- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices for Axios HTTP Calls in React
Discover how to handle errors, implement interceptors, organize API calls, and improve performance.

Best Practices for Making HTTP Calls with Axios in React
Making HTTP calls is a fundamental part of modern web applications, and when it comes to React development, Axios stands out as one of the most popular choices for handling these requests. Let’s dive into some best practices that will help you write cleaner, more maintainable, and more efficient code when working with Axios in your React applications.
Setting Up Axios Instance
One of the most crucial best practices is creating a centralized Axios instance. This approach helps maintain consistency across your application and makes it easier to manage common configurations.
Instead of importing Axios directly in each component, create a separate configuration file:
import axios from 'axios';
const axiosInstance = axios.create({ baseURL: process.env.REACT_APP_API_URL, timeout: 5000, headers: { 'Content-Type': 'application/json' }});
export default axiosInstance;
Error Handling and Interceptors
Implementing proper error handling is essential for a robust application. Axios interceptors provide a clean way to handle errors globally:
axiosInstance.interceptors.response.use( response => response, error => { if (error.response.status === 401) { // Handle unauthorized access localStorage.removeItem('token'); window.location = '/login'; } return Promise.reject(error); });
Organizing API Calls
Create dedicated service files for different features or entities in your application. This separation of concerns makes your code more maintainable and reusable:
import api from '../api/axios.config';
export const userService = { getUser: (id) => api.get(`/users/${id}`), updateUser: (id, data) => api.put(`/users/${id}`, data), deleteUser: (id) => api.delete(`/users/${id}`)};
Using Async/Await with Hooks
When making API calls in React components, combine Axios with React hooks for clean and efficient data fetching:
const UserProfile = ({ userId }) => { const [user, setUser] = useState(null); const [loading, setLoading] = useState(false); const [error, setError] = useState(null);
useEffect(() => { const fetchUser = async () => { try { setLoading(true); const { data } = await userService.getUser(userId); setUser(data); } catch (err) { setError(err.message); } finally { setLoading(false); } };
fetchUser(); }, [userId]);
// Component render logic};
Request Cancellation
Remember to cancel ongoing requests when a component unmounts to prevent memory leaks and unwanted state updates:
useEffect(() => { const abortController = new AbortController();
const fetchData = async () => { try { const { data } = await axiosInstance.get('/endpoint', { signal: abortController.signal }); // Handle data } catch (error) { if (error.name === 'AbortError') { // Handle abort } } };
fetchData();
return () => abortController.abort();}, []);
Cache and Request Deduplication
Implement request caching and deduplication to improve performance and reduce unnecessary network calls:
const cache = new Map();
const fetchWithCache = async (url) => { if (cache.has(url)) { return cache.get(url); }
const response = await axiosInstance.get(url); cache.set(url, response.data); return response.data;};
Remember to clear the cache when needed and implement cache invalidation strategies based on your application’s requirements.

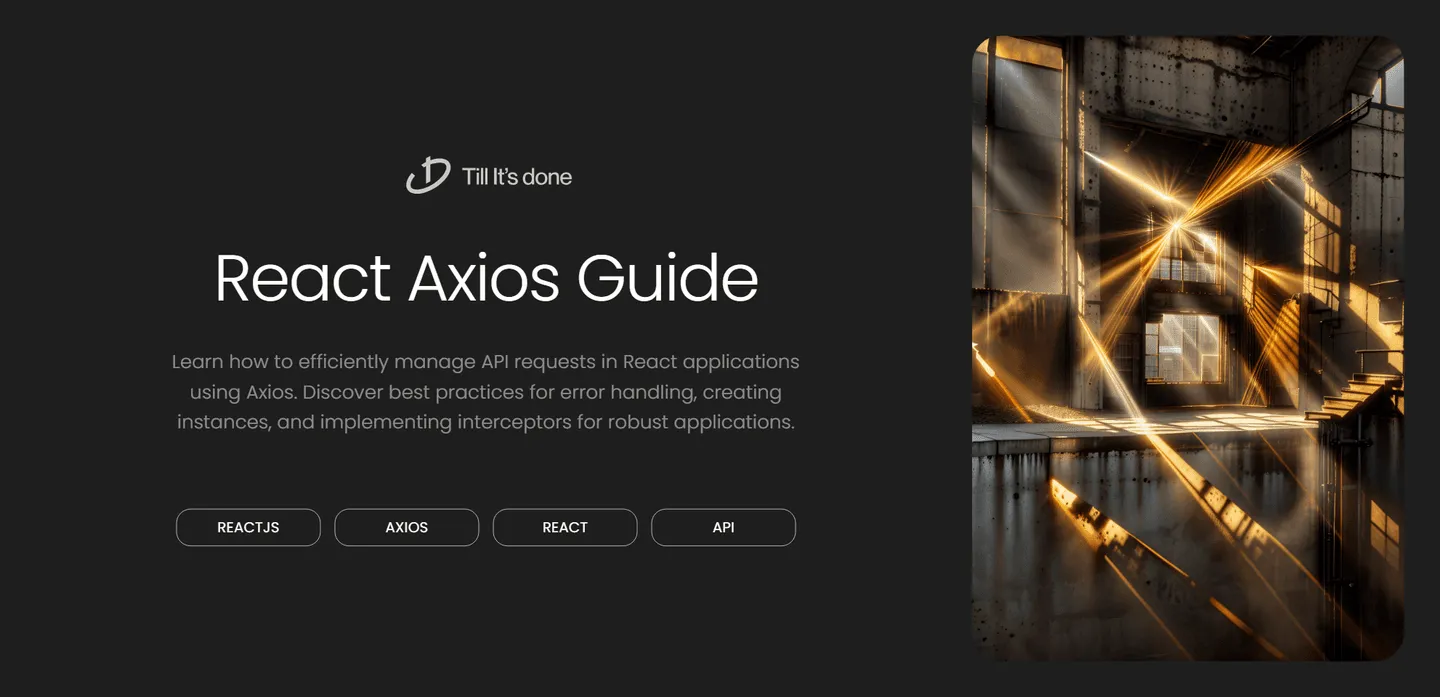

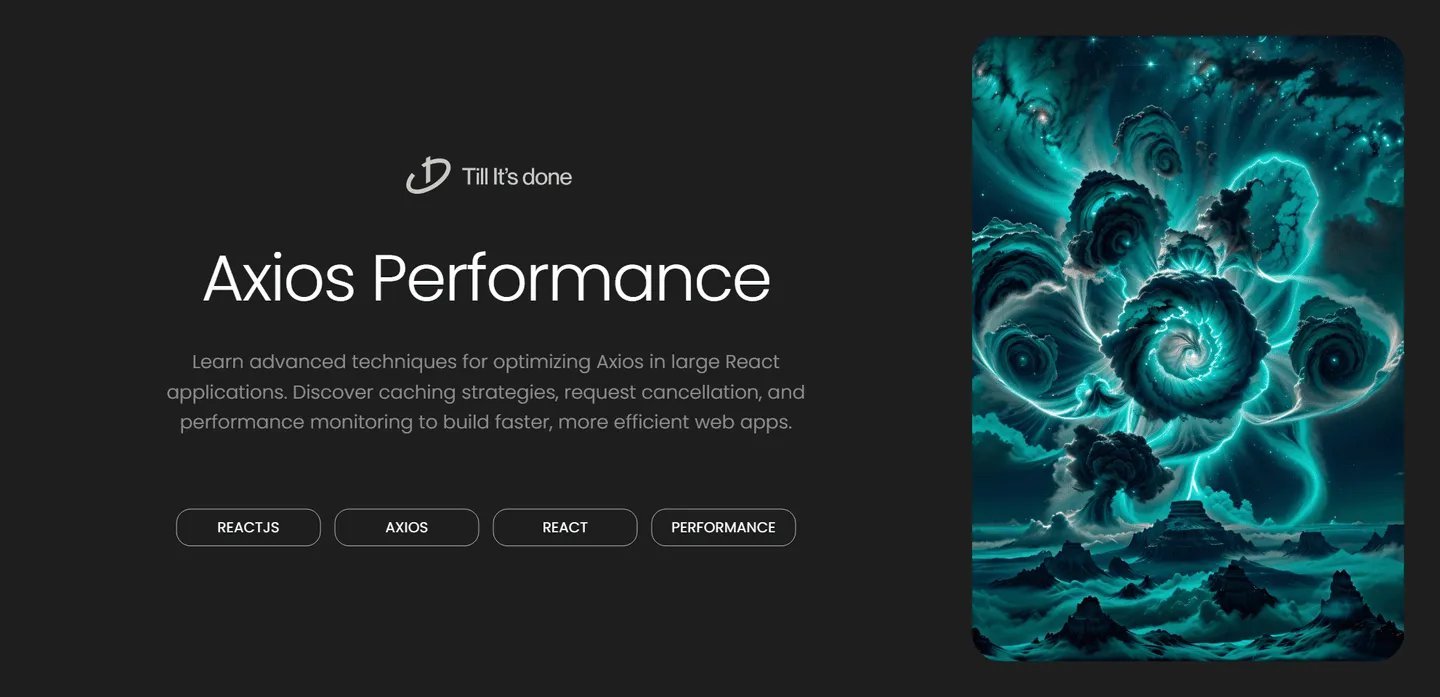


Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.