- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Node.js with MongoDB: Integration Tips
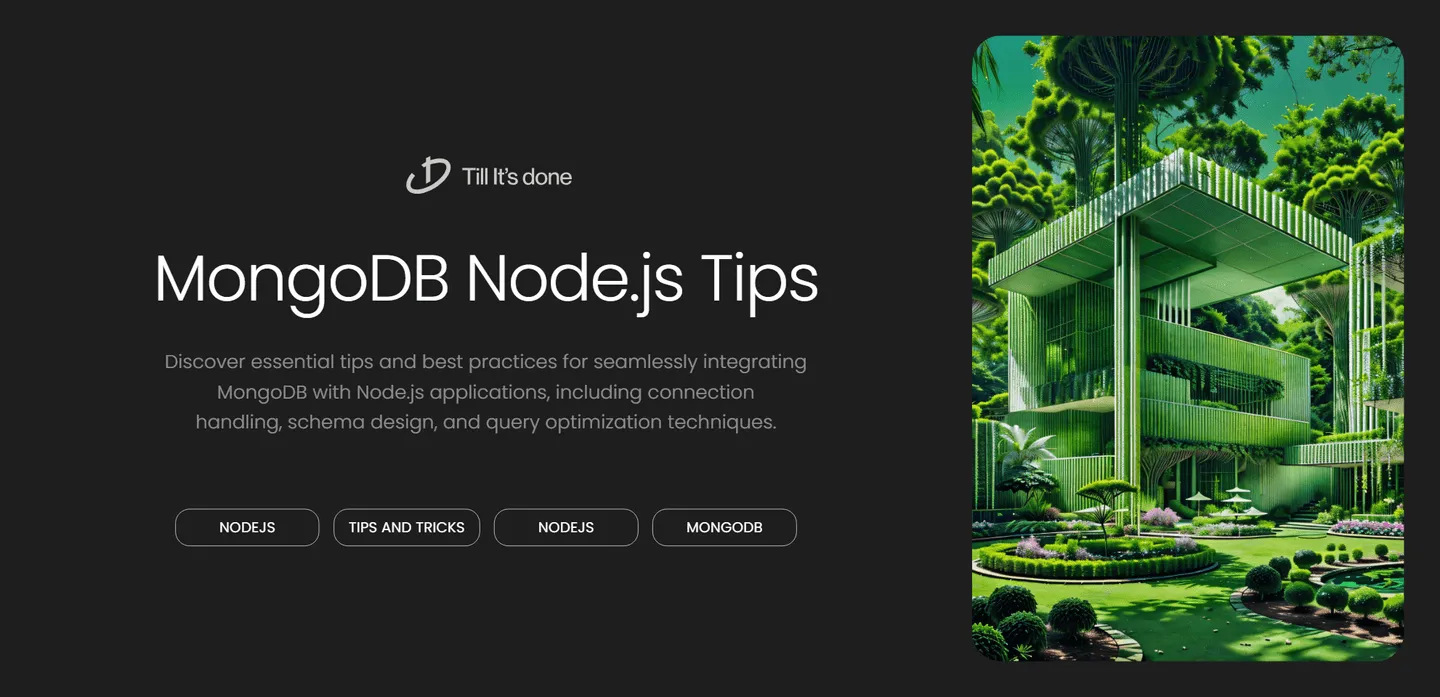
Using Node.js with MongoDB: Tips for Smooth Integration
MongoDB and Node.js make a powerful duo for modern web applications. After years of working with this stack, I’ve gathered some valuable insights that can help you avoid common pitfalls and optimize your database interactions. Let’s dive into some practical tips that will make your development journey smoother.
1. Connection Best Practices
The way you handle your MongoDB connection can significantly impact your application’s performance. Here’s my tried-and-true approach:
const mongoose = require('mongoose');
// Connection optionsconst options = { useNewUrlParser: true, useUnifiedTopology: true, serverSelectionTimeoutMS: 5000, maxPoolSize: 10};
// Connection with retry logicasync function connectWithRetry() { try { await mongoose.connect(process.env.MONGODB_URI, options); console.log('MongoDB connected successfully'); } catch (err) { console.error('MongoDB connection error:', err); setTimeout(connectWithRetry, 5000); }}
2. Schema Design Tips
One thing I learned the hard way is the importance of proper schema design. Here’s a pattern I often use for flexible yet structured schemas:
const productSchema = new mongoose.Schema({ name: { type: String, required: true, trim: true }, metadata: { type: Map, of: String, default: new Map() }}, { timestamps: true, strict: false});
3. Query Optimization Techniques
Efficient queries are crucial for performance. I always follow these guidelines:
// Instead of thisconst users = await User.find({status: 'active'});
// Do thisconst users = await User.find({status: 'active'}) .select('name email') // Select only needed fields .limit(20) .lean(); // Convert to plain JavaScript objects
4. Error Handling and Validation
Here’s a robust approach to handling MongoDB operations:
try { const session = await mongoose.startSession(); session.startTransaction();
try { const result = await YourModel.create([data], { session }); await session.commitTransaction(); return result; } catch (error) { await session.abortTransaction(); throw error; } finally { session.endSession(); }} catch (error) { console.error('Transaction failed:', error); throw new Error('Failed to process the request');}
5. Performance Monitoring
Always monitor your database performance. I recommend using MongoDB’s built-in profiler:
// Enable profiling for slow queriesdb.setProfilingLevel(1, { slowms: 100 });
Conclusion
Remember, the key to successful MongoDB integration with Node.js lies in understanding these fundamental concepts and applying them thoughtfully in your applications. Start with these practices, and you’ll build more reliable and efficient applications.
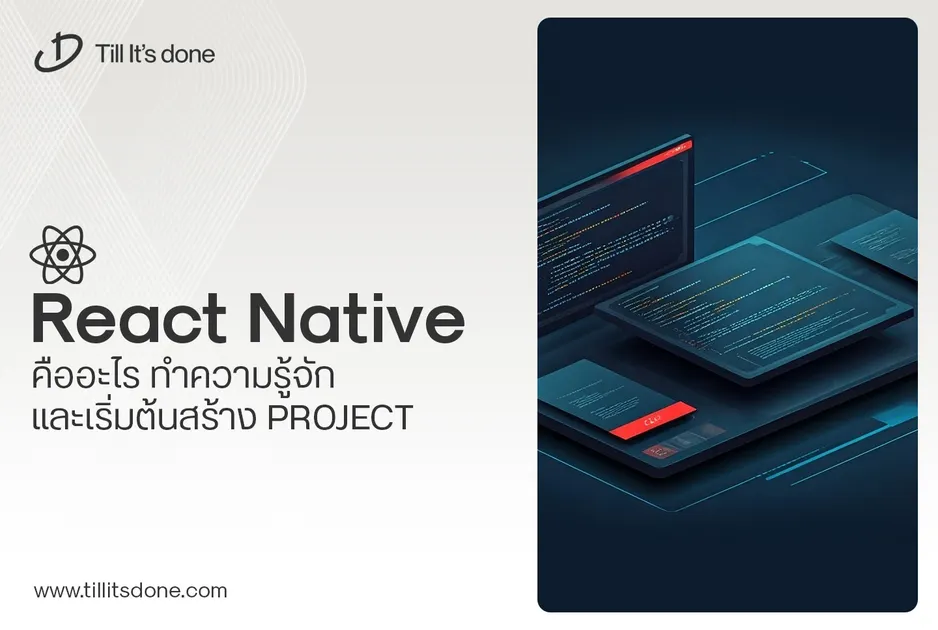
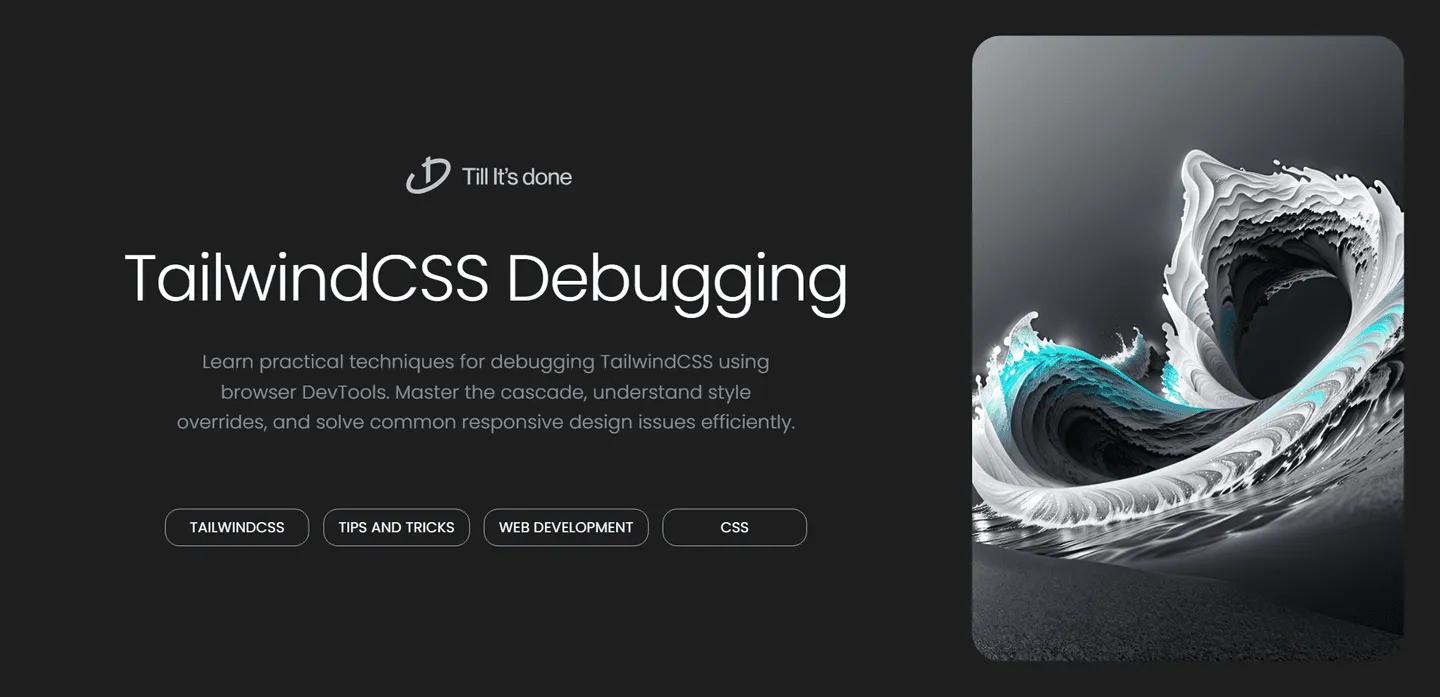
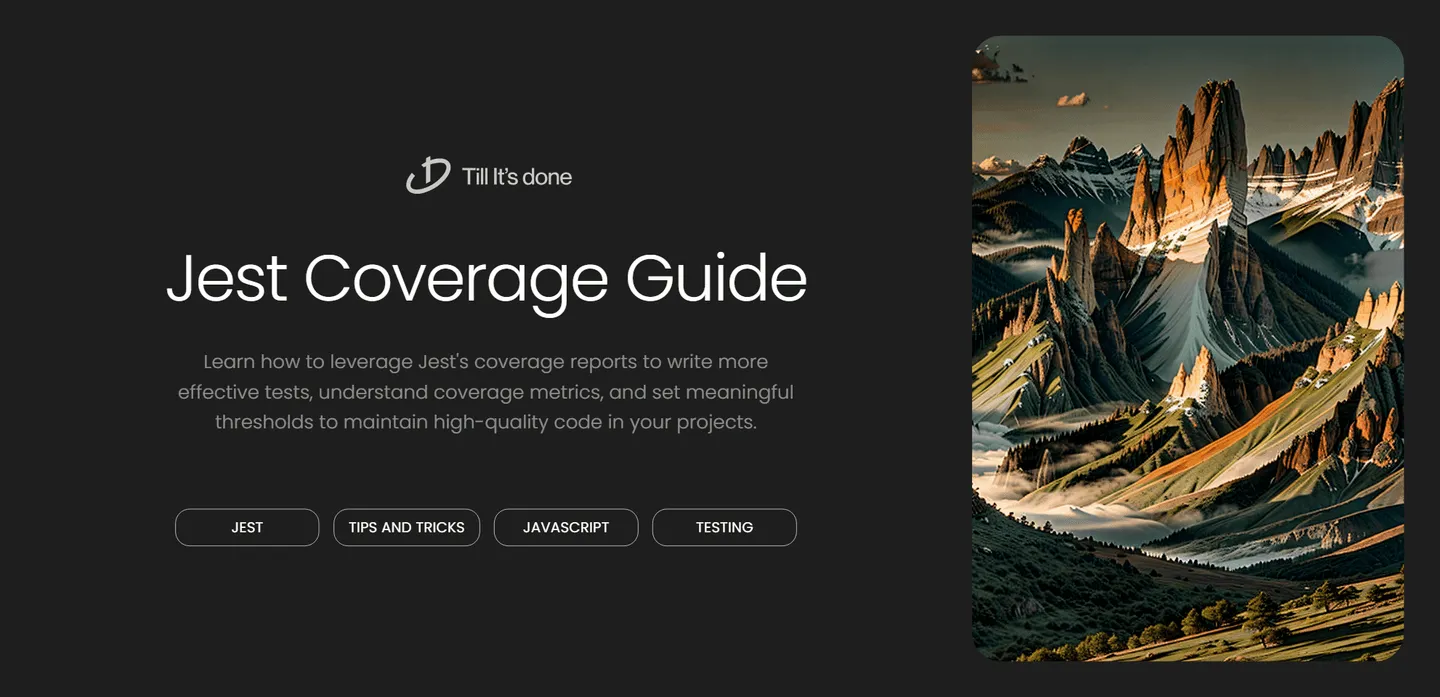
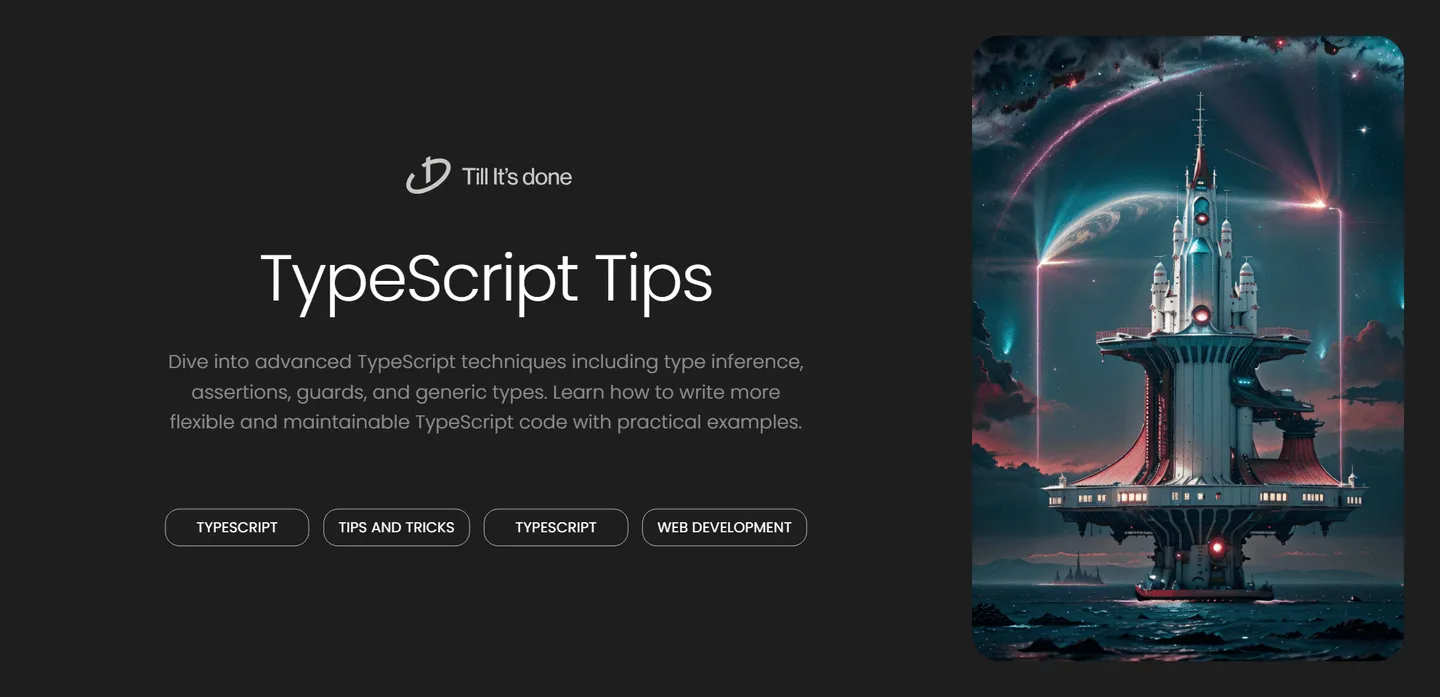
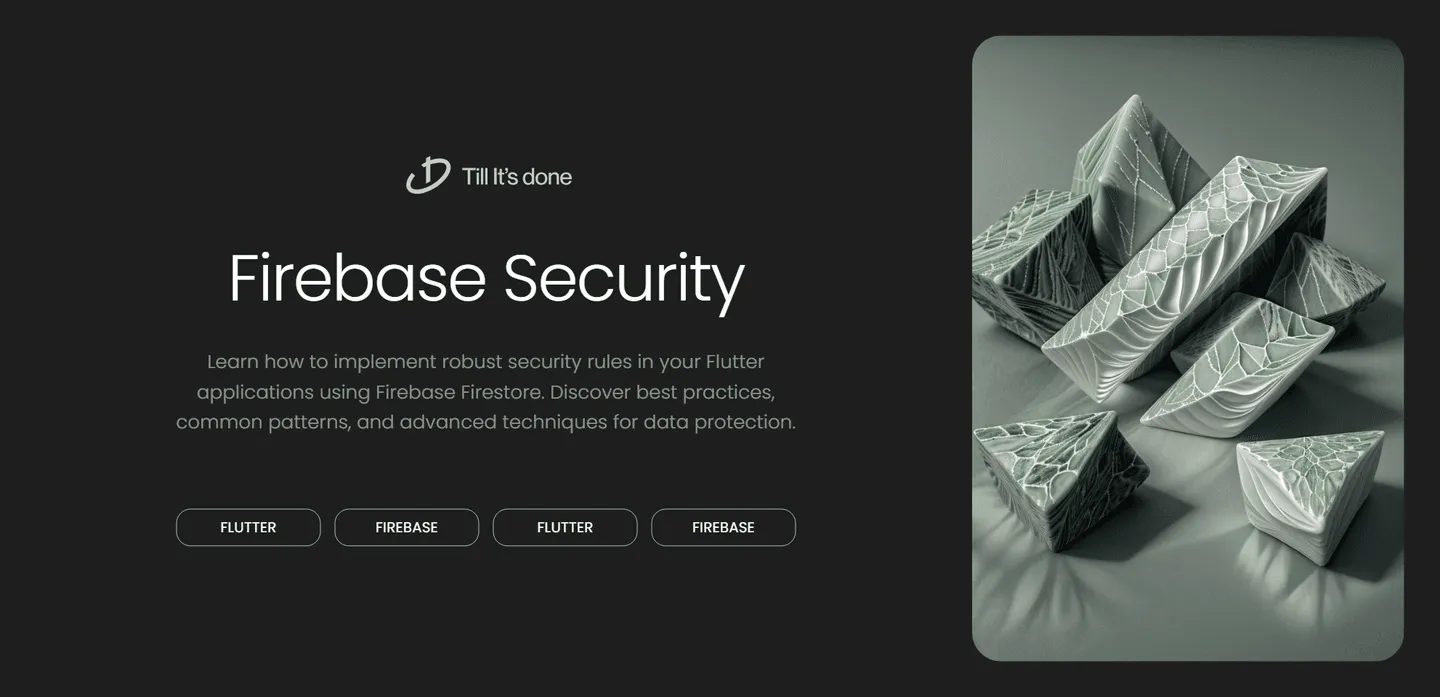
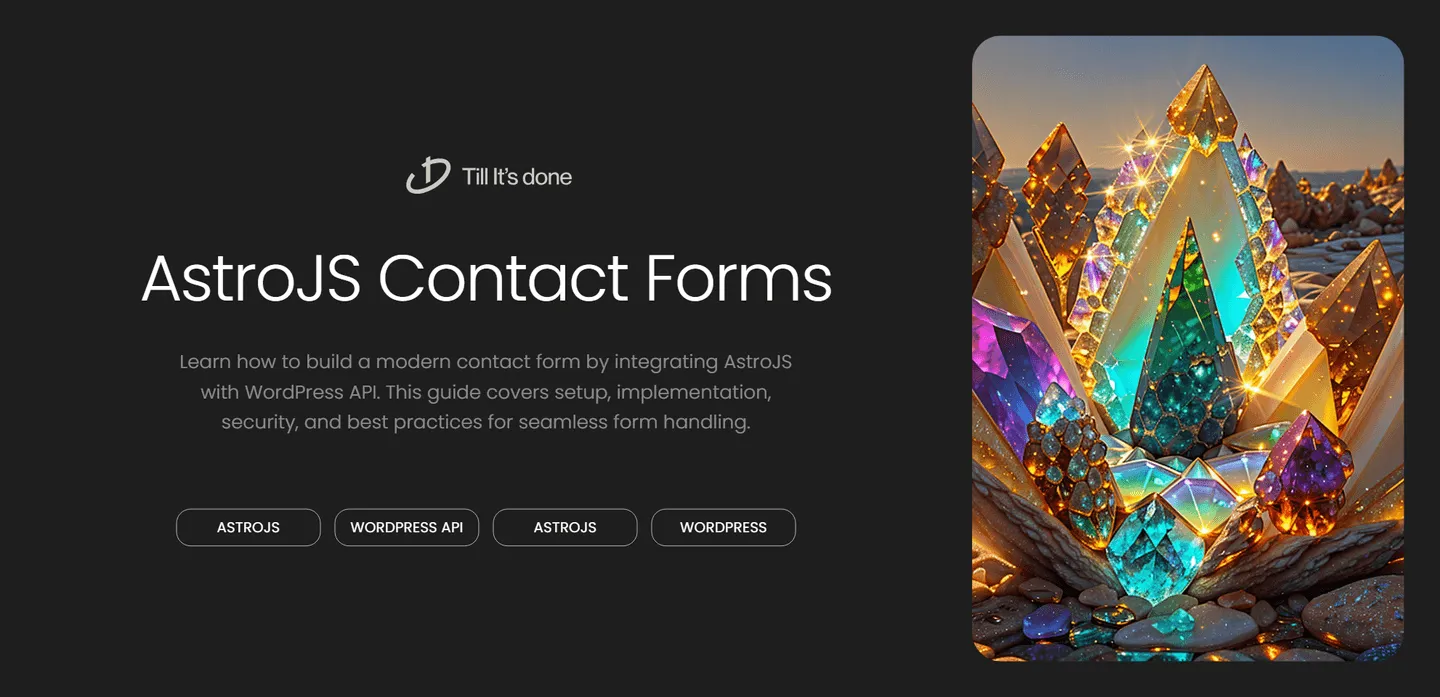
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.