- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Performance Tuning for PGX Queries in Golang
Discover practical tips on connection pooling, batch operations, prepared statements, and memory management.
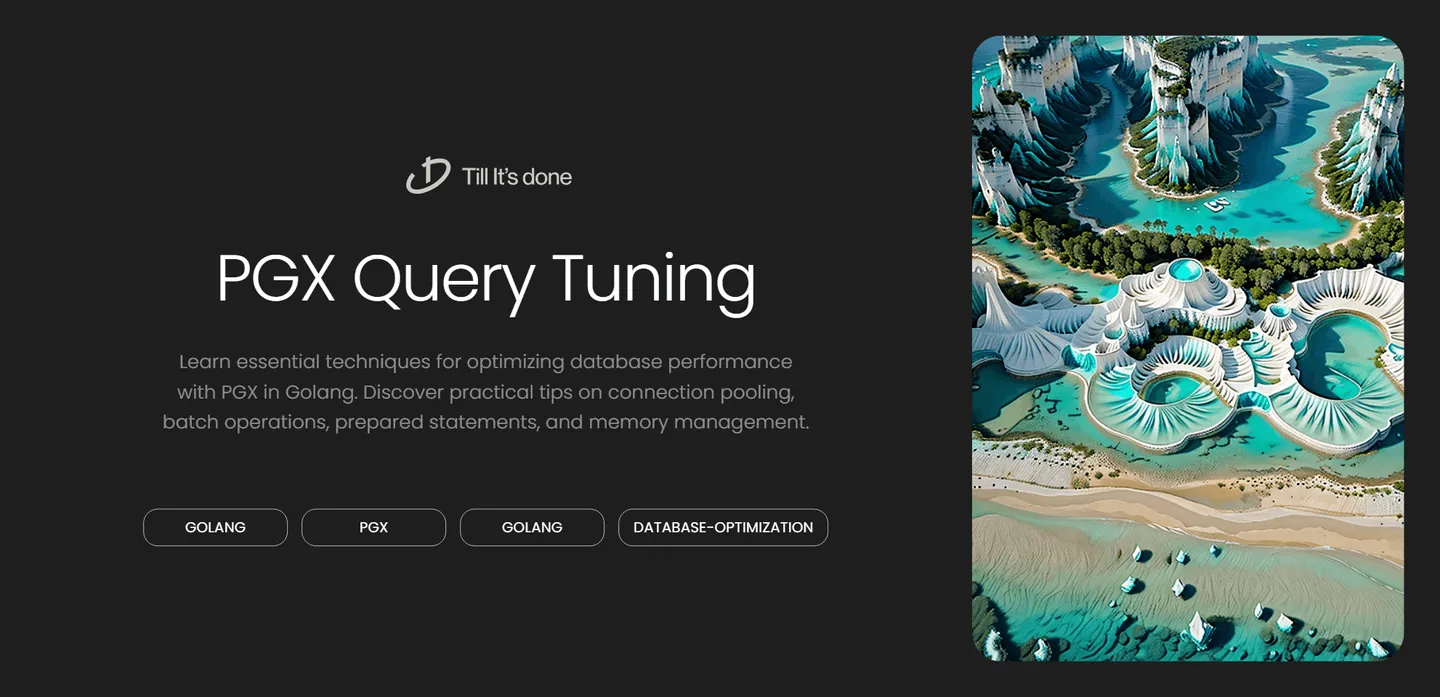
Performance Tuning for PGX Queries in Golang
When building high-performance applications in Go, database interaction often becomes a critical bottleneck. PGX, a popular PostgreSQL driver for Go, offers numerous optimization opportunities that can significantly boost your application’s performance. Let’s dive into some practical techniques for tuning PGX queries and maximizing your database efficiency.
Understanding Connection Pooling
Connection pooling is your first line of defense against performance issues. Instead of creating new connections for each request, PGX maintains a pool of reusable connections. Here’s how to configure it optimally:
pool, err := pgxpool.New(context.Background(), "postgres://username:password@localhost:5432/dbname")if err != nil { panic(err)}defer pool.Close()
pool.Config().MaxConns = 10pool.Config().MinConns = 2
The key is finding the right balance for your specific use case. Too many connections can overwhelm your database, while too few can create bottlenecks.
Batch Operations and Copy Protocol
For bulk operations, PGX’s copy protocol implementation can be a game-changer:
copyCount, err := pool.CopyFrom( context.Background(), pgx.Identifier{"users"}, []string{"id", "name", "email"}, pgx.CopyFromRows(rows),)
This approach can be up to 5x faster than individual inserts, especially when dealing with large datasets.
Prepared Statements: Use Them Wisely
While prepared statements can improve performance by caching query plans, they’re not always the best choice:
// Good for repeated queriesstmt, err := pool.Prepare(context.Background(), "user_by_id", "SELECT * FROM users WHERE id = $1")
Remember that prepared statements consume server resources, so use them primarily for frequently executed queries.
Query Batching with Send/Recv
When you need to execute multiple independent queries, batching them can reduce network roundtrips:
batch := &pgx.Batch{}batch.Queue("SELECT * FROM users WHERE id = $1", 1)batch.Queue("SELECT * FROM orders WHERE user_id = $1", 1)
br := pool.SendBatch(context.Background(), batch)defer br.Close()
Monitoring and Optimization Tips
- Use pgx.QueryTracer to monitor query performance
- Implement appropriate timeouts for long-running queries
- Consider using pgx’s built-in metrics collection
- Regular EXPLAIN ANALYZE to identify query bottlenecks
Memory Management
Be mindful of how you handle large result sets:
rows, err := pool.Query(context.Background(), "SELECT * FROM large_table")if err != nil { panic(err)}defer rows.Close()
Always close your rows to prevent memory leaks, and consider using cursors for very large result sets.
Conclusion
Performance tuning is an iterative process that requires careful monitoring and adjustment. By implementing these techniques thoughtfully, you can significantly improve your application’s database performance with PGX.
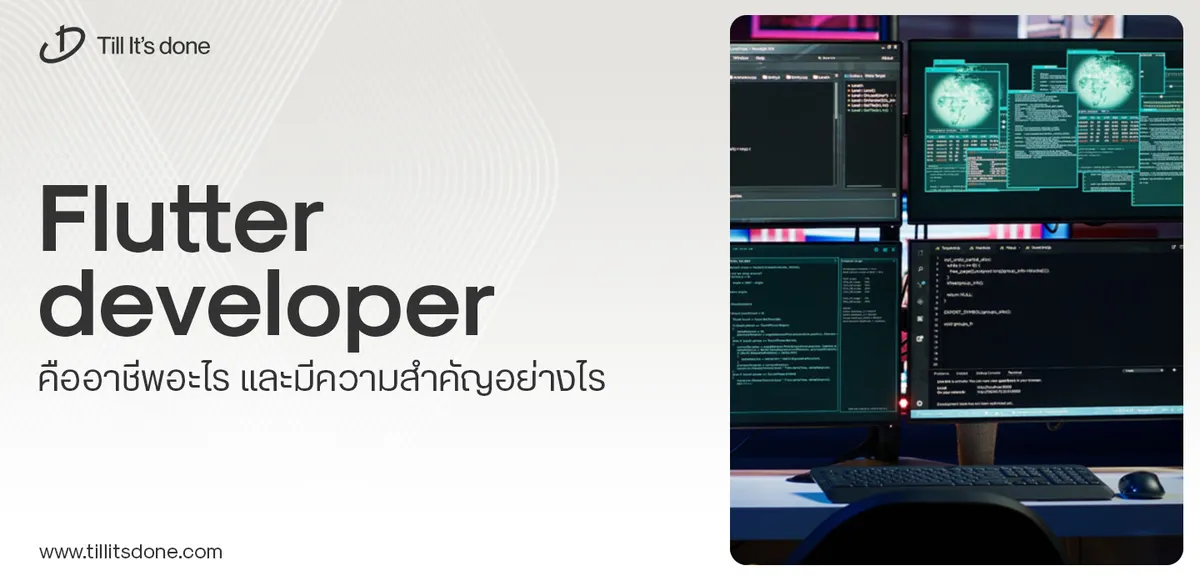
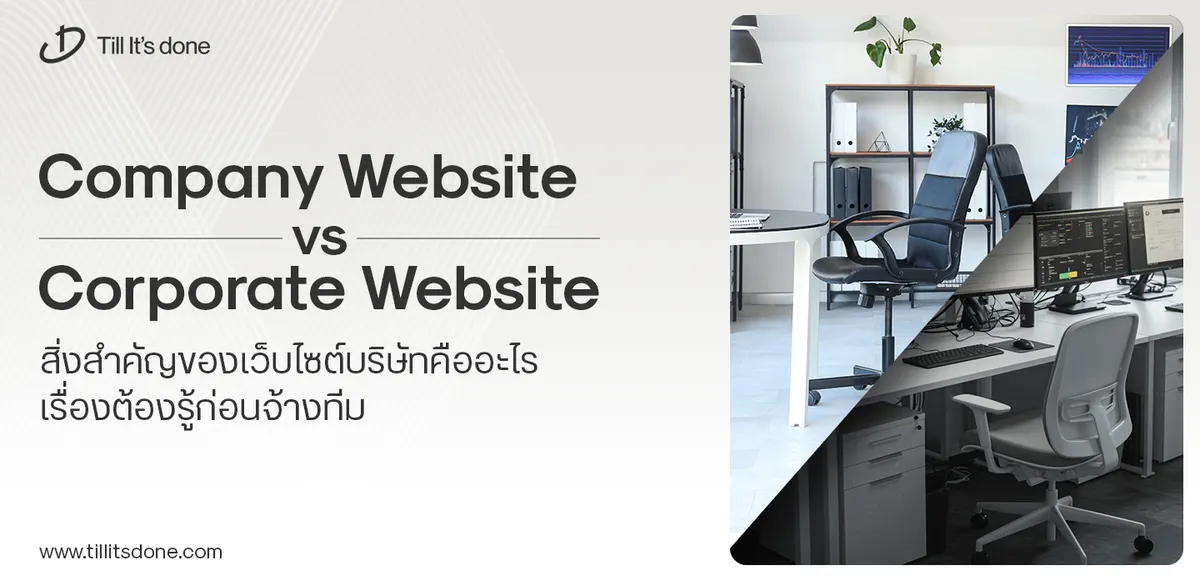
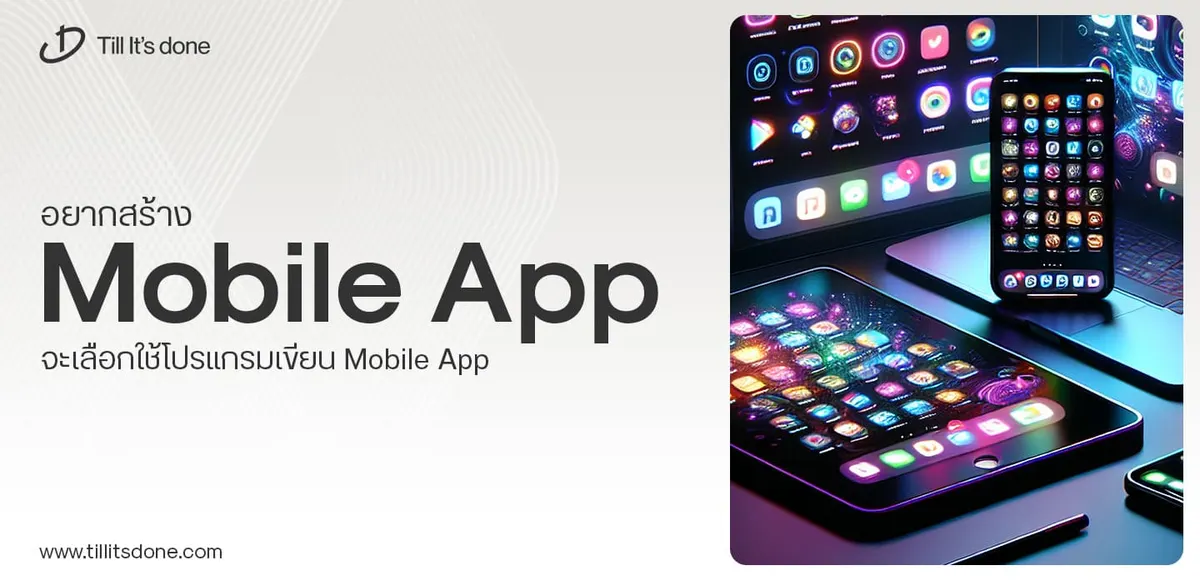
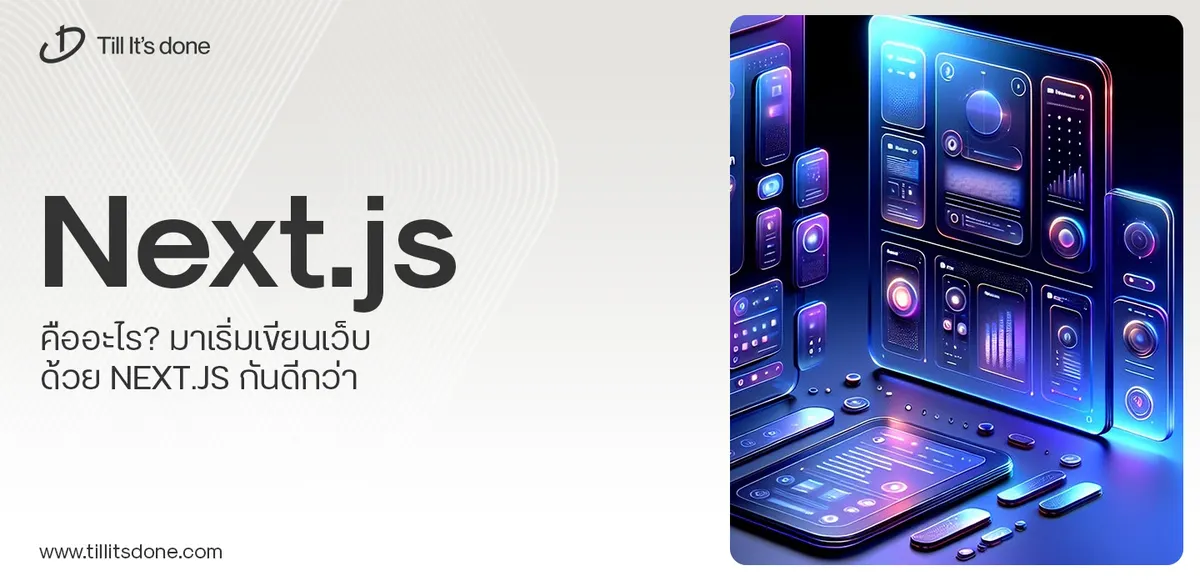
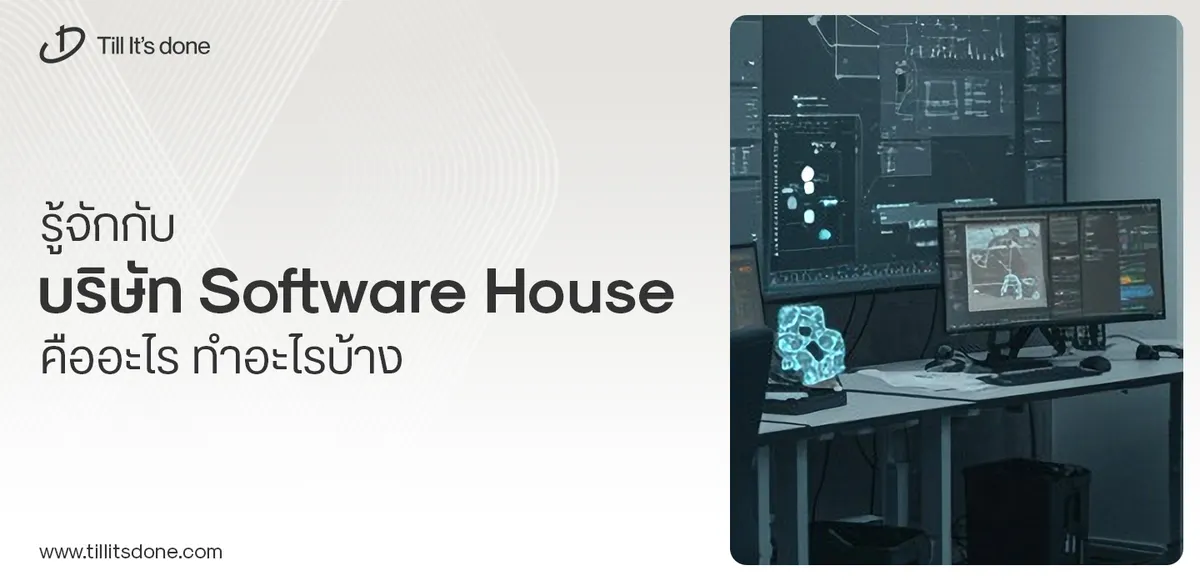
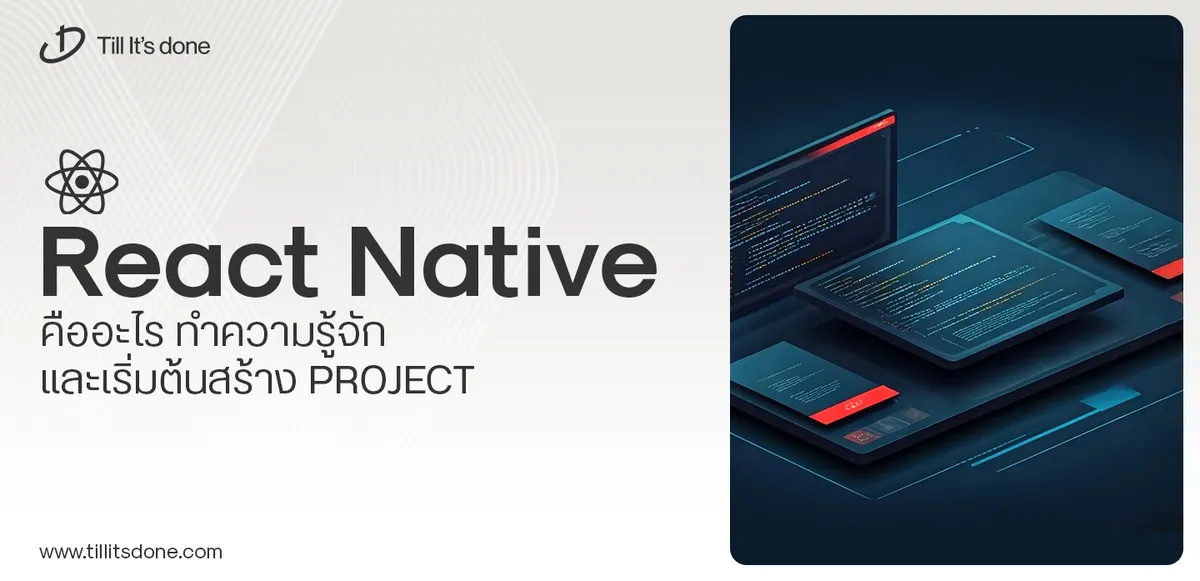
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.