- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Building High-Performance Web Servers in Go
Master concurrent request handling and performance optimization.
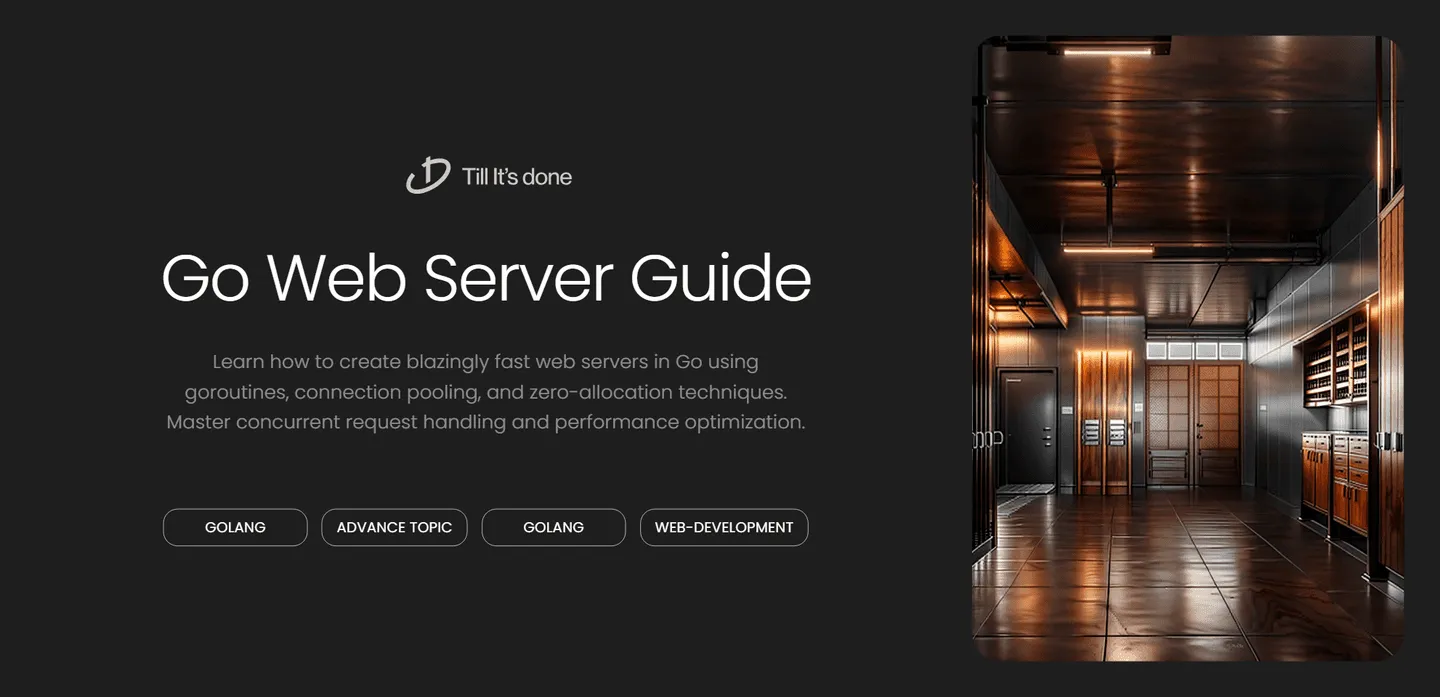
Building High-Performance Web Servers in Go
Go has revolutionized the way we build web servers, offering an impressive combination of performance, simplicity, and built-in concurrency support. In this deep dive, we’ll explore how to create blazingly fast web servers that can handle thousands of concurrent connections while maintaining code clarity and efficiency.
Understanding Go’s Net/HTTP Package
At the heart of Go’s web server capabilities lies the net/http package. Unlike other languages that require external web frameworks, Go provides robust HTTP handling capabilities right out of the box. This native implementation isn’t just convenient - it’s highly optimized and battle-tested by companies like Google.
package main
import ( "net/http" "log")
func main() { http.HandleFunc("/", handler) log.Fatal(http.ListenAndServe(":8080", nil))}
func handler(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Hello, High-Performance World!"))}
Leveraging Goroutines for Concurrent Request Handling
One of Go’s strongest features is its elegant handling of concurrency through goroutines. Each incoming HTTP request is automatically handled in its own goroutine, allowing your server to process multiple requests simultaneously without the overhead of traditional threading.
func complexHandler(w http.ResponseWriter, r *http.Request) { results := make(chan string)
go fetchDataFromDB(results) go processAnalytics(r)
response := <-results w.Write([]byte(response))}
Performance Optimization Techniques
1. Connection Pooling
Implementing connection pooling for database operations is crucial for maintaining high performance:
var db *sql.DB
func initDB() { db.SetMaxOpenConns(100) db.SetMaxIdleConns(25) db.SetConnMaxLifetime(5 * time.Minute)}
2. Response Caching
Implement an efficient caching layer to reduce database load:
type Cache struct { sync.RWMutex items map[string][]byte}
func (c *Cache) Get(key string) ([]byte, bool) { c.RLock() defer c.RUnlock() item, exists := c.items[key] return item, exists}
3. Zero-Allocation Techniques
Minimize garbage collection overhead by reducing allocations:
var bufPool = sync.Pool{ New: func() interface{} { return make([]byte, 0, 64*1024) },}
Load Testing and Monitoring
Always measure your server’s performance under real-world conditions. Use tools like Apache Benchmark or hey for load testing:
hey -n 10000 -c 100 http://localhost:8080/
Monitor key metrics like response time, memory usage, and goroutine count:
func metricsHandler(w http.ResponseWriter, r *http.Request) { var m runtime.MemStats runtime.ReadMemStats(&m) // Log or expose metrics}
Conclusion
Building high-performance web servers in Go is a delightful experience, thanks to the language’s excellent standard library and built-in concurrency support. By following these optimization techniques and best practices, you can create web servers that not only handle massive loads but are also maintainable and efficient.





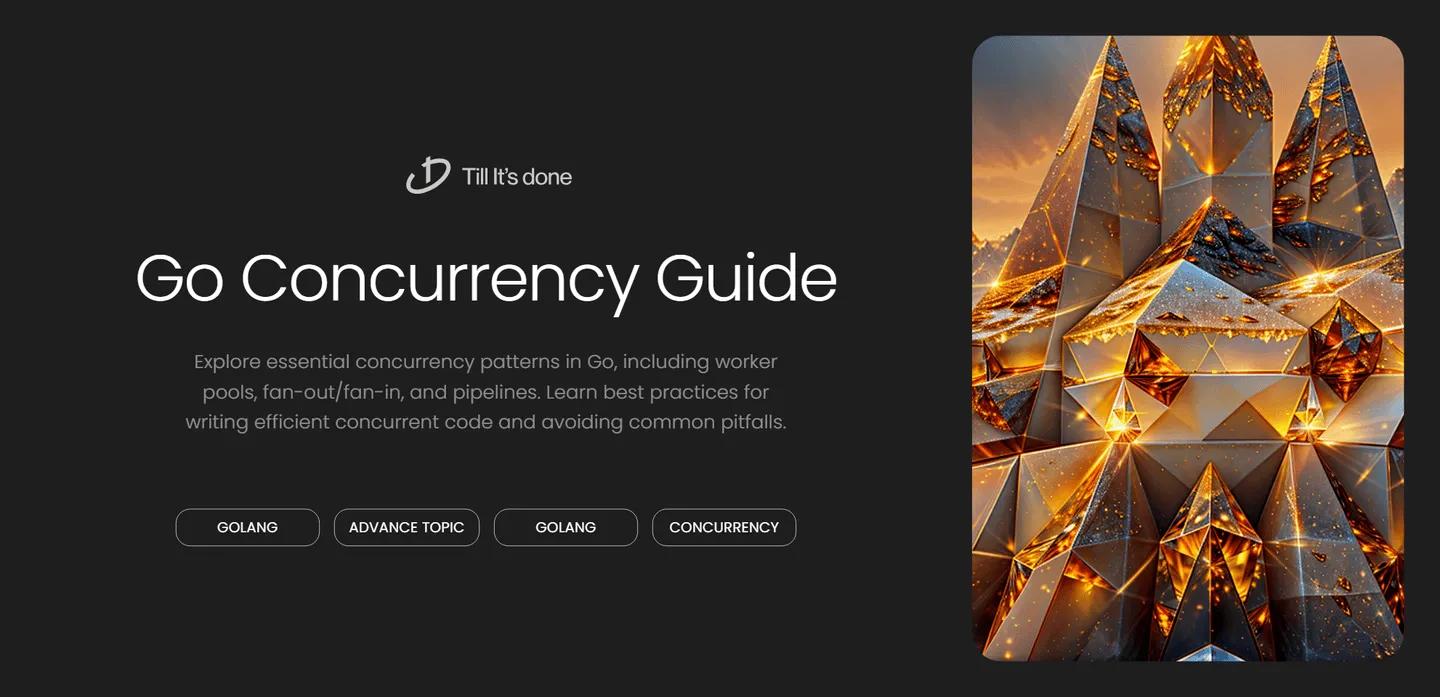
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.