- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Set Up and Configure GORM in Go Project
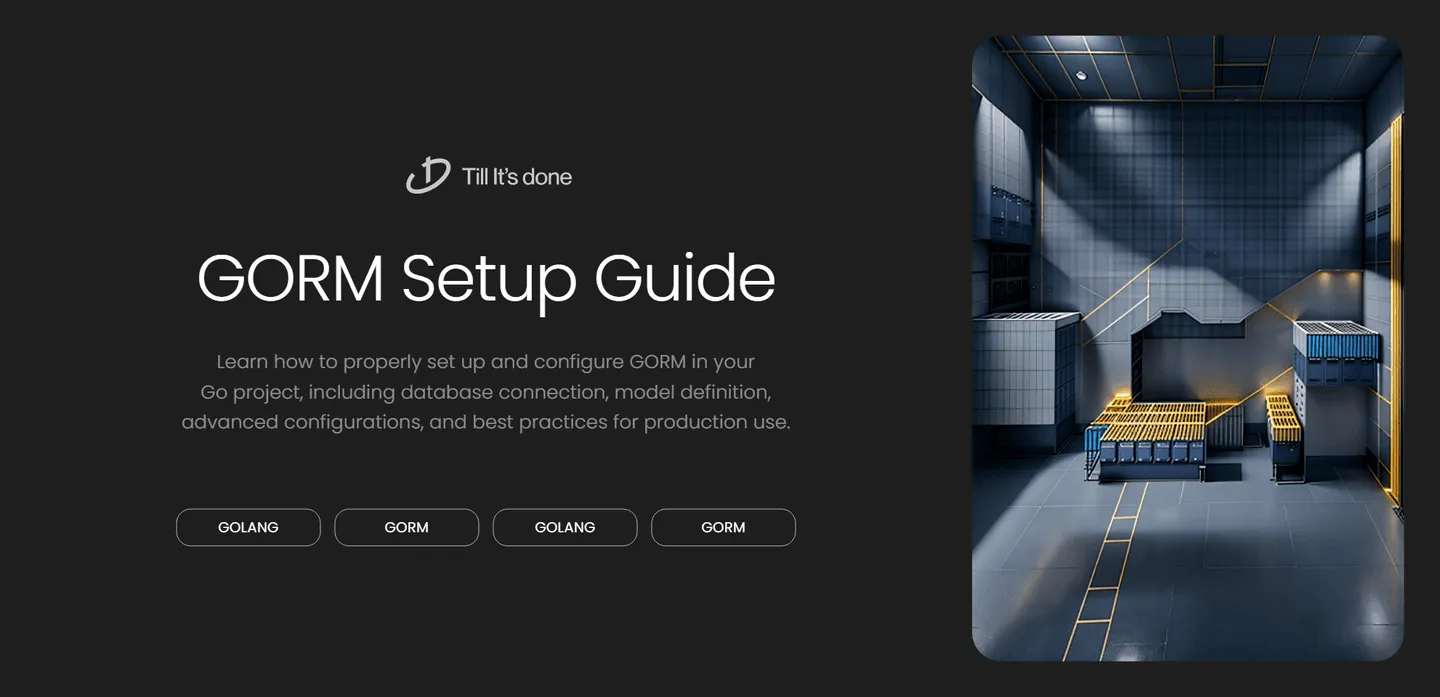
How to Set Up and Configure GORM in Your Go Project
Setting up a robust database layer in your Go application is crucial for building scalable and maintainable systems. GORM, a fantastic ORM library for Golang, makes database operations a breeze while providing powerful features out of the box. Let’s dive into how you can set up and configure GORM in your Go project.
Prerequisites
Before we begin, make sure you have:
- Go installed on your system
- A running database instance (MySQL, PostgreSQL, or SQLite)
- Basic understanding of Go programming
Installing GORM
First things first, let’s get GORM installed in your project. Open your terminal and run:
go get -u gorm.io/gormgo get -u gorm.io/driver/mysql // For MySQL// orgo get -u gorm.io/driver/postgres // For PostgreSQL// orgo get -u gorm.io/driver/sqlite // For SQLite
Basic Configuration
Let’s create a basic database configuration. Here’s a simple example using MySQL:
package database
import ( "fmt" "log" "os"
"gorm.io/driver/mysql" "gorm.io/gorm")
func InitDB() *gorm.DB { dsn := fmt.Sprintf("%s:%s@tcp(%s:%s)/%s?charset=utf8mb4&parseTime=True&loc=Local", os.Getenv("DB_USER"), os.Getenv("DB_PASSWORD"), os.Getenv("DB_HOST"), os.Getenv("DB_PORT"), os.Getenv("DB_NAME"), )
db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { log.Fatal("Failed to connect to database:", err) }
return db}
Defining Models
One of GORM’s strengths is its intuitive model definition. Here’s how you can define a simple user model:
type User struct { gorm.Model Name string `gorm:"size:255;not null"` Email string `gorm:"size:255;not null;unique"` Age uint `gorm:"not null"` IsActive bool `gorm:"default:true"`}
Advanced Configuration
For production applications, you might want to fine-tune your GORM configuration:
func InitDB() *gorm.DB { db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{ Logger: logger.Default.LogMode(logger.Info), NamingStrategy: schema.NamingStrategy{ SingularTable: true, NoLowerCase: true, }, AllowGlobalUpdate: false, })
sqlDB, err := db.DB() if err != nil { log.Fatal(err) }
// Set connection pool settings sqlDB.SetMaxIdleConns(10) sqlDB.SetMaxOpenConns(100) sqlDB.SetConnMaxLifetime(time.Hour)
return db}
Best Practices and Tips
- Always use environment variables for database credentials
- Implement proper error handling
- Set up connection pooling based on your application’s needs
- Use transactions for critical operations
- Create database indexes for frequently queried fields
Remember to close your database connection when your application shuts down:
sqlDB, err := db.DB()defer sqlDB.Close()
Conclusion
Setting up GORM correctly lays the foundation for a robust database layer in your Go application. By following these configurations and best practices, you’ll have a reliable and efficient database setup ready for your project’s needs.
Remember to regularly check GORM’s official documentation for updates and new features that might benefit your application. Happy coding!
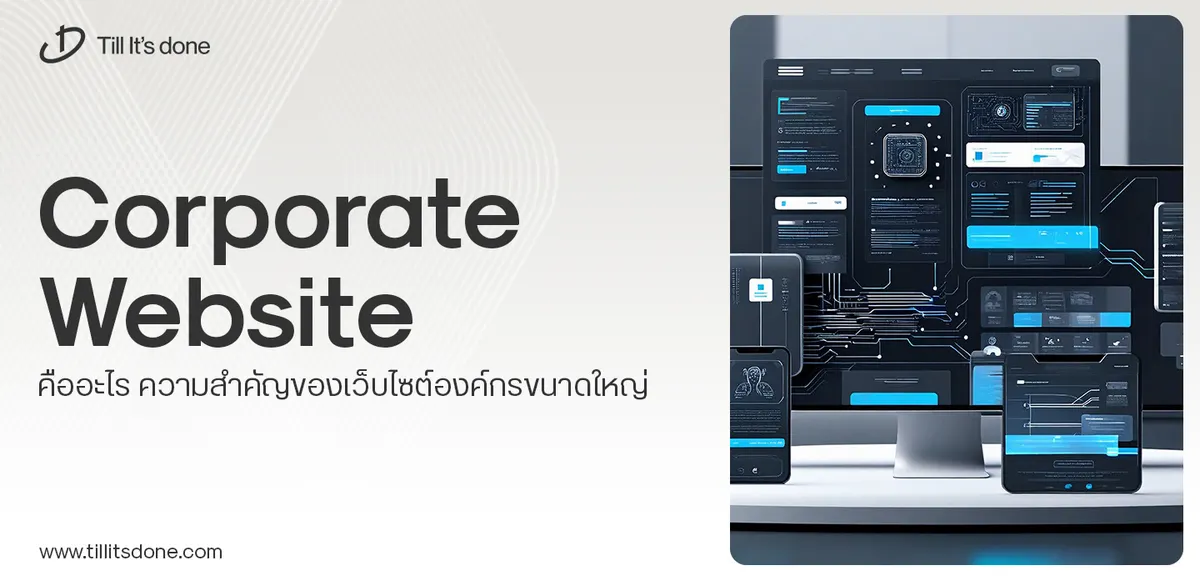
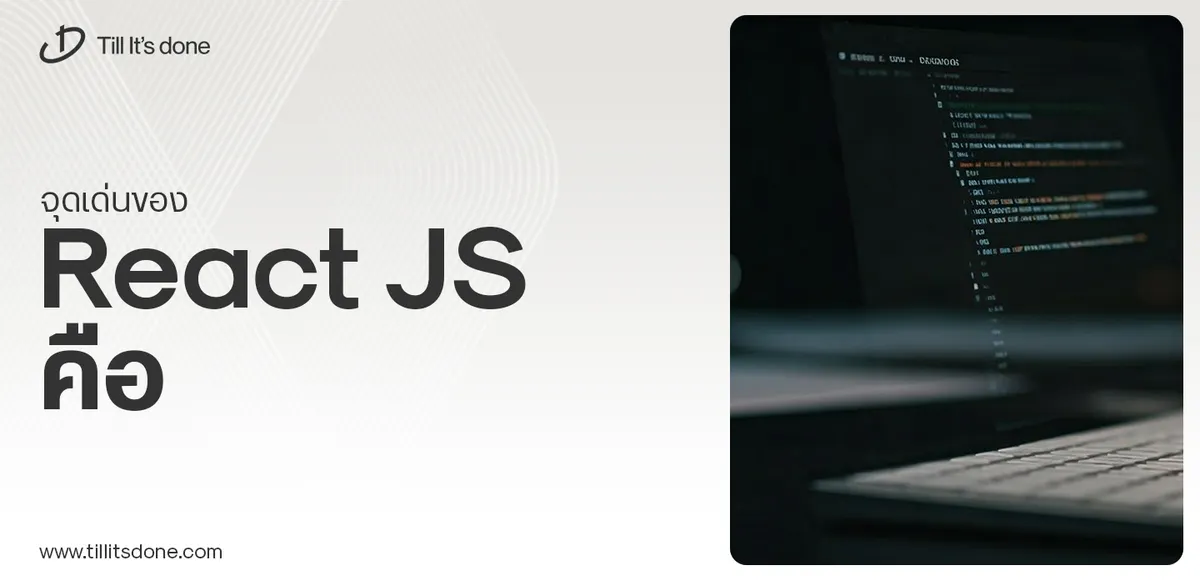
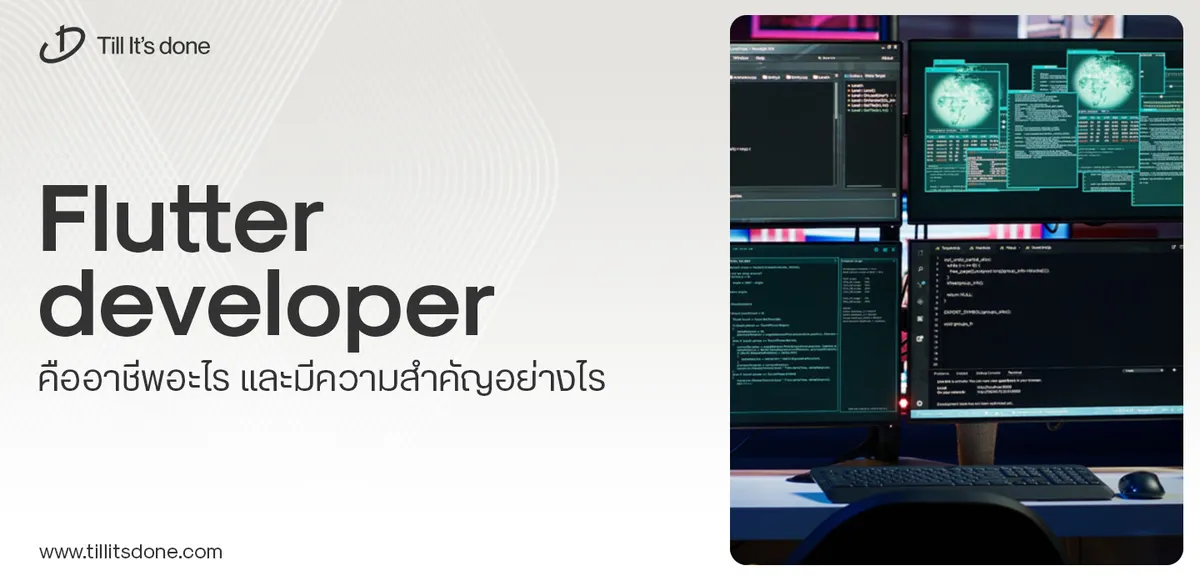
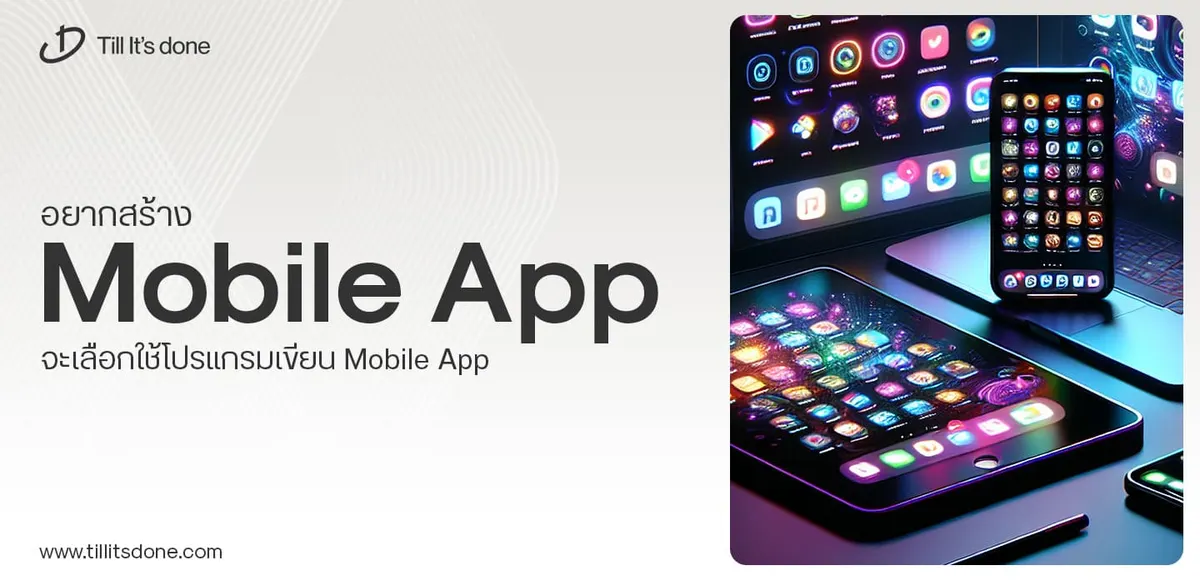
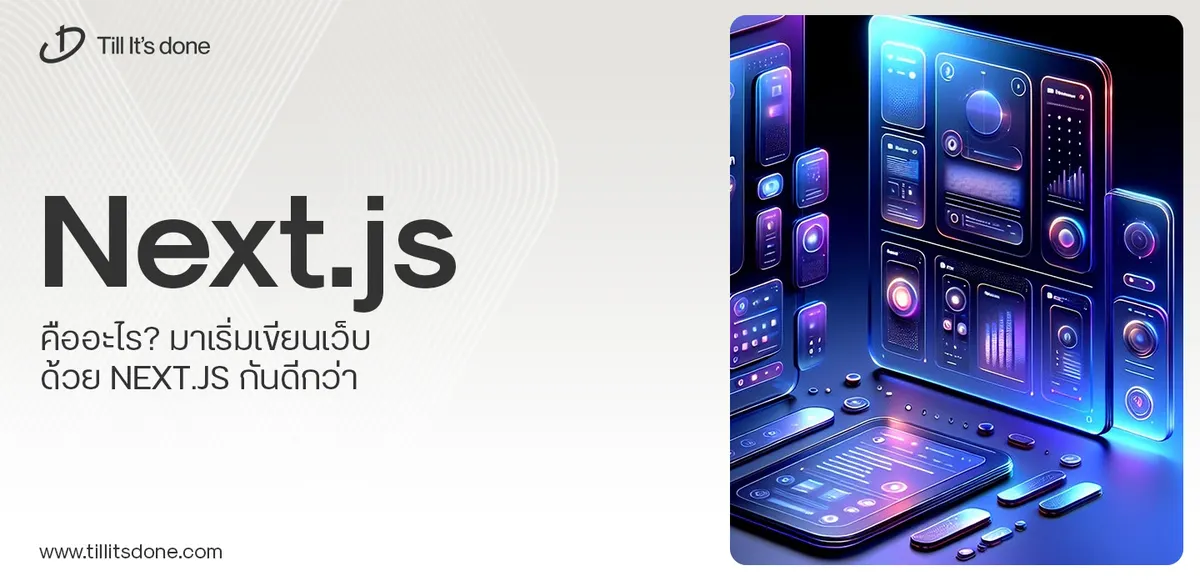
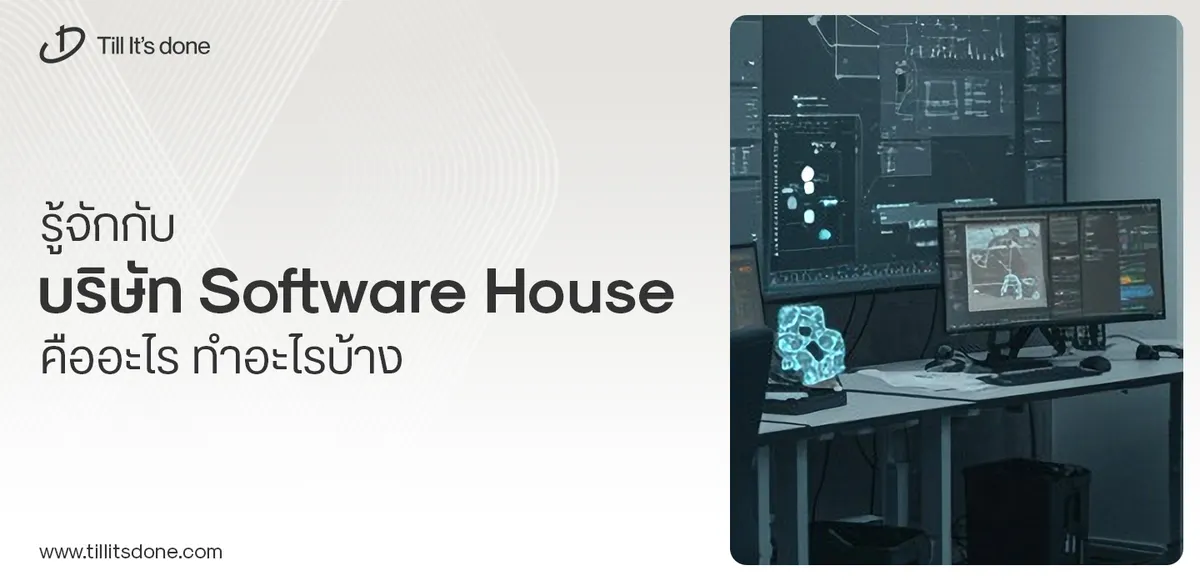
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.