- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using GORM with PostgreSQL: Tips and Tricks
Learn about connection pooling, batch operations, and advanced features for better performance.

Using GORM with PostgreSQL: Tips and Tricks
Working with databases in Go becomes a breeze when you combine GORM with PostgreSQL. After spending countless hours optimizing database operations in production environments, I’ve gathered some valuable insights that I’m excited to share with you. Let’s dive into some practical tips and tricks that will help you make the most of this powerful combination.
Setting Up the Perfect Connection
First things first, let’s talk about establishing a robust database connection. Here’s a battle-tested configuration that’s served me well:
dsn := "host=localhost user=gorm password=gorm dbname=gorm port=5432 sslmode=disable TimeZone=Asia/Shanghai"db, err := gorm.Open(postgres.Open(dsn), &gorm.Config{ PrepareStmt: true, Logger: logger.Default.LogMode(logger.Info),})
One crucial tip is to set up connection pooling correctly. I’ve found these settings to work well for most applications:
sqlDB, err := db.DB()sqlDB.SetMaxIdleConns(10)sqlDB.SetMaxOpenConns(100)sqlDB.SetConnMaxLifetime(time.Hour)
Performance Optimization Techniques
Batch Operations
When working with large datasets, batch operations can significantly improve performance. Here’s my go-to approach:
// Batch insert exampleusers := make([]User, 0, 1000)db.CreateInBatches(users, 100)
Smart Indexing Strategies
Indexes are crucial for performance, but they come with trade-offs. I’ve learned to:
- Create indexes only for frequently queried columns
- Use composite indexes for common query combinations
- Regularly analyze index usage with PostgreSQL’s built-in tools
Efficient Query Patterns
Here’s a pattern I frequently use for optimized queries:
type Result struct { ID uint Name string Email string}
var results []Resultdb.Model(&User{}). Select("id", "name", "email"). Where("created_at > ?", lastWeek). Scan(&results)
Advanced Features and Best Practices
Custom Hooks
One of my favorite features is GORM’s hooks system. I use it for automatic timestamps and data validation:
func (u *User) BeforeCreate(tx *gorm.DB) error { u.UUID = uuid.New() return nil}
Handling Relationships
When dealing with relationships, always remember to:
- Use preloading judiciously
- Consider using joins for better performance
- Implement proper foreign key constraints
Error Handling and Transactions
Always wrap critical operations in transactions:
err := db.Transaction(func(tx *gorm.DB) error { if err := tx.Create(&order).Error; err != nil { return err } return tx.Create(&payment).Error})
Monitoring and Maintenance
Regular maintenance is key to keeping your database healthy. I recommend:
- Setting up query logging for development
- Implementing proper metrics collection
- Regular vacuum and analyze operations
- Monitoring connection pool usage




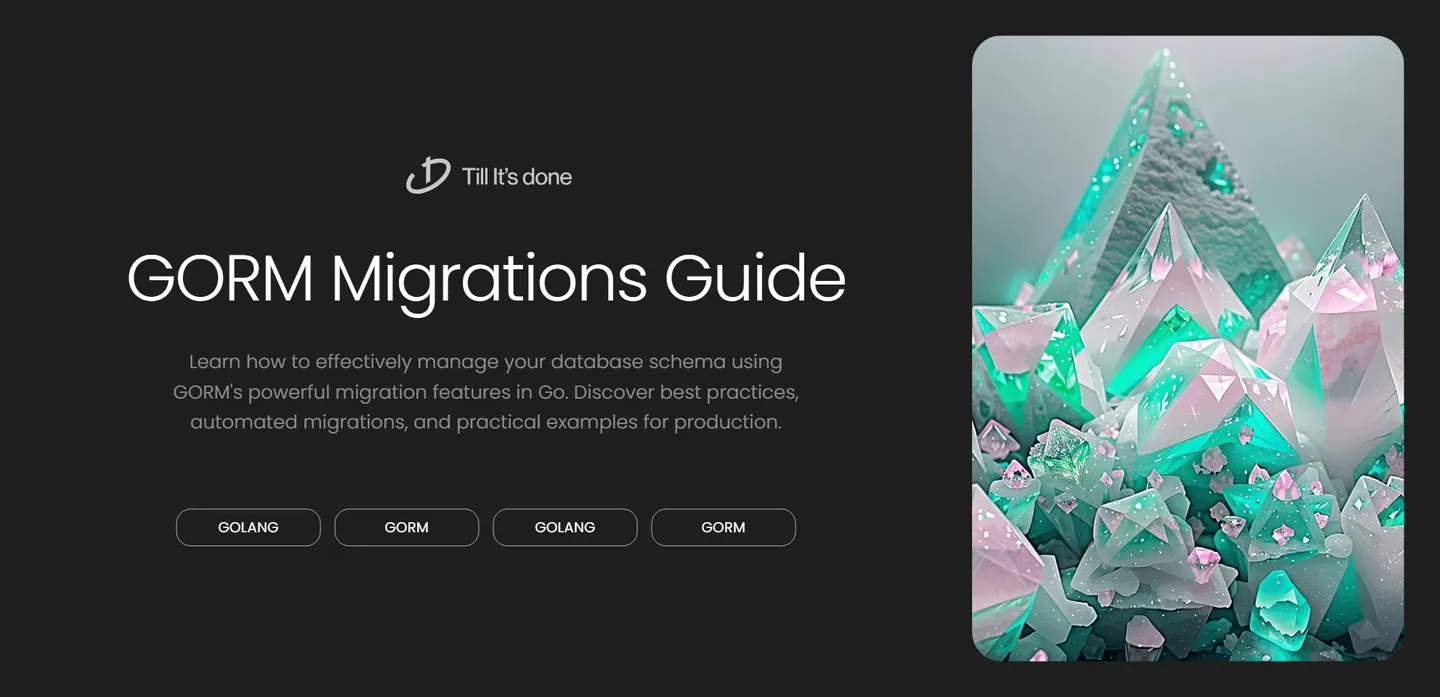

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.