- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Error Boundaries & Axios: Handle API Failures
Discover best practices for managing API failures and creating better user experiences.

Error Boundaries and Axios: Managing API Failures in React
In the world of modern web development, handling API failures gracefully is crucial for creating robust React applications. Today, let’s dive into how we can leverage Error Boundaries alongside Axios to create a better error handling system that keeps our users informed and our application stable.
Understanding Error Boundaries
Error Boundaries were introduced in React 16 as a way to catch and handle JavaScript errors that occur during rendering. Think of them as a safety net that prevents your entire application from crashing when something goes wrong.
Let’s create a basic Error Boundary component:
class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; }
static getDerivedStateFromError(error) { return { hasError: true }; }
componentDidCatch(error, errorInfo) { // Log the error to your error tracking service console.error('Error caught by boundary:', error, errorInfo); }
render() { if (this.state.hasError) { return <h1>Something went wrong. Please try again later.</h1>; }
return this.props.children; }}
Integrating Axios with Error Boundaries
While Error Boundaries are great for handling rendering errors, they won’t catch errors in async operations by default. Here’s where we need to be clever with our Axios implementation:
const DataFetching = () => { const [data, setData] = useState(null); const [error, setError] = useState(null);
useEffect(() => { const fetchData = async () => { try { const response = await axios.get('https://api.example.com/data'); setData(response.data); } catch (error) { setError(error); // Throw error to be caught by Error Boundary throw new Error('API Request failed'); } };
fetchData(); }, []);
if (error) { throw error; }
return <div>{/* Render your data */}</div>;};
Best Practices for Error Handling
- Create Axios Instances: Use axios.create() to set up instances with predefined configurations and interceptors.
const api = axios.create({ baseURL: process.env.REACT_APP_API_URL, timeout: 5000,});
api.interceptors.response.use( response => response, error => { // Handle global errors here throw error; });
- Implement Retry Logic: Add retry mechanisms for transient failures.
const axiosRetry = require('axios-retry');
axiosRetry(api, { retries: 3, retryDelay: axiosRetry.exponentialDelay, retryCondition: (error) => { return axiosRetry.isNetworkOrIdempotentRequestError(error); }});
- Use Custom Error Components: Create informative error states for different scenarios.
const ErrorFallback = ({ error }) => { if (error.response?.status === 404) { return <div>The requested resource was not found.</div>; }
if (error.response?.status === 403) { return <div>You don't have permission to access this resource.</div>; }
return <div>An unexpected error occurred. Please try again later.</div>;};
By combining Error Boundaries with proper Axios error handling, we create a robust system that can gracefully handle API failures while maintaining a good user experience. Remember to always provide meaningful error messages and, when possible, recovery options for your users.
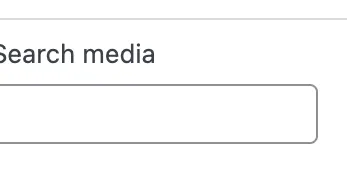





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.