- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices for Using GoMock in Golang Testing
Discover how to write cleaner, more maintainable tests with practical examples and expert tips.

Best Practices for Using GoMock in Golang Testing
Testing is a crucial aspect of software development, and in the Go ecosystem, GoMock has emerged as a powerful mocking framework that helps developers write clean and effective tests. In this article, we’ll explore the best practices for using GoMock in your Golang projects to ensure your tests are maintainable, reliable, and efficient.
Understanding GoMock Fundamentals
Before diving into best practices, let’s quickly review what GoMock is. GoMock is a mocking framework that allows you to create mock objects for interfaces in your Go code. These mocks can simulate the behavior of real objects in a controlled way, making them perfect for unit testing.
Best Practices for GoMock Implementation
1. Keep Mock Definitions Close to Interfaces
Always keep your mock definitions in the same package as your interfaces. The conventional approach is to place them in a file named mock_<interface>_test.go
. This makes it easier to maintain and update mocks when interfaces change.
type UserService interface { GetUser(id string) (*User, error)}
// mock_user_service_test.go//go:generate mockgen -destination=mock_user_service_test.go -package=service UserService
2. Use Constructor Injection
Favor constructor injection to make your code more testable. This makes it easier to inject mocks during testing while using real implementations in production.
type UserHandler struct { userService UserService}
func NewUserHandler(us UserService) *UserHandler { return &UserHandler{userService: us}}
3. Leverage EXPECT() Wisely
Use EXPECT() calls judiciously and make them as specific as possible. Specify only what’s necessary for the test case to avoid brittle tests.
func TestUserHandler_GetUser(t *testing.T) { ctrl := gomock.NewController(t) defer ctrl.Finish()
mockService := NewMockUserService(ctrl) mockService.EXPECT(). GetUser("123"). Return(&User{ID: "123", Name: "John"}, nil). Times(1)}
4. Implement Meaningful Test Scenarios
Focus on testing behavior rather than implementation details. Write tests that verify the expected outcomes rather than the specific sequence of method calls.
func TestUserHandler_GetUser_NotFound(t *testing.T) { ctrl := gomock.NewController(t) defer ctrl.Finish()
mockService := NewMockUserService(ctrl) mockService.EXPECT(). GetUser(gomock.Any()). Return(nil, errors.New("user not found"))
handler := NewUserHandler(mockService) response := handler.HandleGetUser("999")
assert.Equal(t, http.StatusNotFound, response.StatusCode)}
5. Use Matchers Effectively
Take advantage of GoMock’s built-in matchers to make your tests more flexible and maintainable:
mockService.EXPECT(). CreateUser(gomock.Any()). DoAndReturn(func(user *User) error { if user.Age < 18 { return errors.New("user must be 18 or older") } return nil })
6. Clean Up After Tests
Always use defer ctrl.Finish()
after creating a new controller to ensure proper cleanup of mock objects and verification of expectations.
Common Pitfalls to Avoid
- Over-specifying expectations
- Creating mocks for simple structs
- Forgetting to verify mock calls
- Not using the
go:generate
command effectively
Conclusion
Using GoMock effectively can significantly improve your testing workflow in Go projects. By following these best practices, you can write more maintainable, reliable, and efficient tests that truly verify your code’s behavior.



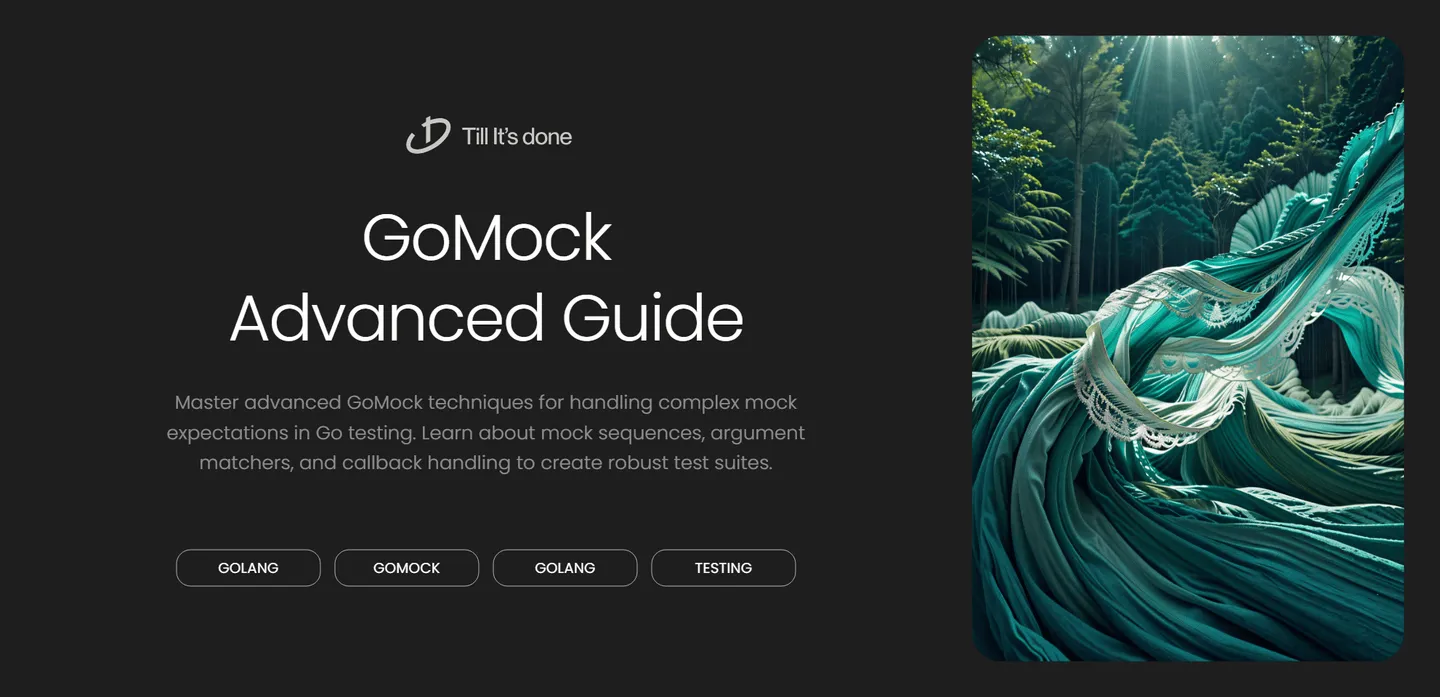


Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.