- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Creating Custom Matchers in GoMock for Testing
This guide covers basic and advanced matcher patterns with practical examples.

Creating Custom Matchers in GoMock for Enhanced Flexibility
Testing is a crucial aspect of software development, and when it comes to Go testing, GoMock stands out as a powerful mocking framework. While GoMock provides several built-in matchers, there are situations where you need more specific matching logic. Let’s dive into creating custom matchers in GoMock to make your tests more flexible and expressive.
Understanding Custom Matchers
Custom matchers in GoMock allow you to define your own matching logic for method arguments. This becomes particularly useful when the standard matchers don’t quite fit your needs or when you want to make your tests more readable and maintainable.
Creating Your First Custom Matcher
Let’s say we’re working with a payment processing system, and we need to verify that a payment amount falls within a specific range. Instead of using multiple comparisons, we can create a custom matcher:
func IsBetween(min, max float64) gomock.Matcher { return &betweenMatcher{min, max}}
type betweenMatcher struct { min, max float64}
func (m *betweenMatcher) Matches(x interface{}) bool { amount, ok := x.(float64) if !ok { return false } return amount >= m.min && amount <= m.max}
func (m *betweenMatcher) String() string { return fmt.Sprintf("is between %v and %v", m.min, m.max)}
Practical Application
Here’s how you might use this custom matcher in your tests:
func TestPaymentProcessor(t *testing.T) { ctrl := gomock.NewController(t) defer ctrl.Finish()
mockProcessor := NewMockPaymentProcessor(ctrl) mockProcessor.EXPECT(). ProcessPayment(IsBetween(100.0, 1000.0)). Return(nil)
// Test implementation}
Advanced Custom Matcher Patterns
Sometimes you might need more complex matching logic. For example, let’s create a matcher that validates a transaction object’s properties:
type TransactionMatcher struct { expectedFields map[string]interface{}}
func NewTransactionMatcher() *TransactionMatcher { return &TransactionMatcher{ expectedFields: make(map[string]interface{}), }}
func (m *TransactionMatcher) WithAmount(amount float64) *TransactionMatcher { m.expectedFields["amount"] = amount return m}
func (m *TransactionMatcher) WithCurrency(currency string) *TransactionMatcher { m.expectedFields["currency"] = currency return m}
func (m *TransactionMatcher) Matches(x interface{}) bool { tx, ok := x.(*Transaction) if !ok { return false }
// Implement matching logic return tx.Amount == m.expectedFields["amount"].(float64) && tx.Currency == m.expectedFields["currency"].(string)}
func (m *TransactionMatcher) String() string { return fmt.Sprintf("matches transaction with %v", m.expectedFields)}
This approach provides a fluent interface for creating matchers and makes your tests more readable and maintainable.
Best Practices
- Keep matchers focused and single-purpose
- Provide clear error messages in the String() method
- Consider making matchers reusable across different test suites
- Document your custom matchers well
- Include error handling for type assertions
Custom matchers are a powerful tool in your testing arsenal. They help you write more expressive tests while keeping your code clean and maintainable. Don’t hesitate to create custom matchers when built-in ones don’t quite fit your needs.


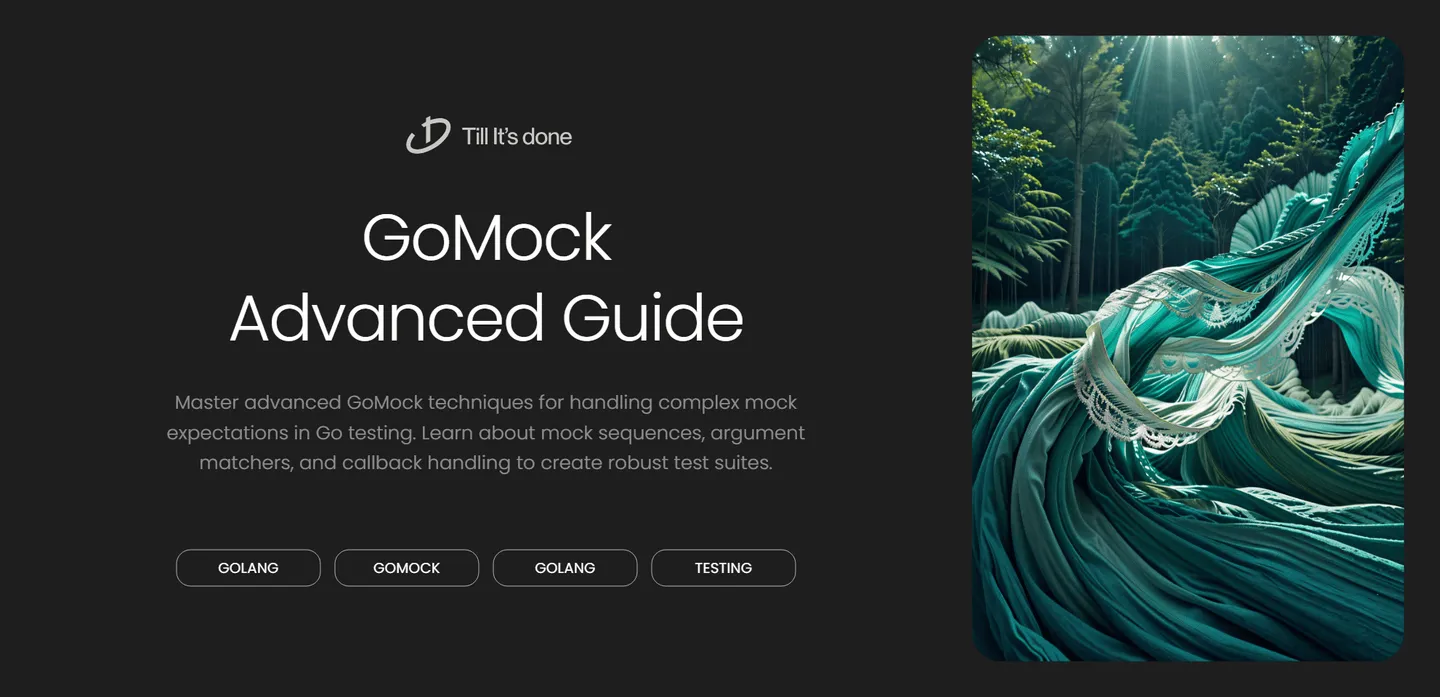

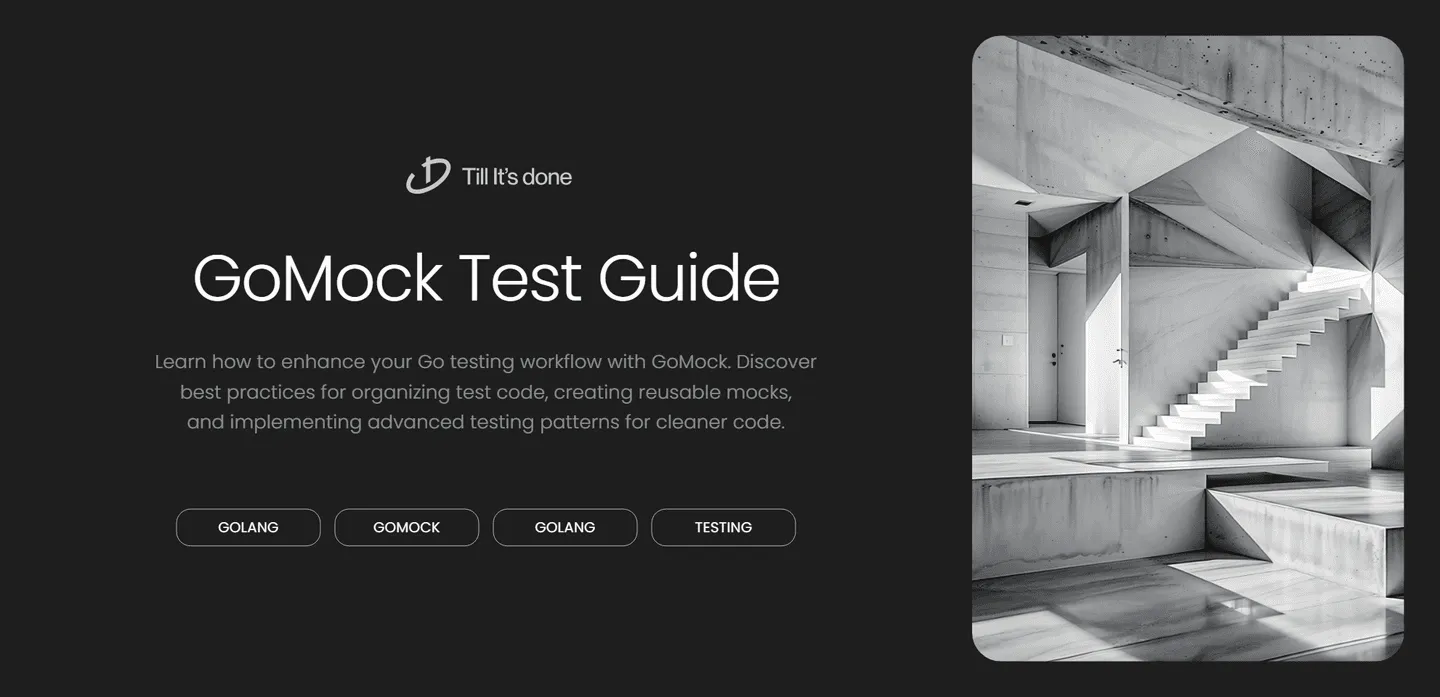

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.