- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Axios Interceptors for Error Handling
Discover best practices for managing API requests, responses, and maintaining clean error management.
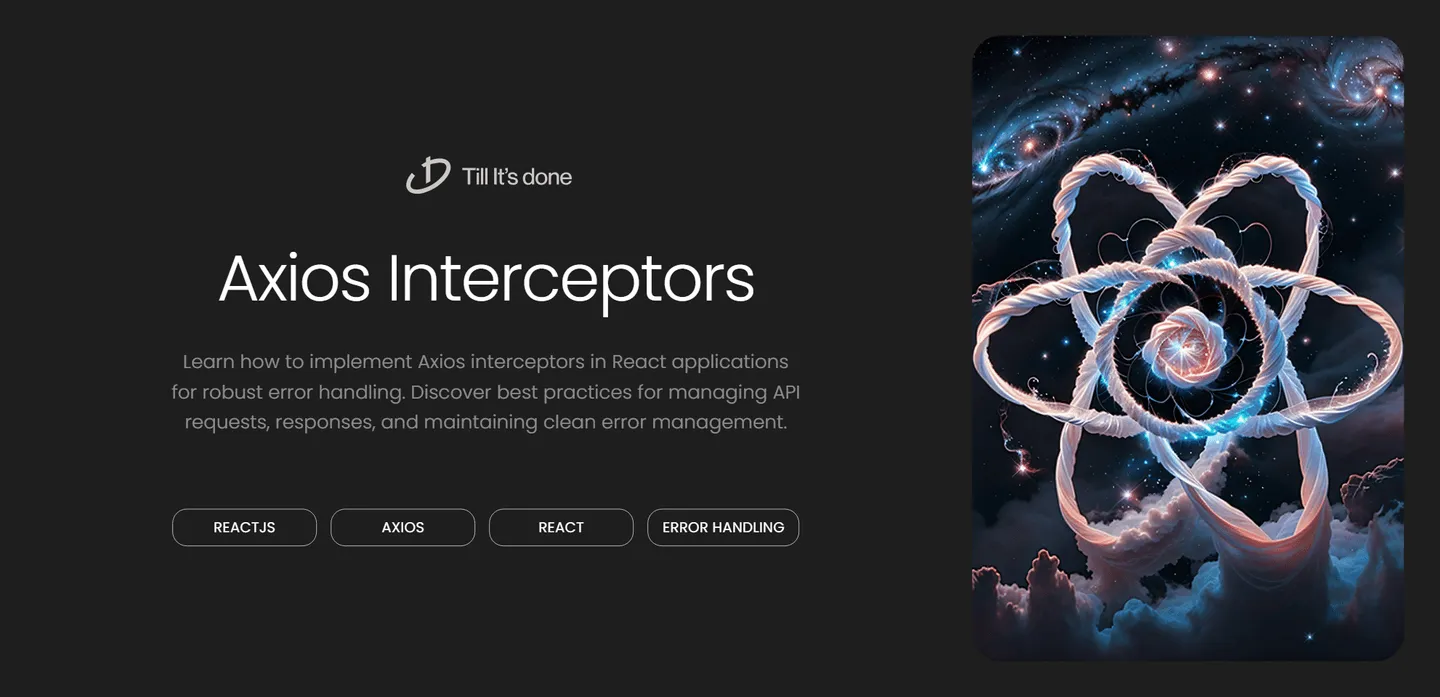
Using Axios Interceptors in React for Error Handling
In the world of modern web development, handling API requests effectively is crucial for building robust React applications. Axios interceptors are powerful tools that can help you manage HTTP requests and responses in a clean, centralized way. Let’s dive into how we can leverage them for better error handling in React applications.
What are Axios Interceptors?
Think of interceptors as security checkpoints at an airport. Before a request takes off (is sent to the server) or after a response lands (comes back from the server), interceptors can inspect, modify, or even cancel these operations. This makes them perfect for implementing consistent error handling across your entire application.
Setting Up Request Interceptors
Request interceptors are particularly useful for adding authentication tokens, setting common headers, or validating data before it reaches your server. Here’s how you can implement them:
// Create an Axios instanceconst api = axios.create({ baseURL: 'https://api.example.com'});
// Add a request interceptorapi.interceptors.request.use( config => { // Add authentication token const token = localStorage.getItem('token'); if (token) { config.headers.Authorization = `Bearer ${token}`; } return config; }, error => { return Promise.reject(error); });
Response Interceptors for Error Handling
Response interceptors are where the magic happens for error handling. They can catch and process errors consistently across your entire application:
api.interceptors.response.use( response => response, error => { if (error.response) { switch (error.response.status) { case 401: // Handle unauthorized access store.dispatch(logout()); break; case 404: // Handle not found notify('Resource not found'); break; case 500: // Handle server errors notify('Something went wrong. Please try again later'); break; default: notify('An error occurred'); } } else if (error.request) { // Handle network errors notify('Network error. Please check your connection'); } return Promise.reject(error); });
Best Practices and Tips
- Create a separate file for interceptor configuration to keep your code organized
- Use custom error messages that are user-friendly
- Implement retry logic for failed requests
- Add logging for debugging purposes
- Handle offline scenarios gracefully
Remember to clean up interceptors when they’re no longer needed, especially in components that create custom instances:
const interceptorId = api.interceptors.request.use(/*...*/);// When doneapi.interceptors.request.eject(interceptorId);
By implementing Axios interceptors effectively, you can create a more robust error handling system that provides better user experience and easier debugging. Remember that good error handling isn’t just about catching errors – it’s about managing them in a way that maintains the stability and reliability of your application.
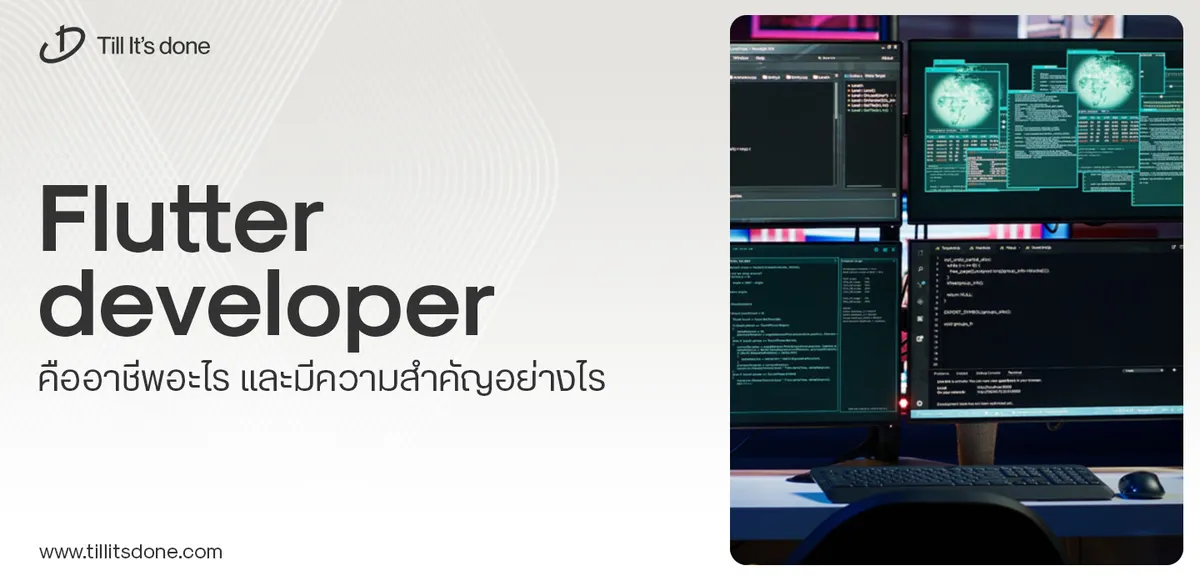
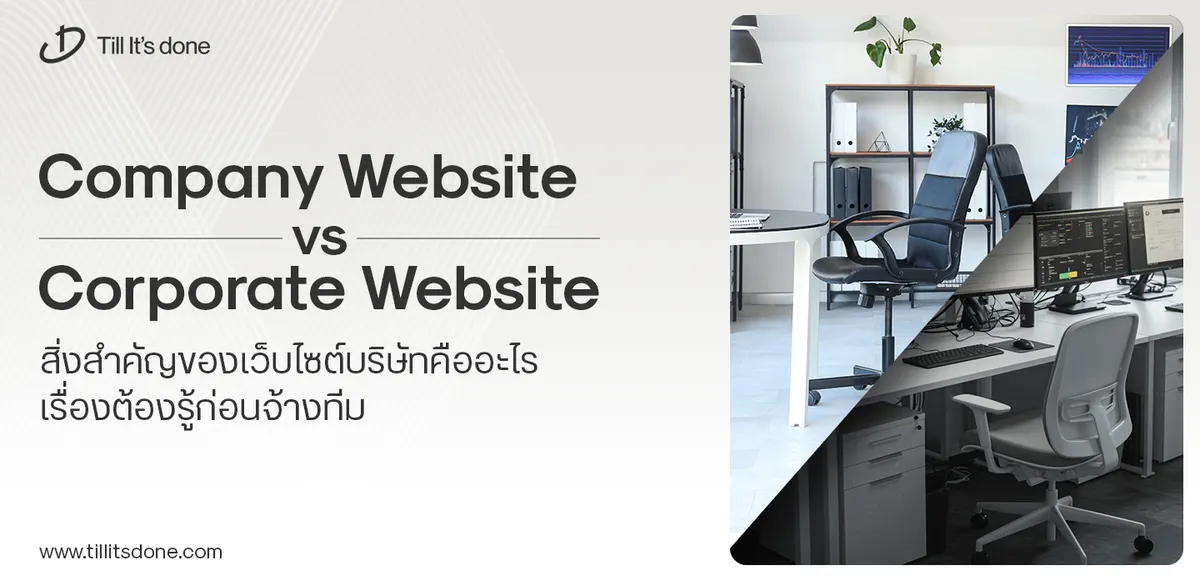
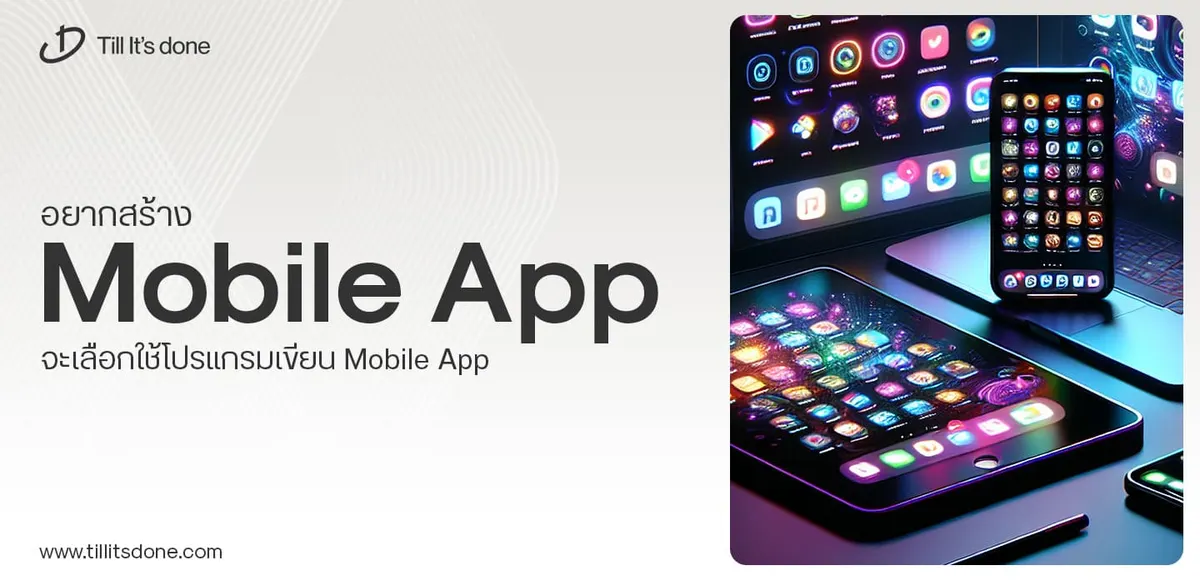
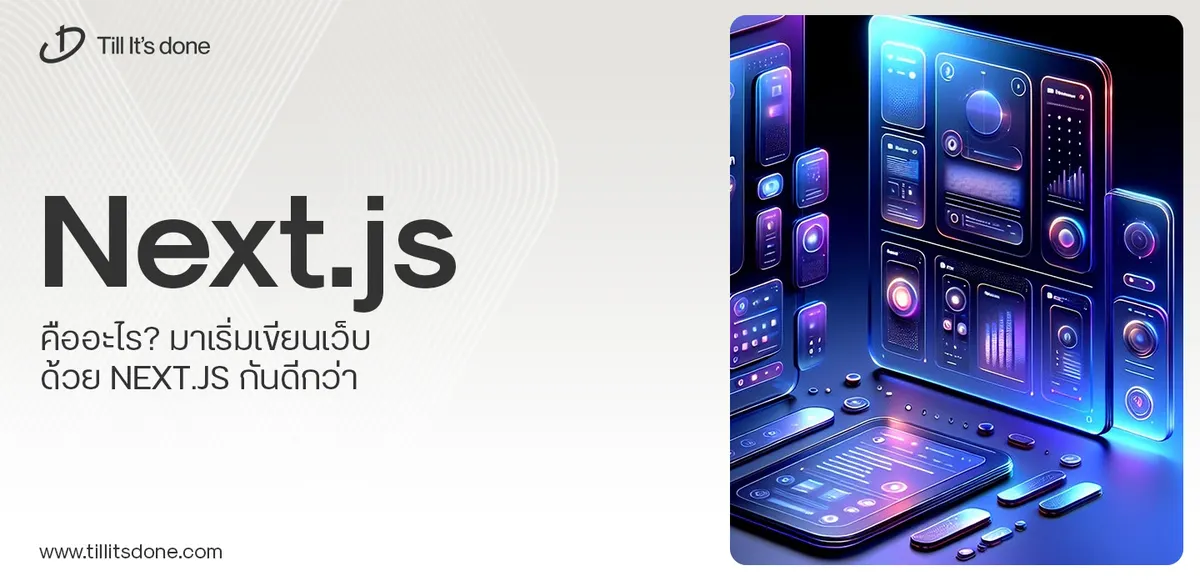
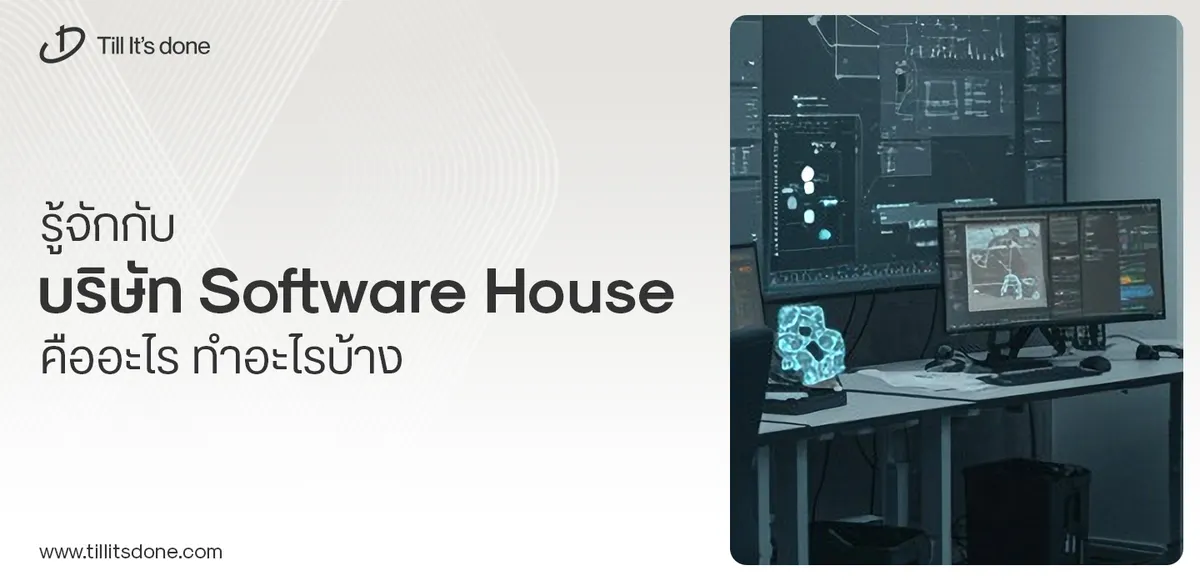
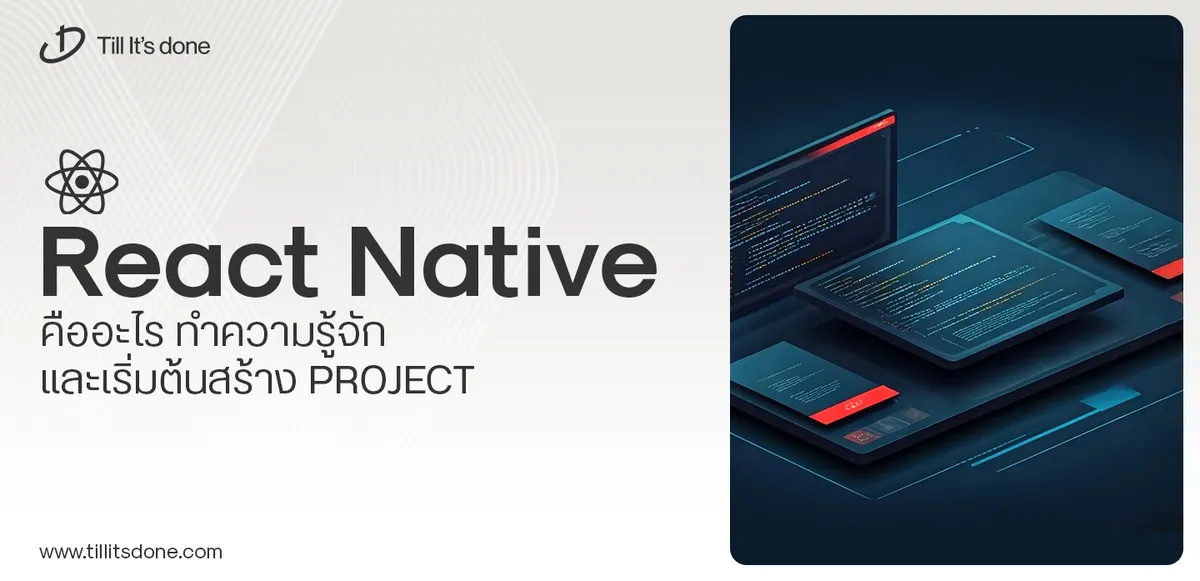
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.