- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Unit Testing & TDD in Go: Best Practices
Learn how to write effective tests and implement TDD workflow.
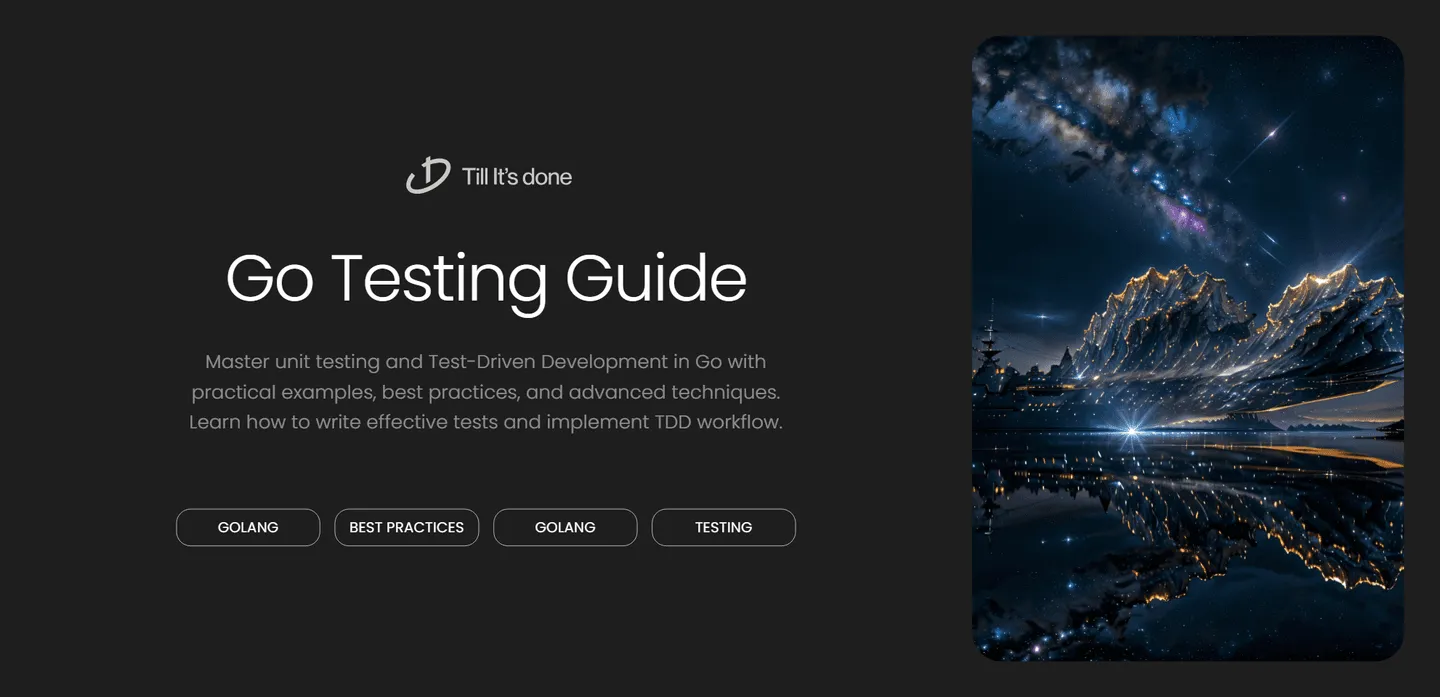
Unit Testing and Test-Driven Development in Go
As software engineers, we all know that writing tests is crucial for maintaining reliable and robust applications. In the Go ecosystem, testing isn’t just a best practice – it’s woven into the fabric of the language itself. Let’s dive deep into the world of unit testing and Test-Driven Development (TDD) in Go, exploring how these practices can elevate your code quality to new heights.
Getting Started with Go Testing
When I first started writing tests in Go, I was immediately struck by its simplicity. Go’s built-in testing package provides everything you need right out of the box. No need for external testing frameworks or complex setup – it’s all there in the standard library.
Here’s what a basic test structure looks like in Go:
func TestCalculateSum(t *testing.T) { result := CalculateSum(2, 3) if result != 5 { t.Errorf("Expected sum of 2 + 3 to be 5, got %d", result) }}
Embracing Test-Driven Development
TDD isn’t just about writing tests – it’s about changing how we think about code development. The cycle is simple but powerful:
- Write a failing test
- Write the minimum code to make it pass
- Refactor while keeping tests green
Let me share a real-world example of how this plays out in Go:
// First, write the testfunc TestUserValidation(t *testing.T) { user := User{Name: "", Email: "invalid"} errors := user.Validate()
if len(errors) != 2 { t.Errorf("Expected 2 validation errors, got %d", len(errors)) }}
// Then implement the codefunc (u User) Validate() []string { var errors []string if u.Name == "" { errors = append(errors, "name is required") } if !strings.Contains(u.Email, "@") { errors = append(errors, "invalid email format") } return errors}
Table-Driven Tests: Go’s Secret Weapon
One of my favorite testing patterns in Go is table-driven tests. They’re incredibly powerful for testing multiple scenarios without duplicating code:
func TestStringOperations(t *testing.T) { tests := []struct { name string input string expected string }{ {"empty string", "", ""}, {"single word", "hello", "HELLO"}, {"multiple words", "hello world", "HELLO WORLD"}, }
for _, tt := range tests { t.Run(tt.name, func(t *testing.T) { result := strings.ToUpper(tt.input) if result != tt.expected { t.Errorf("got %v, want %v", result, tt.expected) } }) }}
Best Practices for Go Testing
Remember these key points when writing tests:
- Keep your test files next to your code files with the _test.go suffix
- Use meaningful test names that describe the scenario being tested
- Aim for test isolation – each test should be independent
- Use t.Parallel() for concurrent test execution when possible
- Leverage test helpers for common setup and teardown operations
Advanced Testing Techniques
As your application grows, you’ll want to explore more advanced testing patterns:
// Using test helpersfunc setupTestDatabase(t *testing.T) *Database { t.Helper() db := NewTestDatabase() t.Cleanup(func() { db.Close() }) return db}
// Benchmark testsfunc BenchmarkStringOperation(b *testing.B) { for i := 0; i < b.N; i++ { strings.ToUpper("hello world") }}
Remember, effective testing isn’t about achieving 100% coverage – it’s about building confidence in your code’s behavior. Start small, focus on critical paths, and gradually expand your test suite as your application grows.
By embracing testing and TDD in your Go projects, you’re not just writing better code – you’re building a foundation for maintainable, reliable software that can stand the test of time.


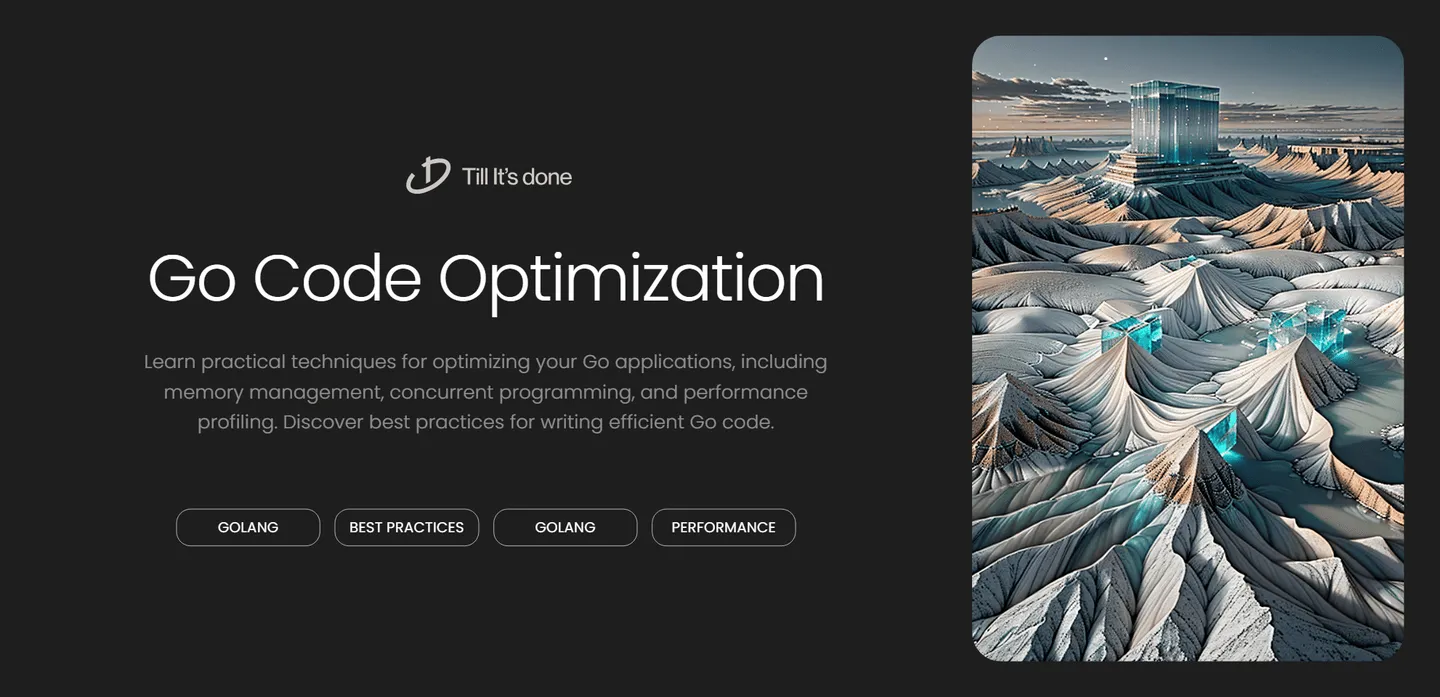



Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.