- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Optimizing Go Code for Better Performance
Discover best practices for writing efficient Go code.
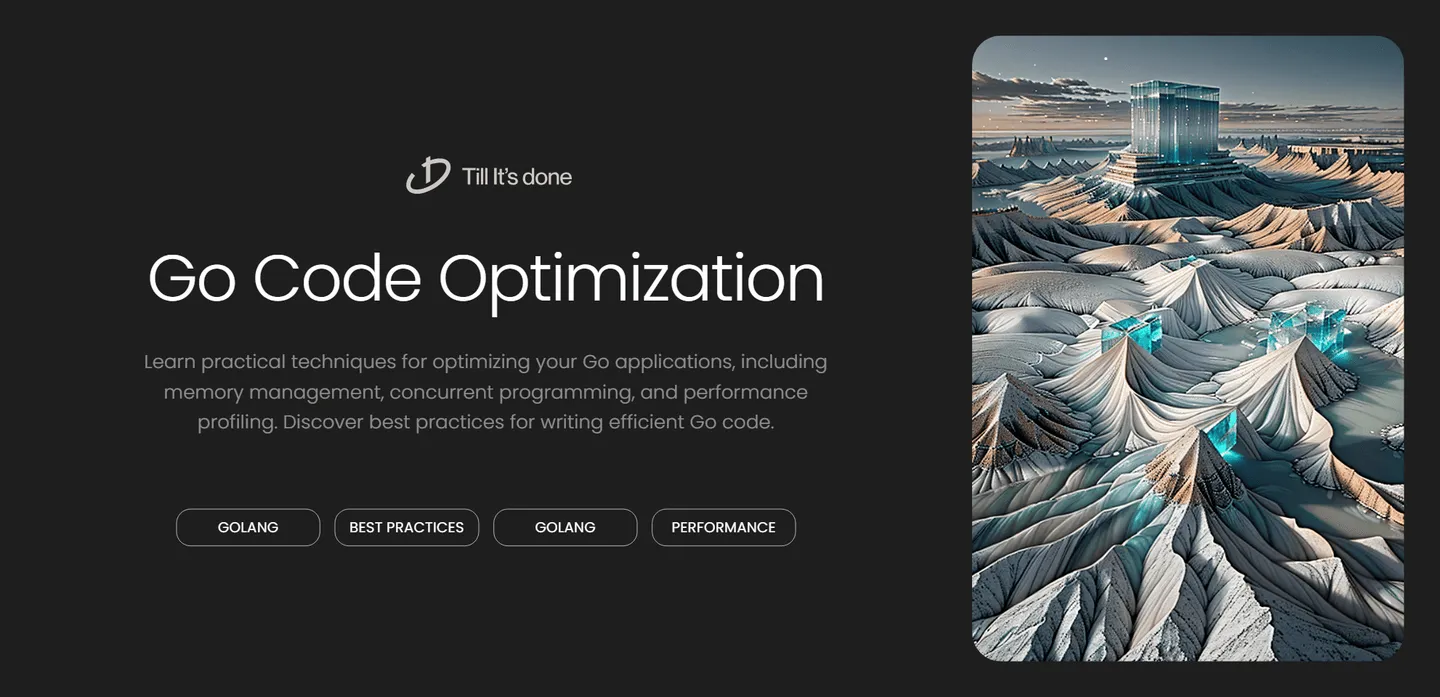
Optimizing Go Code for Performance: A Practical Guide
Performance optimization is a crucial aspect of Go programming that can significantly impact your application’s efficiency and user experience. In this guide, we’ll explore practical techniques to enhance your Go code’s performance while maintaining its readability and maintainability.
Understanding the Basics of Go Performance
Before diving into optimization techniques, it’s essential to understand what makes Go performant. Go’s built-in features like goroutines and garbage collection already provide excellent baseline performance. However, there’s always room for improvement when you know where to look.
Memory Management Optimization
Memory management plays a crucial role in application performance. Here are some effective strategies:
- Use sync.Pool for frequently allocated objects
- Minimize unnecessary allocations in hot paths
- Consider using object pre-allocation for known sizes
- Implement proper buffer sizing in I/O operations
For example, when working with slices that have a predictable size:
// Instead of thisdata := make([]int, 0)for i := 0; i < size; i++ { data = append(data, i)}
// Do thisdata := make([]int, 0, size)for i := 0; i < size; i++ { data = append(data, i)}
Concurrent Programming Optimization
Go’s concurrency model is powerful, but it needs to be used wisely:
- Use appropriate goroutine pool sizes
- Implement proper channel buffering
- Avoid goroutine leaks
- Consider using worker pools for CPU-intensive tasks
Performance Profiling and Monitoring
Always measure before optimizing. Go provides excellent tools for profiling:
import "runtime/pprof"
// CPU profiling examplef, _ := os.Create("cpu.prof")pprof.StartCPUProfile(f)defer pprof.StopCPUProfile()
Best Practices for Optimal Performance
- Use strconv instead of fmt for string conversions
- Implement proper error handling without excessive allocations
- Optimize JSON encoding/decoding with proper struct tags
- Use appropriate data structures for your use case
Remember, premature optimization is the root of all evil. Always profile first to identify actual bottlenecks rather than optimizing blindly.
Conclusion
Optimizing Go code is an iterative process that requires understanding both the language’s strengths and your application’s specific needs. Focus on measuring performance, identifying bottlenecks, and making targeted improvements where they matter most.






Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.