- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Tips for Debugging Node.js Apps Like a Pro
Learn strategic console logging, error handling, and performance profiling methods.
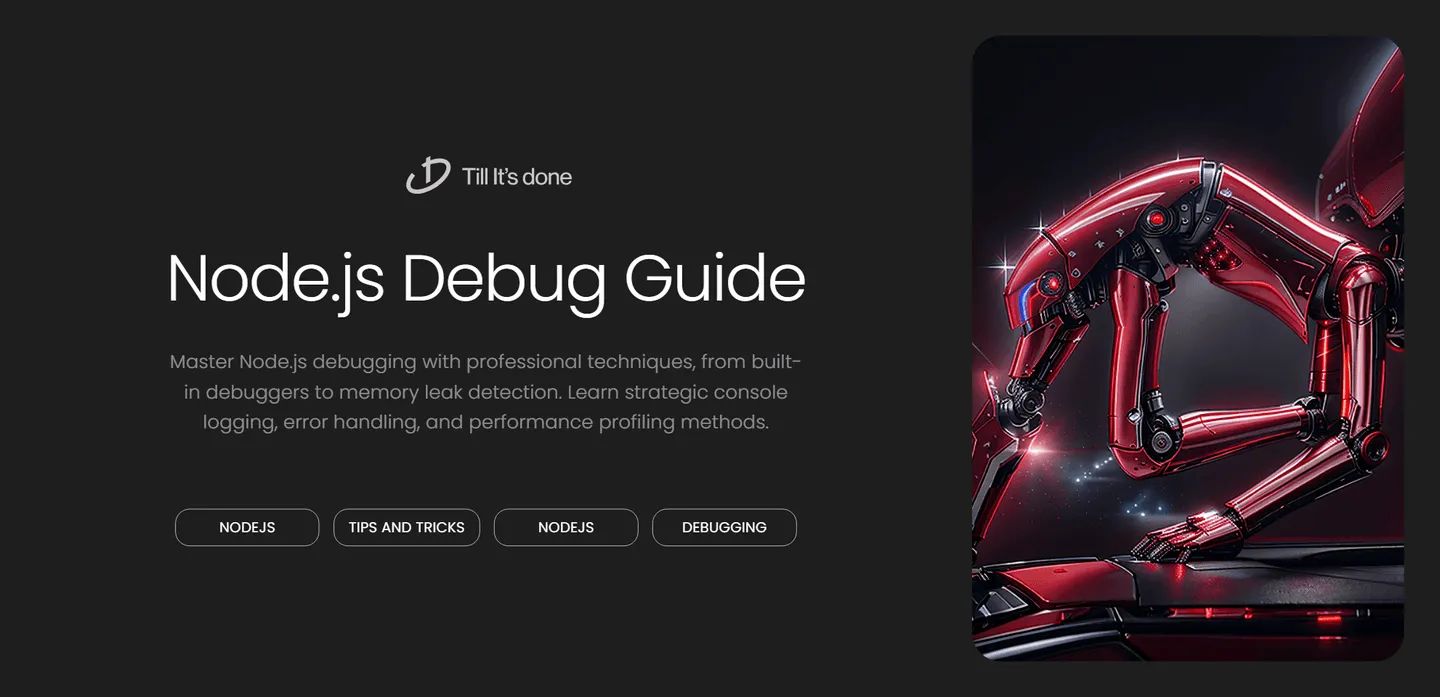
Tips for Debugging Node.js Applications Like a Pro
As a Node.js developer, debugging is an essential skill that can make or break your productivity. Let’s dive into some professional debugging techniques that will help you squash bugs more efficiently.
1. Mastering the Node.js Debugger
The built-in Node.js debugger is your first line of defense. Here’s how to use it effectively:
// Add this line where you want to pause executiondebugger;
// Run your application with the inspect flagnode inspect app.js
2. Strategic Console Logging
While console.log()
is common, there are more powerful console methods:
console.table(complexObject); // Displays objects in table formatconsole.time('operation') && console.timeEnd('operation'); // Measure execution timeconsole.trace(); // Print stack trace
3. Error Handling Best Practices
Implement proper error handling to make debugging easier:
try { await riskyOperation();} catch (error) { console.error({ message: error.message, stack: error.stack, context: 'Additional debugging info' });}
4. Memory Leak Detection
Monitor your application’s memory usage:
const used = process.memoryUsage();console.table({ rss: `${Math.round(used.rss / 1024 / 1024)} MB`, heapTotal: `${Math.round(used.heapTotal / 1024 / 1024)} MB`, heapUsed: `${Math.round(used.heapUsed / 1024 / 1024)} MB`});
5. Performance Profiling
Use the built-in profiler to identify bottlenecks:
node --prof app.jsnode --prof-process isolate-0xnnnnnnnnnnnn-v8.log > processed.txt
6. Environment-Specific Debugging
Set up debugging configurations for different environments:
const debug = require('debug')('app:server');debug('Server starting on port %d', port);
7. Automated Testing
Write tests that help prevent bugs:
describe('User Authentication', () => { it('should validate user credentials', async () => { // Your test code });});
8. Logging Solutions
Implement structured logging for better debugging in production:
const winston = require('winston');const logger = winston.createLogger({ level: 'debug', format: winston.format.json(), transports: [new winston.transports.File({ filename: 'debug.log' })]});
Remember, the key to effective debugging is being systematic and using the right tool for the job. Start with simple techniques and gradually move to more advanced ones as needed.
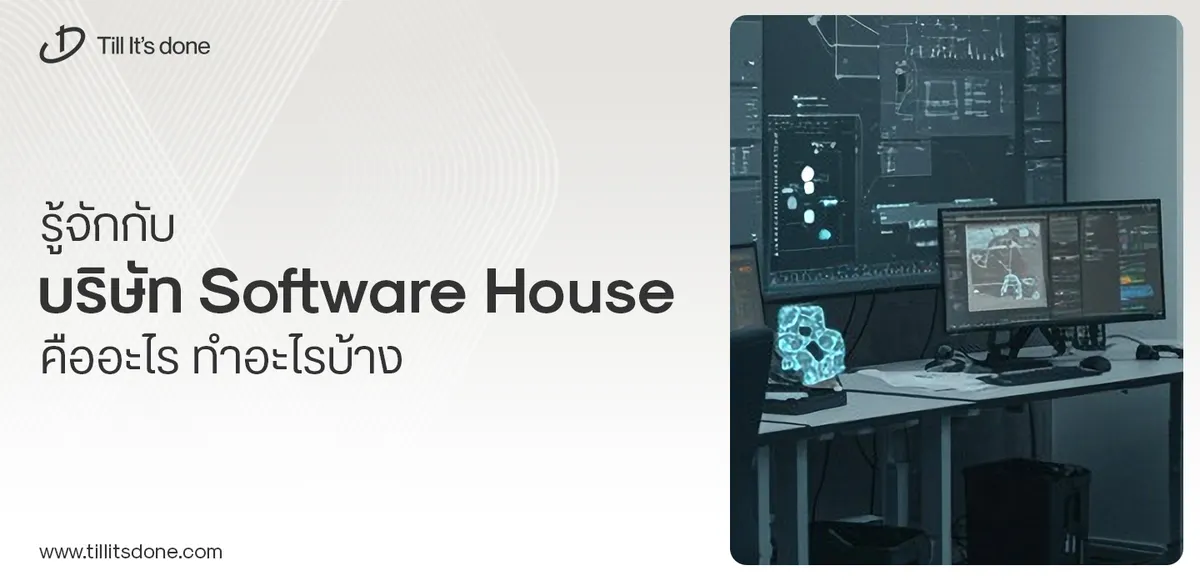
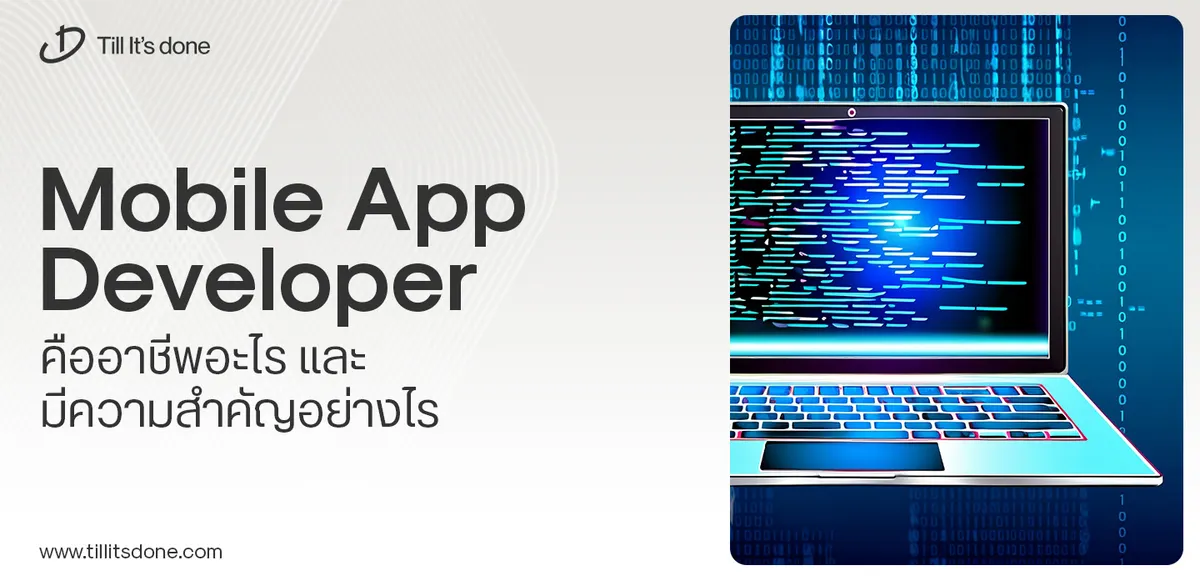
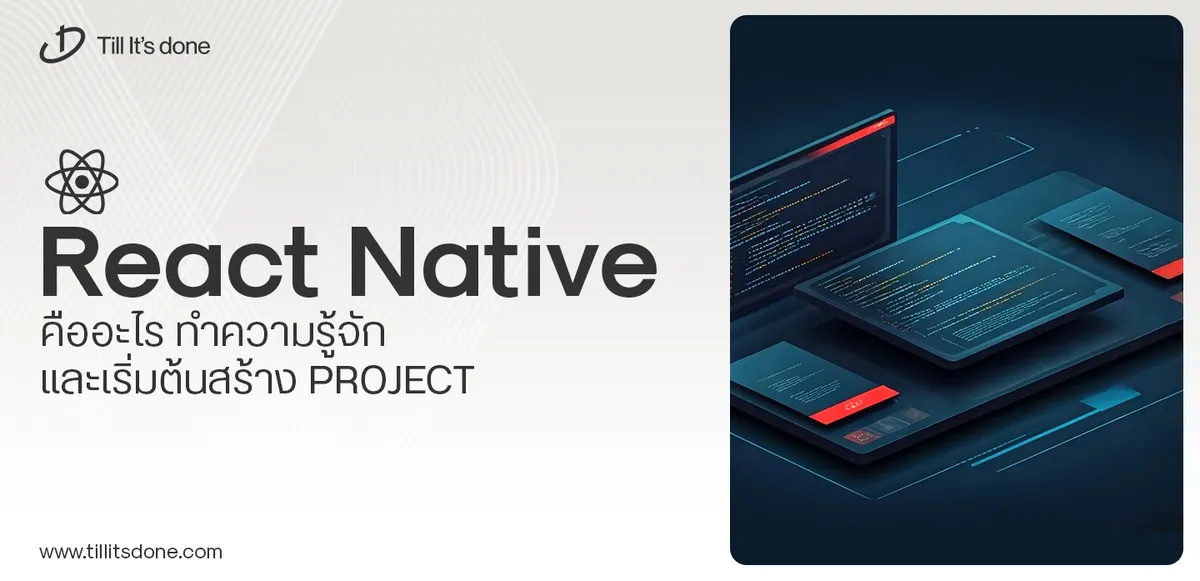
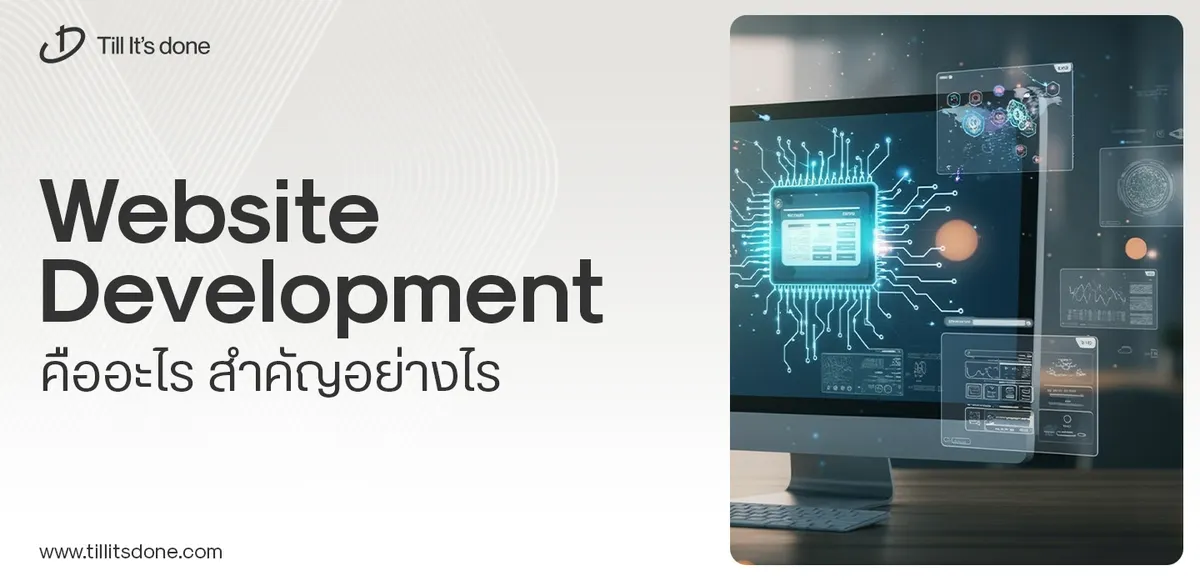
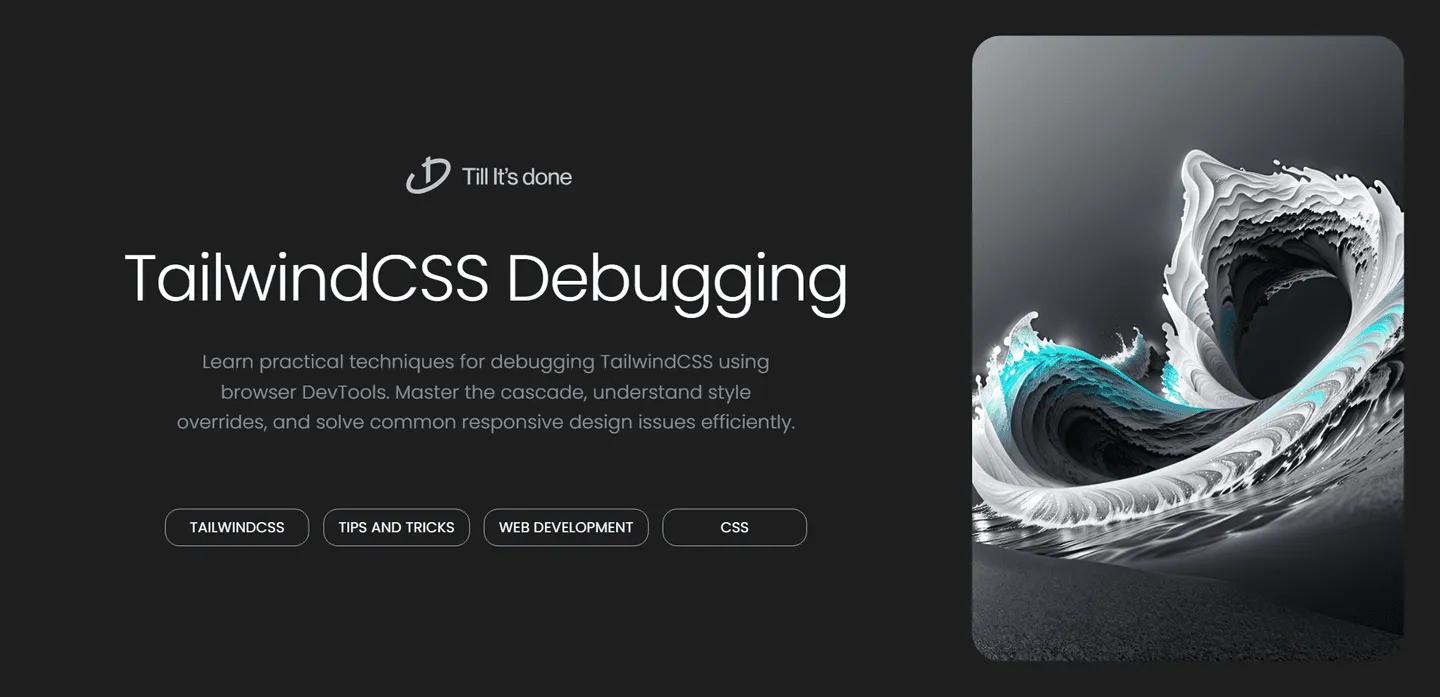
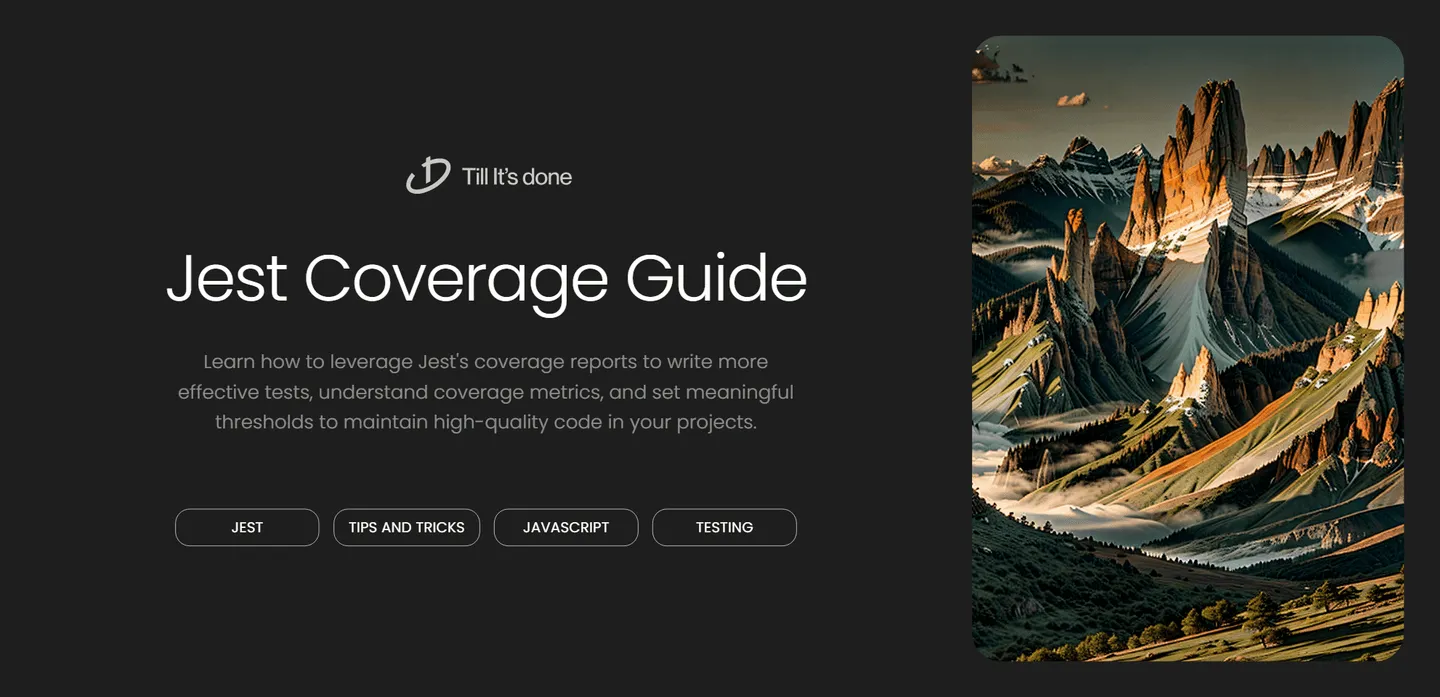
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.