- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Handling Database Transactions with PGX in Go
This guide covers basic and advanced transaction patterns, best practices, isolation levels, and error handling strategies.
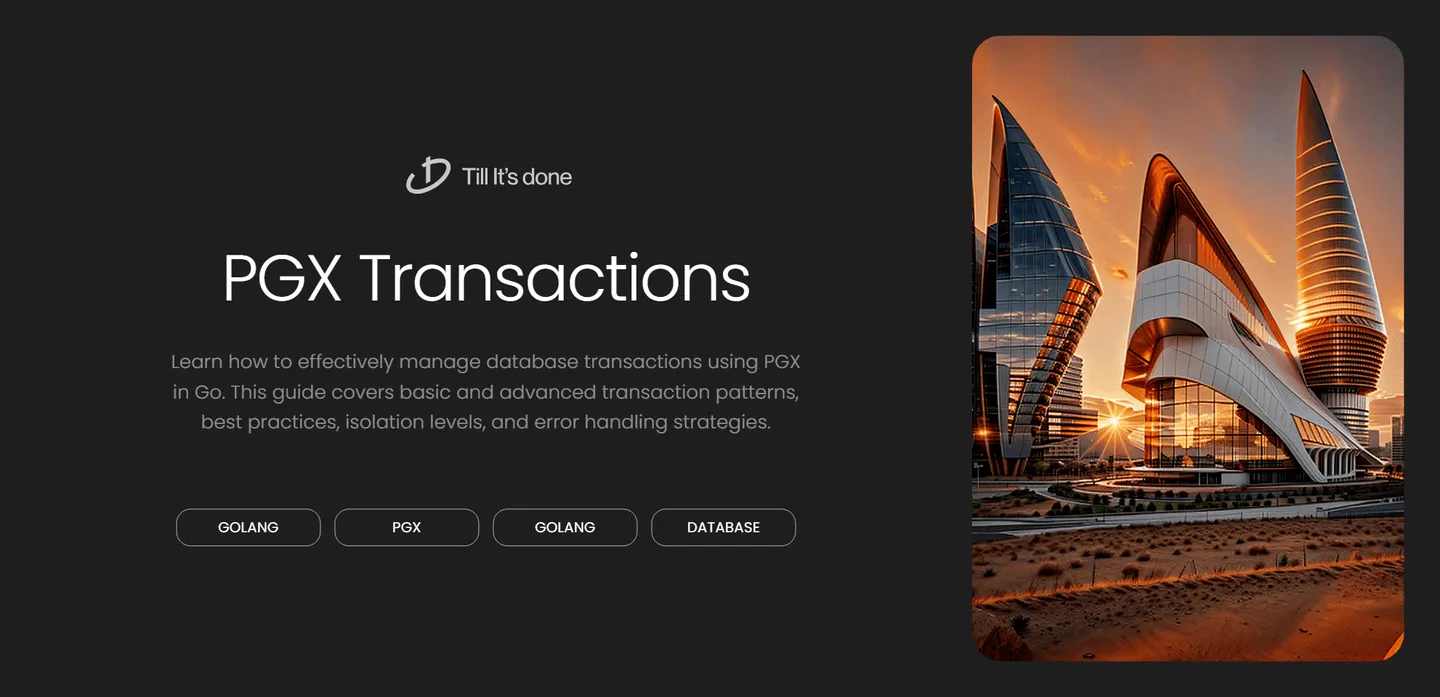
Handling Database Transactions Effectively with PGX in Go
Database transactions are crucial for maintaining data integrity in any application. When working with Go and PostgreSQL, PGX emerges as a powerful driver that provides robust transaction management capabilities. Let’s dive into how to handle database transactions effectively with PGX.
Understanding Transactions in PGX
At its core, a transaction represents a unit of work that must be completed entirely or not at all. PGX provides a clean and intuitive API for managing transactions in Go, making it easier to implement ACID-compliant operations in your applications.
Basic Transaction Handling
Here’s a simple example of how to use transactions with PGX:
func transferMoney(ctx context.Context, conn *pgx.Conn, fromAccount, toAccount string, amount float64) error { tx, err := conn.BeginTx(ctx, pgx.TxOptions{}) if err != nil { return fmt.Errorf("starting transaction: %w", err) } // Defer a rollback in case anything fails defer tx.Rollback(ctx)
// Perform the transfer operations if _, err := tx.Exec(ctx, "UPDATE accounts SET balance = balance - $1 WHERE id = $2", amount, fromAccount); err != nil { return fmt.Errorf("deducting amount: %w", err) }
if _, err := tx.Exec(ctx, "UPDATE accounts SET balance = balance + $1 WHERE id = $2", amount, toAccount); err != nil { return fmt.Errorf("adding amount: %w", err) }
// Commit the transaction if err := tx.Commit(ctx); err != nil { return fmt.Errorf("committing transaction: %w", err) }
return nil}
Advanced Transaction Patterns
Using SavePoints
PGX supports savepoints, allowing you to create checkpoints within a transaction:
func processBatchWithSavepoints(ctx context.Context, tx pgx.Tx) error { for i, item := range items { // Create a savepoint before processing each item savepoint := fmt.Sprintf("sv_%d", i) if _, err := tx.Exec(ctx, "SAVEPOINT "+savepoint); err != nil { return err }
if err := processItem(ctx, tx, item); err != nil { // Rollback to savepoint if processing fails _, rbErr := tx.Exec(ctx, "ROLLBACK TO SAVEPOINT "+savepoint) if rbErr != nil { return rbErr } continue }
// Release savepoint if successful if _, err := tx.Exec(ctx, "RELEASE SAVEPOINT "+savepoint); err != nil { return err } } return nil}
Best Practices
- Always use contexts for timeout management
- Implement proper error handling and rollback mechanisms
- Keep transactions as short as possible
- Use appropriate isolation levels
- Consider implementing retry logic for transient failures
Transaction Isolation Levels
PGX supports all PostgreSQL isolation levels:
tx, err := conn.BeginTx(ctx, pgx.TxOptions{ IsoLevel: pgx.Serializable,})
The available isolation levels are:
- ReadCommitted (default)
- RepeatableRead
- Serializable
Error Handling and Recovery
Implementing robust error handling is crucial:
func executeTransaction(ctx context.Context, conn *pgx.Conn) error { tx, err := conn.BeginTx(ctx, pgx.TxOptions{}) if err != nil { return fmt.Errorf("begin transaction: %w", err) }
defer func() { if err := recover(); err != nil { tx.Rollback(ctx) panic(err) // re-panic after rollback } }()
// Transaction operations here...
if err := tx.Commit(ctx); err != nil { return fmt.Errorf("commit transaction: %w", err) } return nil}
Remember that effective transaction management is key to building reliable applications. PGX provides all the tools you need to implement robust transaction handling in your Go applications.
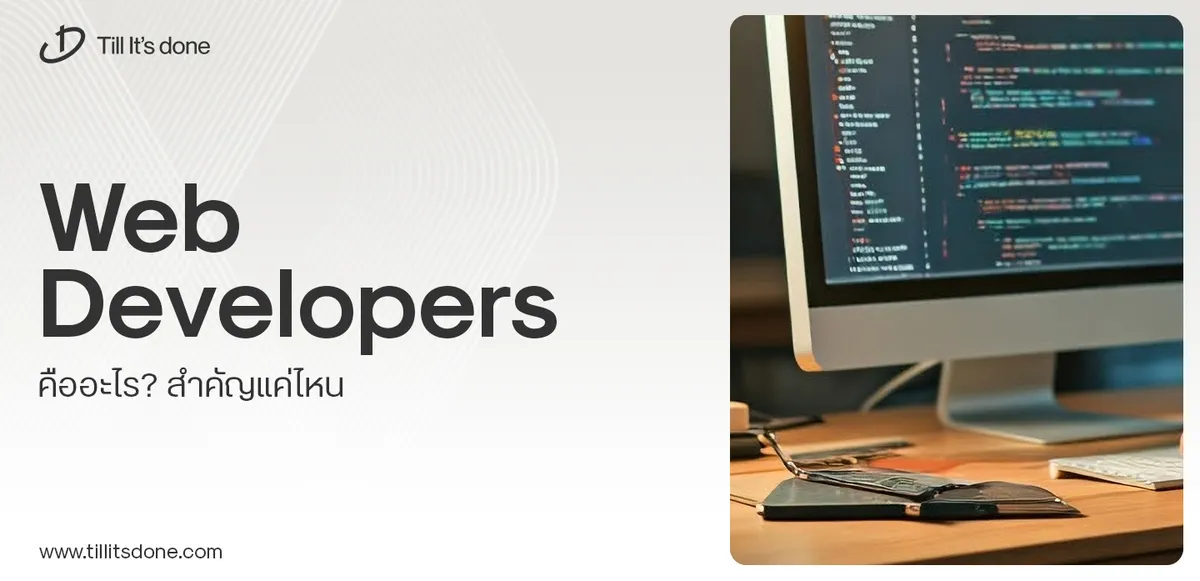
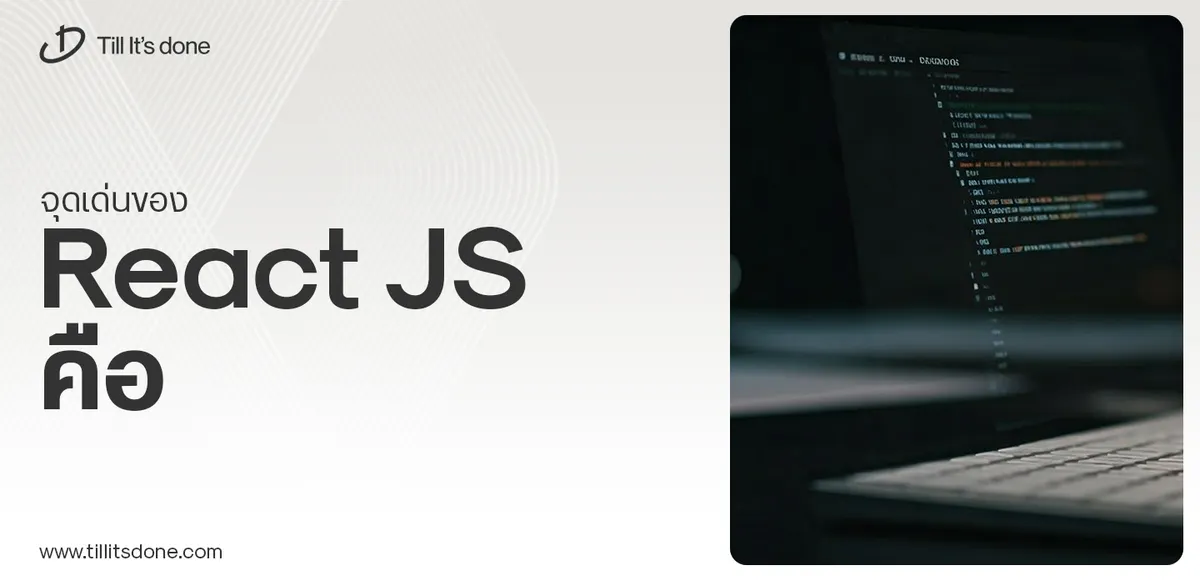
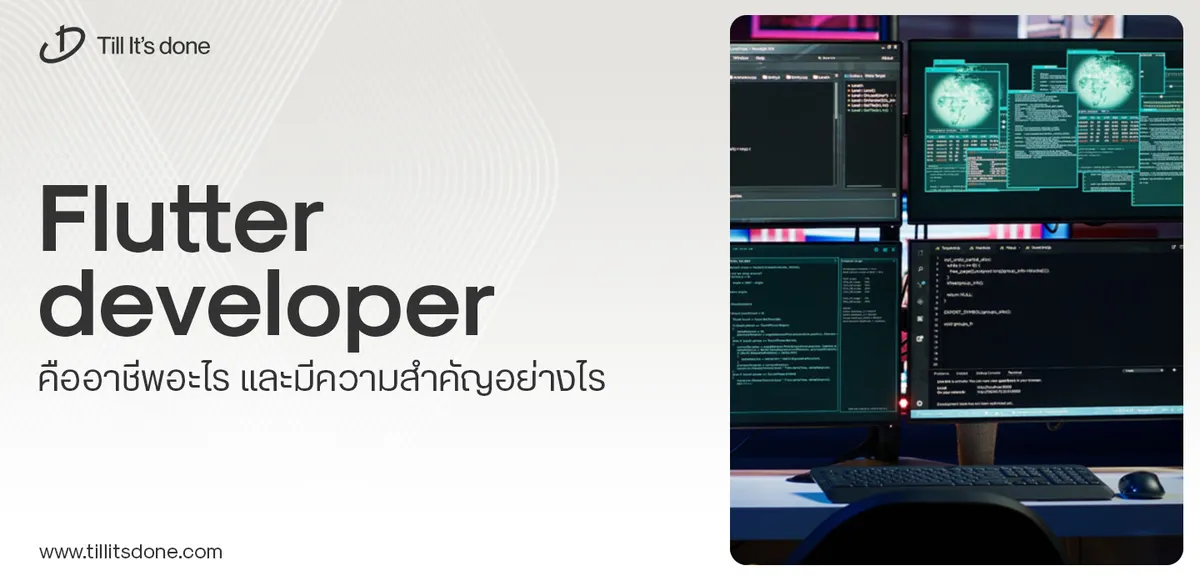
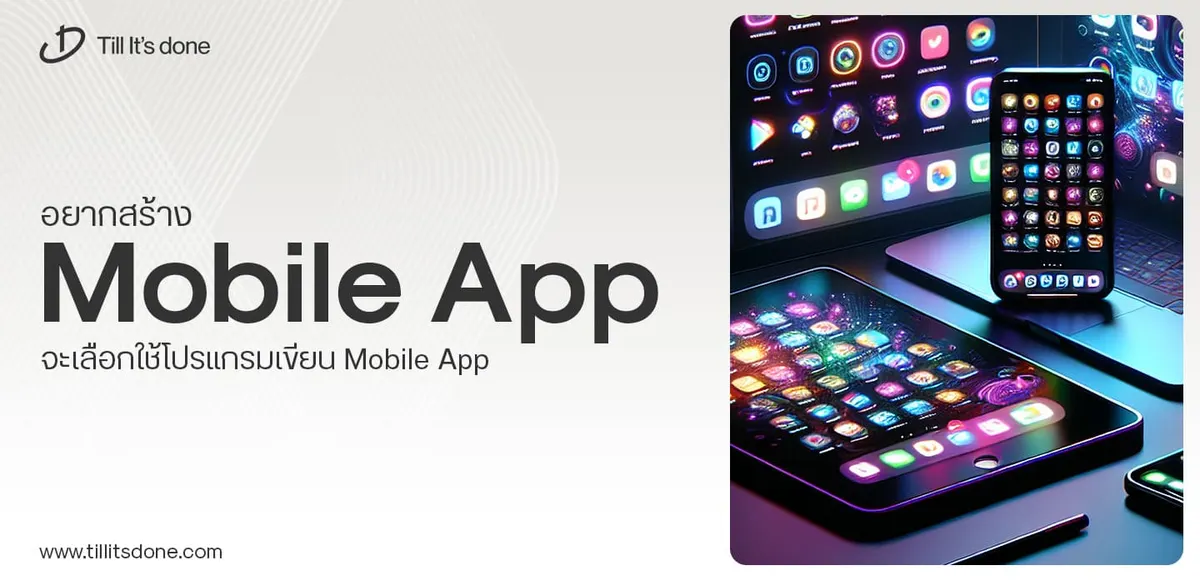
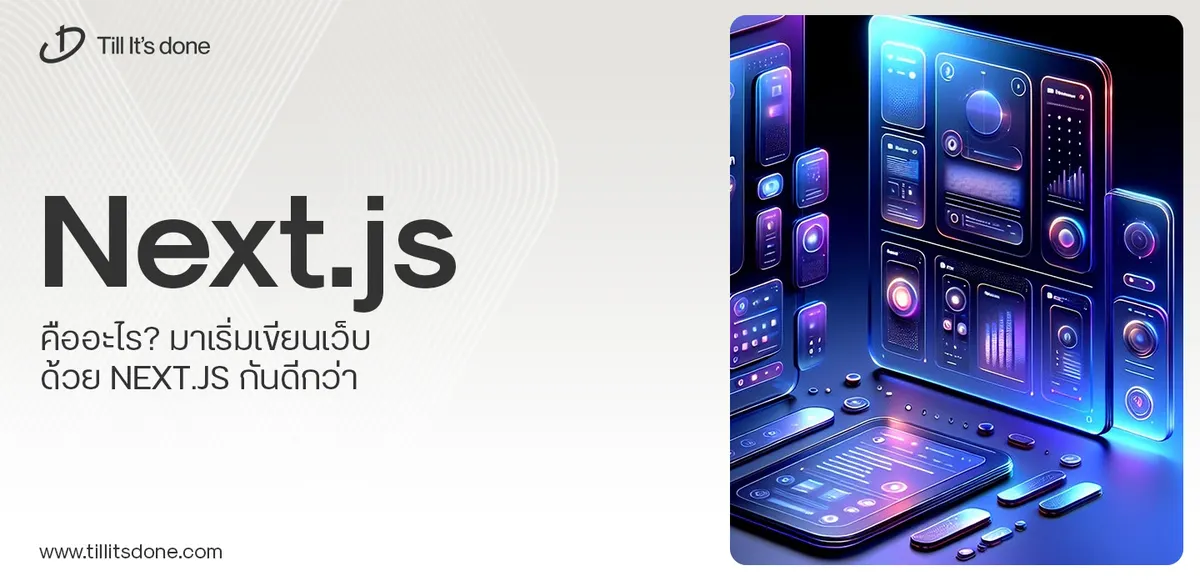
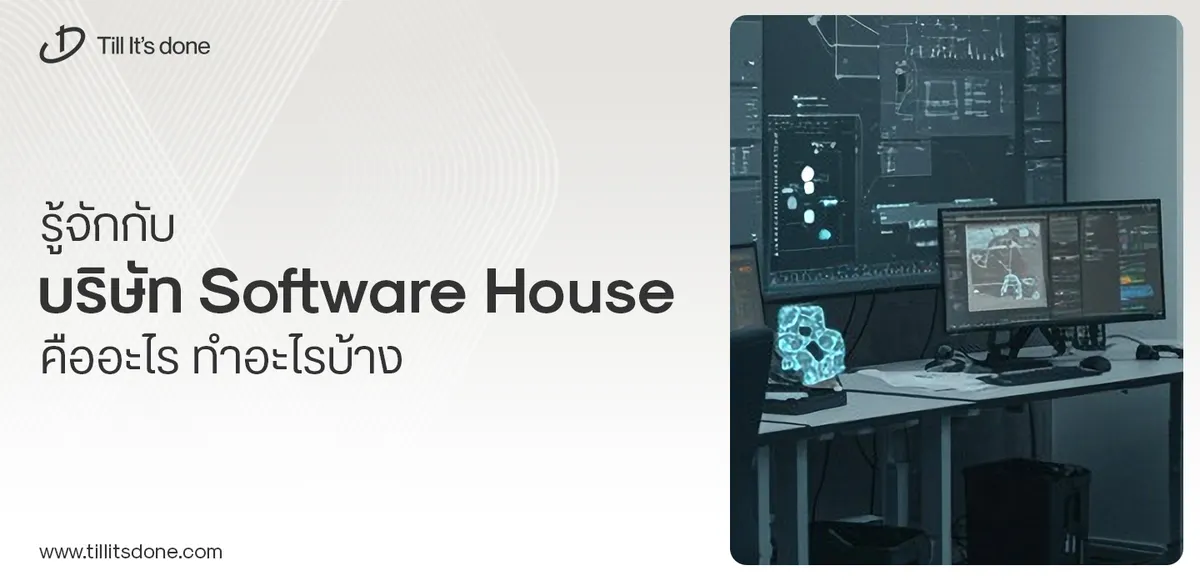
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.