- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices for Concurrency in Go
Discover how to properly manage goroutines, handle errors, and avoid common pitfalls in concurrent programming.
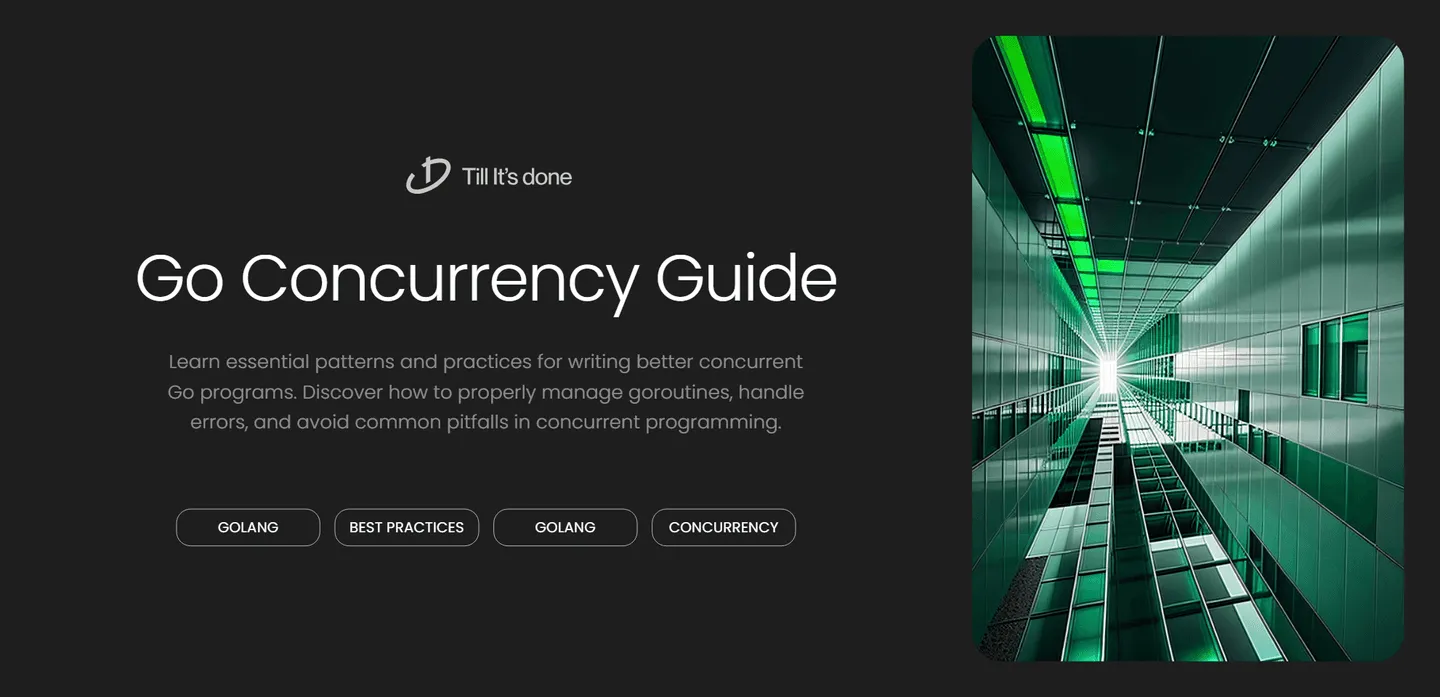
Best Practices for Concurrency in Go
Concurrency is one of Go’s most powerful features, but with great power comes great responsibility. As someone who’s spent countless hours debugging concurrent programs, I’ve learned that following certain best practices can save you from many headaches. Let’s dive into some essential patterns and practices that will help you write better concurrent Go code.
Understanding Go’s Concurrency Model
Before jumping into best practices, it’s crucial to understand that Go’s concurrency model is built around two main concepts: goroutines and channels. Think of goroutines as lightweight threads and channels as the pipes connecting them. This model follows the philosophy of “Don’t communicate by sharing memory; share memory by communicating.”
Essential Best Practices
1. Always Clean Up Your Goroutines
One of the most common mistakes I see is launching goroutines without proper cleanup. Leaked goroutines can consume resources and potentially cause memory leaks. Here’s how to handle them properly:
func main() { ctx, cancel := context.WithCancel(context.Background()) defer cancel() // Always remember to cancel when done
go worker(ctx) // Rest of your code}
func worker(ctx context.Context) { for { select { case <-ctx.Done(): return default: // Do work } }}
2. Use Buffered Channels Wisely
Buffered channels can be powerful, but they’re not a silver bullet. Use them when you know the exact number of elements that will be sent:
// Good: Known number of elementsch := make(chan int, len(workers))
// Bad: Arbitrary buffer sizech := make(chan int, 100) // Why 100?
3. Implement Proper Error Handling
Error handling in concurrent programs requires special attention. Always propagate errors through channels or error groups:
func main() { errCh := make(chan error) go func() { defer close(errCh) if err := doWork(); err != nil { errCh <- err } }()
if err := <-errCh; err != nil { log.Fatal(err) }}
4. Rate Limiting and Throttling
When dealing with external services or resource-intensive operations, implement rate limiting to prevent overwhelming your system:
rate := time.Second / 10 // 10 operations per secondthrottle := time.Tick(rate)
for items := range work { <-throttle // Rate limit our operations go process(items)}
Common Pitfalls to Avoid
- Don’t create goroutines in loops without limits
- Avoid sharing memory without synchronization
- Don’t forget to handle panic recovery in goroutines
- Never ignore the context cancellation
Conclusion
Mastering concurrency in Go is a journey, not a destination. Start with these best practices, but always remember that the best code is the one that’s readable, maintainable, and reliable. Keep experimenting, learning, and most importantly, keep your concurrent code simple and purposeful.
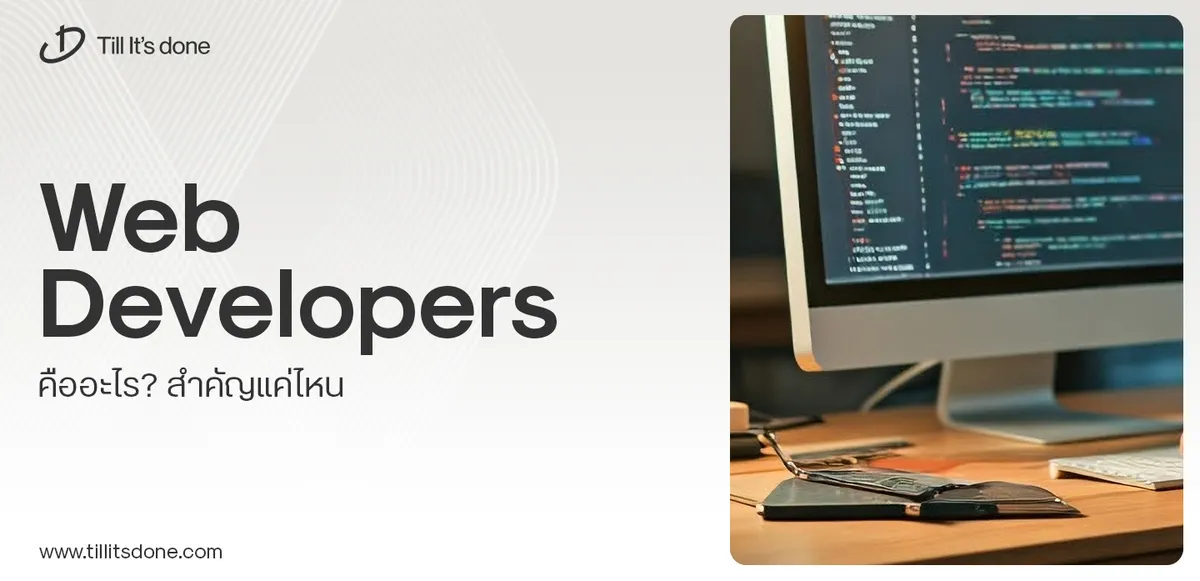
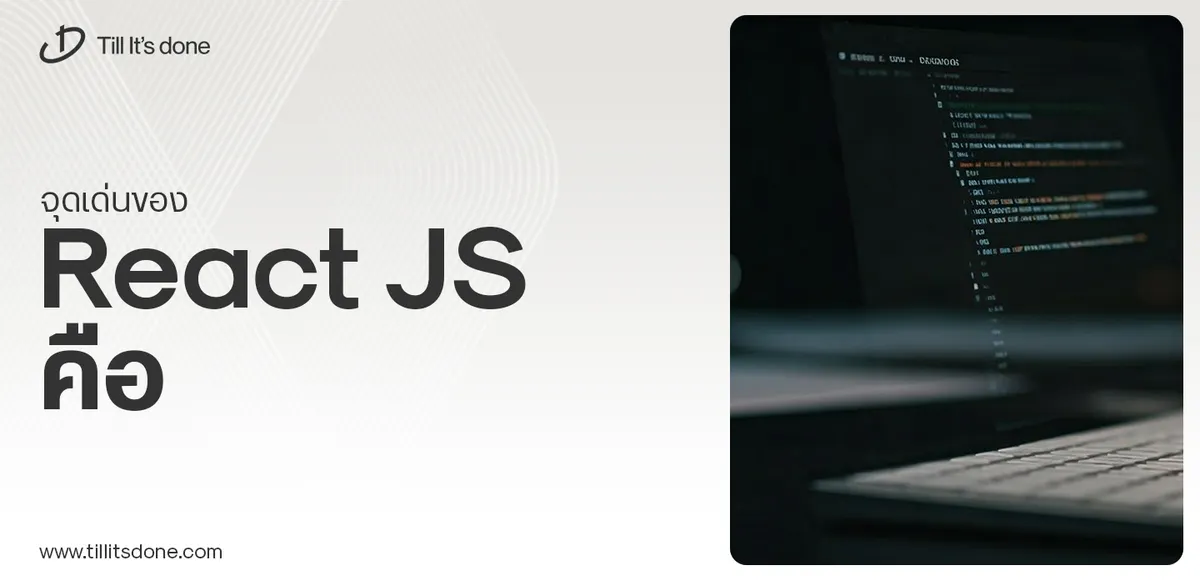
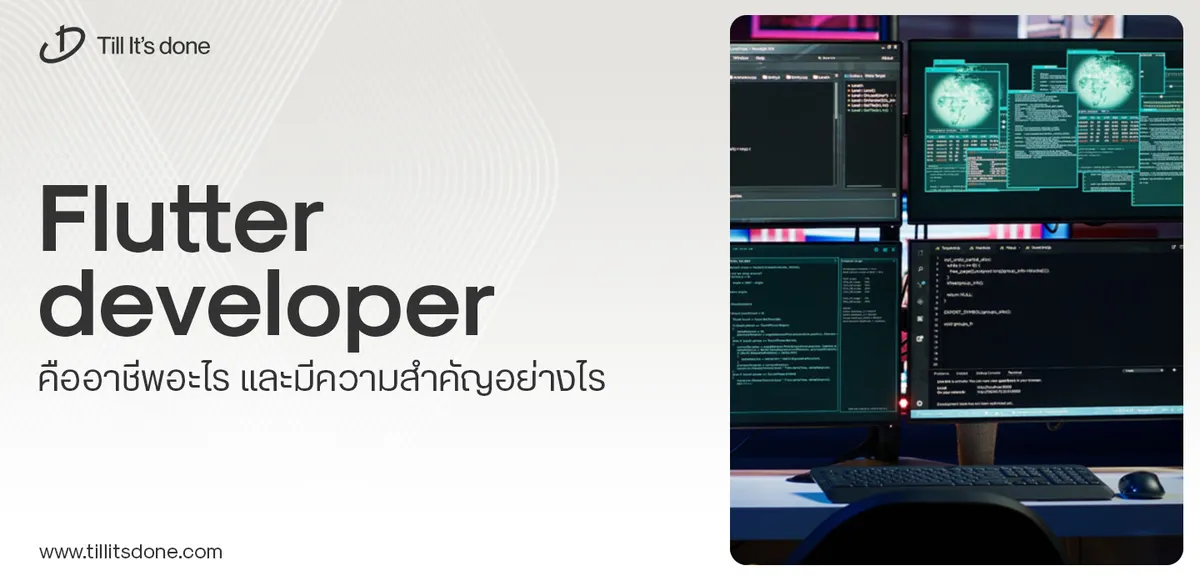
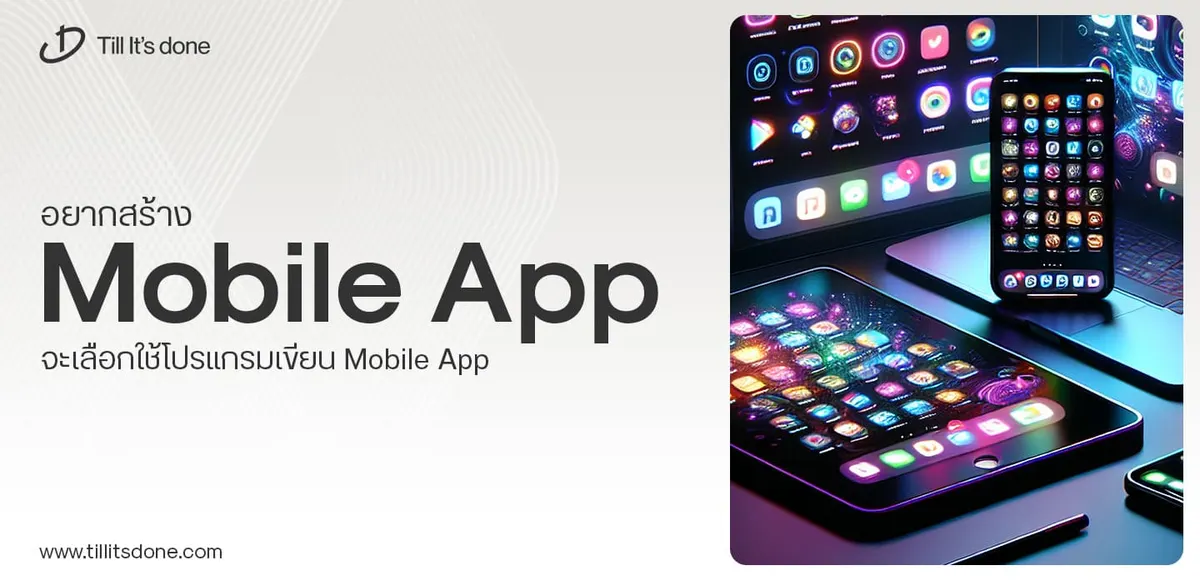
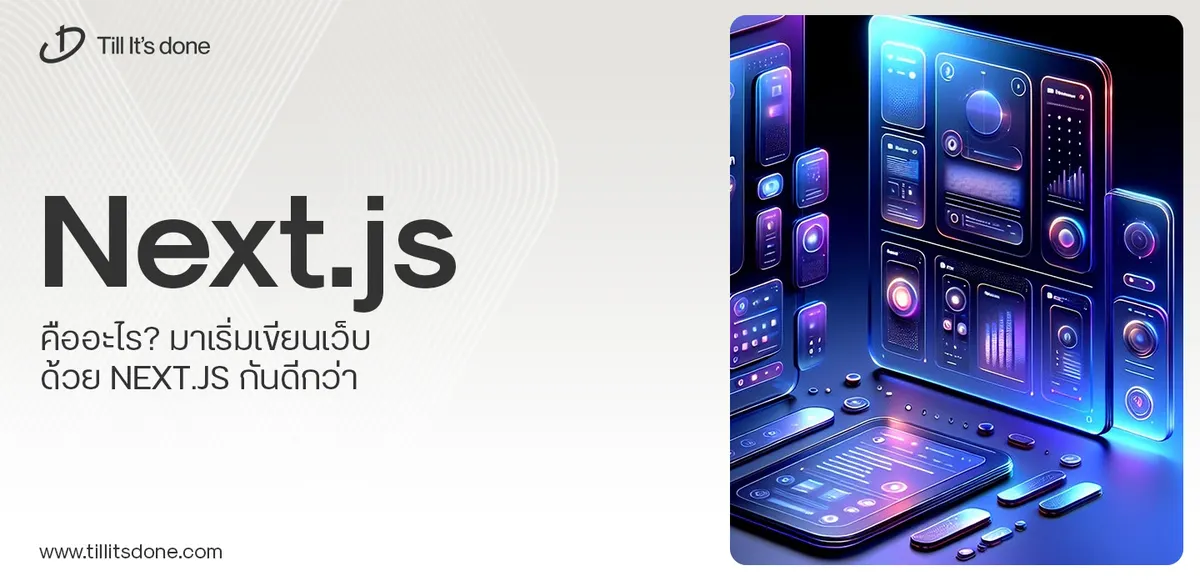
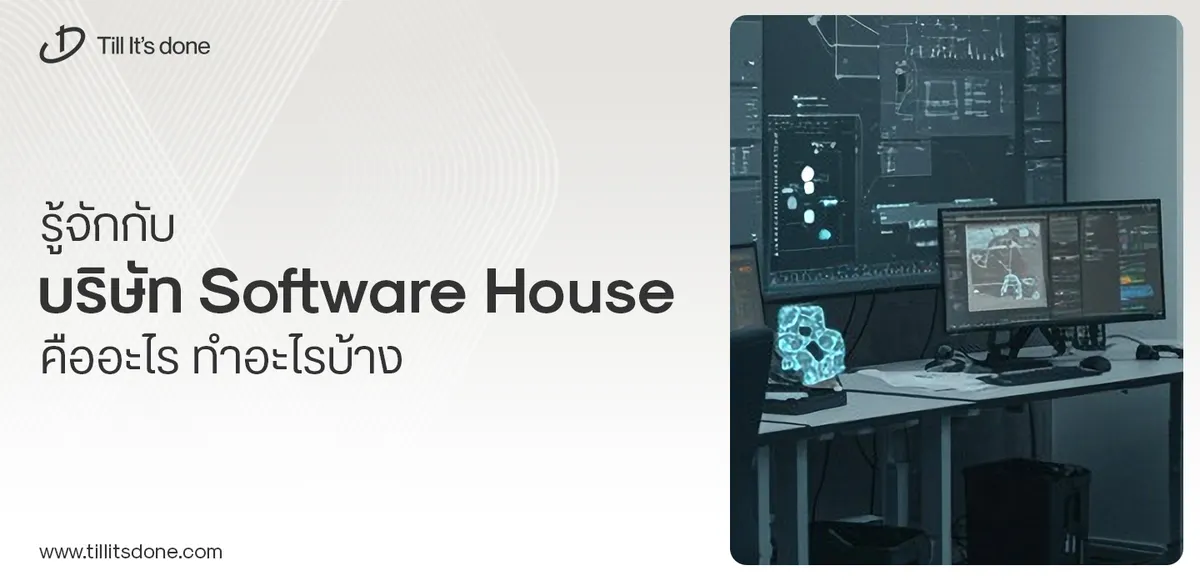
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.