- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Error Handling Patterns in PGX for Go Apps
Discover best practices for managing database errors, implementing retry logic, and creating resilient systems.

Error Handling Patterns in PGX for Robust Go Applications
Effective error handling is crucial for building resilient PostgreSQL applications in Go. When working with the popular PGX library, implementing proper error handling patterns can mean the difference between a production-ready application and one that fails unpredictably. Let’s dive into some battle-tested patterns for handling database errors in your Go applications.
Understanding PGX Error Types
PGX provides several specific error types that help us handle database-related issues more gracefully. Instead of treating all errors as generic ones, PGX allows us to make informed decisions based on the specific error type we encounter.
Common Error Patterns
if err := tx.Commit(context.Background()); err != nil { if pgErr, ok := err.(*pgconn.PgError); ok { switch pgErr.Code { case "23505": // unique_violation return fmt.Errorf("duplicate record: %v", err) case "23503": // foreign_key_violation return fmt.Errorf("invalid reference: %v", err) } } return fmt.Errorf("commit failed: %v", err)}
Best Practices for Error Handling
- Use Context Timeouts
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)defer cancel()
if err := conn.QueryRow(ctx, "SELECT...").Scan(&result); err != nil { if errors.Is(err, context.DeadlineExceeded) { log.Error("query timeout exceeded") return nil, ErrDatabaseTimeout } return nil, fmt.Errorf("failed to execute query: %w", err)}
- Implement Retry Logic
func queryWithRetry(ctx context.Context, db *pgxpool.Pool, query string) error { backoff := time.Second for attempts := 0; attempts < 3; attempts++ { err := db.QueryRow(ctx, query).Scan() if err == nil { return nil }
if pgErr, ok := err.(*pgconn.PgError); ok && pgErr.Code == "40001" { time.Sleep(backoff) backoff *= 2 continue } return err } return errors.New("max retry attempts reached")}
Advanced Error Handling Patterns
Custom Error Types
Creating custom error types helps in providing more context and makes error handling more maintainable:
type DatabaseError struct { Operation string Err error}
func (e *DatabaseError) Error() string { return fmt.Sprintf("database error during %s: %v", e.Operation, e.Err)}
func (e *DatabaseError) Unwrap() error { return e.Err}
Transaction Error Handling
Always ensure your transactions are properly handled with deferred rollbacks:
tx, err := pool.Begin(ctx)if err != nil { return fmt.Errorf("failed to begin transaction: %w", err)}defer tx.Rollback(ctx) // will be no-op if tx.Commit() is called
// ... transaction operations ...
if err := tx.Commit(ctx); err != nil { return fmt.Errorf("failed to commit transaction: %w", err)}
Monitoring and Logging
Implement comprehensive logging for database errors to help with debugging and monitoring:
if err := row.Scan(&result); err != nil { logger.With( "query", query, "error", err, "timestamp", time.Now(), ).Error("failed to scan row") return nil, fmt.Errorf("failed to scan row: %w", err)}
Remember that proper error handling is not just about catching errors – it’s about making your application resilient and maintainable. By implementing these patterns, you’ll build more robust applications that can handle database errors gracefully and provide better reliability for your users.
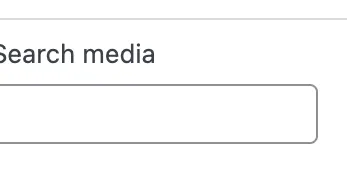





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.