- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
10 Performance Optimization Tips for Node.js Apps

10 Performance Optimization Tips for Node.js Applications
Performance optimization is crucial for building scalable Node.js applications. Here are ten battle-tested tips to supercharge your Node.js apps and deliver a blazing-fast experience to your users.
1. Implement Caching Strategies
Caching is your first line of defense against performance bottlenecks. Utilize memory caching solutions like Redis or Node-Cache to store frequently accessed data. This reduces database load and speeds up response times significantly.
const NodeCache = require('node-cache');const cache = new NodeCache({ stdTTL: 600 });
async function fetchUserData(userId) { const cachedData = cache.get(userId); if (cachedData) return cachedData;
const userData = await database.fetch(userId); cache.set(userId, userData); return userData;}
2. Use Compression Middleware
Enable compression for your HTTP responses to reduce payload size and improve loading times:
const compression = require('compression');app.use(compression());
3. Optimize Your Database Queries
Write efficient database queries and implement indexing strategies. Use database connection pooling to manage connections effectively:
const pool = mysql.createPool({ connectionLimit: 10, host: 'localhost', user: 'user', password: 'password', database: 'myapp'});
4. Leverage Cluster Module
Utilize all available CPU cores using the cluster module to handle more requests simultaneously:
const cluster = require('cluster');const numCPUs = require('os').cpus().length;
if (cluster.isMaster) { for (let i = 0; i < numCPUs; i++) { cluster.fork(); }} else { // Your server code}
5. Implement Proper Error Handling
Use try-catch blocks and Promise error handling to prevent memory leaks and application crashes:
process.on('uncaughtException', (error) => { console.error('Uncaught Exception:', error); // Implement proper error logging});
6. Use Asynchronous Operations Wisely
Leverage async/await and avoid blocking operations. Use Promise.all() for parallel operations:
async function fetchMultipleUsers(userIds) { const promises = userIds.map(id => fetchUser(id)); return Promise.all(promises);}
7. Implement Rate Limiting
Protect your application from abuse and maintain performance under heavy load:
const rateLimit = require('express-rate-limit');
app.use(rateLimit({ windowMs: 15 * 60 * 1000, max: 100}));
8. Optimize Static Assets
Use middleware like serve-static with proper cache headers and consider using a CDN for static content delivery.
9. Monitor Memory Usage
Implement memory monitoring and garbage collection optimization:
const used = process.memoryUsage();console.log(`Memory usage: ${Math.round(used.heapUsed / 1024 / 1024 * 100) / 100} MB`);
10. Use Production Mode
Always run your application in production mode to enable optimizations:
if (process.env.NODE_ENV !== 'production') { console.log('Remember to set NODE_ENV to production');}
Remember, performance optimization is an ongoing process. Regularly monitor your application’s performance metrics and adjust these optimizations based on your specific use case.
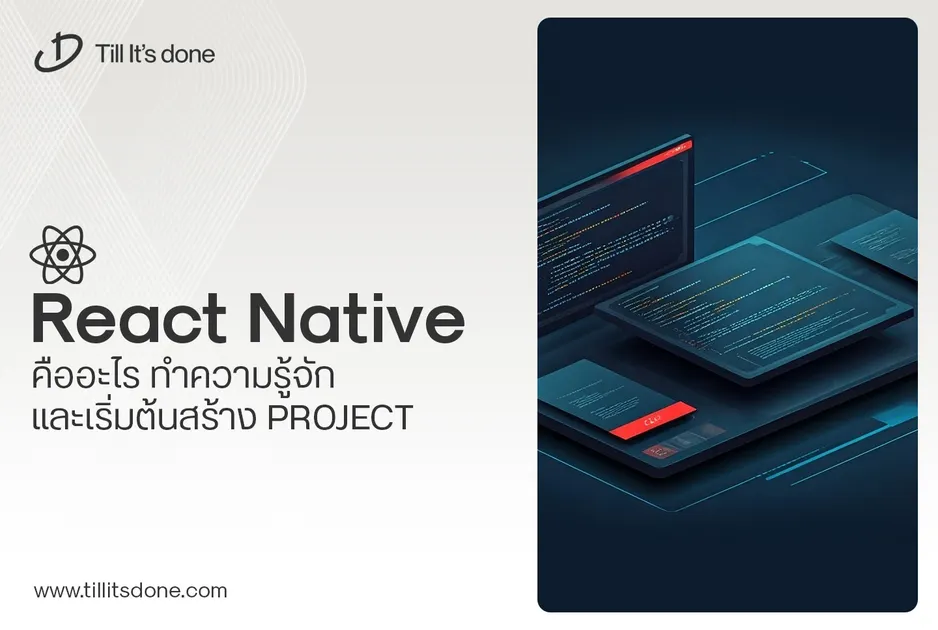





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.