- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Node.js App Structure Best Practices Guide
Discover modular architecture, environment configuration, error handling, and monitoring strategies for robust backends.
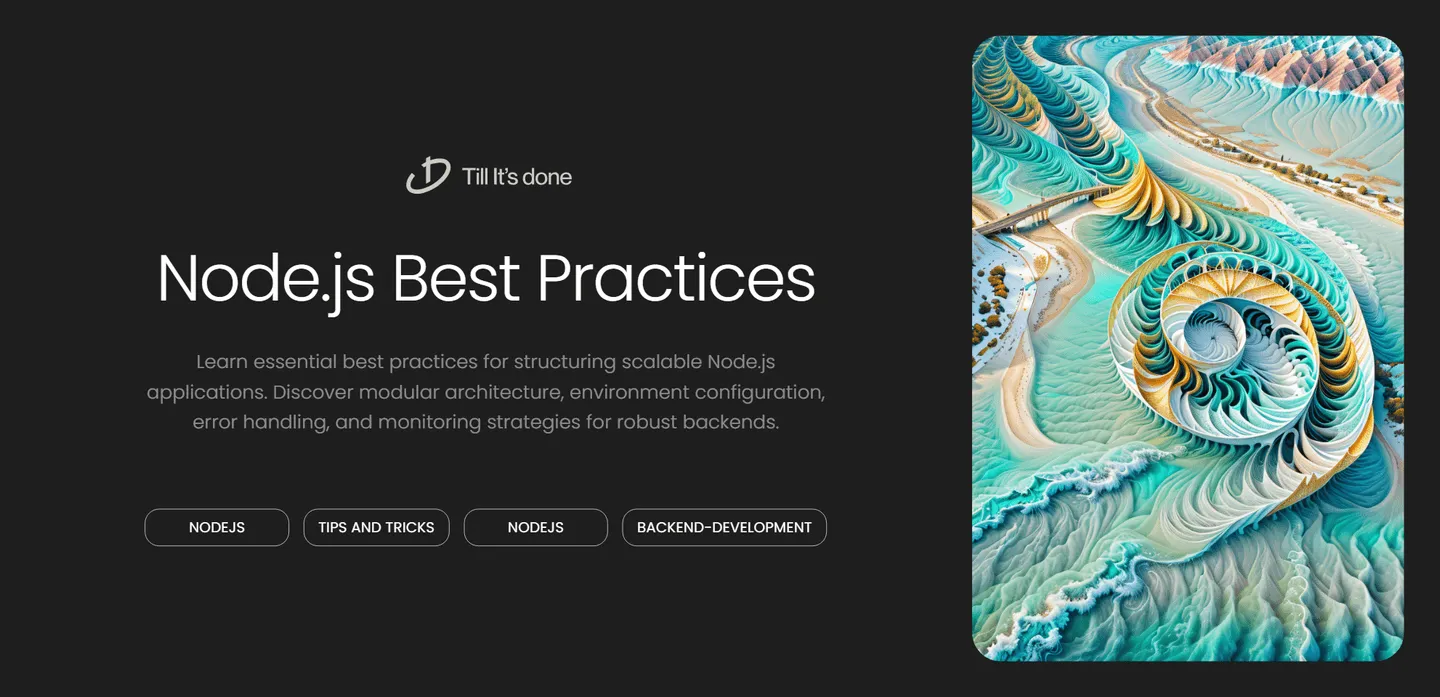
Best Practices for Structuring a Scalable Node.js Application
Building a scalable Node.js application isn’t just about writing code - it’s about creating a robust architecture that can grow with your needs. Let’s dive into some battle-tested practices that will help you build applications that can scale effortlessly.
Project Structure Matters
Think of your project structure as the foundation of a building. A solid foundation means easier maintenance and better scalability. Here’s a clean way to organize your Node.js application:
src/ ├── config/ # Configuration files ├── controllers/ # Request handlers ├── middlewares/ # Custom middleware ├── models/ # Data models ├── routes/ # Route definitions ├── services/ # Business logic ├── utils/ # Helper functions └── app.js # App entry point
Key Best Practices
1. Embrace Modular Architecture
Break your application into small, focused modules. Each module should have a single responsibility. This makes your code more maintainable and easier to test.
2. Implement Environment-Based Configuration
const config = { development: { port: 3000, database: 'mongodb://localhost/dev_db' }, production: { port: process.env.PORT, database: process.env.DATABASE_URL }};
module.exports = config[process.env.NODE_ENV || 'development'];
3. Use Dependency Injection
Instead of hard-coding dependencies, inject them. This makes your code more flexible and testable.
4. Implement Error Handling Middleware
Create a centralized error handling system to manage errors consistently across your application.
5. API Versioning
Version your APIs from day one. It’s easier to implement versioning early than to add it later:
router.get('/v1/users', userController.getUsers);
6. Database Operations
- Use connection pooling
- Implement database migrations
- Create database indexes strategically
- Use ORMs or query builders wisely
7. Caching Strategy
Implement caching at different levels:
- Application-level caching
- API-level caching
- Database query caching
8. Security Best Practices
- Use helmet.js for security headers
- Implement rate limiting
- Validate input data
- Use CORS properly
Monitoring and Logging
Set up comprehensive monitoring and logging from the start. Use tools like:
- Winston or Pino for logging
- PM2 for process management
- New Relic or Datadog for monitoring
Remember, scalability isn’t just about handling more users - it’s about maintaining performance and code quality as your application grows.
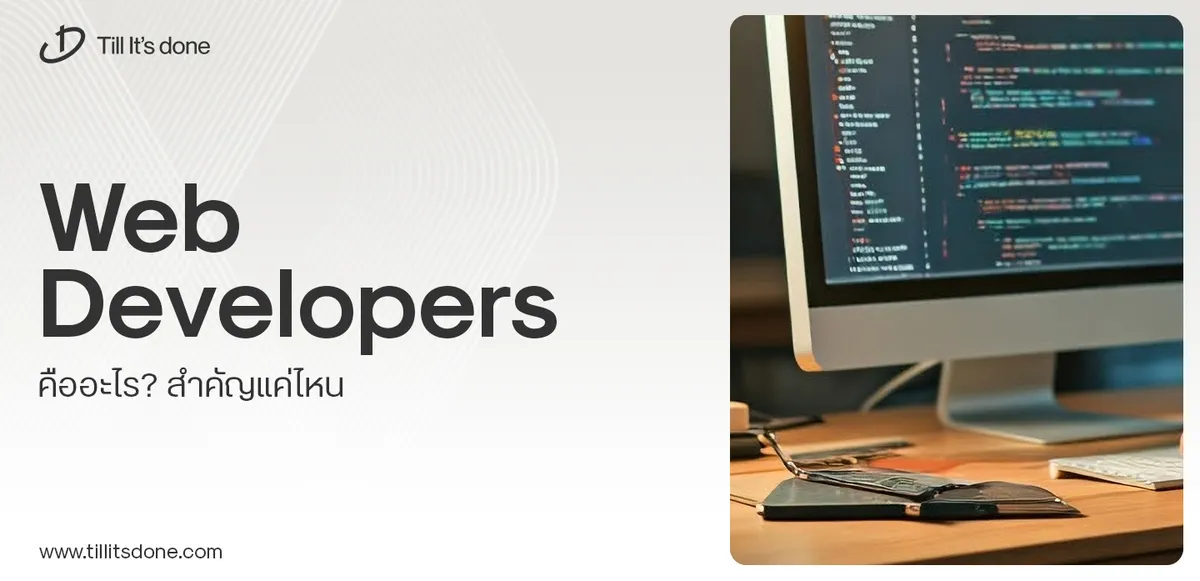
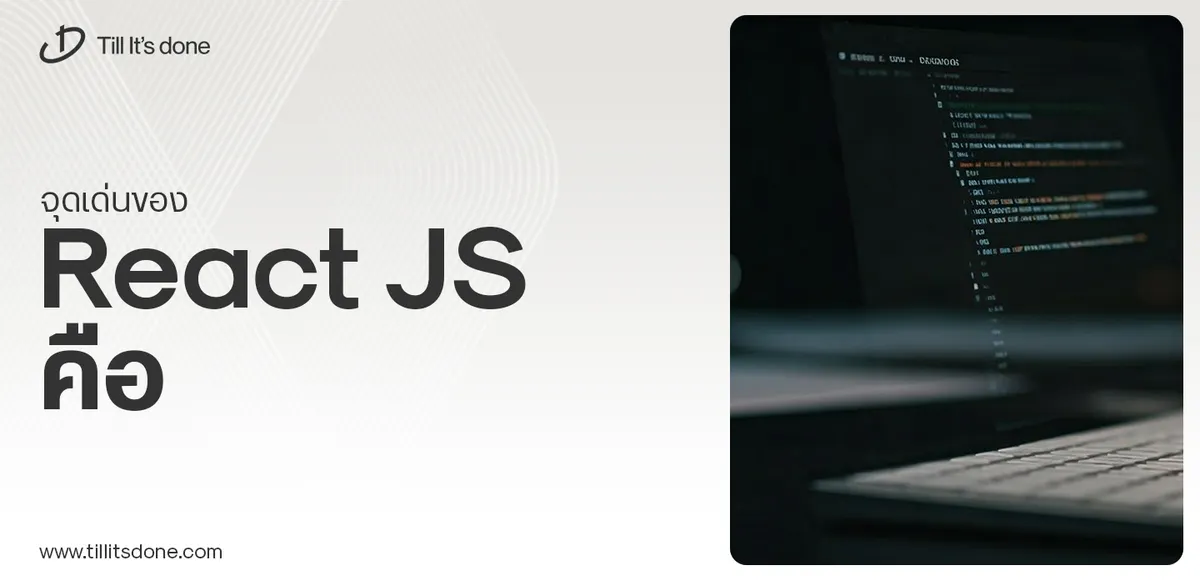
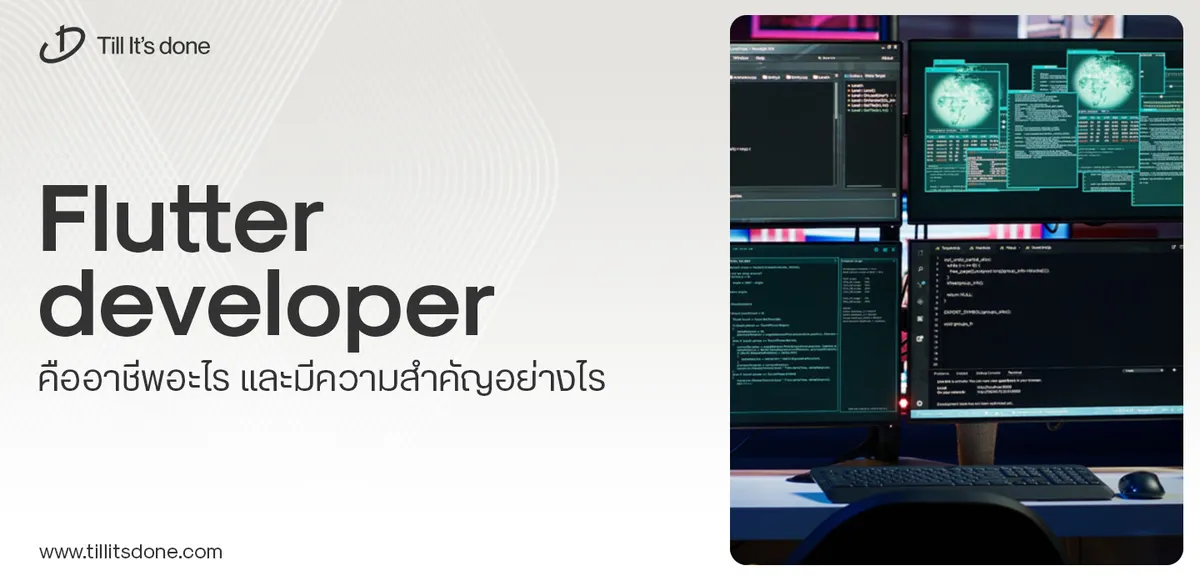
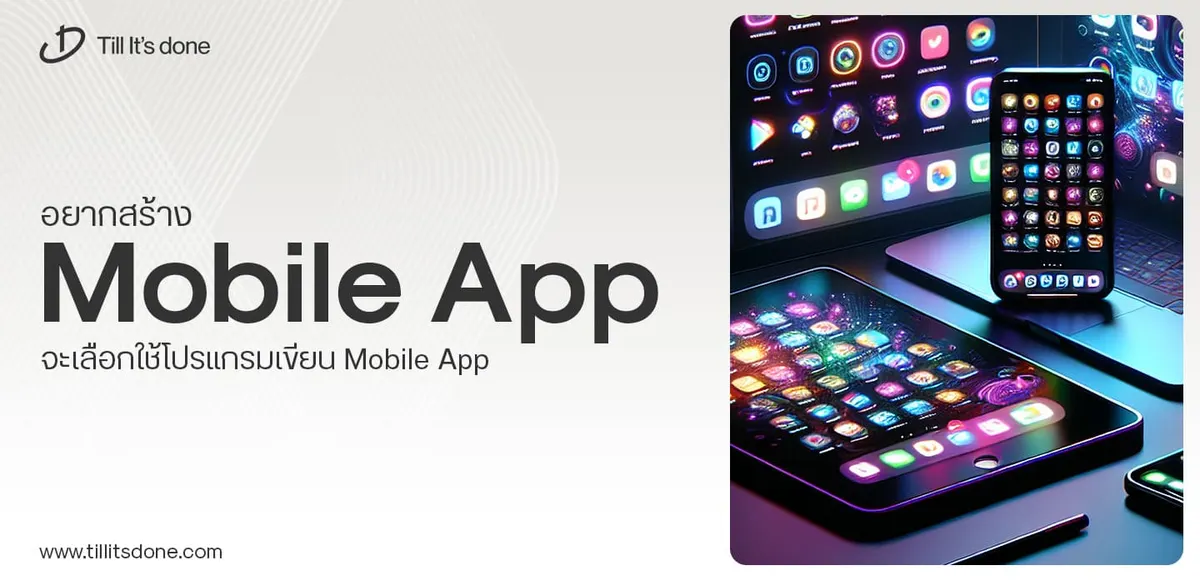
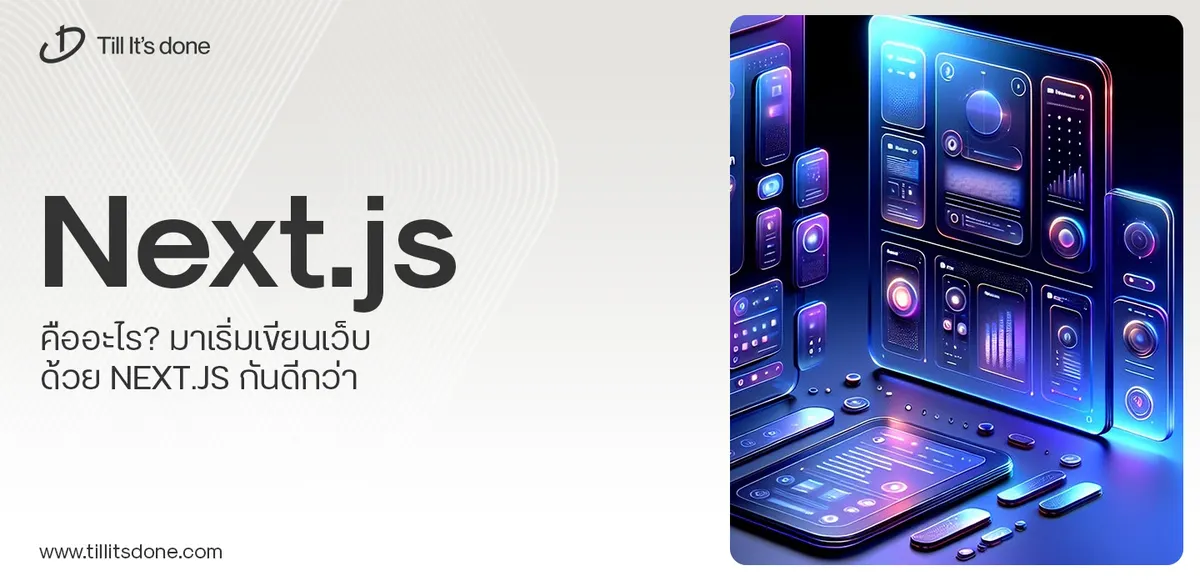
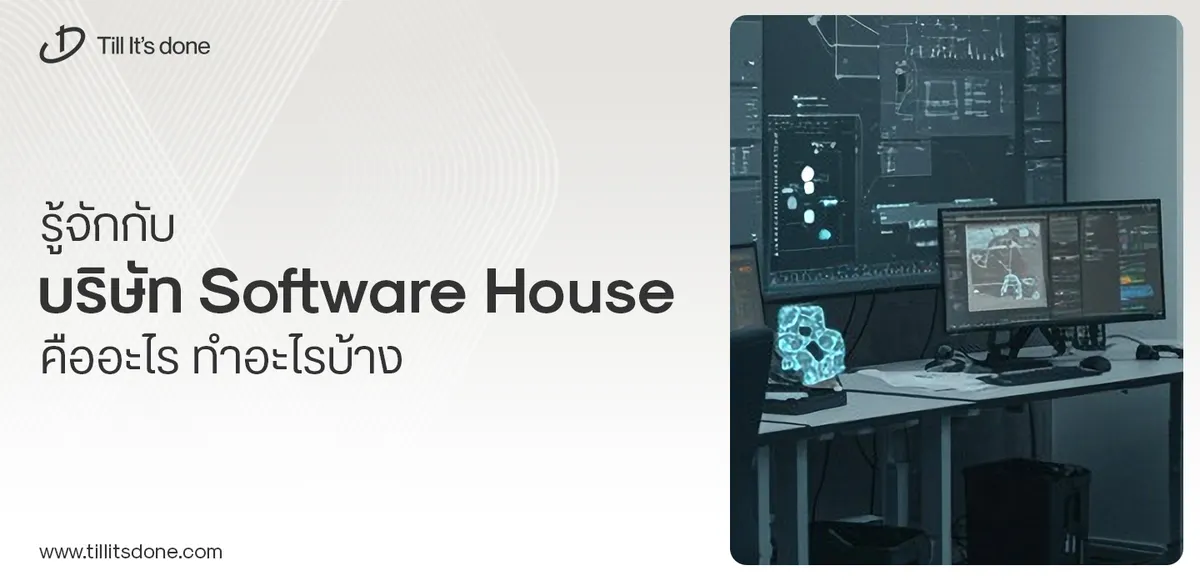
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.