- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Managing Database Relationships in GORM Guide
Includes best practices and performance optimization tips.
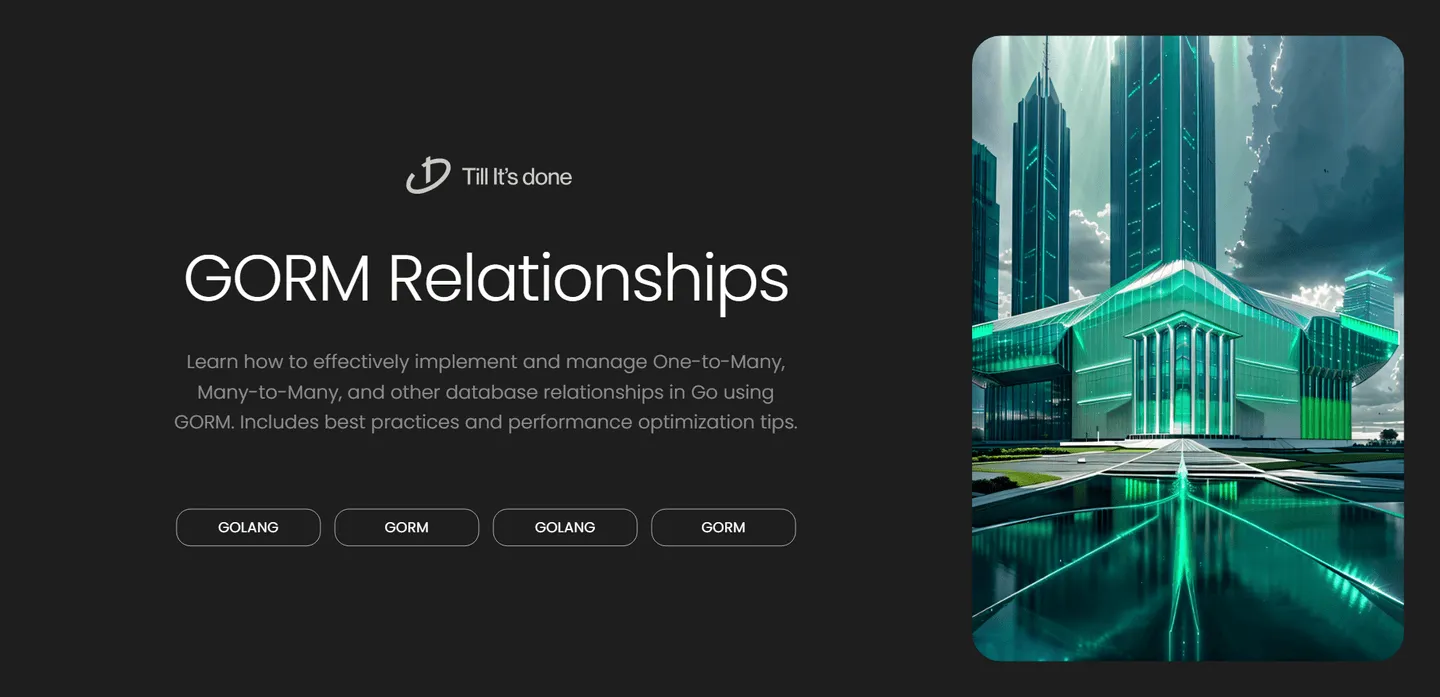
Managing Database Relationships in GORM: One-to-Many, Many-to-Many, and More
When building applications with Go, managing database relationships effectively is crucial for maintaining clean and efficient data structures. GORM, the fantastic ORM library for Golang, makes this task much more manageable with its intuitive API and powerful relationship handling capabilities. Let’s dive into how we can implement various types of relationships in GORM and explore some best practices along the way.
Understanding Basic Relationships
Before we jump into the implementation details, it’s essential to understand the primary types of relationships we’ll be working with:
- One-to-Many: A single record in one table is related to multiple records in another table (like a user having multiple posts)
- Many-to-Many: Records in both tables can have multiple relationships with each other (like students and courses)
- One-to-One: A record in one table is related to exactly one record in another table (like a user and their profile)
Implementing One-to-Many Relationships
Let’s start with a common example: a blog where users can create multiple posts. Here’s how we can structure this relationship in GORM:
type User struct { gorm.Model Name string Posts []Post // One-to-Many relationship}
type Post struct { gorm.Model Title string Content string UserID uint // Foreign key User User // Belongs to relationship}
The magic happens through GORM’s automatic foreign key detection. When you define a slice of related models and a corresponding foreign key in the related model, GORM automatically handles the relationship for you.
Working with Many-to-Many Relationships
Many-to-Many relationships are slightly more complex as they require a join table. Let’s look at a practical example with students and courses:
type Student struct { gorm.Model Name string Courses []Course `gorm:"many2many:student_courses"`}
type Course struct { gorm.Model Name string Students []Student `gorm:"many2many:student_courses"`}
Best Practices and Advanced Features
When working with relationships in GORM, keep these tips in mind:
- Always use proper indexes on foreign key columns
- Consider using eager loading with Preload() for better performance
- Implement soft deletes when necessary to maintain data integrity
- Use transactions for operations that affect multiple related records
Here’s an example of efficient querying with relationships:
var user User// Eager loading of postsdb.Preload("Posts").First(&user)
// Nested preloadingdb.Preload("Posts.Comments").First(&user)
Handling Relationship Callbacks
GORM provides powerful callbacks that you can use to handle related data operations:
func (u *User) AfterDelete(tx *gorm.DB) error { // Clean up related posts when a user is deleted return tx.Delete(&Post{}, "user_id = ?", u.ID).Error}
Performance Considerations
When dealing with relationships, especially in large applications, consider these performance optimization techniques:
- Use batch operations for bulk updates
- Implement pagination for large datasets
- Create composite indexes for frequently queried relationships
- Use database constraints to maintain data integrity
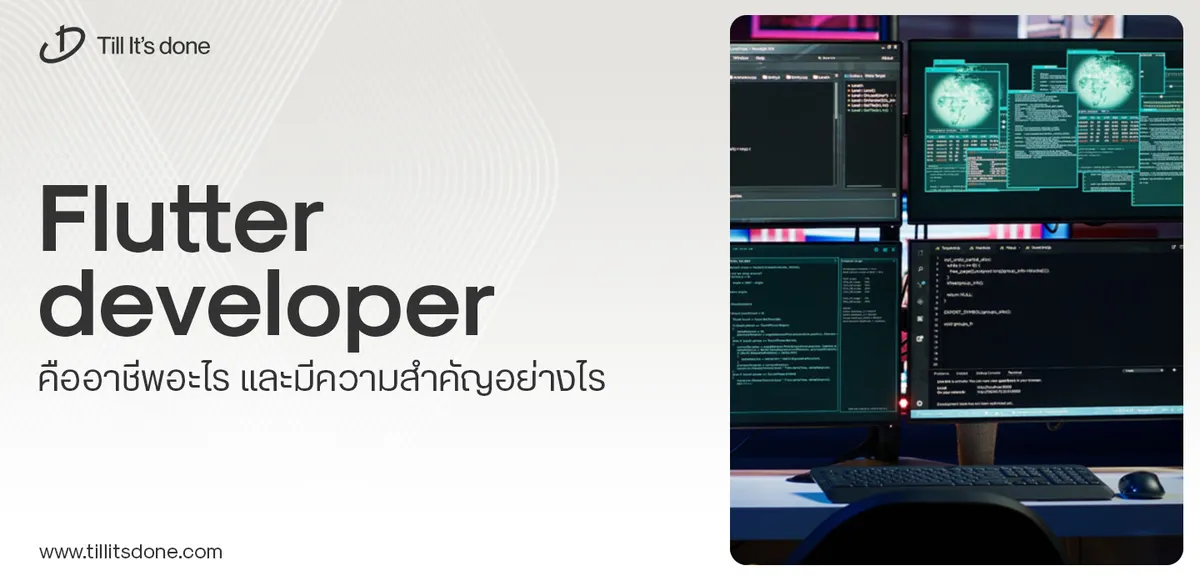
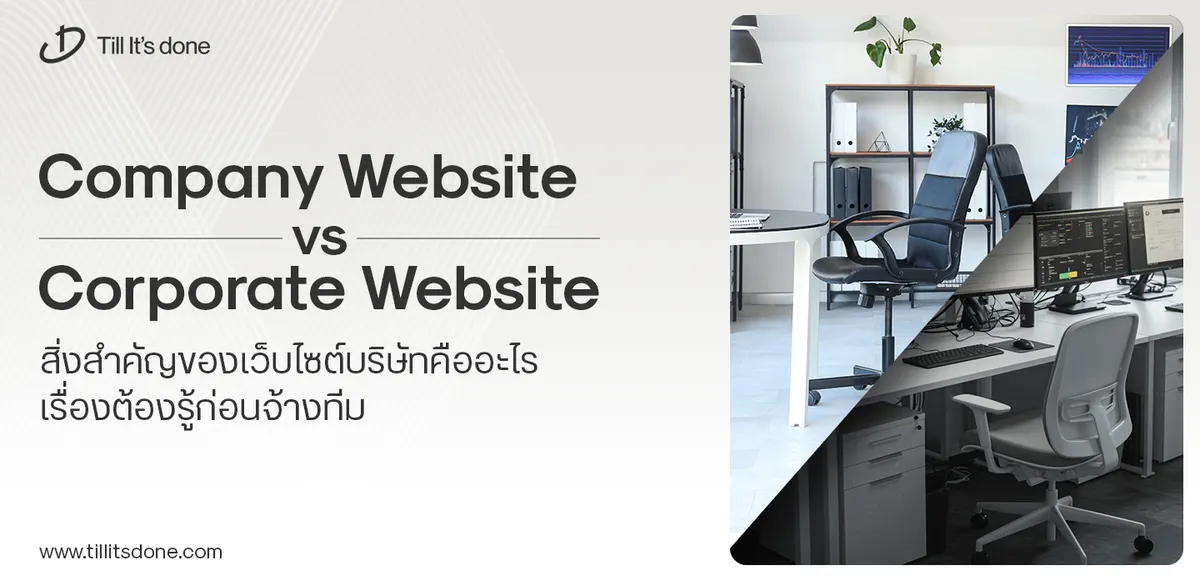
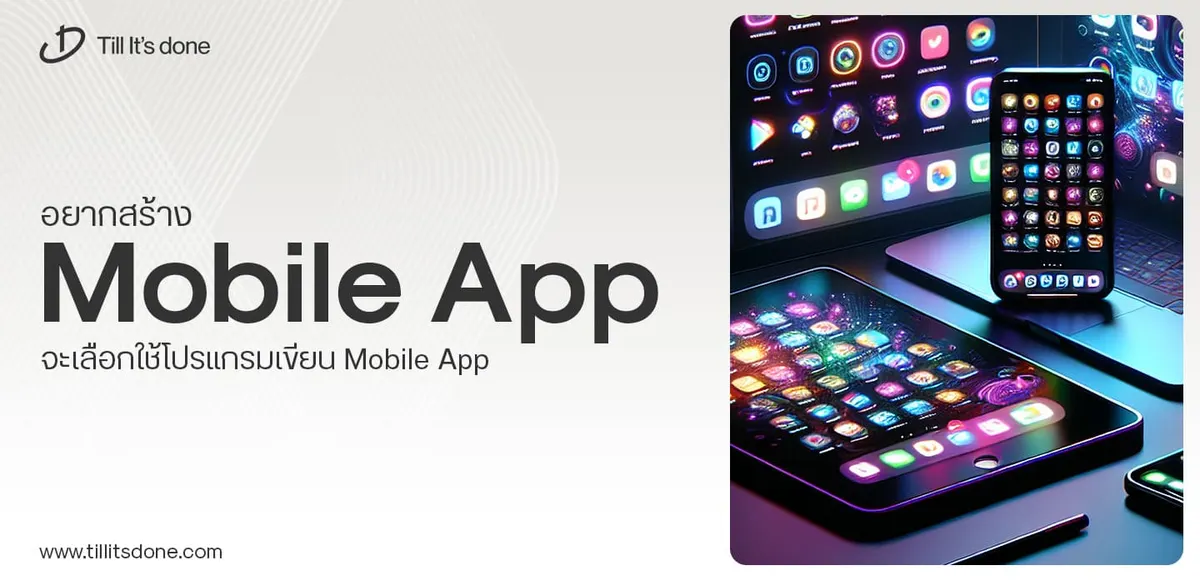
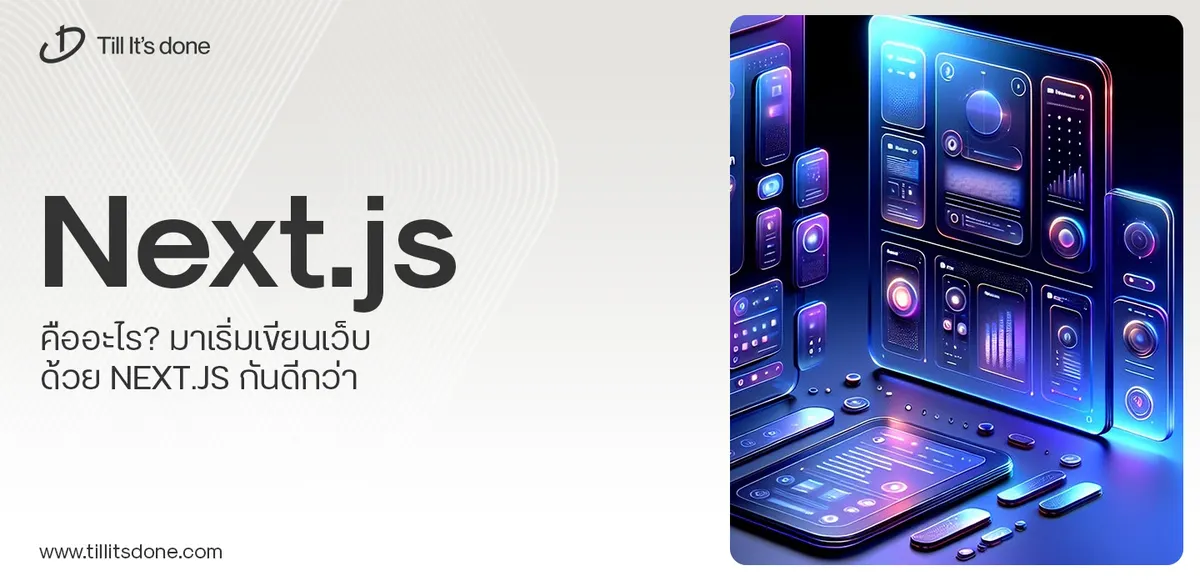
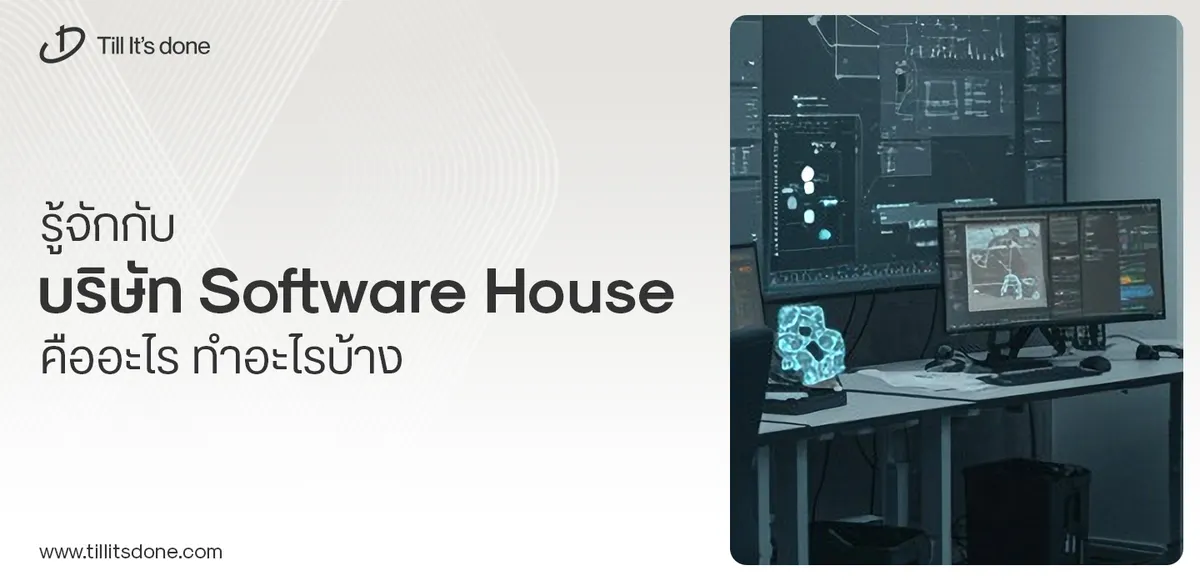
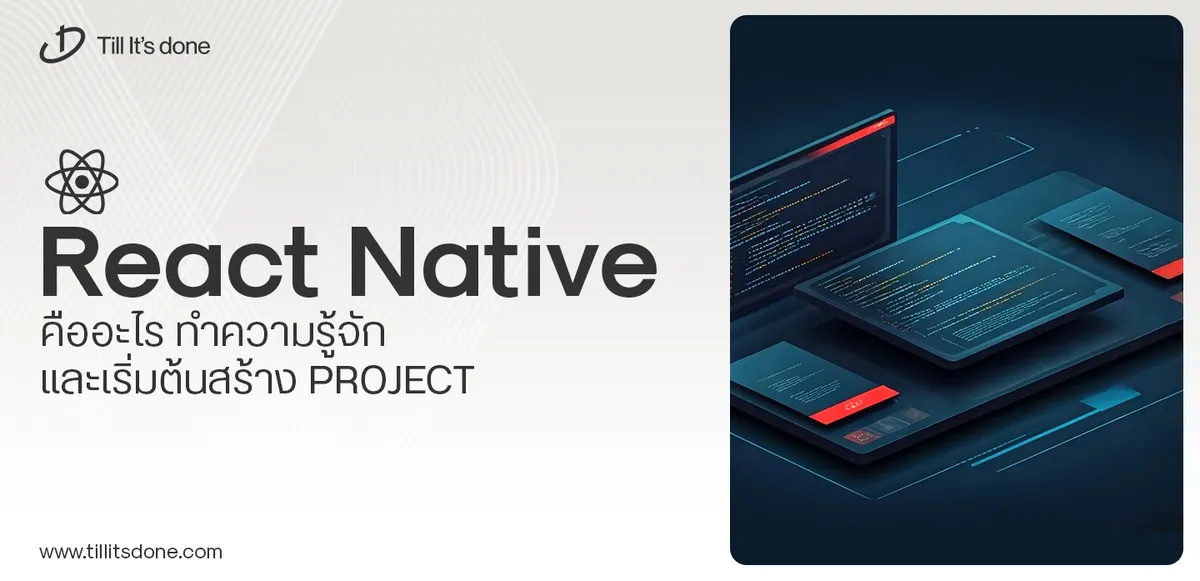
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.