- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using PGX with PostgreSQL JSON Columns in Go
Discover best practices for storing, querying, and managing complex JSON data structures in your Go applications.
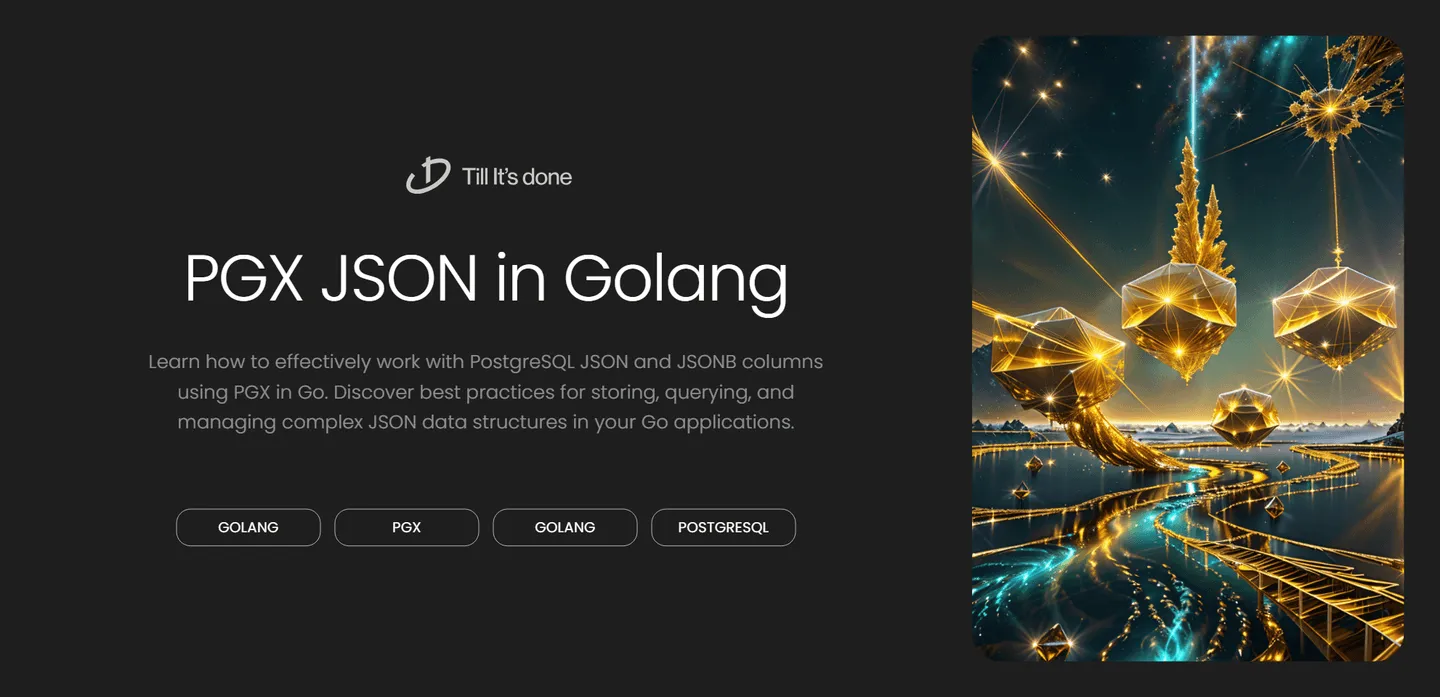
Using PGX to Interact with PostgreSQL JSON/JSONB Columns in Go
Working with JSON data in PostgreSQL has become increasingly common in modern applications. Thanks to PostgreSQL’s powerful JSON and JSONB data types, we can store and query complex data structures efficiently. In this article, we’ll explore how to use pgx, a popular Go PostgreSQL driver, to work with JSON/JSONB columns effectively.
Understanding JSON vs JSONB in PostgreSQL
Before diving into the code, it’s important to understand the difference between JSON and JSONB columns:
- JSON: Stores data in text format, preserving whitespace and key order
- JSONB: Stores data in a decomposed binary format, which is more efficient for processing but doesn’t preserve formatting
JSONB is generally preferred as it offers better performance for querying and indexing.
Setting Up Our Environment
First, let’s set up our project with the necessary dependencies:
go get github.com/jackc/pgx/v4
Working with JSON Data
Let’s look at some common scenarios for working with JSON data using pgx:
1. Storing JSON Data
type User struct { ID int Profile map[string]interface{}}
func insertUser(ctx context.Context, conn *pgx.Conn, user User) error { _, err := conn.Exec(ctx, "INSERT INTO users (id, profile) VALUES ($1, $2)", user.ID, user.Profile) return err}
2. Querying JSON Fields
func getUserByInterest(ctx context.Context, conn *pgx.Conn, interest string) ([]User, error) { rows, err := conn.Query(ctx, "SELECT id, profile FROM users WHERE profile->>'interests' ? $1", interest) if err != nil { return nil, err } defer rows.Close()
var users []User // Process rows... return users, nil}
Best Practices and Tips
-
Use JSONB for Better Performance
- JSONB supports indexing
- Queries execute faster
- Takes slightly more storage space
-
Leverage PostgreSQL JSON Operators
->
: Gets JSON object field as JSON->>
: Gets JSON object field as text@>
: Contains operator?
: Key exists operator
-
Error Handling Always validate your JSON data before inserting:
if !json.Valid([]byte(jsonString)) { return errors.New("invalid JSON data")}
Conclusion
Working with JSON/JSONB in PostgreSQL through pgx provides a flexible and powerful way to handle complex data structures in your Go applications. By understanding the different operators and following best practices, you can build robust applications that efficiently manage JSON data.
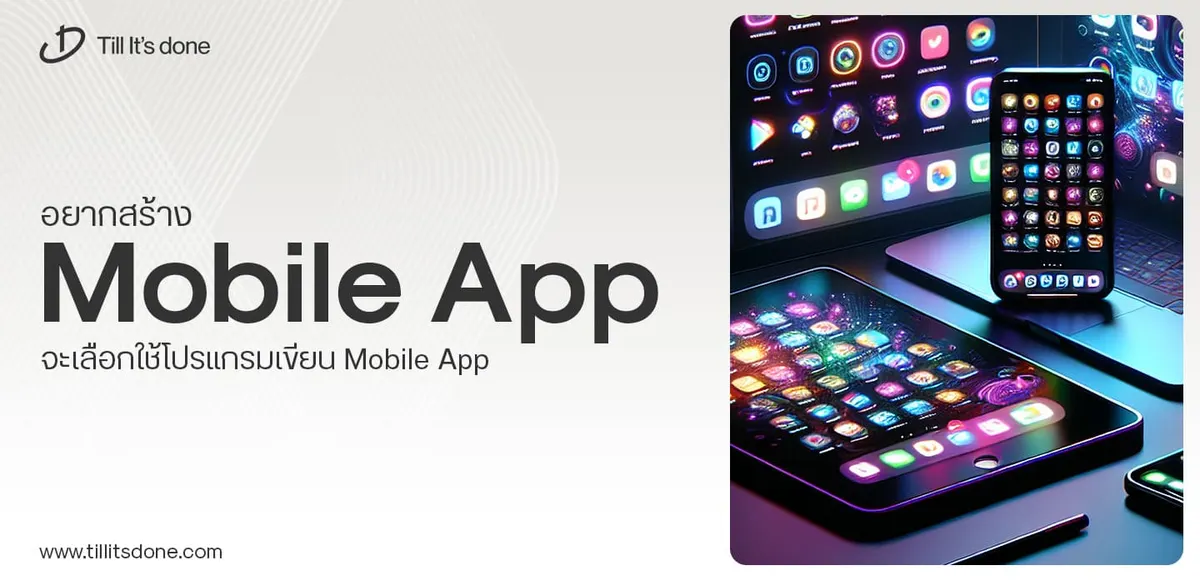
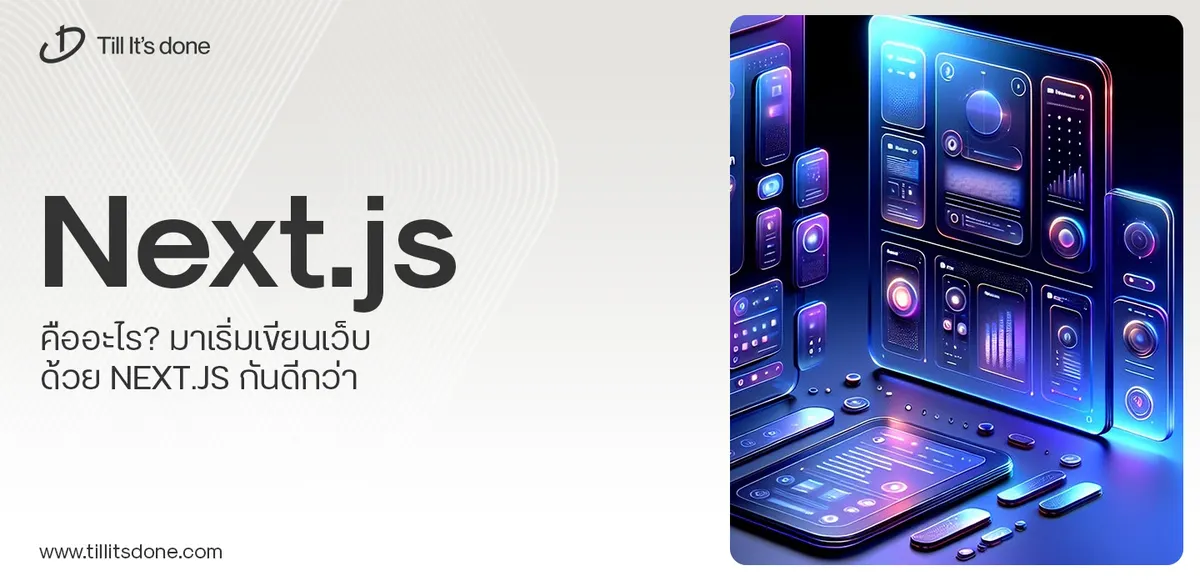
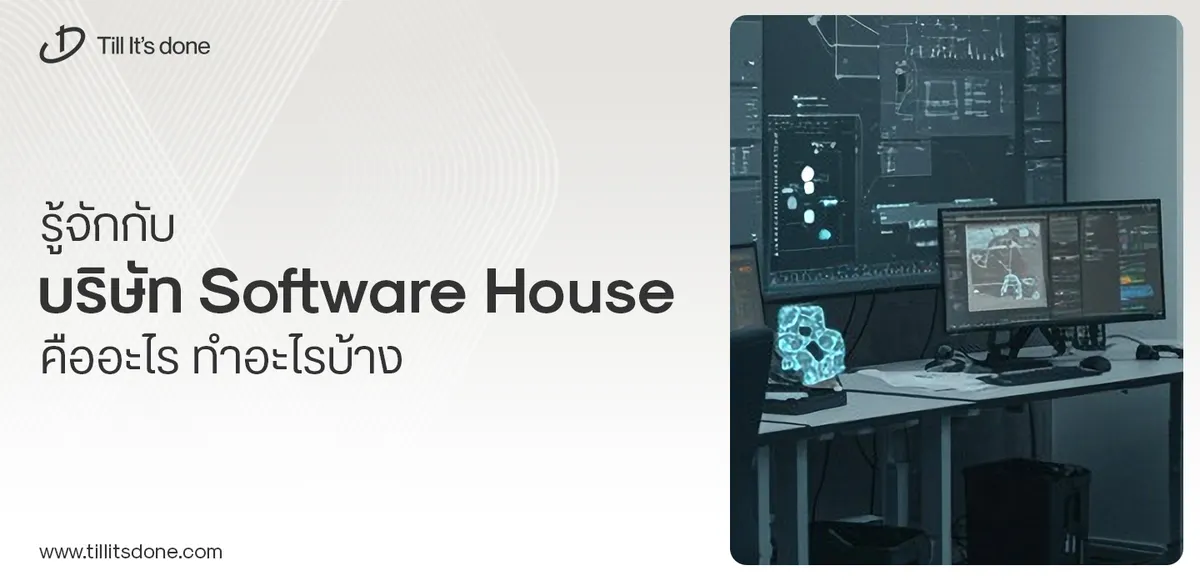
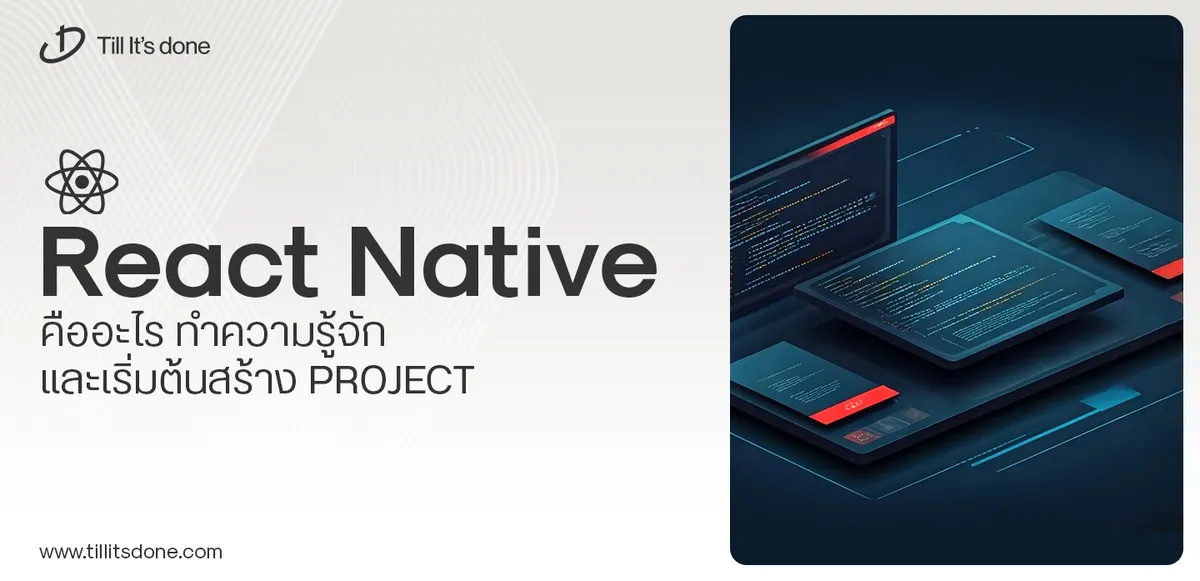
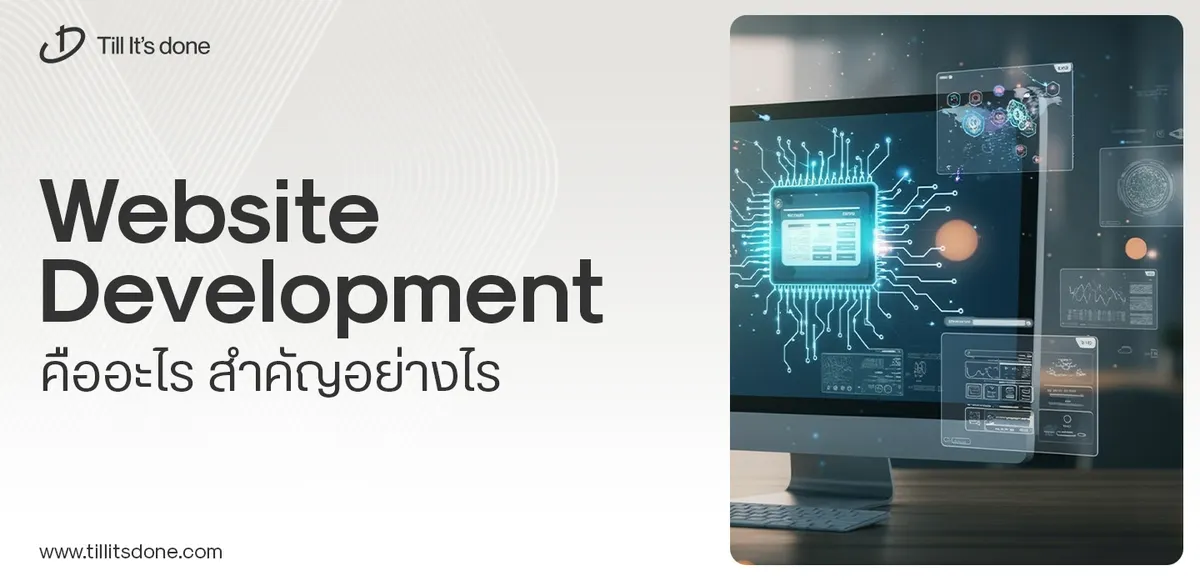
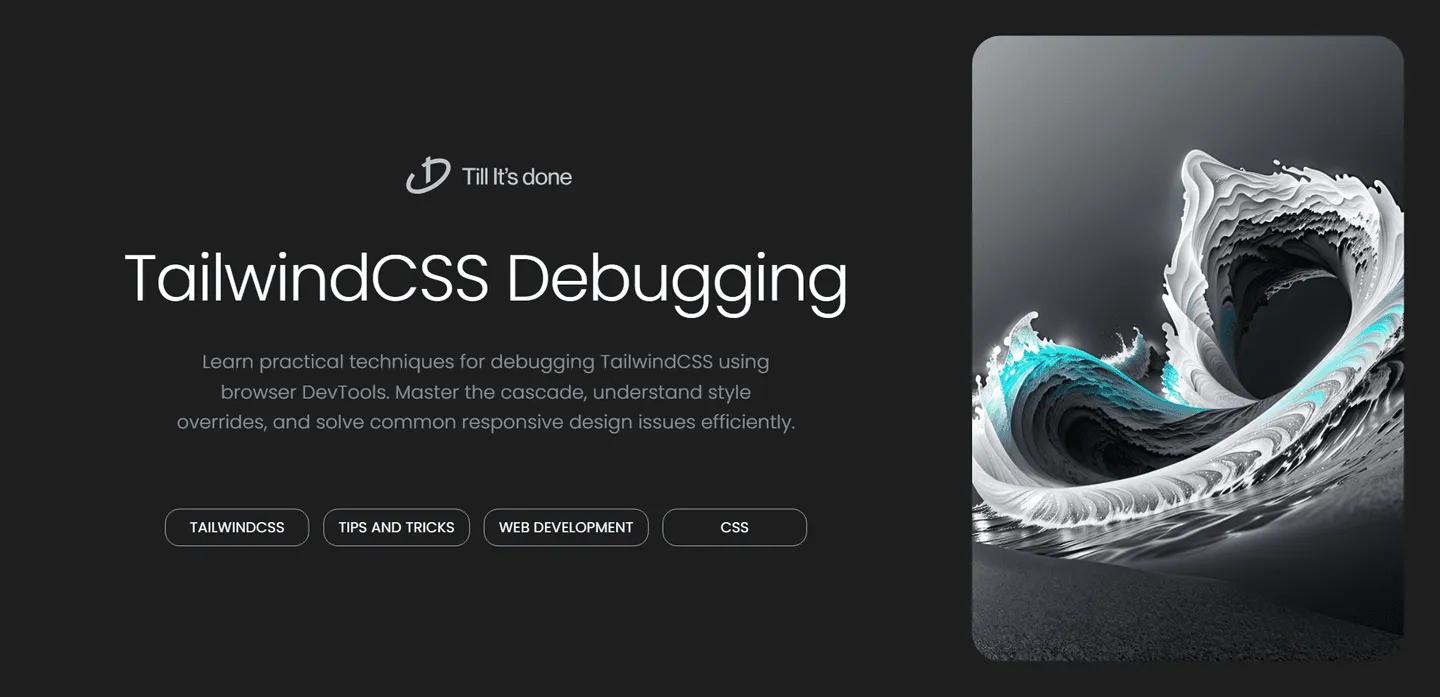
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.