- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
rick@tillitsdone.com
+66824564755
Handle Async Errors in Node.js Effectively
Learn battle-tested patterns and best practices for handling asynchronous errors in Node.js applications.
Discover how to implement robust error handling using try-catch, global handlers, and more.
Discover how to implement robust error handling using try-catch, global handlers, and more.

How to Handle Async Errors in Node.js Effectively
Dealing with asynchronous errors in Node.js can be tricky. One small oversight in error handling can bring down your entire application. Let’s explore some battle-tested patterns and best practices to handle async errors effectively.
Understanding Async Error Patterns
The asynchronous nature of Node.js means errors can occur in various contexts - Promises, async/await, callbacks, and event emitters. Each requires a specific approach to handle errors gracefully.
Key Strategies for Robust Error Handling
1. Using try-catch with async/await
async function fetchUserData(userId) { try { const user = await database.users.findById(userId); return user; } catch (error) { logger.error('Failed to fetch user:', error); throw new CustomError('UserFetchError', error.message); }}
2. Implementing Global Error Handlers
process.on('unhandledRejection', (reason, promise) => { console.error('Unhandled Rejection:', reason); // Proper error logging and handling});
process.on('uncaughtException', (error) => { console.error('Uncaught Exception:', error); // Graceful shutdown procedures process.exit(1);});
3. Creating Error Boundaries
class ErrorBoundary { async execute(fn) { try { return await fn(); } catch (error) { this.handleError(error); throw error; } }
handleError(error) { // Custom error handling logic logger.error(error); metrics.recordError(error); }}
Best Practices
- Always use async/await with try-catch
- Create custom error classes for better error handling
- Implement proper logging and monitoring
- Use middleware for REST API error handling
- Never ignore errors in promises or callbacks
Common Pitfalls to Avoid
- Forgetting to handle rejected promises
- Ignoring errors in event emitters
- Not implementing proper cleanup in catch blocks
- Missing error types in TypeScript implementations
Advanced Error Handling Patterns
// Error handler middleware for Expressapp.use((error, req, res, next) => { logger.error(error); res.status(error.status || 500).json({ error: { message: error.message, code: error.code } });});
Remember, effective error handling is not just about preventing crashes - it’s about maintaining system reliability and providing a great user experience.
JavaScript runtime for building scalable, high-performance server-side applications.


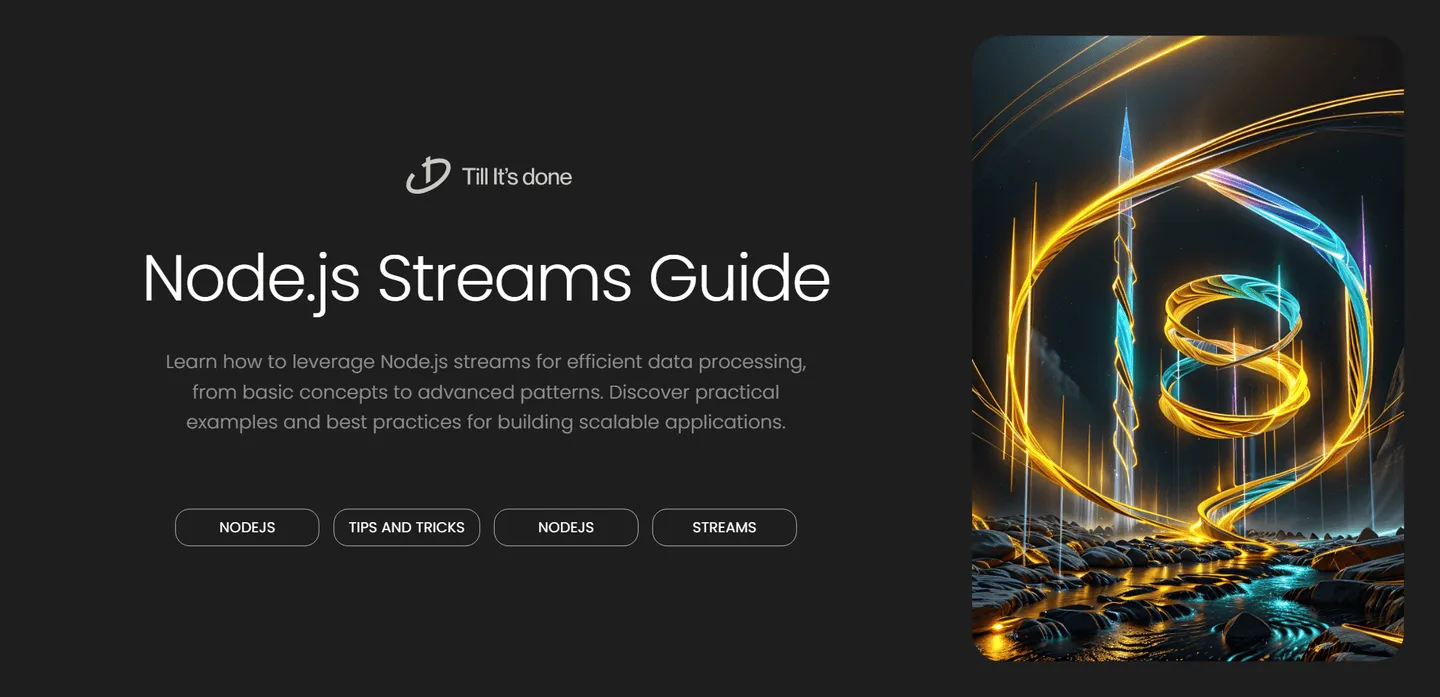



Talk with CEO
Ready to bring your web/app to life or boost your team with expert Thai developers?
Contact us today to discuss your needs, and let’s create tailored solutions to achieve your goals. We’re here to help at every step!
🖐️ Contact us 209 Articles
Explore Popular JavaScript library for building user interfaces with a component-based architecture.
169 Articles
Explore UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase.
150 Articles
Explore JavaScript runtime for building scalable, high-performance server-side applications.
60 Articles
Explore React framework enabling server-side rendering and static site generation for optimized performance.
40 Articles
Explore Superset of JavaScript adding static types for improved code quality and maintainability.
39 Articles
Explore Utility-first CSS framework for rapid UI development.
130 Articles
Explore Programming language known for its simplicity, concurrency model, and performance.
70 Articles
Explore Astro is an all-in-one web framework. It includes everything you need to create a website, built-in.
40 Articles
Explore Versatile testing framework for JavaScript applications supporting various test types.
337 Articles
Explore CSS3 is the latest version of Cascading Style Sheets, offering advanced styling features like animations, transitions, shadows, gradients, and responsive design.
Let's keep in Touch
Thank you for your interest in Tillitsdone! Whether you have a question about our services, want to discuss a potential project, or simply want to say hello, we're here and ready to assist you.
We'll be right here with you every step of the way.
We'll be right here with you every step of the way.
Contact Information
rick@tillitsdone.com+66824564755
Address
9 Phahonyothin Rd, Khlong Nueng, Khlong Luang District, Pathum Thani, Bangkok Thailand
We anticipate your communication and look forward to discussing how we can contribute to your business's success.
We'll be here, prepared to commence this promising collaboration.
We'll be here, prepared to commence this promising collaboration.
Frequently Asked Questions
Explore frequently asked questions about our products and services.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.