- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Structuring Go Projects for Scalability Guide
Covers project layout, domain-driven design, testing strategies, and practical implementation examples.
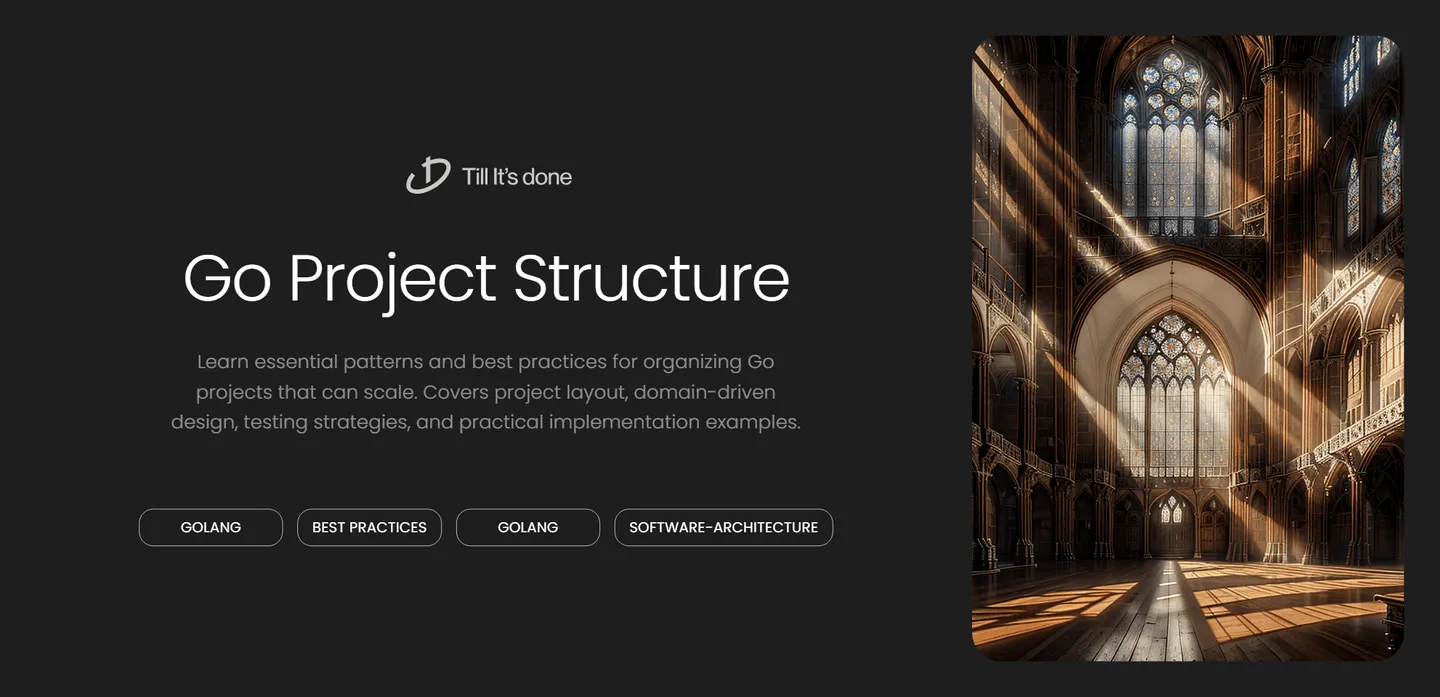
Structuring Go Projects for Scalability
In the ever-evolving landscape of software development, structuring your Go projects for scalability isn’t just a best practice—it’s a necessity. Let’s dive into how you can architect your Go projects to maintain clarity, efficiency, and scalability as your application grows.
The Foundation: Project Layout
The way you organize your Go project sets the tone for its entire lifecycle. A well-structured project is like a well-designed city—everything has its place, and navigation becomes intuitive. Here’s a battle-tested project structure:
my-project/├── cmd/│ └── server/│ └── main.go├── internal/│ ├── domain/│ ├── service/│ └── repository/├── pkg/│ ├── middleware/│ └── utils/├── api/│ └── handlers/├── config/└── scripts/
Domain-Driven Design in Go
One of the most powerful approaches to structuring Go applications is embracing Domain-Driven Design (DDD). This methodology helps you organize code around business concepts and maintain clear boundaries between different parts of your application.
Key Principles for Scalable Structure
- Separation of Concerns
type User struct { ID string Name string Email string}
// service/user.gotype UserService struct { repo UserRepository cache Cache}
- Interface-Driven Development
type UserRepository interface { Find(id string) (*User, error) Save(user *User) error}
- Dependency Injection Your services should receive their dependencies rather than creating them:
func NewUserService(repo UserRepository, cache Cache) *UserService { return &UserService{ repo: repo, cache: cache, }}
Scaling Considerations
When building for scale, consider these crucial aspects:
1. Configuration Management
Store configuration in environment variables or configuration files, never hardcode:
type Config struct { DatabaseURL string `envconfig:"DATABASE_URL"` RedisURL string `envconfig:"REDIS_URL"` Port int `envconfig:"PORT" default:"8080"`}
2. Error Handling
Implement consistent error handling patterns:
type AppError struct { Code int Message string Err error}
func (e *AppError) Error() string { return fmt.Sprintf("%s: %v", e.Message, e.Err)}
3. Middleware Chain
Create modular middleware that can be easily composed:
func Chain(handler http.Handler, middleware ...Middleware) http.Handler { for i := len(middleware) - 1; i >= 0; i-- { handler = middleware[i](handler) } return handler}
Testing Strategy
A scalable project needs a robust testing strategy. Organize your tests to mirror your project structure:
my-project/├── internal/│ └── service/│ ├── user_service.go│ ├── user_service_test.go│ └── user_service_integration_test.go
Remember to follow these testing principles:
- Write unit tests for core business logic
- Use interfaces for easy mocking
- Implement integration tests for critical paths
- Maintain test coverage above 80%
Continuous Integration and Deployment
Set up CI/CD pipelines that enforce your project’s quality standards:
- Run tests automatically
- Check code coverage
- Perform static code analysis
- Build and deploy artifacts
Conclusion
A well-structured Go project is the foundation for building scalable applications. By following these patterns and principles, you’re setting yourself up for success as your application grows. Remember, good structure isn’t about following rules blindly—it’s about making intentional decisions that support your application’s growth and maintainability.
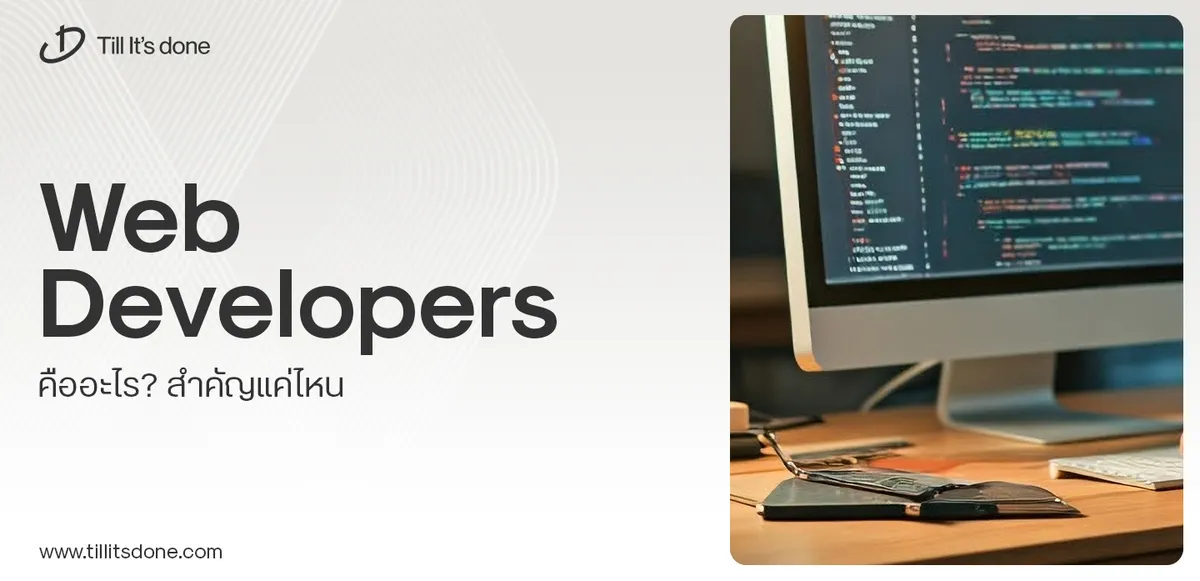
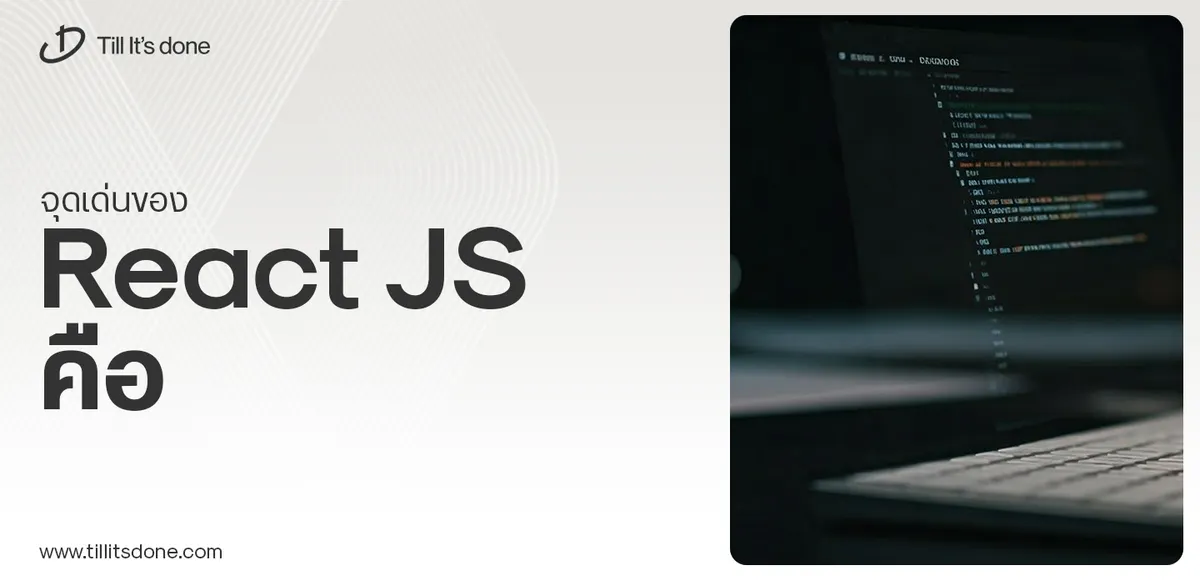
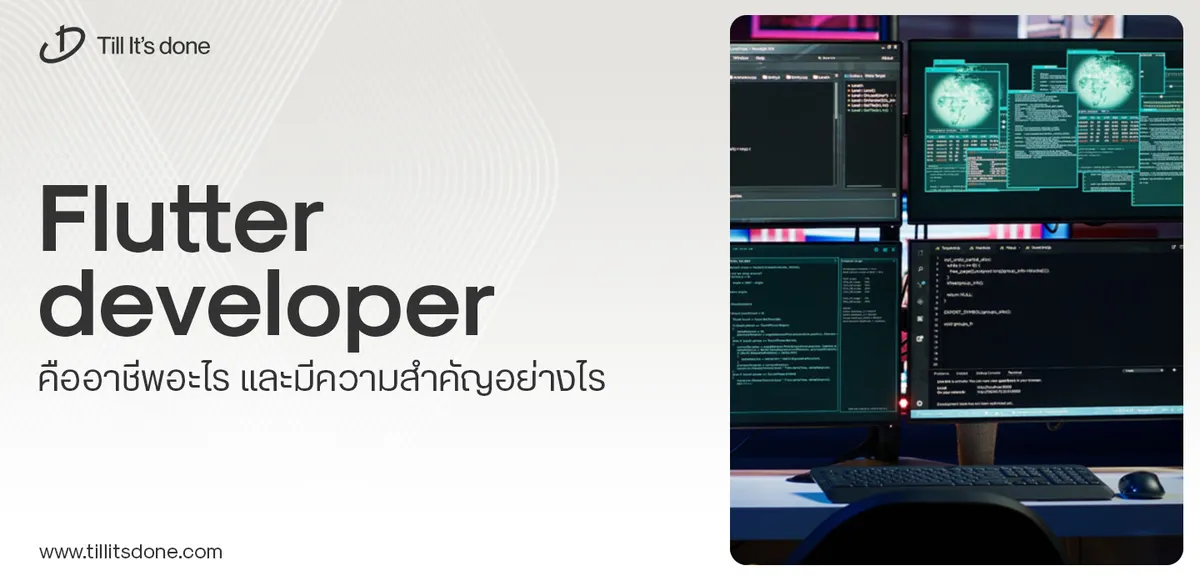
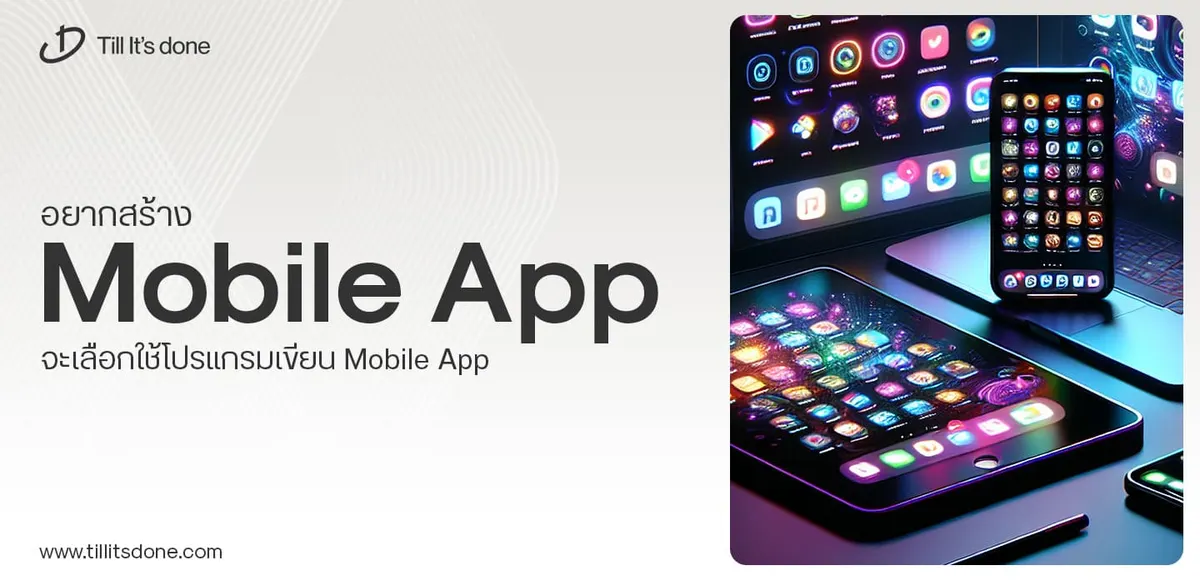
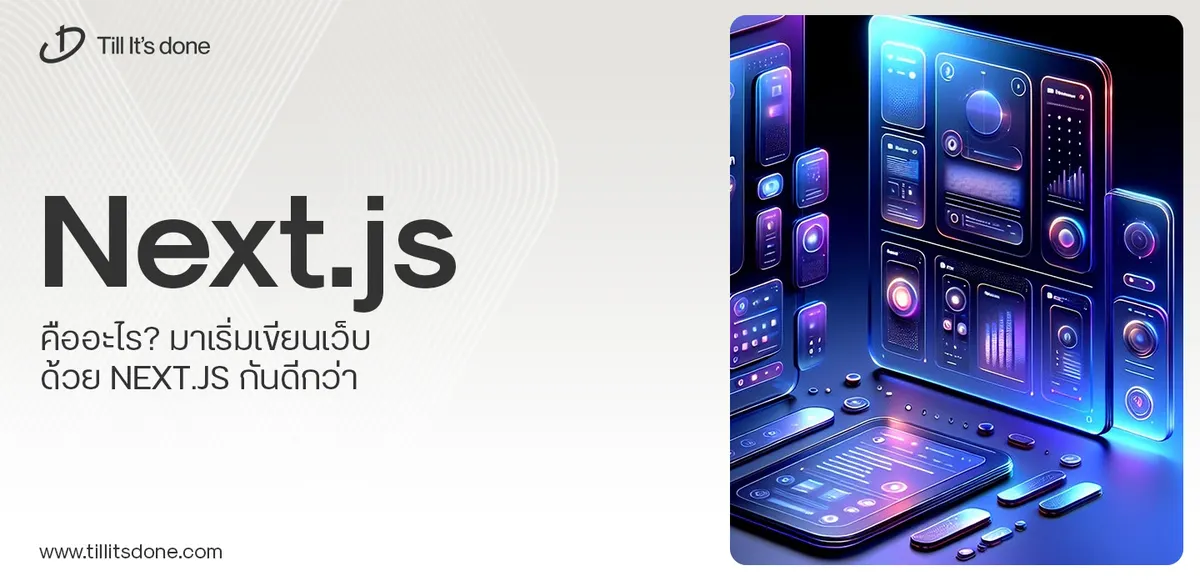
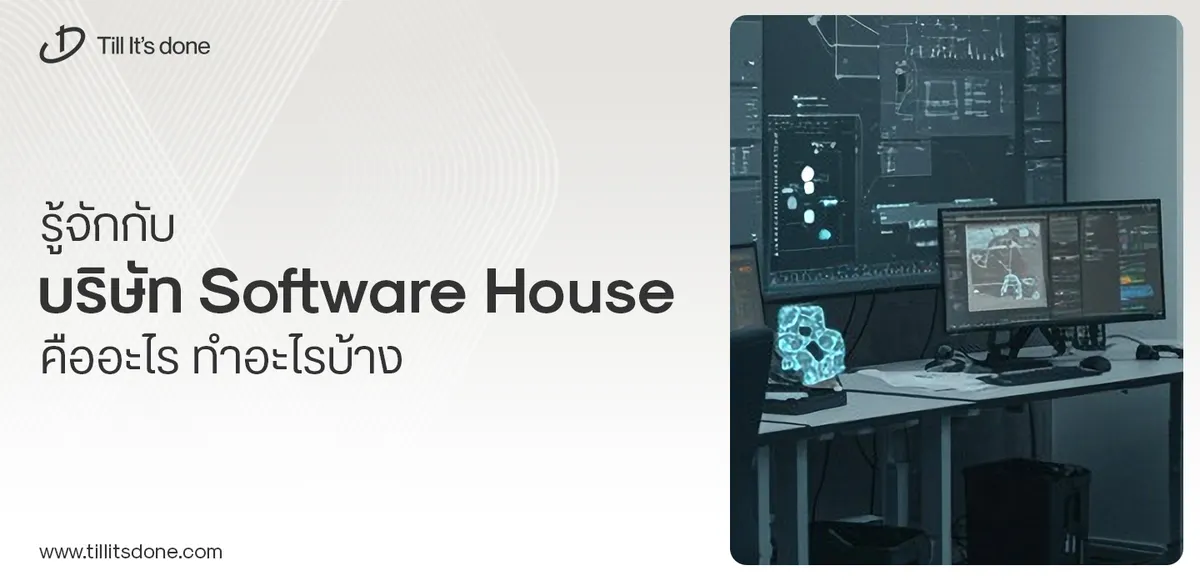
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.