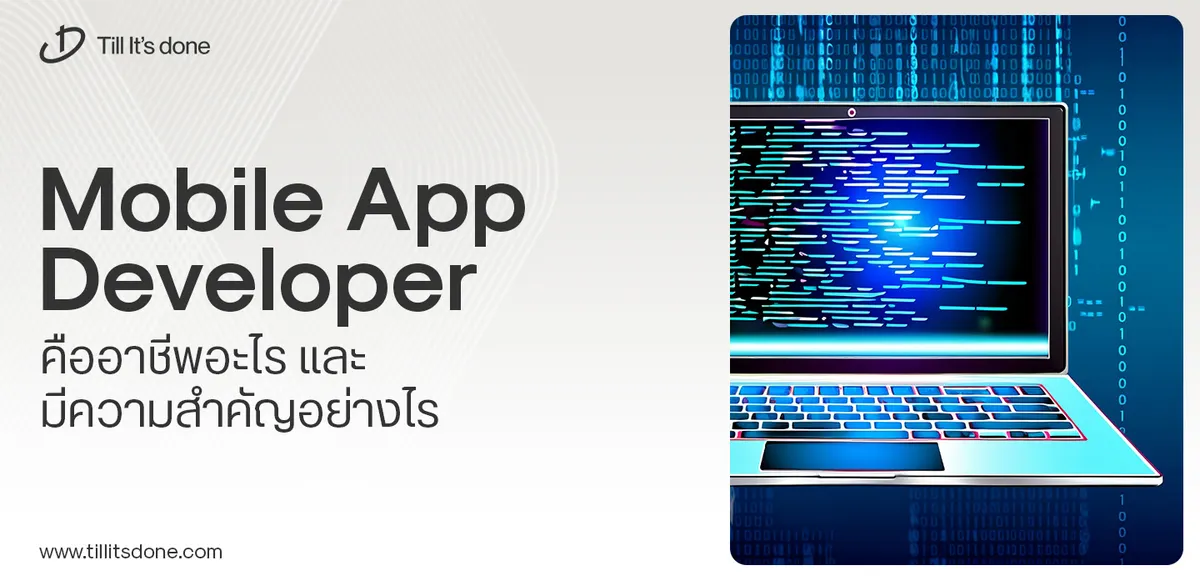
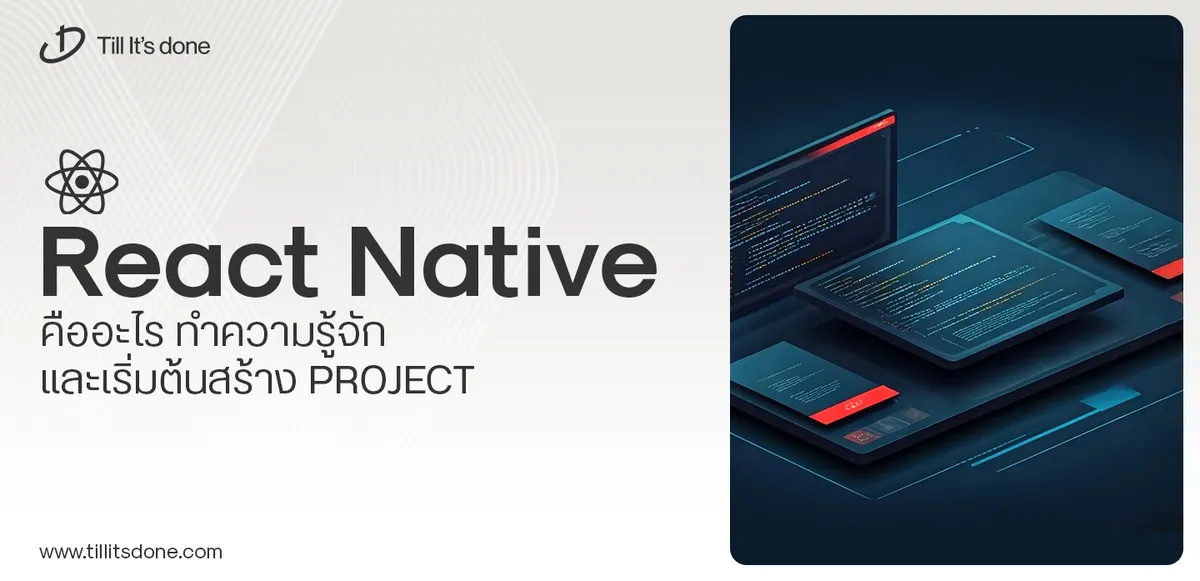
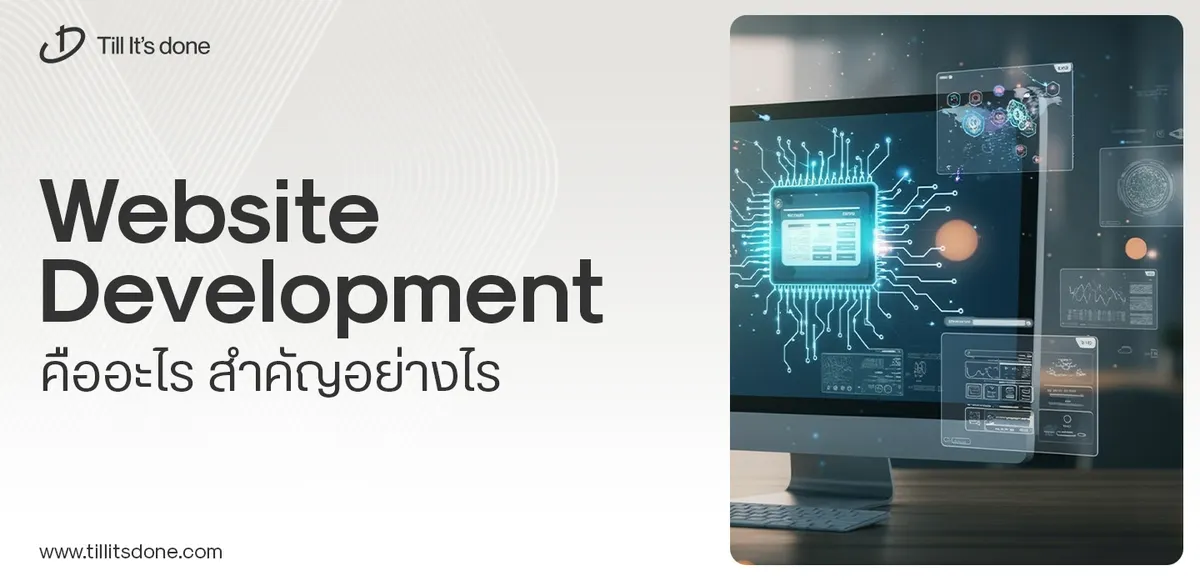
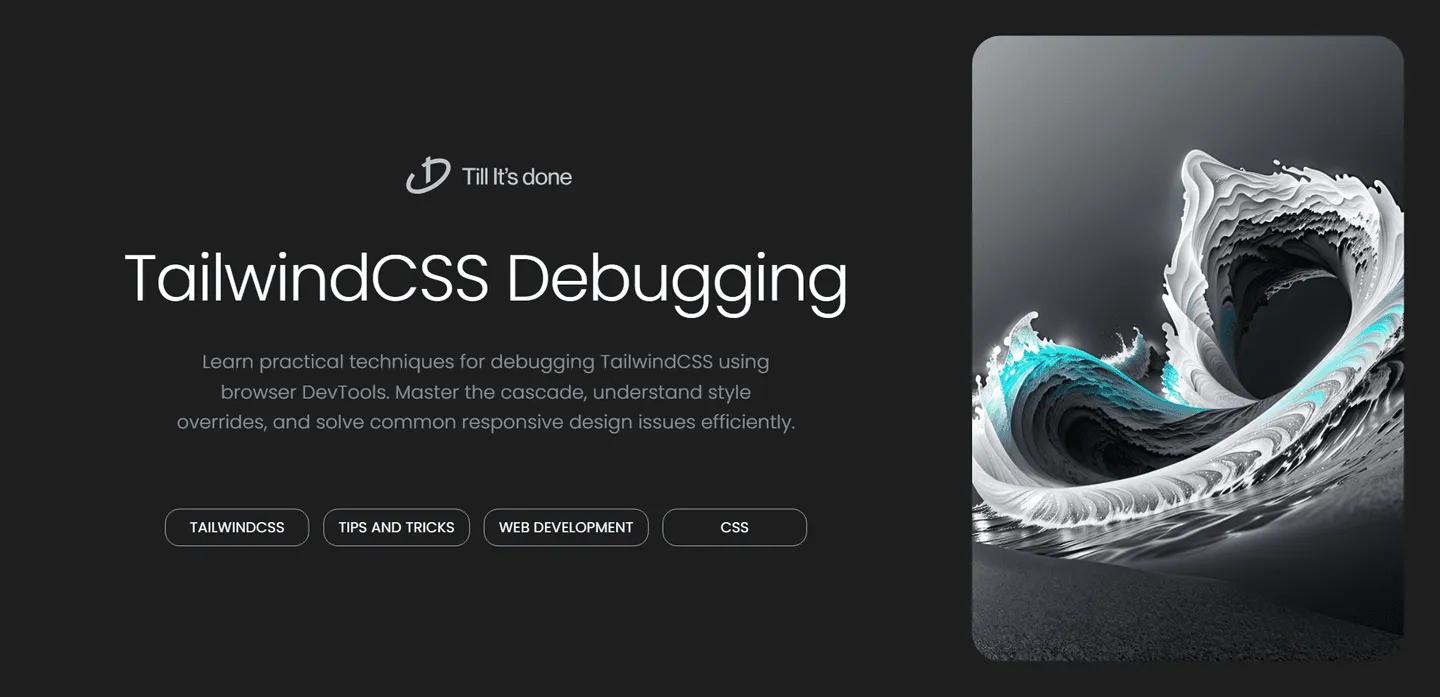
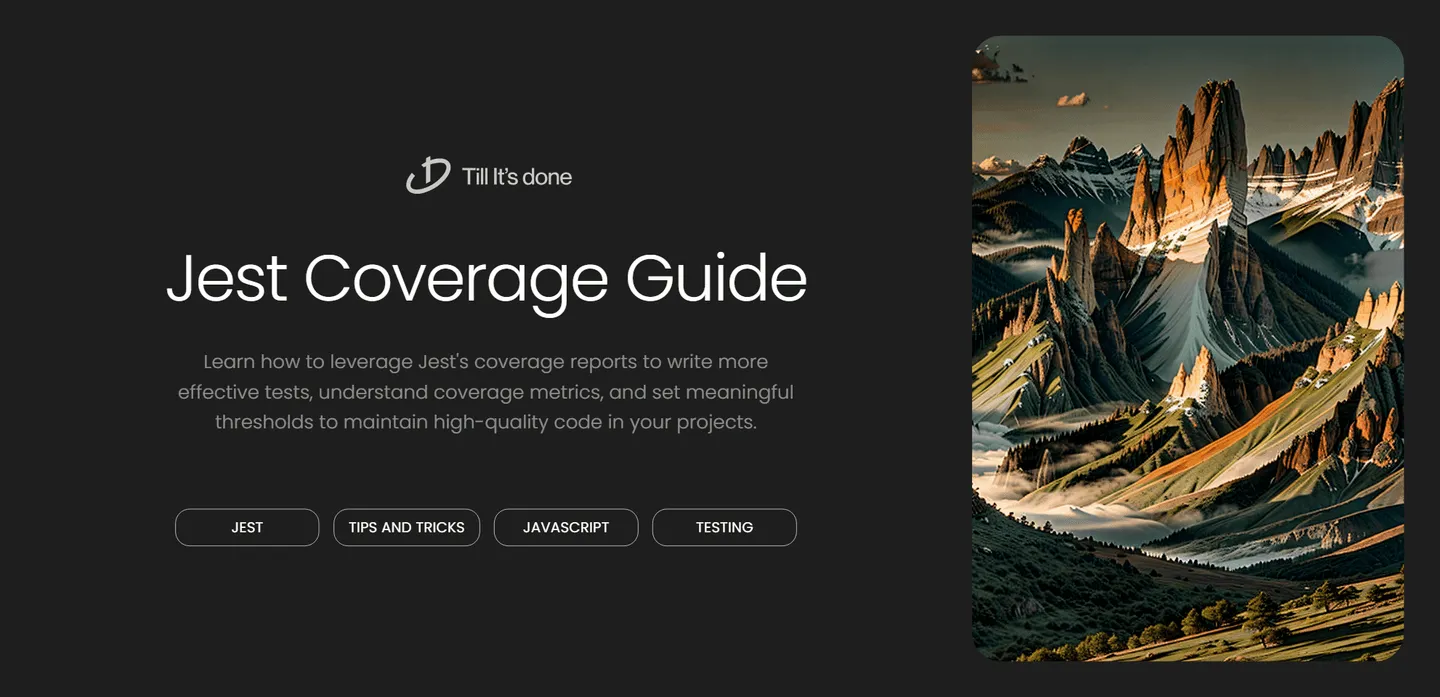
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.