- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Optimize Axios Performance in React Apps
Discover caching strategies, request cancellation, and performance monitoring to build faster, more efficient web apps.
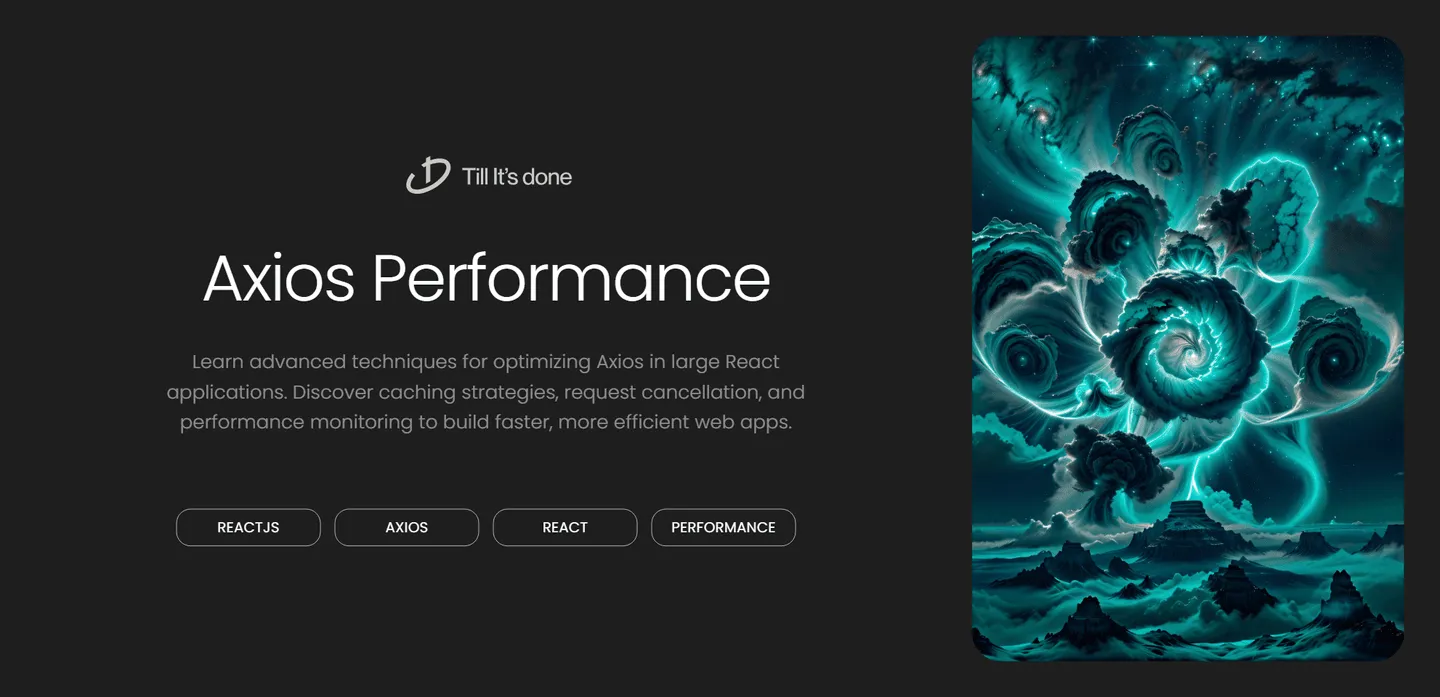
Optimizing Performance with Axios in Large React Applications
In today’s world of complex web applications, efficient data handling can make or break your user experience. As React applications grow larger, managing API calls becomes increasingly crucial. Let’s dive into some advanced techniques for optimizing Axios in your React applications that go beyond the basics.
Understanding the Performance Bottlenecks
When building large-scale React applications, we often encounter scenarios where multiple components require the same data, leading to redundant API calls. This not only impacts performance but also increases server load and potentially costs.
Implementation Strategies
1. Request Interceptors and Caching
One of the most effective ways to optimize Axios performance is implementing a robust caching system. Here’s an advanced approach that significantly reduces unnecessary network requests:
const cache = new Map();
axios.interceptors.request.use(config => { const cachedResponse = cache.get(config.url); if (cachedResponse && Date.now() - cachedResponse.timestamp < 60000) { return Promise.reject({ useCache: true, data: cachedResponse.data }); } return config;});
axios.interceptors.response.use(response => { cache.set(response.config.url, { data: response.data, timestamp: Date.now() }); return response;});
2. Request Cancellation and Rate Limiting
In single-page applications, managing concurrent requests and preventing race conditions is crucial:
function useAPIWithCancel() { const cancelTokenRef = useRef();
const makeRequest = async (url) => { if (cancelTokenRef.current) { cancelTokenRef.current.cancel('Operation cancelled due to new request'); }
cancelTokenRef.current = axios.CancelToken.source();
try { const response = await axios.get(url, { cancelToken: cancelTokenRef.current.token }); return response.data; } catch (error) { if (!axios.isCancel(error)) { throw error; } } };
return makeRequest;}
3. Response Transformation and Error Handling
Implementing a standardized response transformation layer can significantly improve code maintainability and performance:
const axiosInstance = axios.create({ baseURL: process.env.REACT_APP_API_URL, transformResponse: [...axios.defaults.transformResponse, data => { // Custom data transformation const transformed = someTransformationLogic(data); return transformed; }], transformError: (error) => { // Centralized error handling if (error.response?.status === 429) { return retryRequest(error.config); } throw error; }});
Advanced Optimization Techniques
-
Request Deduplication: Implement a request queue system to prevent duplicate API calls for the same data within a specified time window.
-
Prefetching Strategy: Utilize route-based prefetching to load data before users navigate to specific sections of your application.
-
Selective Polling: Implement intelligent polling that adjusts its frequency based on user activity and data staleness.
Monitoring and Performance Metrics
To ensure your optimizations are effective, implement performance monitoring:
axios.interceptors.request.use(config => { config.metadata = { startTime: new Date() }; return config;}, error => { return Promise.reject(error);});
axios.interceptors.response.use(response => { const duration = new Date() - response.config.metadata.startTime; // Log or track the duration return response;}, error => { return Promise.reject(error);});
Conclusion
Optimizing Axios in large React applications requires a multi-faceted approach. By implementing these strategies, you can significantly improve your application’s performance, reduce server load, and enhance the user experience.
Remember that optimization is an iterative process. Regularly monitor your application’s performance metrics and adjust your strategies based on real-world usage patterns.
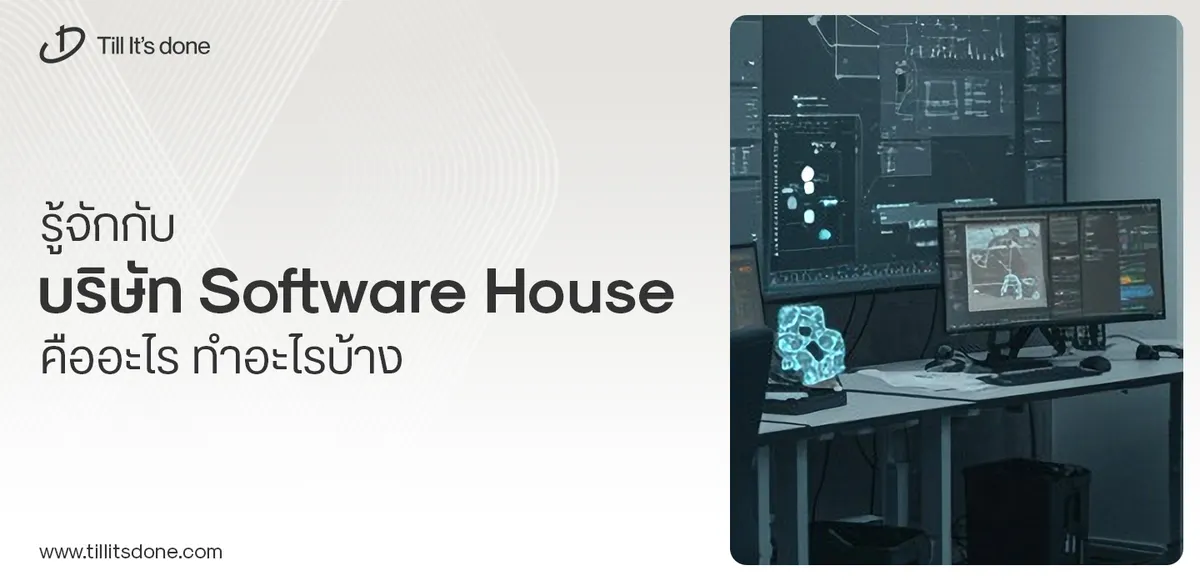
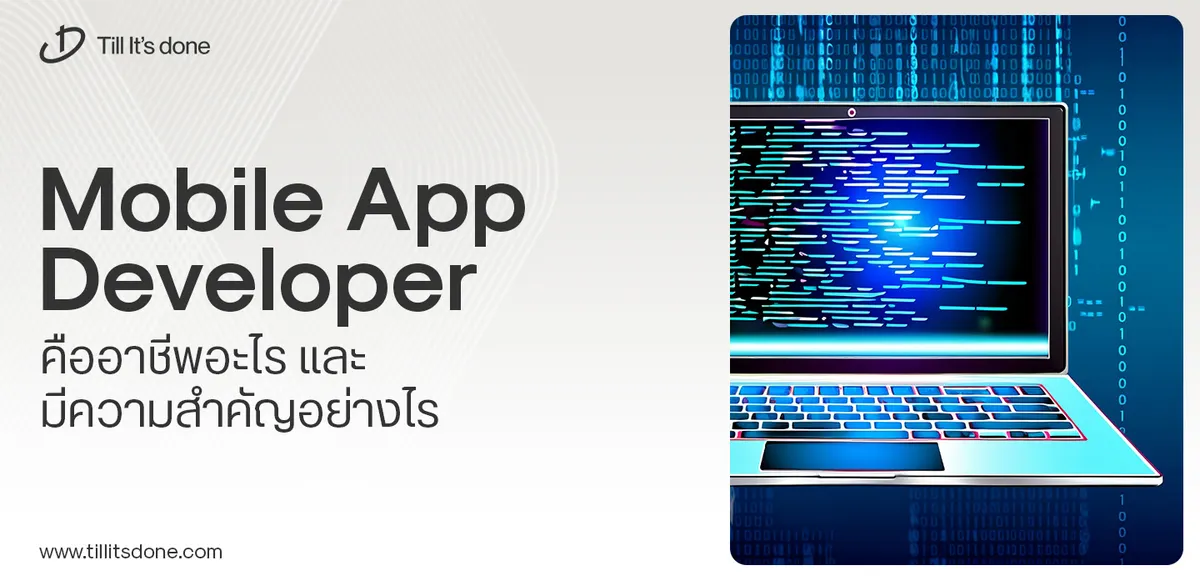
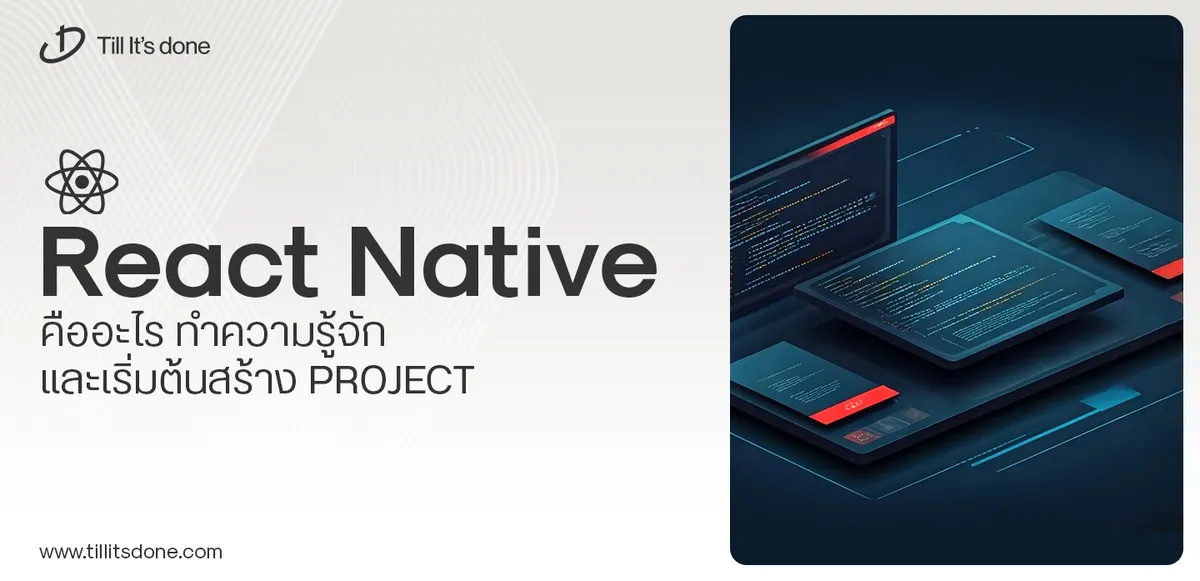
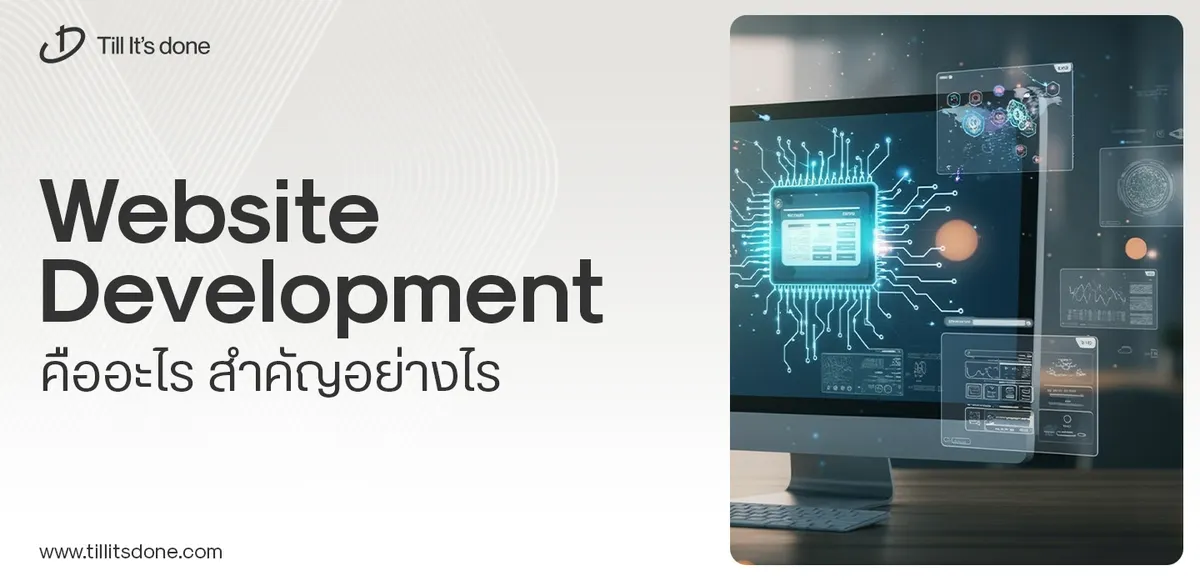
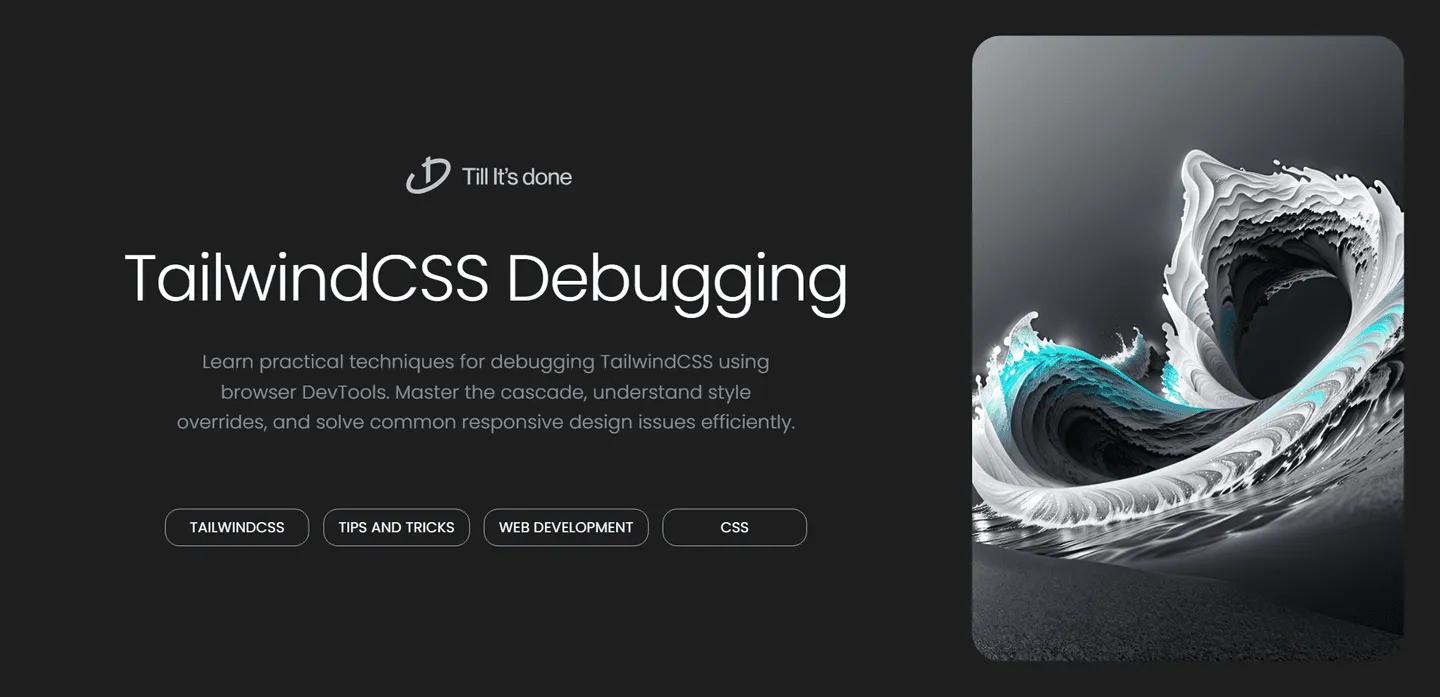
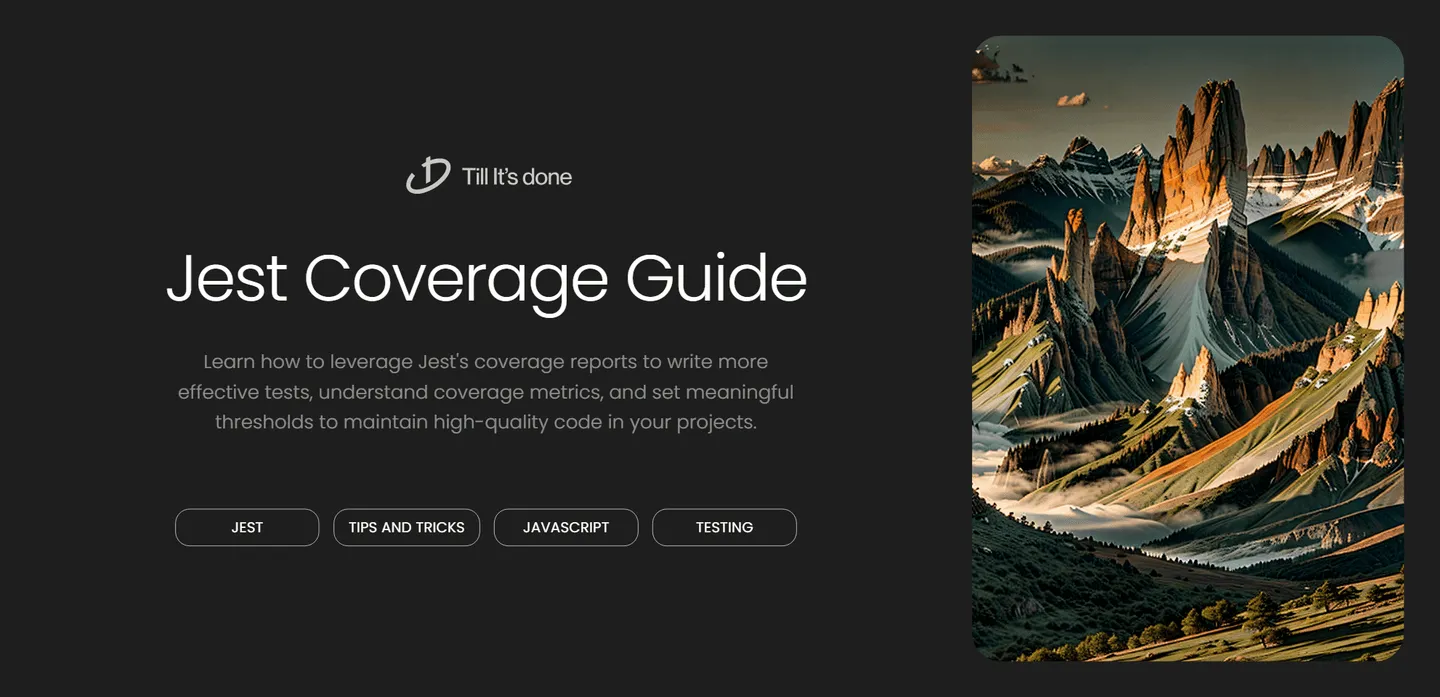
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.