- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Migrate from SQL Driver to PGX in Golang
Learn step-by-step migration, best practices, and key benefits.
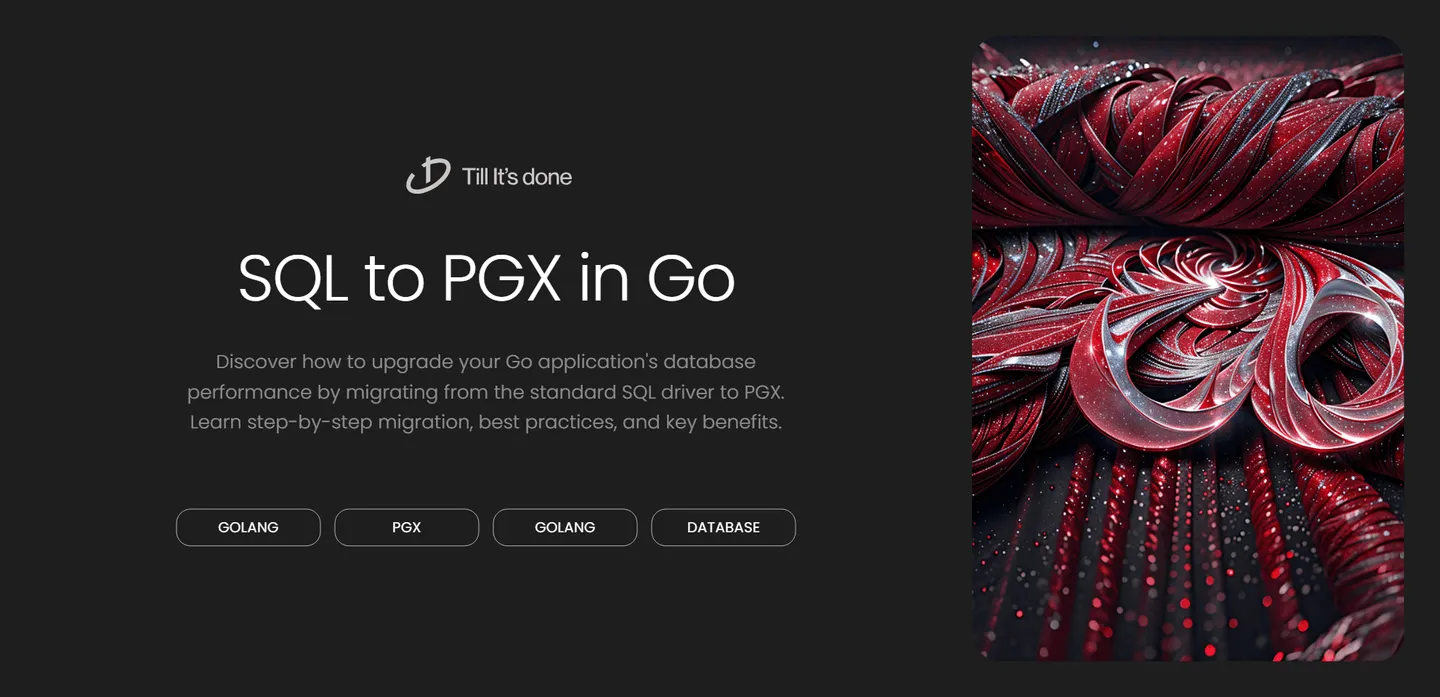
Migrating from Standard SQL Driver to PGX in Golang: A Journey to Better Database Performance
Are you still using the standard database/sql package with lib/pq for your PostgreSQL operations in Go? While it’s served us well, there’s a more powerful alternative that could significantly improve your application’s performance: pgx. Let’s explore why and how to make this transition.
Why Consider PGX?
The journey from the standard SQL driver to pgx is like upgrading from a reliable sedan to a high-performance sports car. PGX brings several compelling advantages:
- Better performance with optimized PostgreSQL-specific protocols
- Native support for more PostgreSQL data types
- Built-in connection pooling
- Automatic prepared statement caching
- Batch operations support
- Enhanced error handling
Making the Switch: Step-by-Step Migration
1. Update Dependencies
First, let’s update our dependencies. Remove the old one and bring in the new:
// Remove this_ "github.com/lib/pq"
// Add these"github.com/jackc/pgx/v4""github.com/jackc/pgx/v4/pgxpool"
2. Connection String Updates
PGX uses a slightly different connection string format. Here’s how to modify it:
// Old style// "postgres://username:password@localhost:5432/dbname?sslmode=disable"
// New style// "postgres://username:password@localhost:5432/dbname"
3. Establishing Connections
The connection setup changes from using sql.Open to pgxpool.Connect:
// Beforedb, err := sql.Open("postgres", connString)
// Afterpool, err := pgxpool.Connect(context.Background(), connString)
4. Updating Queries
Most of your existing queries will work with minimal changes. The main difference is the need for context:
// Beforerows, err := db.Query("SELECT * FROM users WHERE active = $1", true)
// Afterrows, err := pool.Query(context.Background(), "SELECT * FROM users WHERE active = $1", true)
5. Handling Transactions
Transactions get a slight syntax update but become more straightforward:
// Using pgxtx, err := pool.Begin(context.Background())if err != nil { return err}defer tx.Rollback(context.Background())
// Perform transactionserr = tx.Commit(context.Background())
Best Practices and Tips
- Always use connection pooling for better performance
- Leverage batch operations for multiple queries
- Take advantage of pgx’s native PostgreSQL type support
- Use context timeouts for better control over query execution
- Consider using pgx’s copy protocol for bulk insertions
Remember to test thoroughly in a staging environment before deploying to production. The switch to pgx might reveal edge cases in your current code that need attention.
The migration to pgx might seem daunting at first, but the performance benefits and enhanced features make it worth the effort. Take it step by step, and you’ll soon be enjoying a more robust and efficient database interaction in your Go applications.
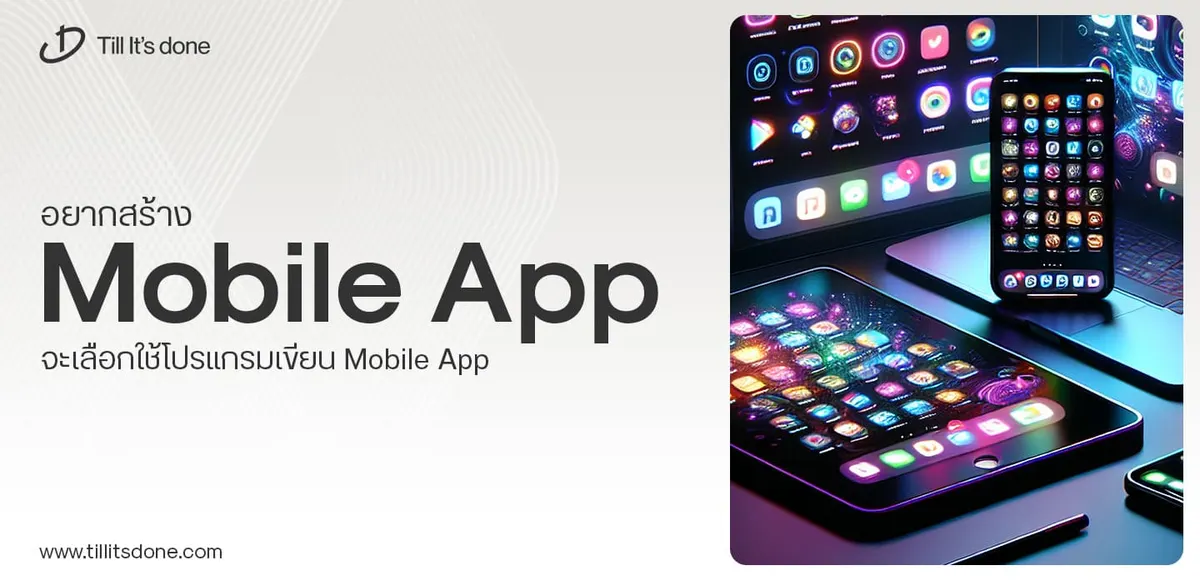
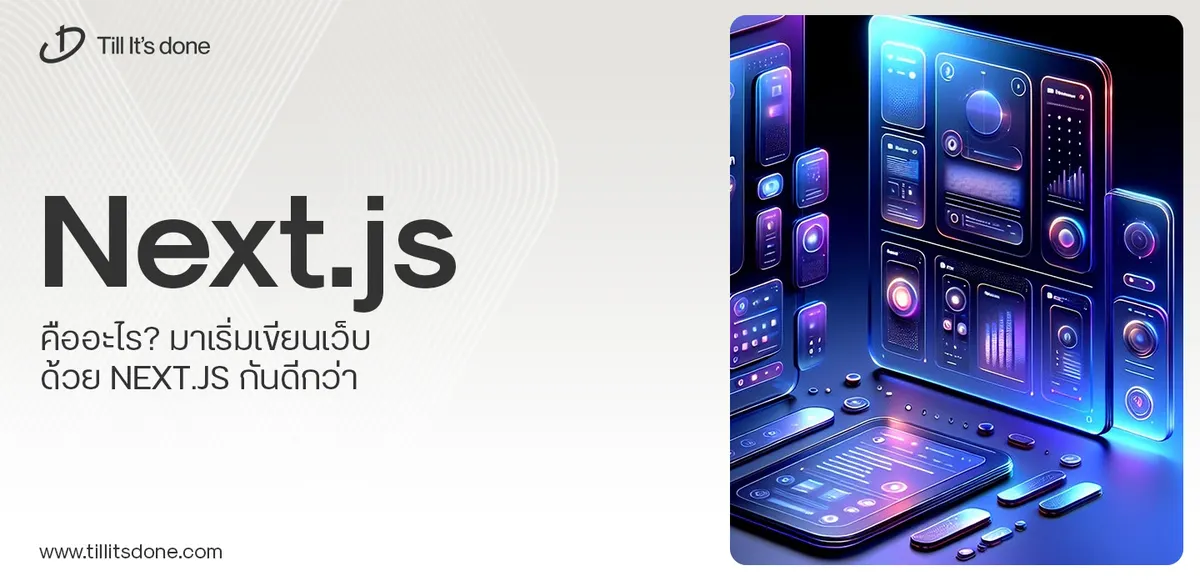
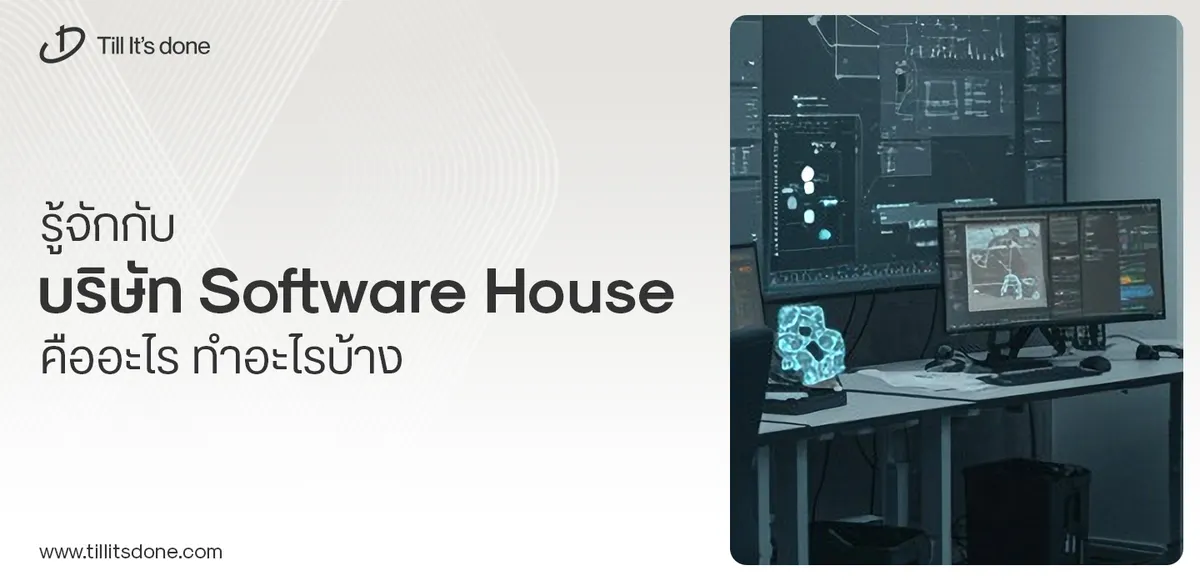
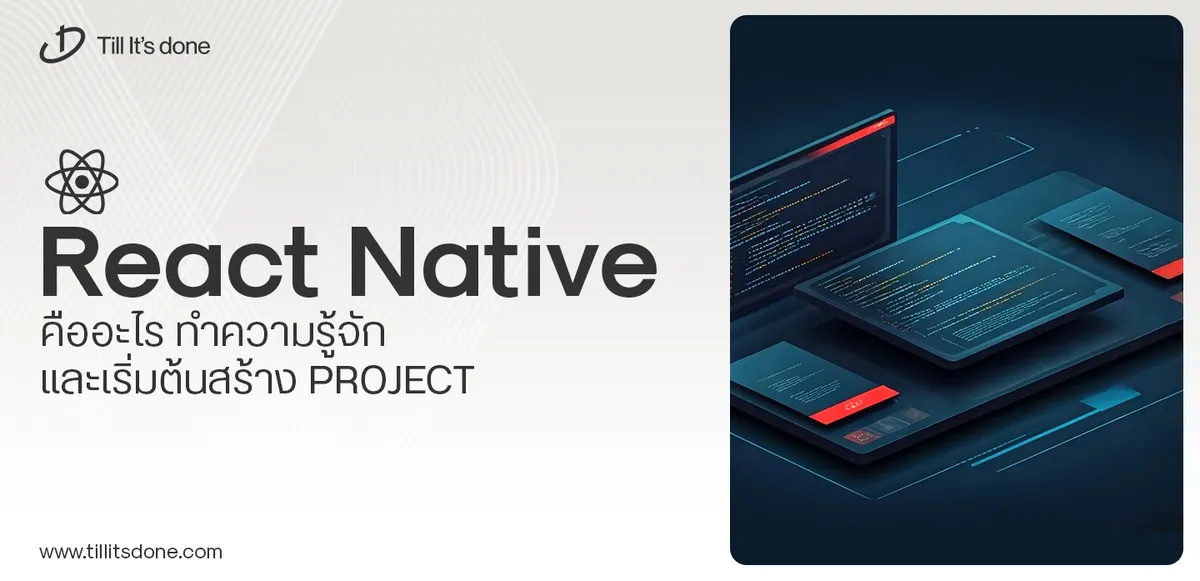
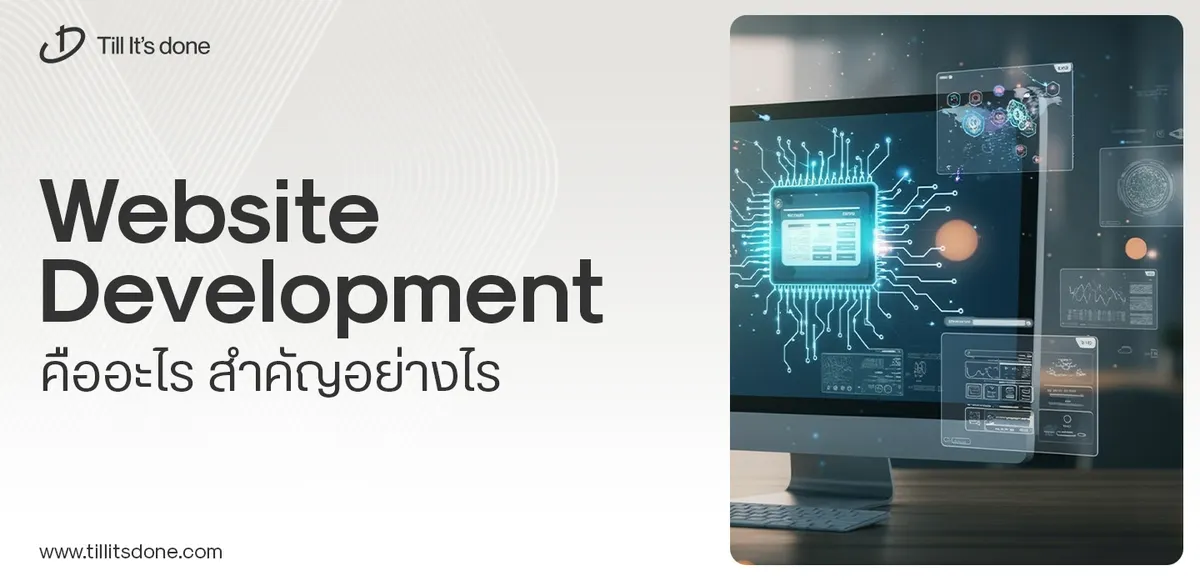
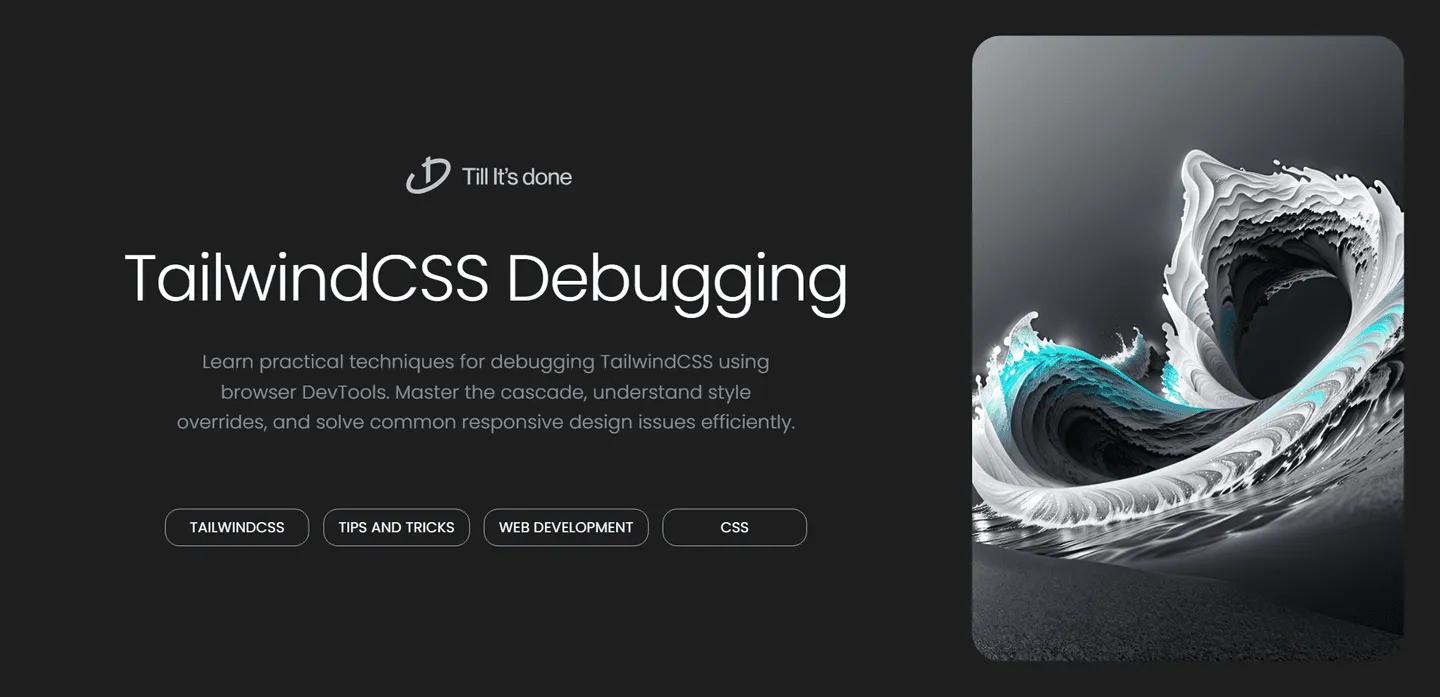
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.