- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Handling Migrations with GORM: Database Guide
Discover best practices, automated migrations, and practical examples for production.
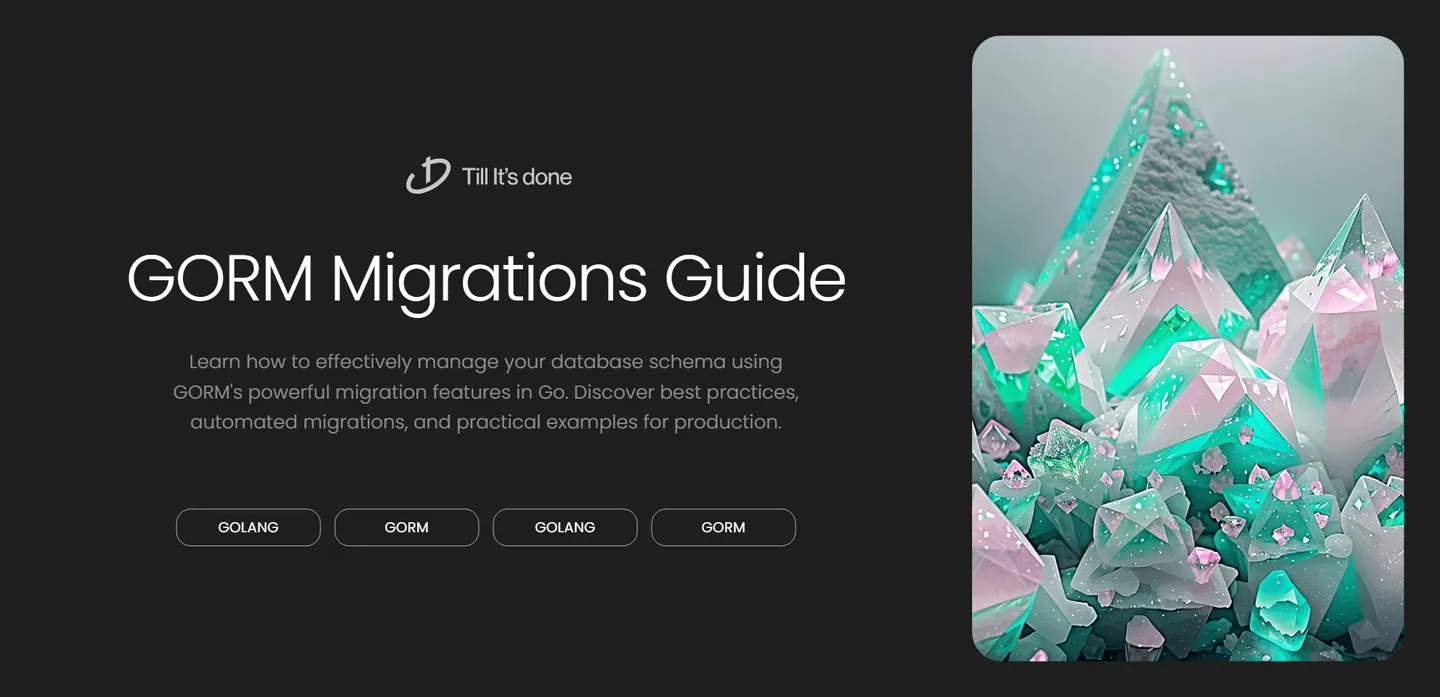
Handling Migrations with GORM: Automating Your Database Schema
Database migrations can be a headache for developers, but they don’t have to be. If you’re working with Go and GORM, you’re in luck – GORM provides powerful migration capabilities that can make your life easier. Let’s dive into how you can effectively manage your database schema using GORM’s migration features.
Understanding GORM Migrations
GORM migrations are like a version control system for your database. They help you keep track of changes to your database schema and ensure that all environments (development, staging, and production) stay in sync.
Getting Started with Auto-Migration
The simplest way to start with GORM migrations is using AutoMigrate. Here’s how you can set it up:
type User struct { ID uint `gorm:"primarykey"` Name string Email string `gorm:"uniqueIndex"` CreatedAt time.Time}
func main() { db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { panic("failed to connect database") }
// Automatically migrate your schema db.AutoMigrate(&User{})}
While AutoMigrate is convenient, remember that it only adds new fields and indexes – it won’t delete unused columns to protect your data.
Best Practices for Production
When working with production databases, it’s crucial to handle migrations carefully. Here are some key practices:
- Always backup your database before running migrations
- Test migrations in a staging environment first
- Use transactions when possible
- Keep track of migration versions
Creating Custom Migration Scripts
For more complex scenarios, you might want to create custom migration scripts:
func Migrate(db *gorm.DB) error { return db.Transaction(func(tx *gorm.DB) error { // Create new tables if err := tx.AutoMigrate(&User{}); err != nil { return err }
// Add custom migrations if err := tx.Exec("ALTER TABLE users ADD COLUMN IF NOT EXISTS status VARCHAR(20)").Error; err != nil { return err }
return nil })}
Handling Data Migrations
Sometimes you need to migrate not just the schema but also the data itself. Here’s a pattern for handling data migrations:
func MigrateUserStatus(db *gorm.DB) error { return db.Transaction(func(tx *gorm.DB) error { // Update all existing users to 'active' status return tx.Model(&User{}).Where("status IS NULL").Update("status", "active").Error })}
Remember to always handle errors appropriately and log important migration events for future debugging.
Conclusion
GORM’s migration capabilities provide a robust foundation for managing your database schema. By following these practices and patterns, you can maintain a clean and organized database structure while ensuring safe and reliable deployments.
Remember that migrations are a critical part of your application’s lifecycle. Take the time to plan them carefully, test thoroughly, and always have a rollback strategy in place.
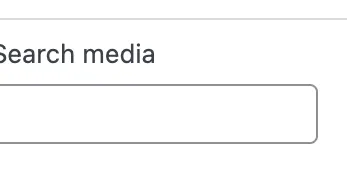





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.