- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Use Axios for CRUD in React Apps
Discover best practices for API integration, error handling, and state management with practical examples.
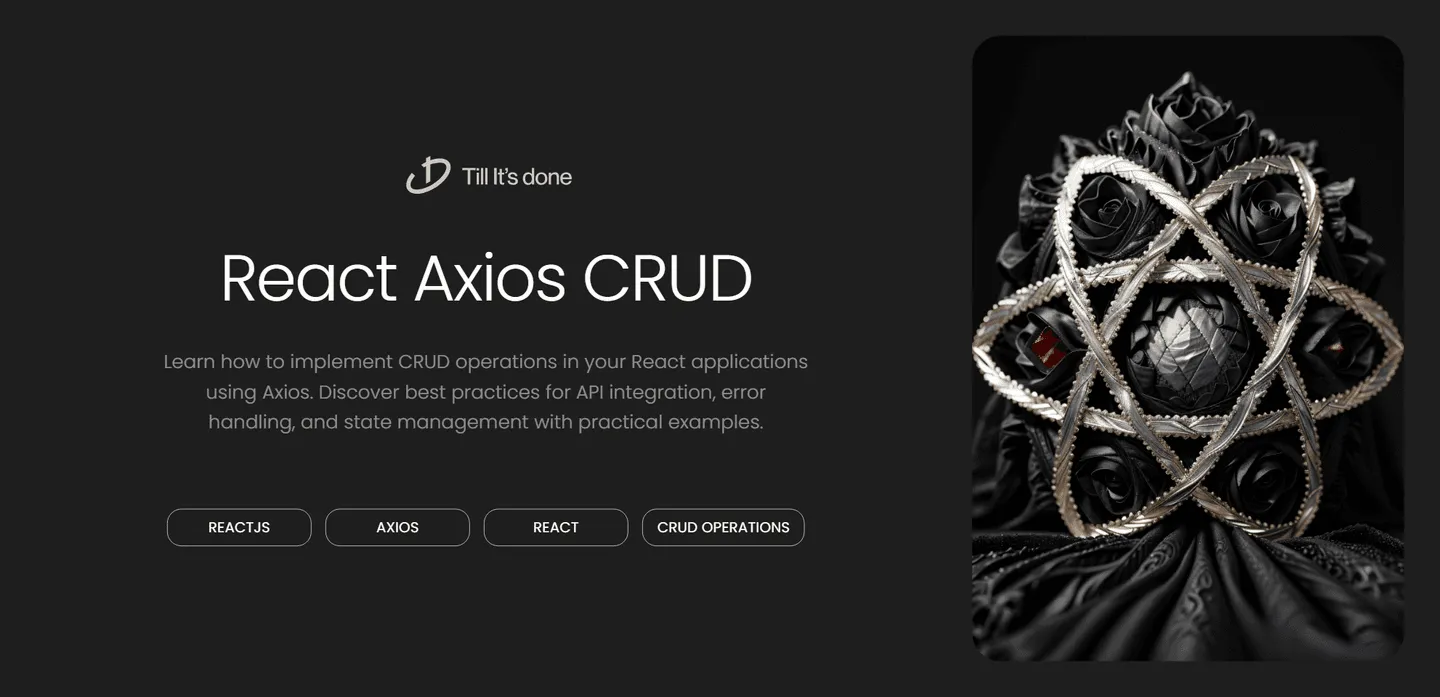
How to Use Axios for CRUD Operations in a React Application
Have you ever wondered how to efficiently handle API calls in your React applications? Let me walk you through implementing CRUD operations using Axios, one of the most popular HTTP clients for JavaScript. As a developer who’s worked extensively with these technologies, I’ll share some practical insights and best practices.
Setting Up Your Project
First things first, let’s get our project ready. We’ll need to install the necessary dependencies. In your React project directory, run:
npm install axios
Once installed, let’s create a simple configuration file to set up our Axios instance. This helps us maintain consistent API calls throughout our application:
import axios from 'axios';
const axiosInstance = axios.create({ baseURL: 'https://api.example.com', timeout: 5000, headers: { 'Content-Type': 'application/json' }});
export default axiosInstance;
Implementing CRUD Operations
Let’s create a custom hook to handle our CRUD operations. This approach keeps our code organized and reusable:
import { useState } from 'react';import axiosInstance from '../api/axios.config';
export const useApi = () => { const [loading, setLoading] = useState(false); const [error, setError] = useState(null);
// CREATE const createItem = async (endpoint, data) => { setLoading(true); try { const response = await axiosInstance.post(endpoint, data); setLoading(false); return response.data; } catch (err) { setError(err.message); setLoading(false); throw err; } };
// READ const getItems = async (endpoint) => { setLoading(true); try { const response = await axiosInstance.get(endpoint); setLoading(false); return response.data; } catch (err) { setError(err.message); setLoading(false); throw err; } };
// UPDATE const updateItem = async (endpoint, data) => { setLoading(true); try { const response = await axiosInstance.put(endpoint, data); setLoading(false); return response.data; } catch (err) { setError(err.message); setLoading(false); throw err; } };
// DELETE const deleteItem = async (endpoint) => { setLoading(true); try { const response = await axiosInstance.delete(endpoint); setLoading(false); return response.data; } catch (err) { setError(err.message); setLoading(false); throw err; } };
return { loading, error, createItem, getItems, updateItem, deleteItem };};
Using the Hook in Components
Now, let’s see how we can use this hook in a real component:
import React, { useEffect, useState } from 'react';import { useApi } from '../hooks/useApi';
const ItemList = () => { const [items, setItems] = useState([]); const { loading, error, getItems, createItem, updateItem, deleteItem } = useApi();
useEffect(() => { fetchItems(); }, []);
const fetchItems = async () => { try { const data = await getItems('/items'); setItems(data); } catch (error) { console.error('Failed to fetch items:', error); } };
const handleCreate = async (newItem) => { try { await createItem('/items', newItem); fetchItems(); // Refresh the list } catch (error) { console.error('Failed to create item:', error); } };
const handleUpdate = async (id, updatedItem) => { try { await updateItem(`/items/${id}`, updatedItem); fetchItems(); } catch (error) { console.error('Failed to update item:', error); } };
const handleDelete = async (id) => { try { await deleteItem(`/items/${id}`); fetchItems(); } catch (error) { console.error('Failed to delete item:', error); } };
if (loading) return <div>Loading...</div>; if (error) return <div>Error: {error}</div>;
return ( // Your JSX for rendering items and handling CRUD operations );};
export default ItemList;
Best Practices and Tips
- Always handle loading states and errors appropriately
- Use try-catch blocks to manage exceptions
- Implement proper error feedback for users
- Consider implementing request cancellation for pending requests
- Use environment variables for API endpoints
- Add request/response interceptors for global error handling and authentication
Remember to handle your API responses appropriately and provide proper feedback to your users. This makes your application more user-friendly and easier to debug.
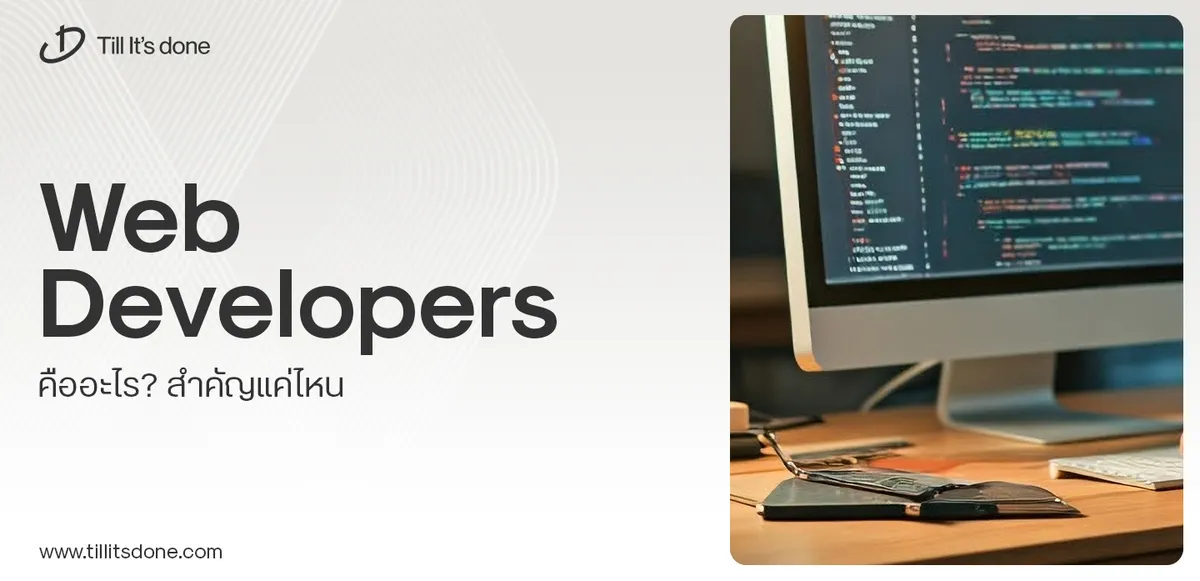
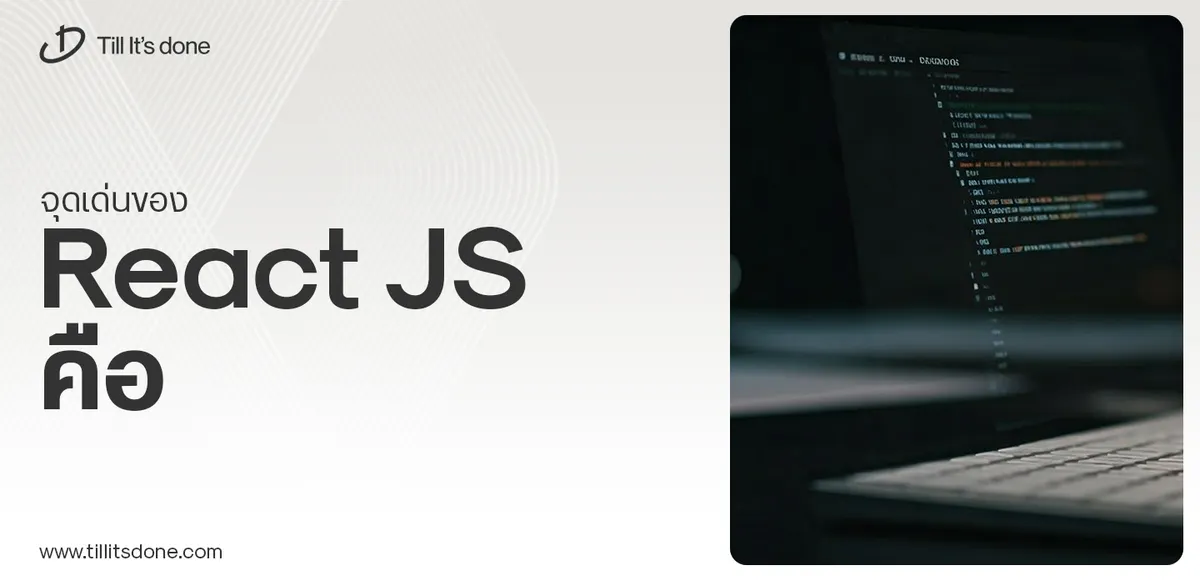
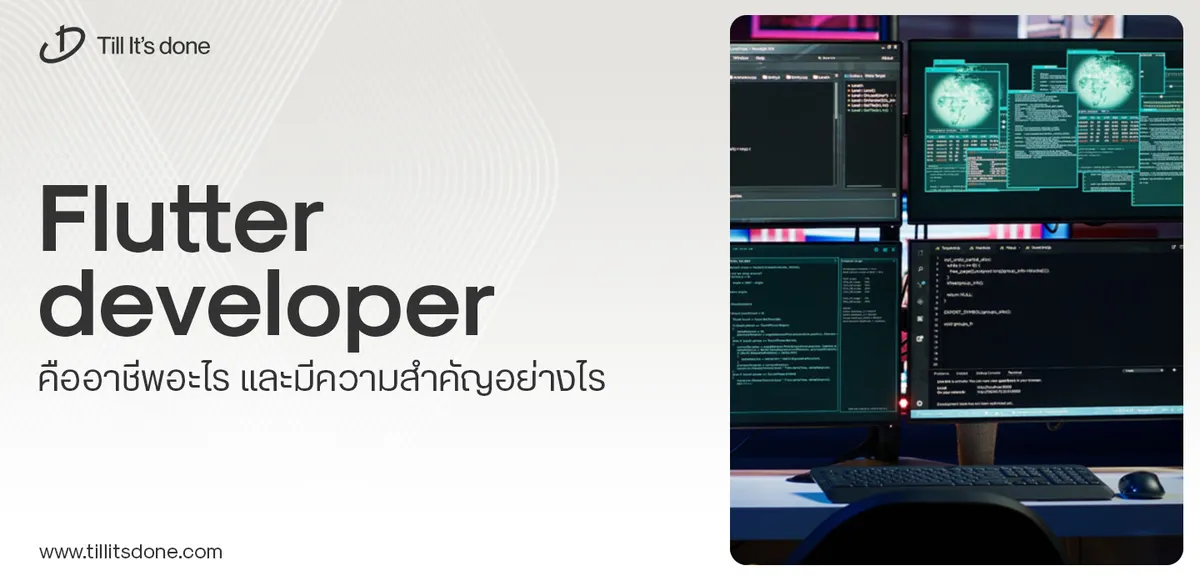
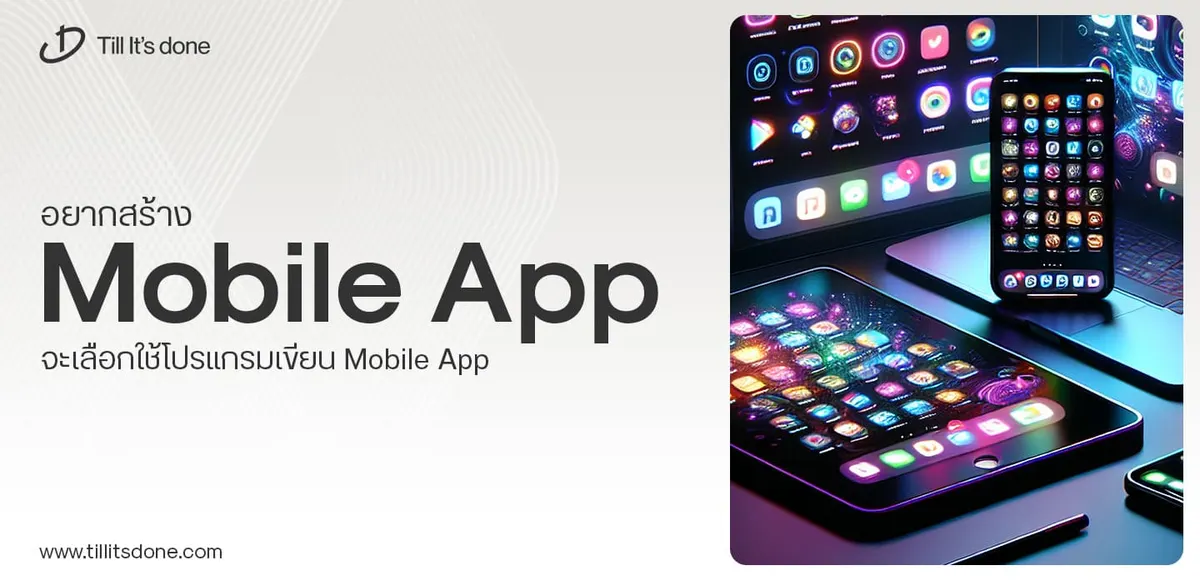
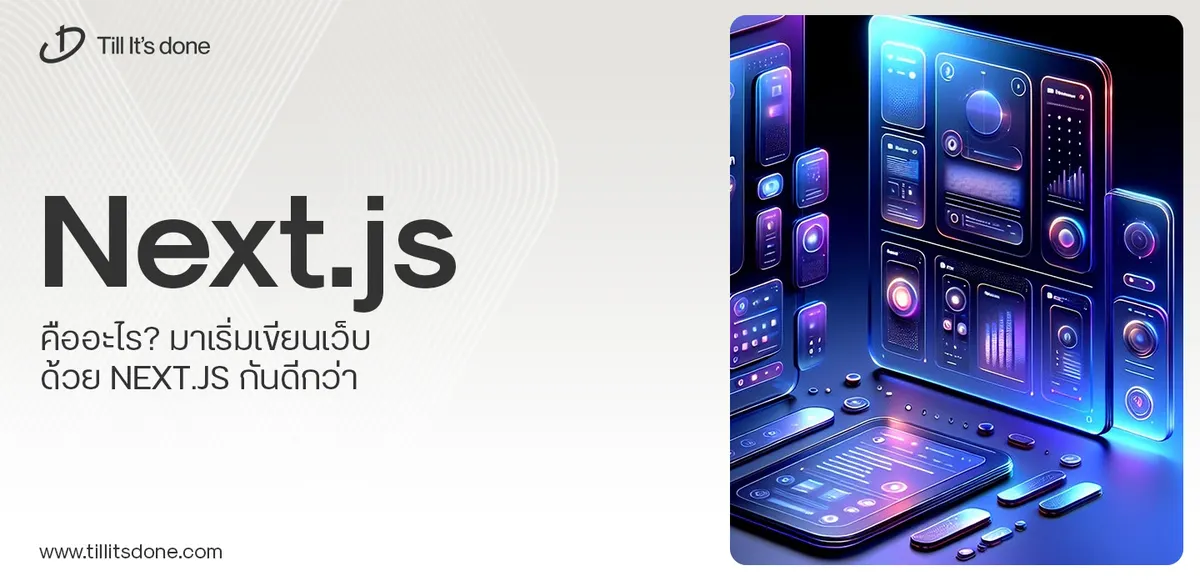
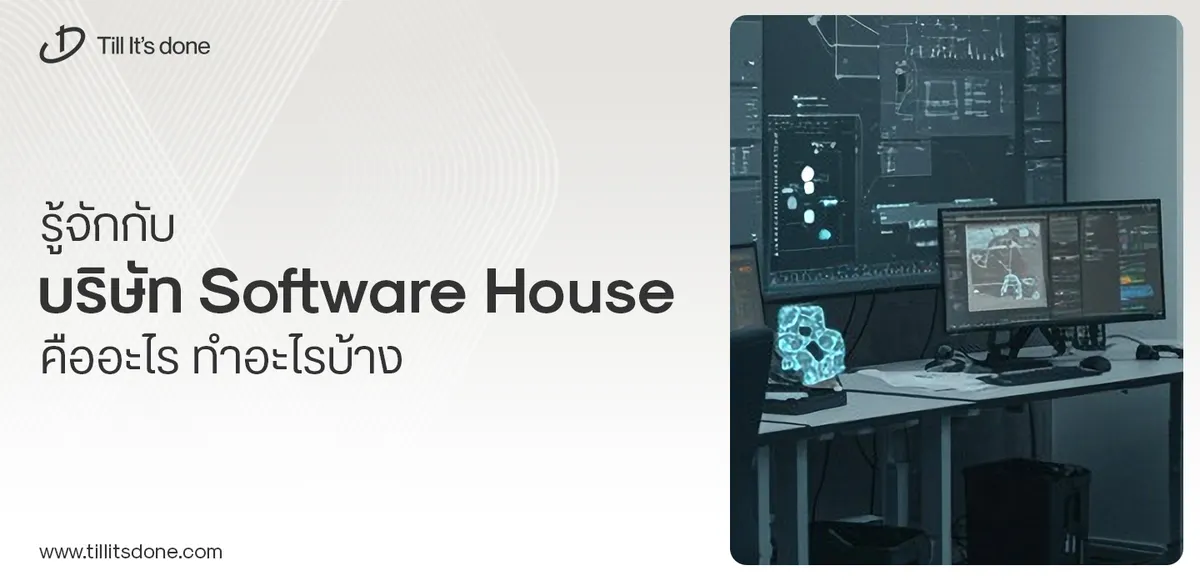
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.