- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Introduction to GORM: A Beginner's Guide to ORM
This beginner-friendly guide covers basic concepts, setup, and best practices for efficient database operations in Go.

Introduction to GORM: A Beginner’s Guide to ORM in Golang
If you’re diving into Golang web development, you’ll quickly realize that managing database operations efficiently is crucial. This is where GORM comes into play – a fantastic Object-Relational Mapping (ORM) library that makes database interactions in Go both elegant and straightforward.
What is GORM?
GORM is the most widely-used ORM library for Golang, providing a beautiful and simple interface to interact with your database. Instead of writing raw SQL queries, you can work with database operations using Go structs – making your code more maintainable and less prone to errors.
Key Features That Make GORM Awesome
Before we dive into the code, let’s understand why GORM has become the go-to choice for many developers:
- Auto Migrations: GORM automatically handles database schema changes
- Associations: Easily manage relationships between models
- Hooks: Customize your data operations with before/after hooks
- Transactions: Built-in support for database transactions
- Multiple Databases: Works with MySQL, PostgreSQL, SQLite, and SQL Server
Getting Started with GORM
Let’s walk through setting up GORM in your project. First, you’ll need to install GORM:
go get -u gorm.io/gormgo get -u gorm.io/driver/mysql // or your preferred database driver
Here’s a simple example to get you started:
package main
import ( "gorm.io/gorm" "gorm.io/driver/mysql")
type User struct { ID uint `gorm:"primaryKey"` Name string Email string `gorm:"uniqueIndex"` Age uint8}
func main() { dsn := "user:password@tcp(127.0.0.1:3306)/dbname?charset=utf8mb4&parseTime=True&loc=Local" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { panic("failed to connect database") }
// Auto Migrate db.AutoMigrate(&User{})
// Create user := User{Name: "John Doe", Email: "john@example.com", Age: 25} db.Create(&user)}
Best Practices and Common Patterns
When working with GORM, keep these best practices in mind:
- Always define proper model structures with appropriate tags
- Use transactions for operations that need to be atomic
- Implement proper error handling
- Use preloading wisely to avoid N+1 query problems
- Index frequently queried fields
Here’s a more advanced example incorporating these practices:
type Order struct { ID uint `gorm:"primaryKey"` UserID uint `gorm:"index"` Products []Product `gorm:"many2many:order_products;"` Total float64 CreatedAt time.Time}
func CreateOrder(db *gorm.DB, order *Order) error { return db.Transaction(func(tx *gorm.DB) error { if err := tx.Create(order).Error; err != nil { return err }
// Additional operations within the transaction return nil })}
Conclusion
GORM simplifies database operations in Go while providing powerful features that help you build robust applications. As you continue your journey with GORM, you’ll discover more advanced features like custom hooks, scopes, and complex associations that make it an invaluable tool in your Go development toolkit.




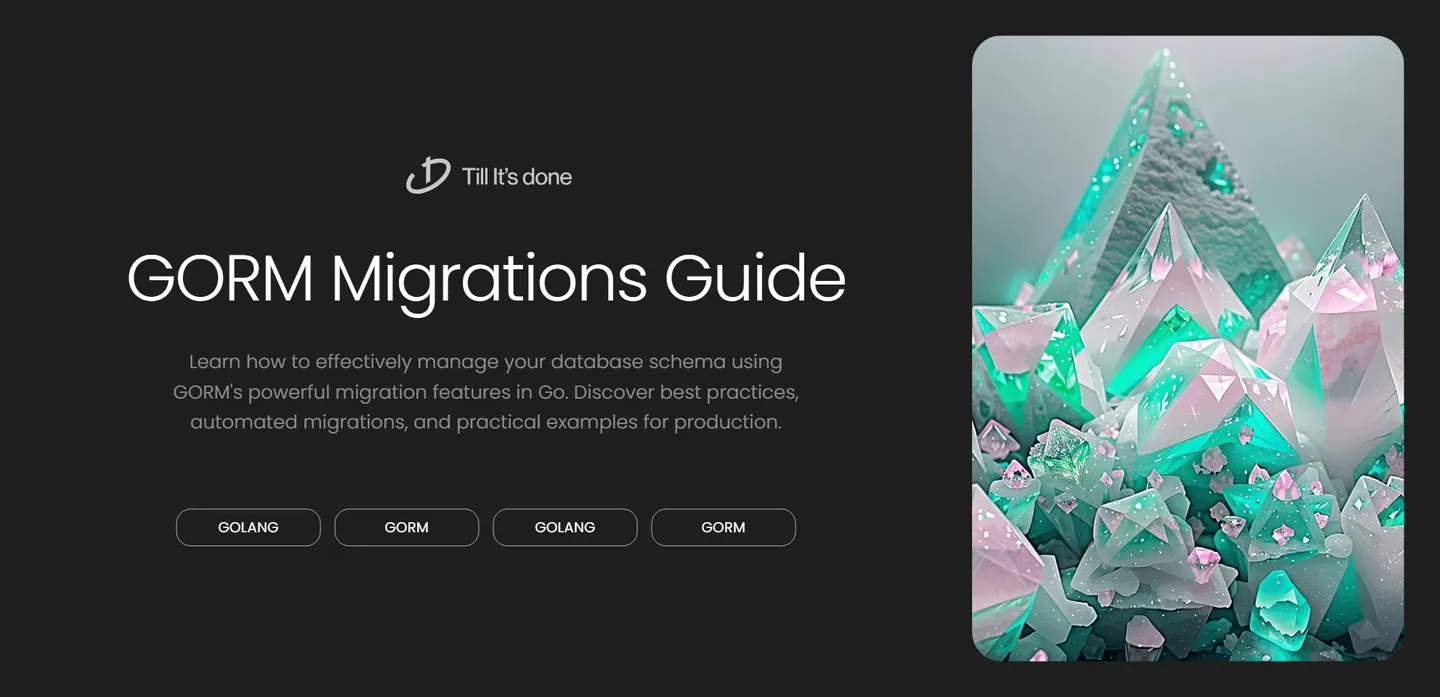

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.