- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Advanced Query Optimization with PGX in Go
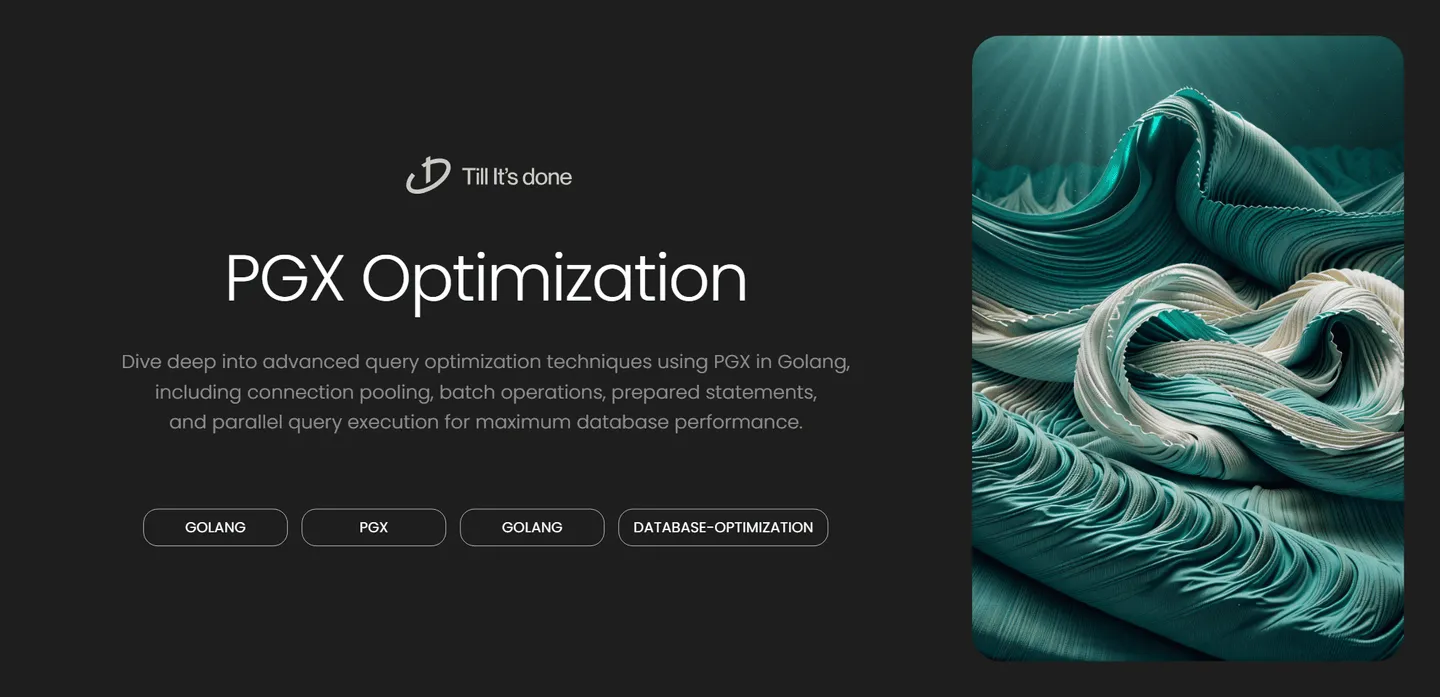
Advanced Query Optimization Techniques Using PGX in Golang
In the world of Go database programming, performance is everything. While PGX is already one of the most efficient PostgreSQL drivers available, knowing how to optimize your queries can make the difference between a sluggish application and one that flies. Let’s dive into some advanced techniques that can help you squeeze every ounce of performance from your database operations.
Understanding PGX Connection Pooling
One of the most crucial aspects of database performance is proper connection management. PGX provides a robust connection pooling mechanism, but it needs to be configured correctly to achieve optimal results.
pool, err := pgxpool.New(context.Background(), "postgres://username:password@localhost:5432/dbname?pool_max_conns=10")if err != nil { log.Fatal(err)}defer pool.Close()
The key is finding the right balance in your connection pool settings. Too many connections can overwhelm your database, while too few can create bottlenecks in your application.
Batch Operations for Better Performance
When dealing with multiple similar queries, batching can significantly improve performance:
batch := &pgx.Batch{}for _, id := range ids { batch.Queue("SELECT name, email FROM users WHERE id = $1", id)}
results := pool.SendBatch(context.Background(), batch)defer results.Close()
Prepared Statements: The Hidden Gem
Prepared statements are your secret weapon for queries that run frequently:
stmt, err := pool.Prepare(context.Background(), "user_by_id", "SELECT * FROM users WHERE id = $1")if err != nil { log.Fatal(err)}
// Later in your coderow := pool.QueryRow(context.Background(), "user_by_id", 123)
Advanced Techniques for Complex Queries
Parallel Query Execution
When dealing with large datasets, parallel query execution can be a game-changer:
_, err := pool.Exec(context.Background(), "SET max_parallel_workers_per_gather = 4")
Using CopyFrom for Bulk Inserts
For large-scale data insertions, CopyFrom
is significantly faster than individual inserts:
copyCount, err := pool.CopyFrom( context.Background(), pgx.Identifier{"users"}, []string{"id", "name", "email"}, pgx.CopyFromRows(rows),)
Monitoring and Optimization
Remember to use PGX’s built-in logging and metrics capabilities to identify bottlenecks:
config, err := pgxpool.ParseConfig(connectionString)config.ConnConfig.Logger = logrusadapter.NewLogger(logrus.New())
By implementing these optimization techniques, you can significantly improve your application’s database performance. Remember that optimization is an iterative process - monitor, measure, and adjust based on your specific use case and requirements.
The key to successful query optimization isn’t just about implementing these techniques blindly, but understanding when and where to apply them. Start with the basics, measure your performance improvements, and gradually incorporate more advanced techniques as needed.
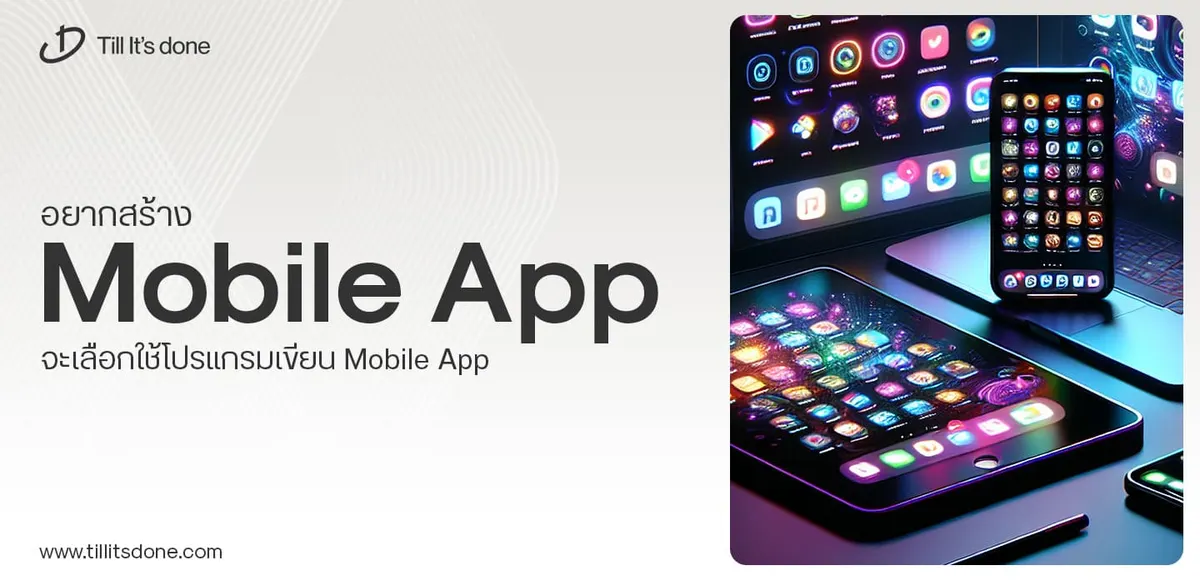
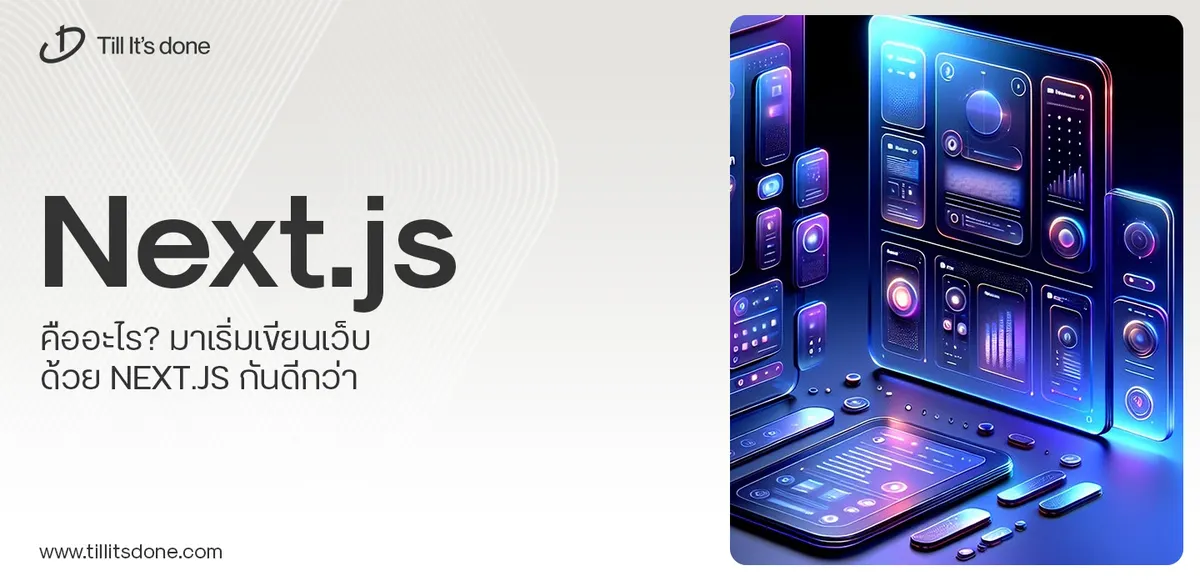
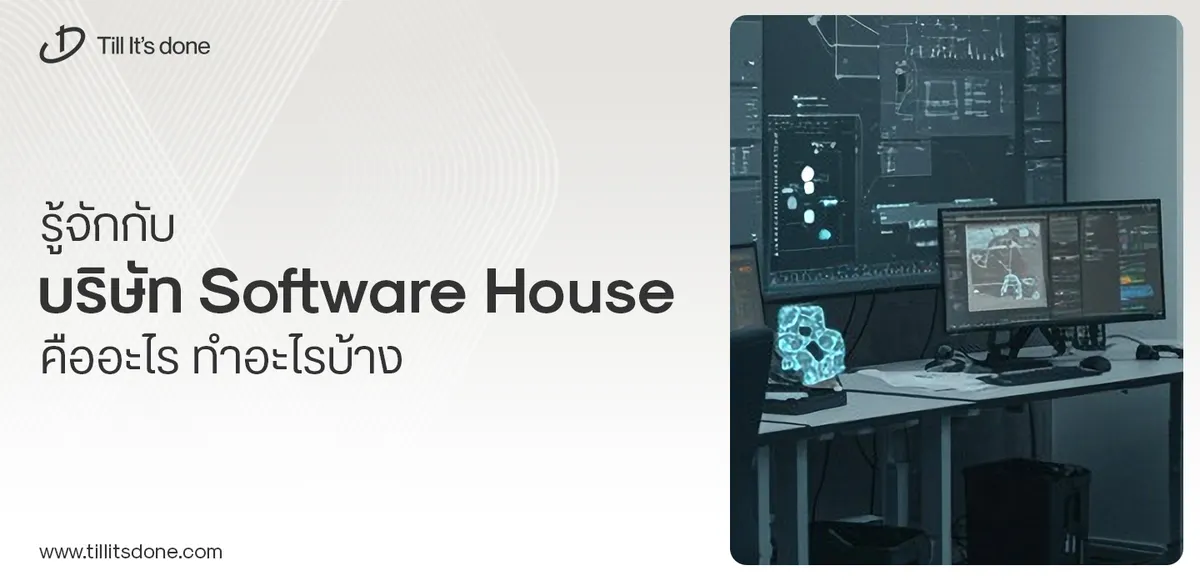
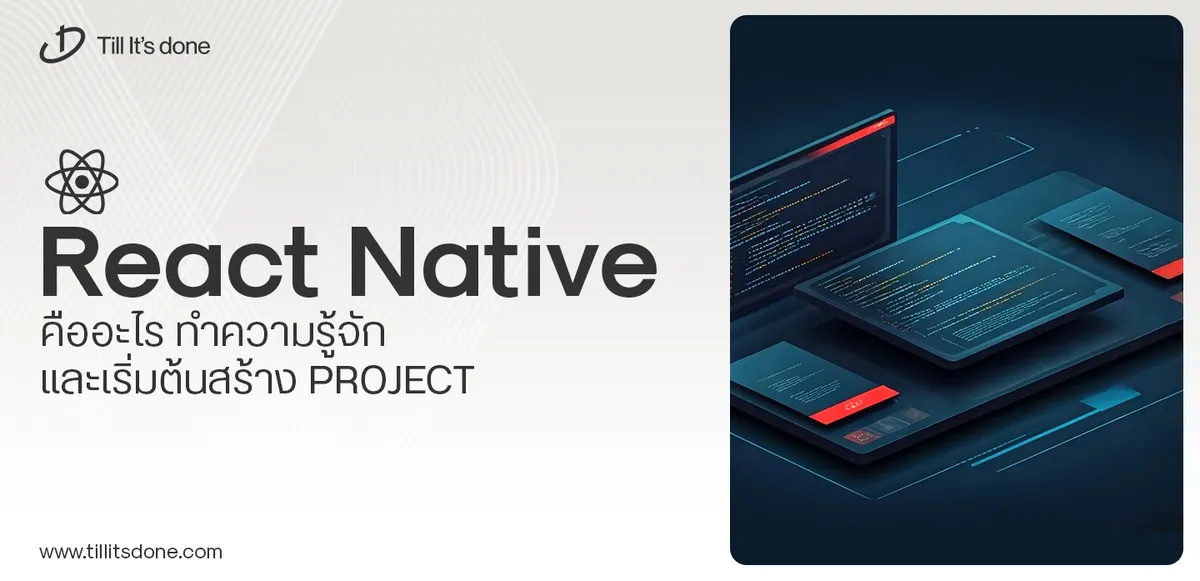
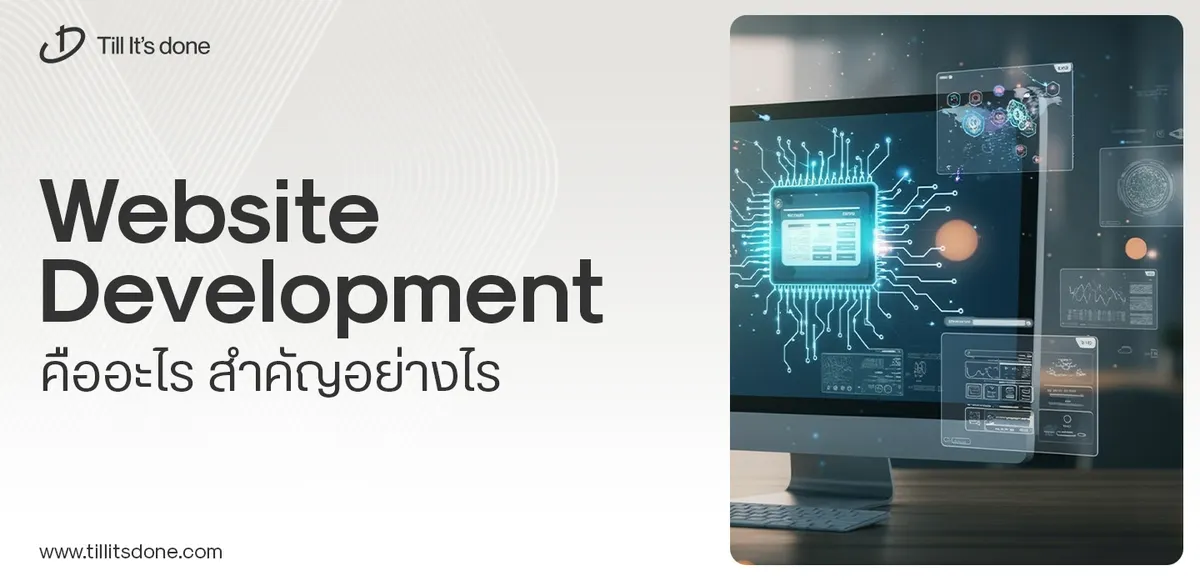
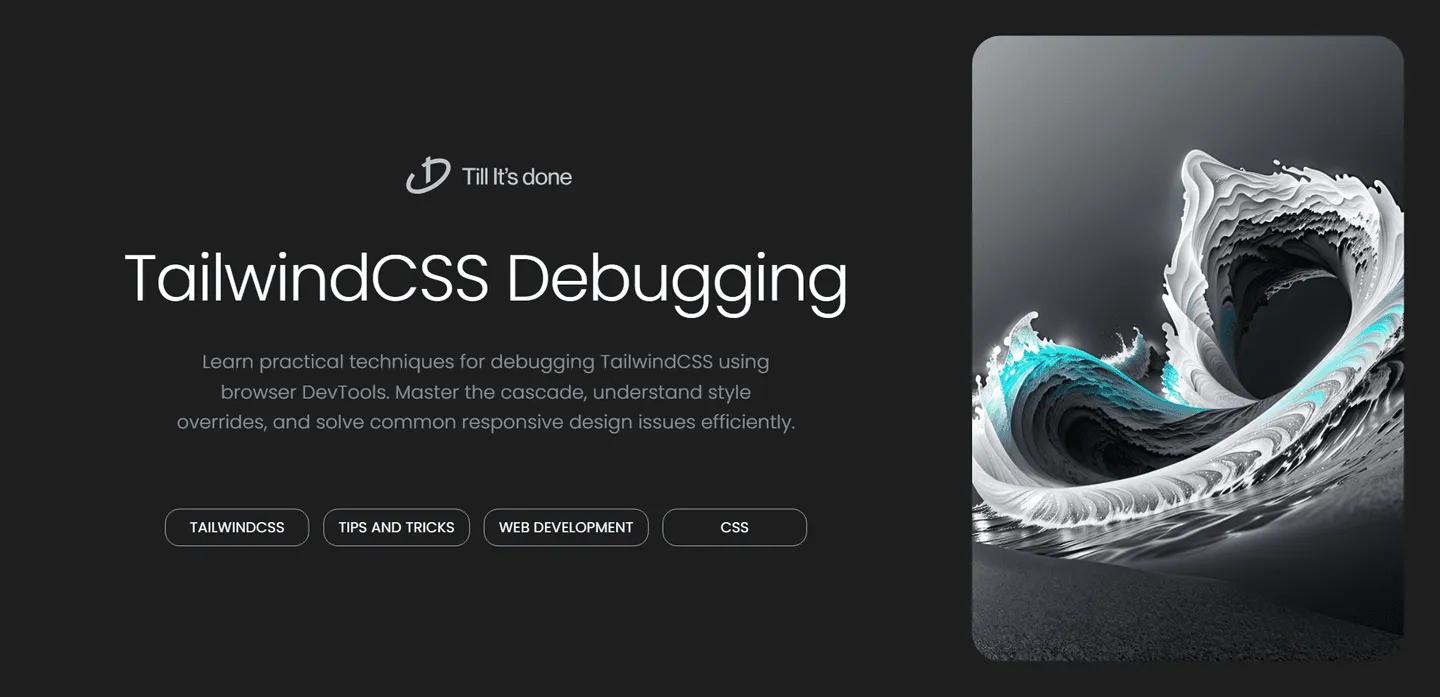
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.