- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Setting Up GoMock in Your Golang Project
Discover installation steps, writing effective tests, and best practices for mock implementation.

Setting Up GoMock in Your Golang Project: Step-by-Step Guide
Testing is a crucial part of software development, and mocking is an essential technique for writing effective unit tests. Today, let’s explore how to set up GoMock in your Golang project to create more reliable and maintainable tests.
What is GoMock?
GoMock is a powerful mocking framework for Go that helps you create mock objects for your interfaces. It’s particularly useful when you need to test components that depend on external services or complex implementations.
Prerequisites
Before we dive in, make sure you have:
- Go installed on your system
- A Go project initialized with go modules
- Basic understanding of Go testing
Installation Steps
First, we need to install two essential components:
# Install gomock librarygo get github.com/golang/mock/gomock
# Install mockgen toolgo install github.com/golang/mock/mockgen@latest
Creating Your First Mock
Let’s say we have a simple interface for a user service:
package service
type UserService interface { GetUser(id string) (*User, error) CreateUser(user *User) error}
Now, let’s generate a mock for this interface:
mockgen -source=service/user_service.go -destination=mocks/mock_user_service.go
Writing Tests with GoMock
Here’s how to use your newly created mock in a test:
func TestUserHandler(t *testing.T) { // Create a new controller ctrl := gomock.NewController(t) defer ctrl.Finish()
// Create a mock instance mockService := mocks.NewMockUserService(ctrl)
// Set expectations mockService.EXPECT(). GetUser("123"). Return(&User{ID: "123", Name: "John"}, nil)
// Use the mock in your test handler := NewUserHandler(mockService) user, err := handler.HandleGetUser("123")
// Assert results assert.NoError(t, err) assert.Equal(t, "John", user.Name)}
Best Practices
-
Organization: Keep your mocks in a separate directory (e.g.,
mocks/
) to maintain clean project structure. -
Versioning: Include generated mocks in version control to ensure consistent testing across the team.
-
Minimal Mocking: Only mock what you need. Over-mocking can lead to brittle tests.
-
Clean Setup: Use
defer ctrl.Finish()
to ensure proper cleanup of mock controllers.
Common Pitfalls to Avoid
- Don’t forget to set expectations before using the mock
- Avoid setting unnecessary expectations that aren’t part of the test
- Remember to handle error cases in your mocks
- Don’t mock structs; only mock interfaces
Conclusion
Setting up GoMock might seem daunting at first, but it’s an invaluable tool for writing effective unit tests in Go. With proper setup and understanding, you can create more reliable and maintainable tests for your Go applications.



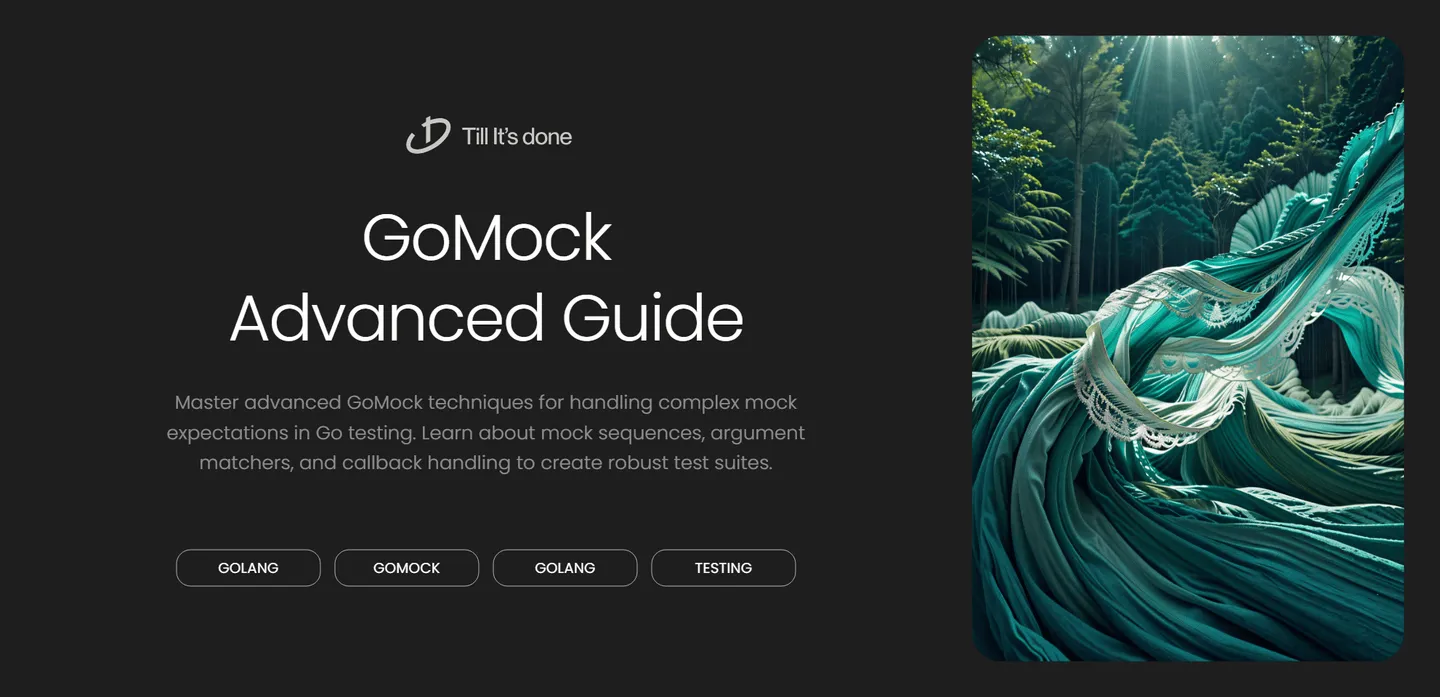


Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.