- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Build Real-Time Apps with PGX and PostgreSQL
Discover patterns for building scalable, event-driven systems with database triggers.

Building a Real-Time Application with PGX and PostgreSQL LISTEN/NOTIFY in Go
Real-time applications have become increasingly important in modern software development. In this post, we’ll explore how to build a real-time application using Go, PGX, and PostgreSQL’s powerful LISTEN/NOTIFY feature. This combination allows us to create responsive applications that can react to database changes instantly.
Understanding PostgreSQL LISTEN/NOTIFY
PostgreSQL’s LISTEN/NOTIFY is a powerful pub/sub mechanism that enables real-time communication between database clients. Think of it as a built-in messaging system where database events can trigger notifications to connected applications.
Setting Up the Project
First, let’s set up our Go project with PGX. We’ll need to install the necessary dependencies:
go mod init realtime-appgo get github.com/jackc/pgx/v4
Implementing the Listener
Here’s how we can create a basic listener that connects to PostgreSQL and waits for notifications:
func createListener(connString string) (*pgx.Conn, error) { conn, err := pgx.Connect(context.Background(), connString) if err != nil { return nil, fmt.Errorf("unable to connect to database: %v", err) }
_, err = conn.Exec(context.Background(), "LISTEN data_changes") if err != nil { return nil, fmt.Errorf("unable to start listening: %v", err) }
return conn, nil}
Creating the Notification Trigger
We need to create a PostgreSQL trigger that will send notifications when data changes:
CREATE OR REPLACE FUNCTION notify_data_changes()RETURNS trigger AS $$BEGIN PERFORM pg_notify('data_changes', row_to_json(NEW)::text); RETURN NEW;END;$$ LANGUAGE plpgsql;
Handling Notifications in Real-Time
Here’s how we can process the notifications in our Go application:
func handleNotifications(conn *pgx.Conn) { for { notification, err := conn.WaitForNotification(context.Background()) if err != nil { log.Printf("Error waiting for notification: %v", err) continue }
// Process the notification fmt.Printf("Received notification: %s\n", notification.Payload) }}
Best Practices and Considerations
- Always handle connection losses gracefully
- Implement reconnection logic
- Use connection pooling for better performance
- Consider payload size limitations
- Implement error handling and logging
Remember that LISTEN/NOTIFY is not guaranteed delivery - if your application is offline, it will miss notifications. Consider implementing additional mechanisms like timestamp-based polling for critical data synchronization.
Conclusion
Using PGX with PostgreSQL’s LISTEN/NOTIFY feature provides a powerful foundation for building real-time applications in Go. This approach is particularly useful for scenarios requiring immediate updates, such as chat applications, real-time dashboards, or collaborative tools.
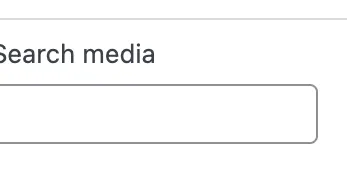





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.