- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Interfaces in Go for Better Design
Discover interface composition, best practices, and real-world examples for building better Go applications.

Using Interfaces in Go for Better Design
Go’s interface system is one of its most powerful features, yet many developers don’t tap into its full potential. Let’s dive into how interfaces can transform your code from rigid and tightly coupled into something more elegant and maintainable.
Understanding Go Interfaces: The Basics
Think of interfaces as contracts. Instead of telling your code exactly what type of data it should expect, you’re telling it what behavior it should expect. This subtle shift in thinking opens up a world of possibilities for better software design.
type Writer interface { Write([]byte) (int, error)}
This simple interface is at the heart of many Go standard library components. Any type that implements a Write method with this signature automatically satisfies this interface - no explicit declaration needed. This is what we call implicit interface satisfaction, and it’s beautiful in its simplicity.
Real-World Interface Design
Let’s look at a practical example. Imagine you’re building a notification system:
type Notifier interface { Notify(message string) error}
type EmailNotifier struct { // email configuration}
type SlackNotifier struct { // slack configuration}
func (e EmailNotifier) Notify(message string) error { // Send email implementation return nil}
func (s SlackNotifier) Notify(message string) error { // Send slack message implementation return nil}
By using interfaces, we’ve created a flexible system that can handle different types of notifications without changing the core logic. Want to add SMS notifications? Just create a new type that satisfies the Notifier interface.
Interface Composition: Building Blocks for Complex Systems
One of Go’s strengths is the ability to compose interfaces:
type Reader interface { Read(p []byte) (n int, err error)}
type Writer interface { Write(p []byte) (n int, err error)}
type ReadWriter interface { Reader Writer}
This composability allows you to build complex systems from simple, focused interfaces. Each interface should have a single responsibility, following the Interface Segregation Principle.
Best Practices for Interface Design
- Keep interfaces small - aim for one to three methods
- Define interfaces at the package that uses them, not the package that implements them
- Use interfaces to decouple packages and improve testability
- Start with concrete types and extract interfaces when you need flexibility
Remember, interfaces aren’t just a language feature - they’re a design tool. Use them to make your code more modular, testable, and maintainable.
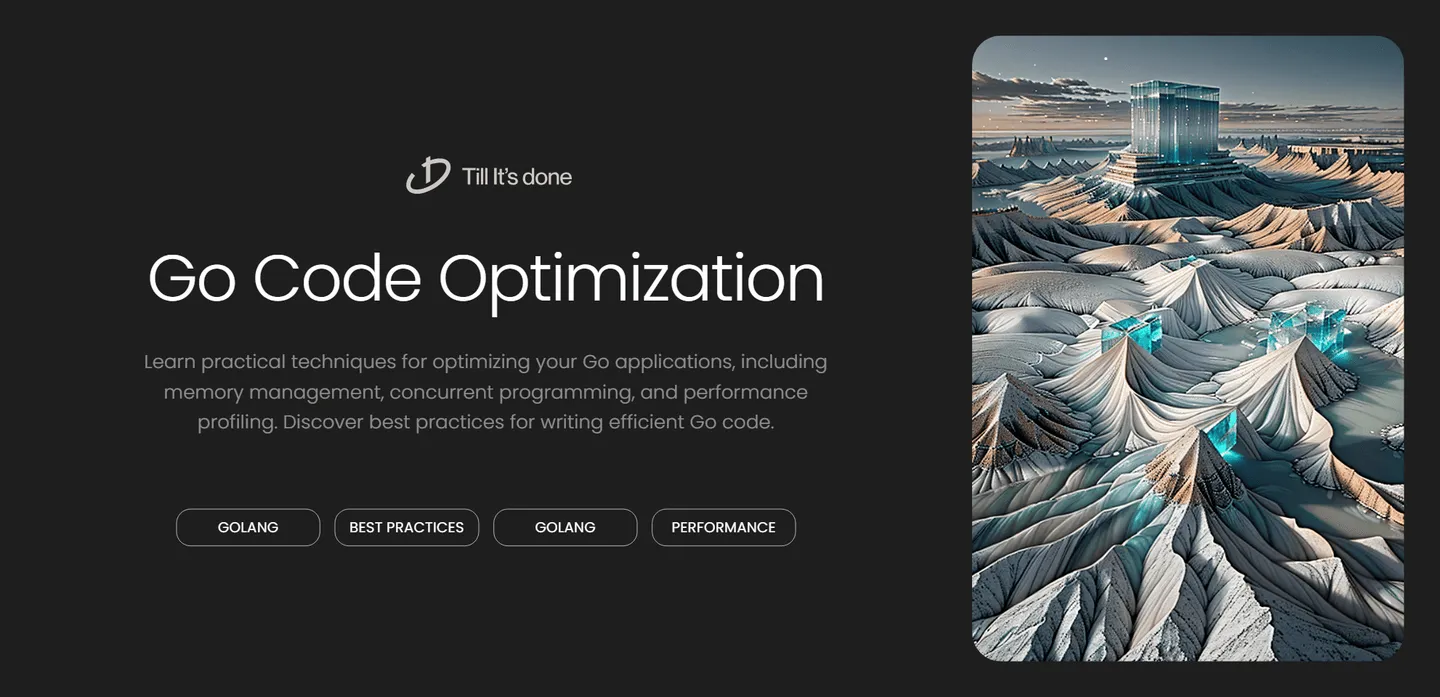





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.