- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Go Memory Management and GC Tips for Devs
Learn about stack vs heap allocation, object pooling, and memory profiling.
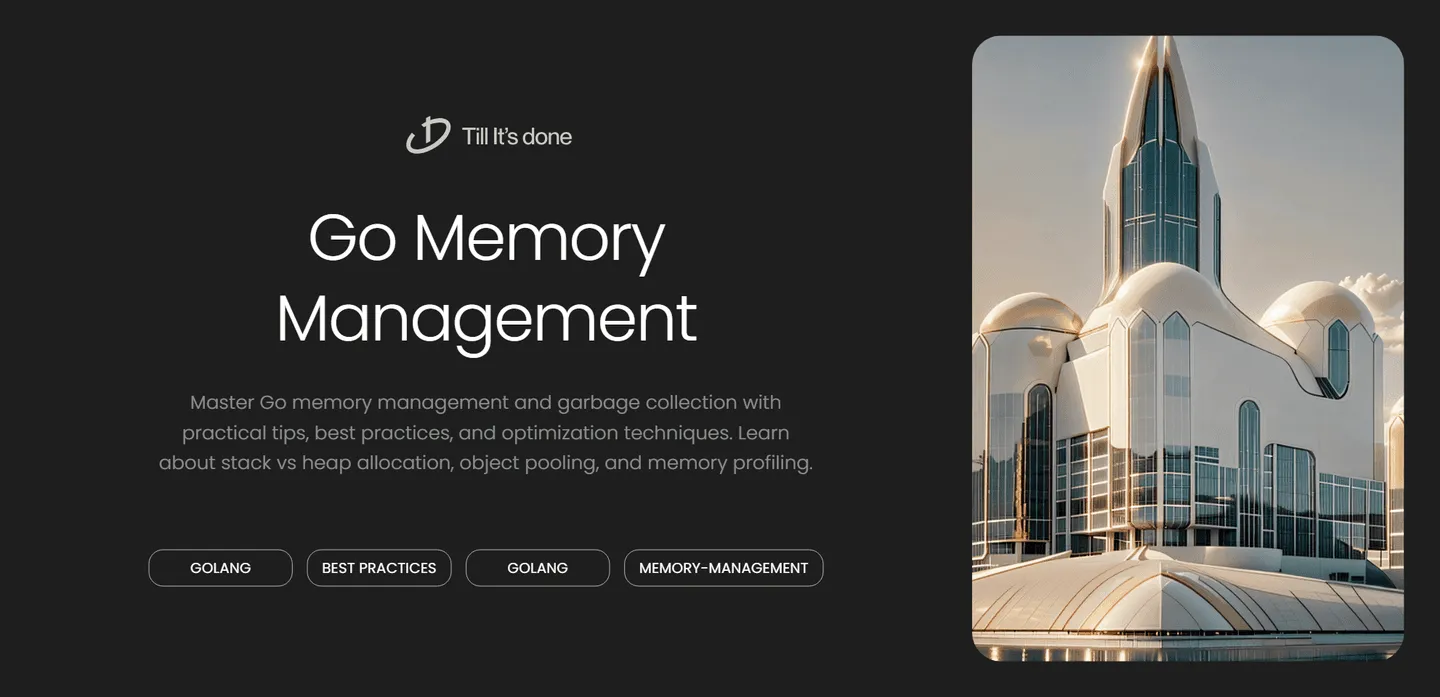
Mastering Go Memory Management and Garbage Collection: A Developer’s Guide
Memory management is one of the most critical aspects of building efficient Go applications. Today, let’s dive deep into how Go handles memory and explore some practical tips to optimize your applications.
Understanding Go’s Memory Model
Go’s memory management is designed to be simple yet powerful. Unlike languages like C or C++, Go handles memory allocation and deallocation automatically through its garbage collector. However, this doesn’t mean we can ignore memory management entirely.
The Stack and Heap
In Go, memory allocation happens in two places:
- Stack: Fast, automatically managed memory for local variables
- Heap: Larger, dynamically allocated memory managed by the garbage collector
Here’s what makes Go’s approach unique: the compiler automatically decides whether to allocate variables on the stack or heap through “escape analysis.” This process determines if a variable needs to persist beyond its function’s lifecycle.
Practical Tips for Efficient Memory Management
1. Optimize Slice and Map Initialization
When you know the approximate size of your slices or maps beforehand, initialize them with a capacity:
// Instead ofusers := make([]User, 0)
// Useusers := make([]User, 0, estimatedSize)
This simple change can significantly reduce memory reallocations and improve performance.
2. Pool Frequently Used Objects
For objects that are frequently allocated and deallocated, use sync.Pool:
var bufferPool = sync.Pool{ New: func() interface{} { return new(bytes.Buffer) },}
3. Watch for Memory Leaks
Common sources of memory leaks in Go include:
- Forgotten goroutines
- Unbounded caches
- Unclosed channels
- Large maps that grow but never shrink
4. Garbage Collection Tuning
While Go’s garbage collector is highly optimized, you can still tune it:
// Set GC percentagedebug.SetGCPercent(100)
// Force garbage collectionruntime.GC()
Monitoring and Profiling
Always monitor your application’s memory usage. Go provides excellent tools:
- Use
runtime.MemStats
to track memory statistics - Employ pprof for detailed memory profiling
- Implement metrics collection for production monitoring
Best Practices Summary
- Use proper data structures and initialize them with appropriate sizes
- Implement object pooling for frequently allocated objects
- Regularly profile your application’s memory usage
- Be mindful of goroutine lifecycles
- Consider garbage collection impact during critical operations
Remember: premature optimization is the root of all evil. Profile first, optimize second.
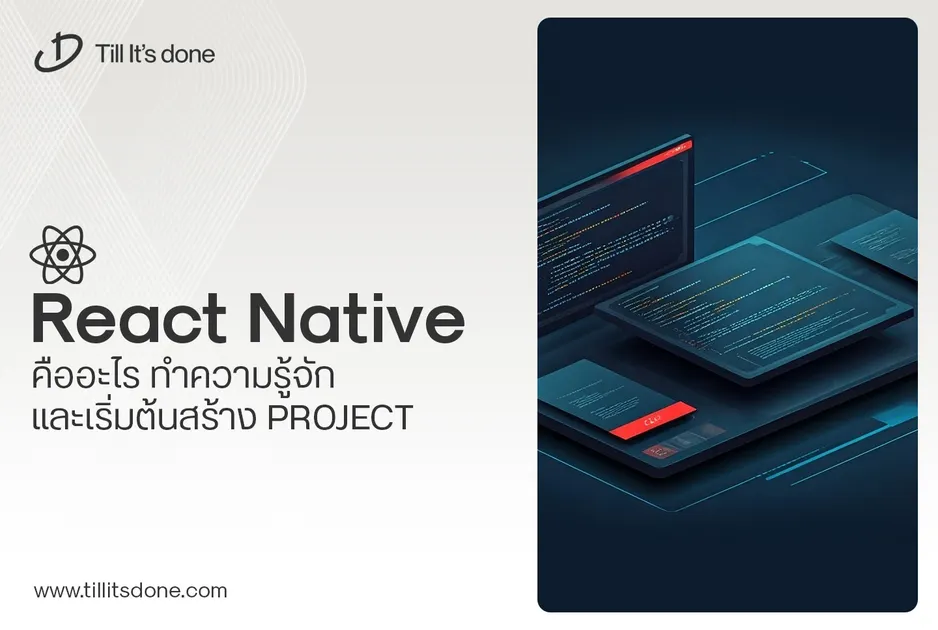
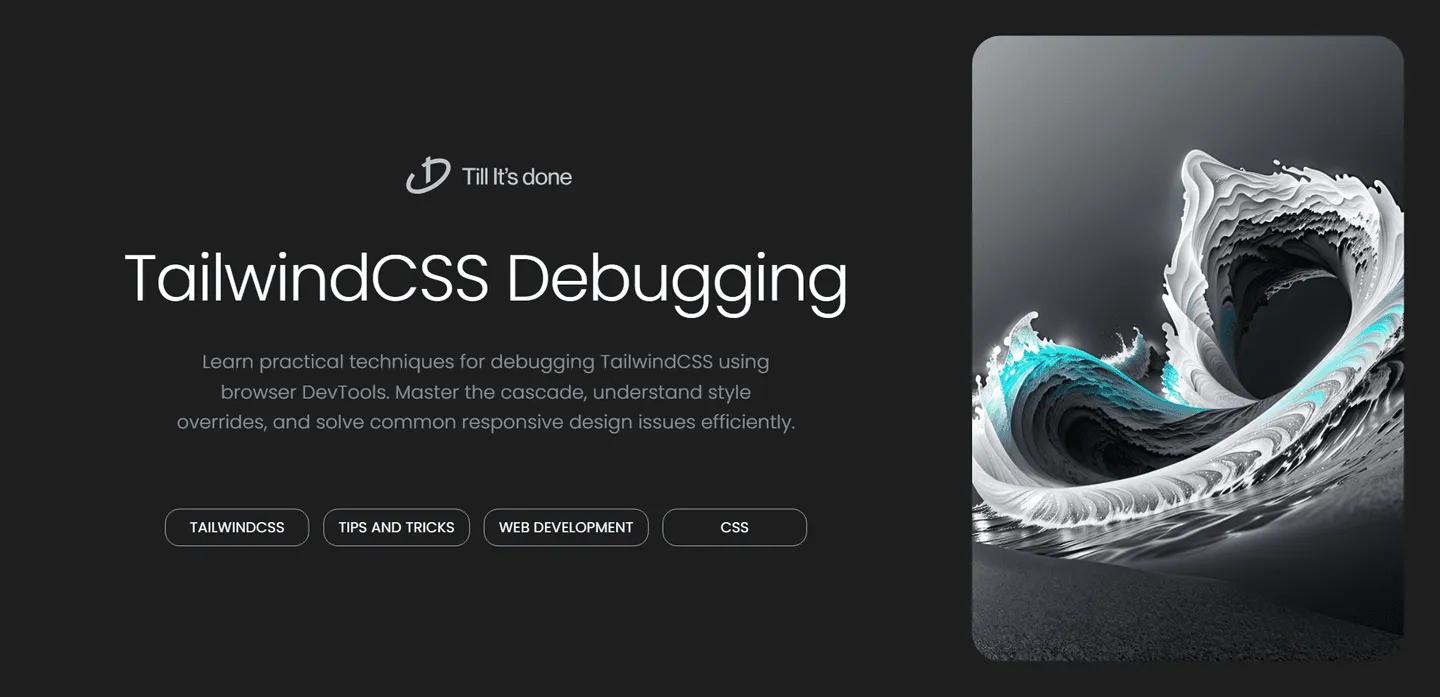
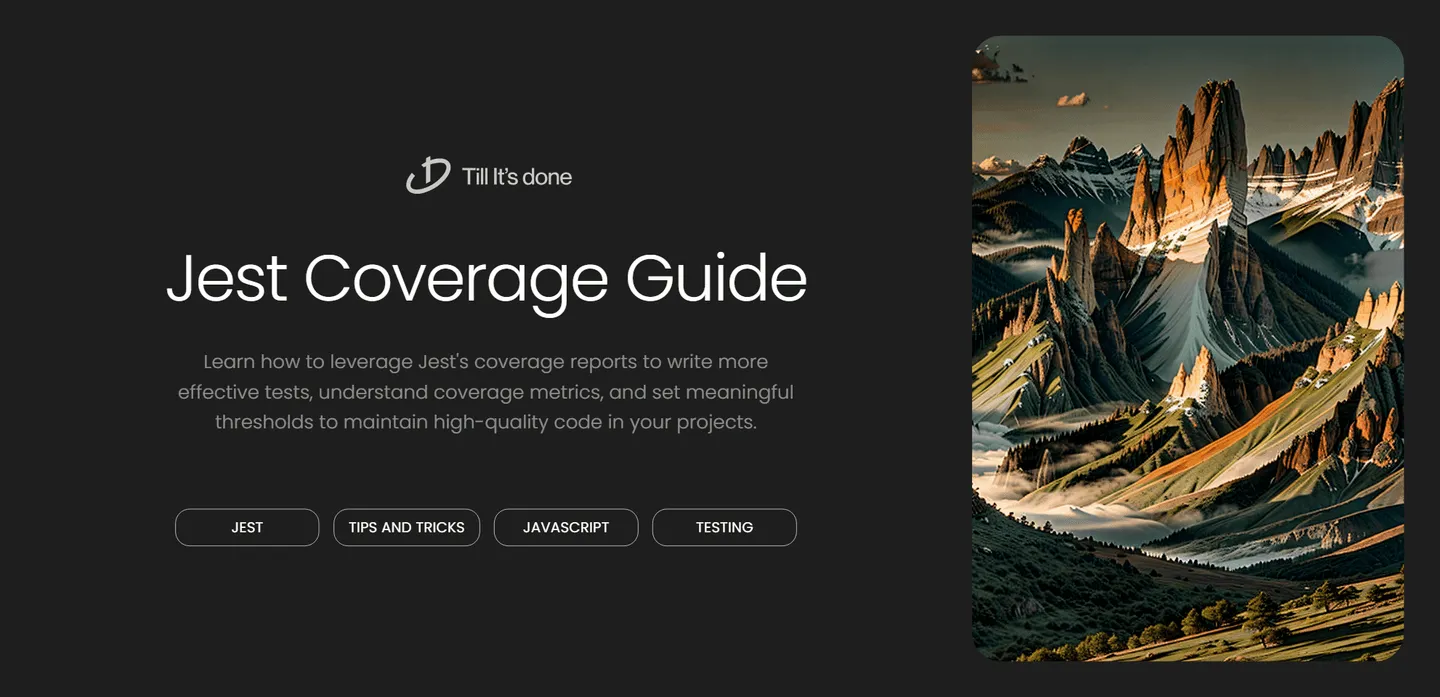
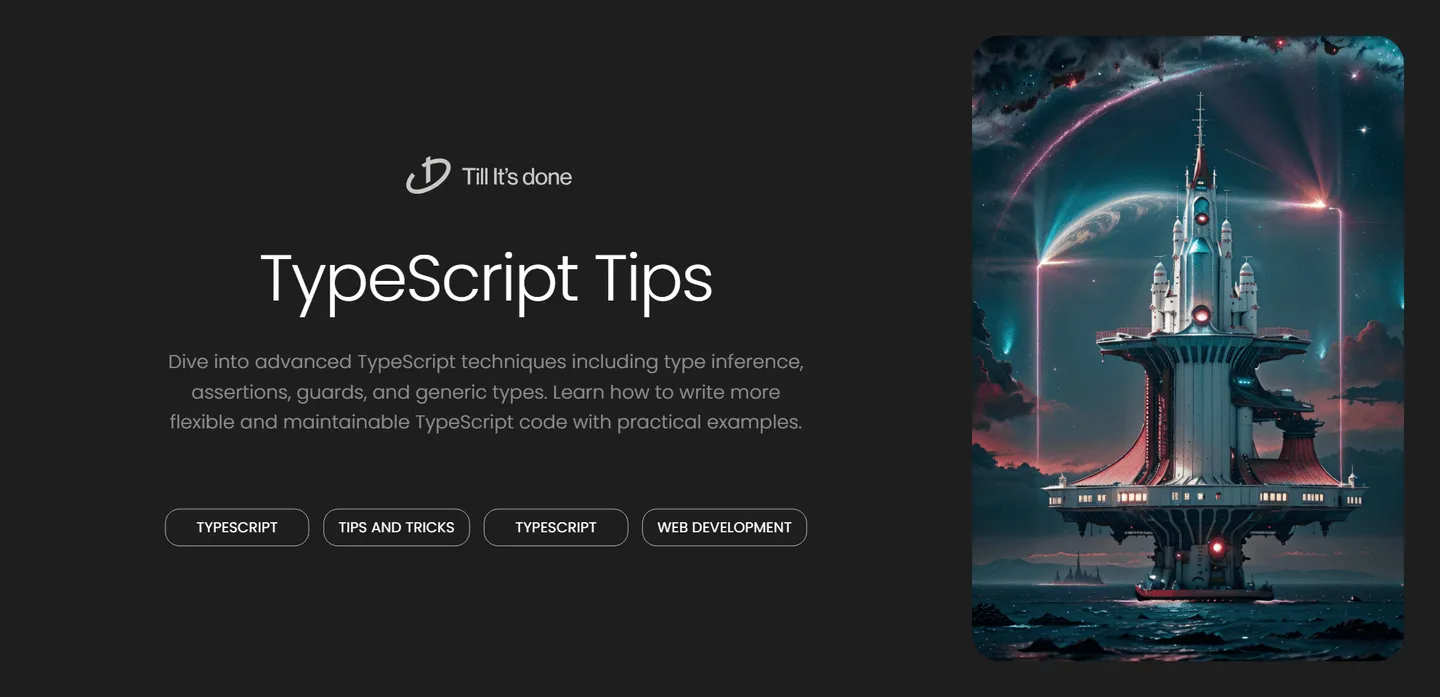
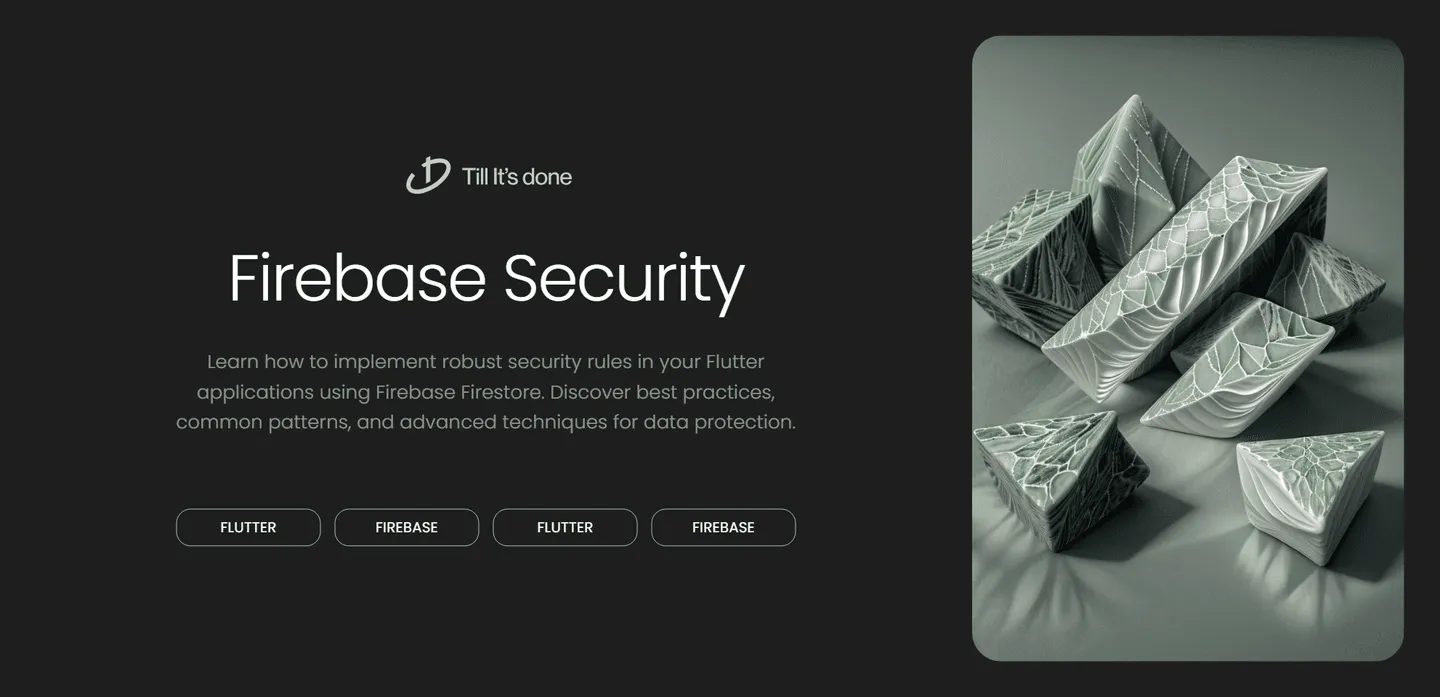
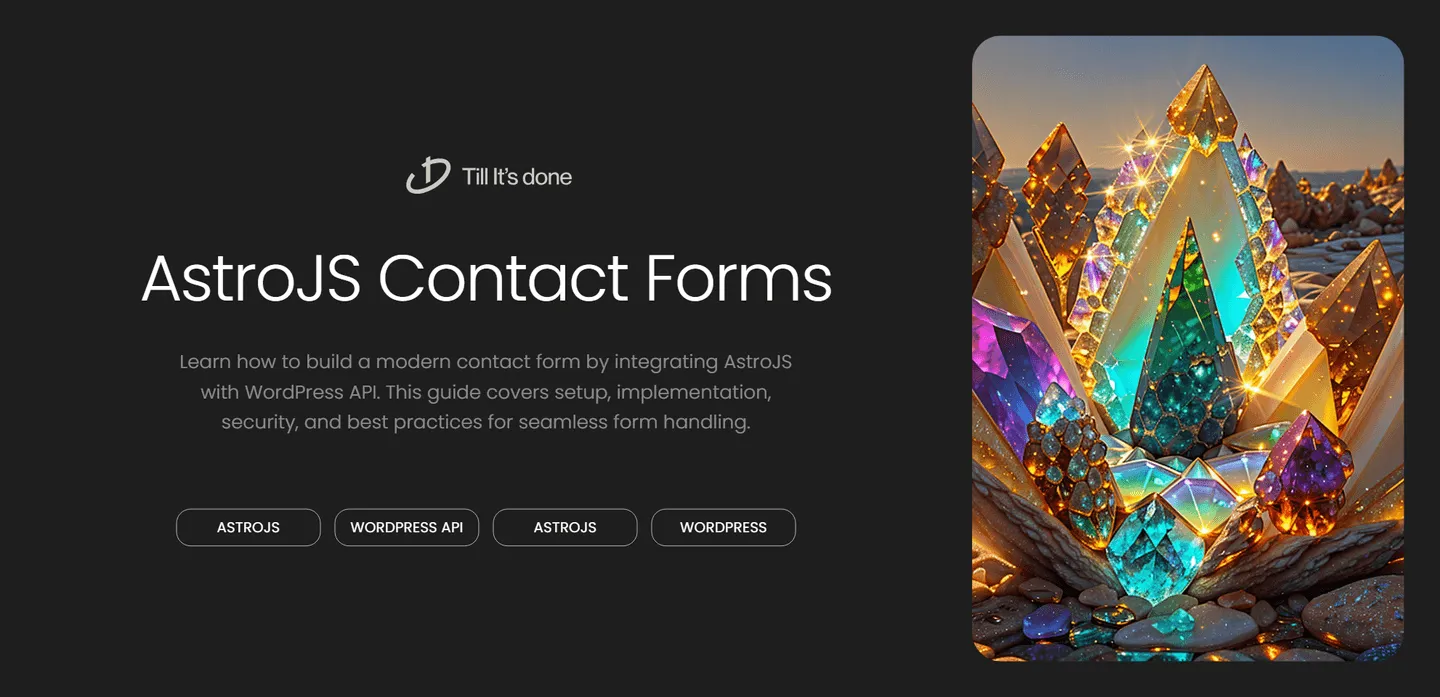
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.