- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Improving Test Readability with GoMock
Discover best practices for organizing test code, creating reusable mocks, and implementing advanced testing patterns for cleaner code.
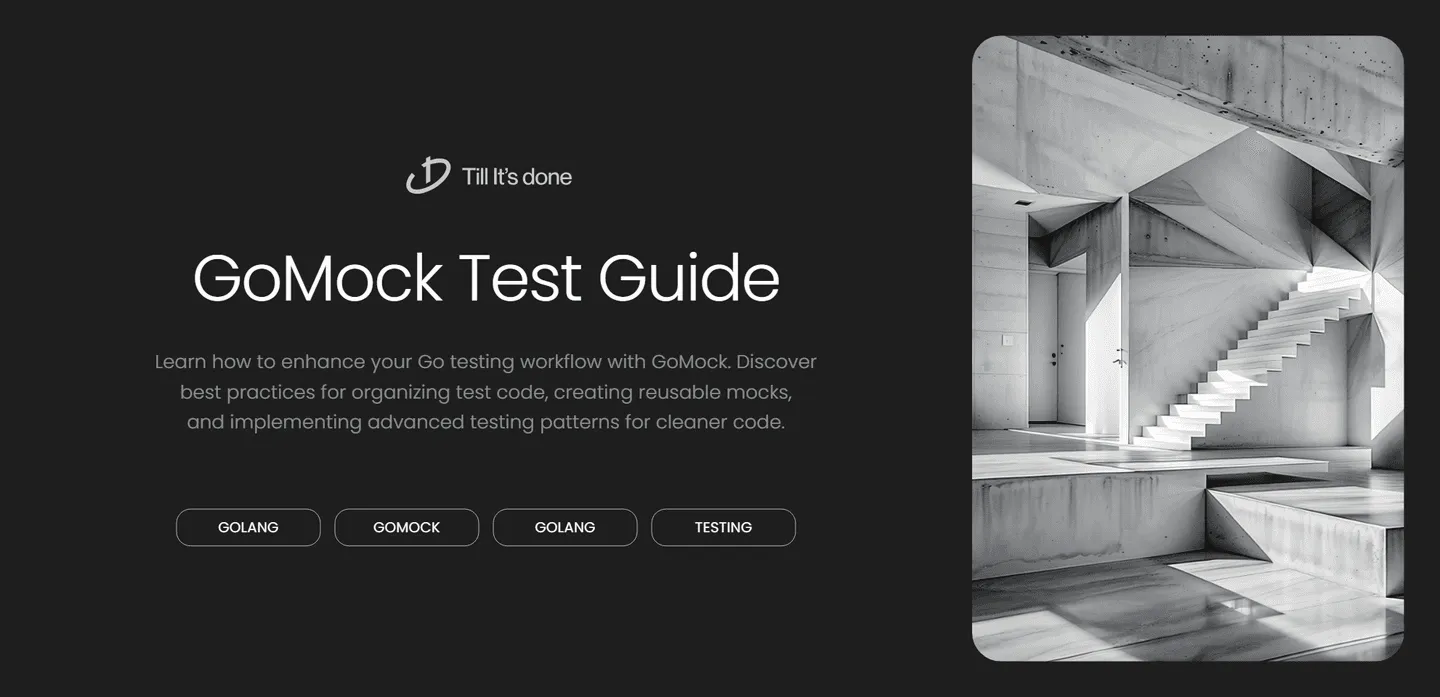
Improving Test Readability with GoMock and Code Organization
Writing clean and maintainable tests is just as important as writing good production code. As your Go projects grow larger, managing test dependencies and mocks becomes increasingly challenging. In this article, we’ll explore how to leverage GoMock effectively while maintaining clean and readable test code.
The Challenge with Test Organization
When working with larger Go applications, you’ll often find yourself dealing with complex interfaces and dependencies. Without proper organization, test files can quickly become cluttered with mock setup code, making them difficult to read and maintain.
Best Practices for Mock Organization
Centralized Mock Definitions
Instead of generating mocks in the same directory as your test files, consider creating a dedicated mocks
directory. This separation helps maintain a clean project structure and makes it easier to reuse mocks across different test files.
project/ ├── internal/ │ └── service/ │ ├── user_service.go │ └── user_service_test.go └── mocks/ └── mock_repositories.go
Creating Helper Functions
One effective way to improve test readability is by creating helper functions that encapsulate common mock setup patterns:
func setupUserRepositoryMock(ctrl *gomock.Controller) *mocks.MockUserRepository { mock := mocks.NewMockUserRepository(ctrl) return mock}
func TestUserService_GetUser(t *testing.T) { ctrl := gomock.NewController(t) defer ctrl.Finish()
mockRepo := setupUserRepositoryMock(ctrl) mockRepo.EXPECT(). GetByID(gomock.Any()). Return(&User{ID: "1", Name: "John"}, nil)
service := NewUserService(mockRepo) // Test implementation}
Advanced GoMock Techniques
Using Matcher Functions
GoMock provides powerful matching capabilities that can make your tests more flexible and readable. Instead of using exact values, you can create custom matchers:
func userMatcher(expected User) gomock.Matcher { return matcherFunc(func(x interface{}) bool { actual, ok := x.(User) if !ok { return false } return actual.ID == expected.ID })}
Mock Sequences
When testing complex workflows, you can use sequences to verify that methods are called in the correct order:
mockRepo.EXPECT(). BeginTransaction(). Return(nil). Times(1)
mockRepo.EXPECT(). SaveUser(gomock.Any()). Return(nil). Times(1)
mockRepo.EXPECT(). CommitTransaction(). Return(nil). Times(1)
Conclusion
By following these organization patterns and leveraging GoMock’s features effectively, you can create more maintainable and readable tests. Remember that well-organized tests serve as documentation for your code and make it easier for other developers to understand your system’s behavior.


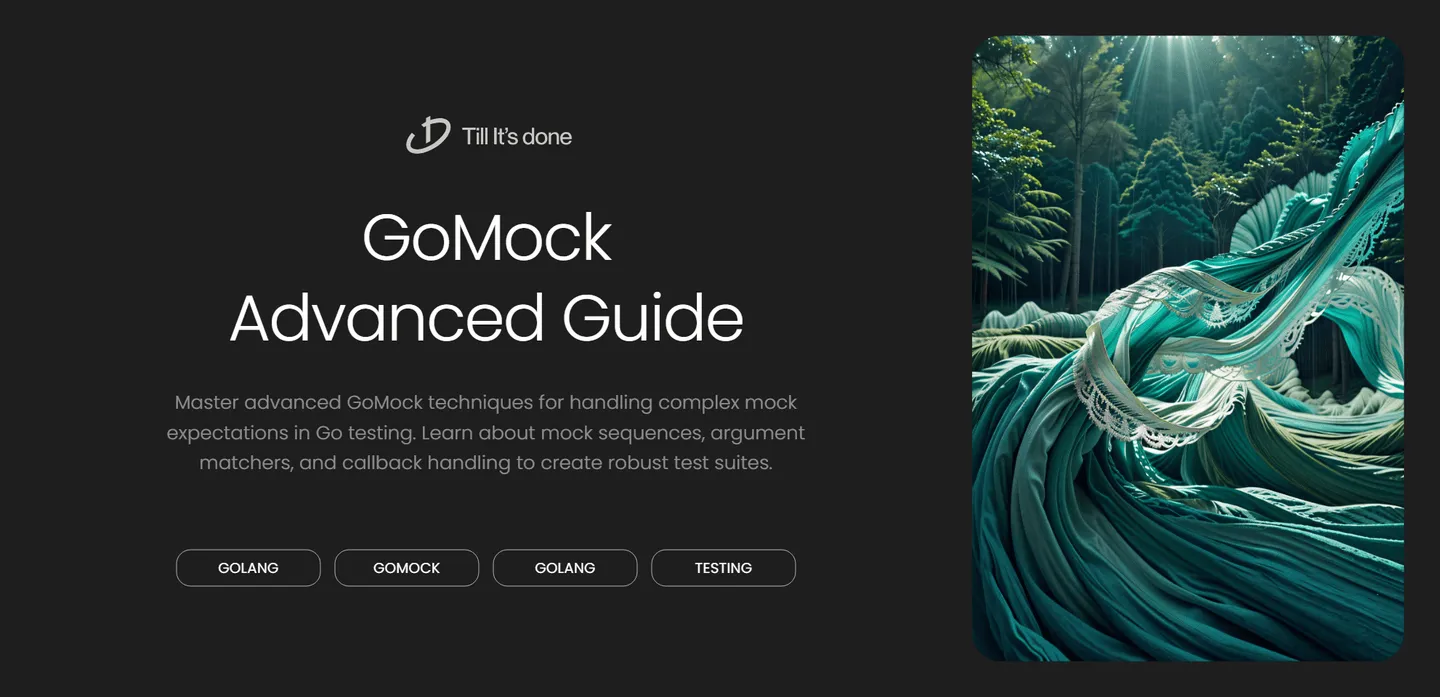



Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.