- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Concurrency Patterns in Go: Best Practices
Learn best practices for writing efficient concurrent code and avoiding common pitfalls.
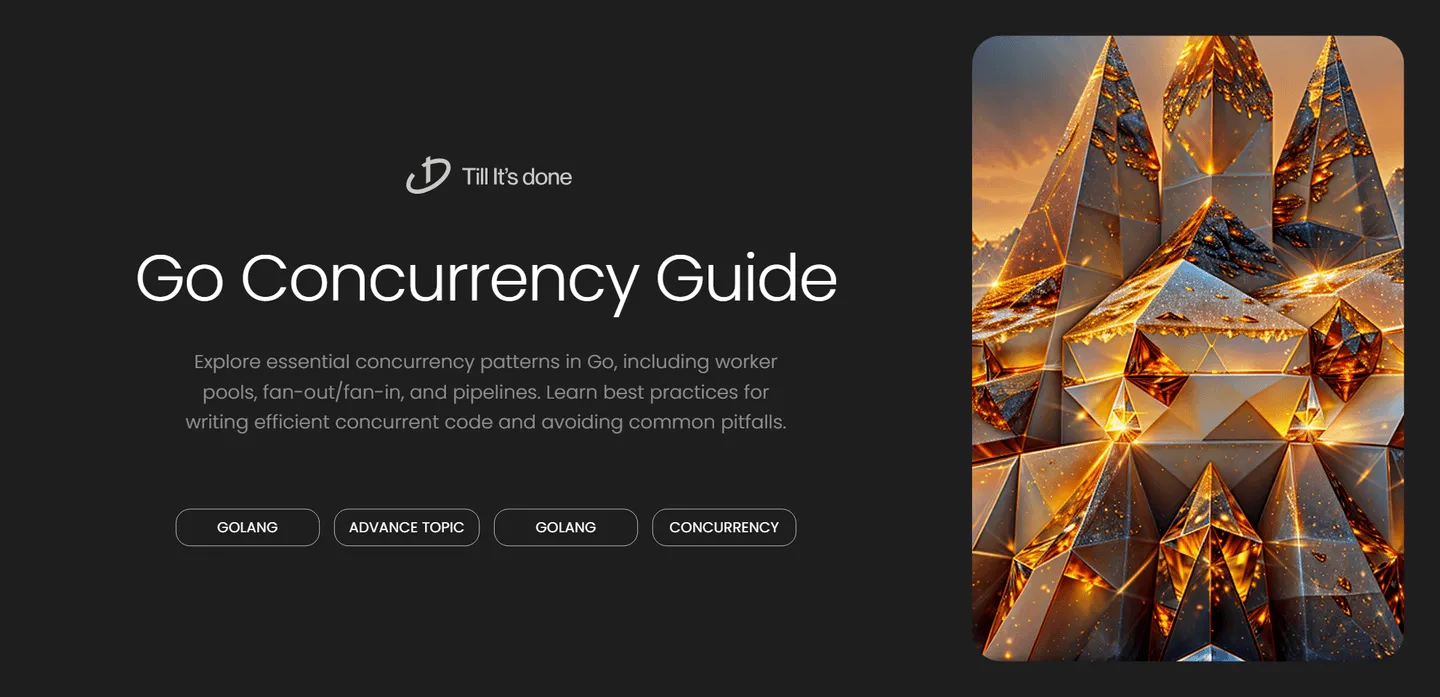
Concurrency Patterns in Go: Best Practices
Concurrency is one of Go’s most powerful features, setting it apart from many other programming languages. Today, let’s dive deep into some battle-tested concurrency patterns that will help you write more efficient and maintainable concurrent code.
Understanding Go’s Concurrency Model
At its core, Go’s concurrency model is built around goroutines and channels. While these primitives are simple to understand, combining them effectively requires careful consideration and established patterns.
The Worker Pool Pattern
One of the most commonly used concurrency patterns is the worker pool. This pattern is perfect when you have multiple tasks that can be processed independently and want to limit the number of concurrent operations.
func workerPool(numWorkers int, tasks []Task) { jobs := make(chan Task, len(tasks)) results := make(chan Result, len(tasks))
// Start workers for i := 0; i < numWorkers; i++ { go worker(jobs, results) }
// Send jobs for _, task := range tasks { jobs <- task } close(jobs)
// Collect results for range tasks { result := <-results // Process result }}
The Fan-Out, Fan-In Pattern
When you need to split work across multiple goroutines and then combine their results, the fan-out, fan-in pattern becomes invaluable. This pattern is particularly useful for CPU-intensive tasks that can benefit from parallel processing.
func fanOut(input []int) []int { channels := make([]<-chan int, len(processors)) for i := range processors { channels[i] = processor(input) } return fanIn(channels...)}
The Pipeline Pattern
Pipelines are perfect for creating a series of data transformations where each stage processes data independently. This pattern helps break down complex operations into manageable, reusable components.
func pipeline() { input := generateInput() step1 := processStep1(input) step2 := processStep2(step1) output := processStep3(step2)
for result := range output { // Handle final results }}
Best Practices and Common Pitfalls
- Always use select statements with timeouts when dealing with channel operations
- Remember to properly close channels to prevent goroutine leaks
- Use context for cancellation and timeout management
- Consider using sync.WaitGroup for coordinating multiple goroutines
- Monitor goroutine count in production applications
Conclusion
Mastering these concurrency patterns will significantly improve your Go applications’ performance and reliability. Remember that the key to successful concurrent programming is not just knowing these patterns, but understanding when to apply them appropriately.
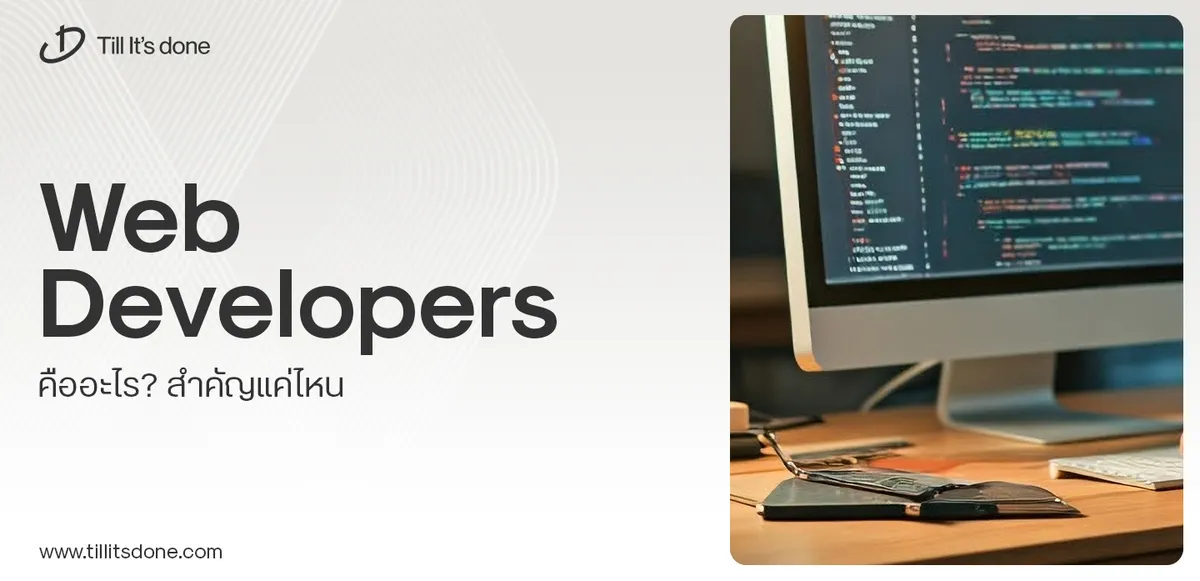
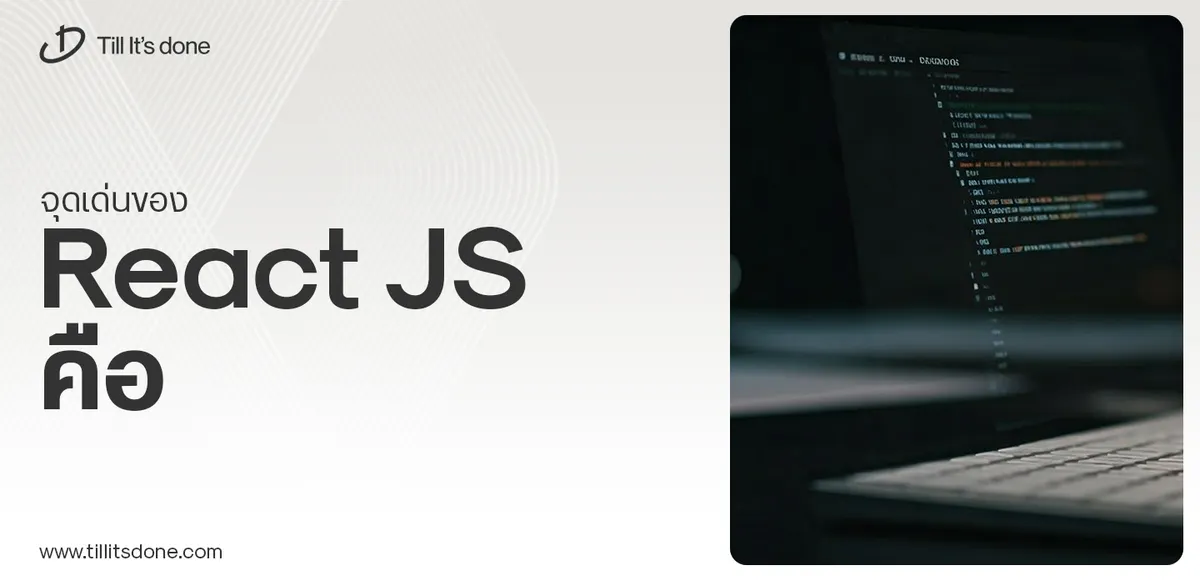
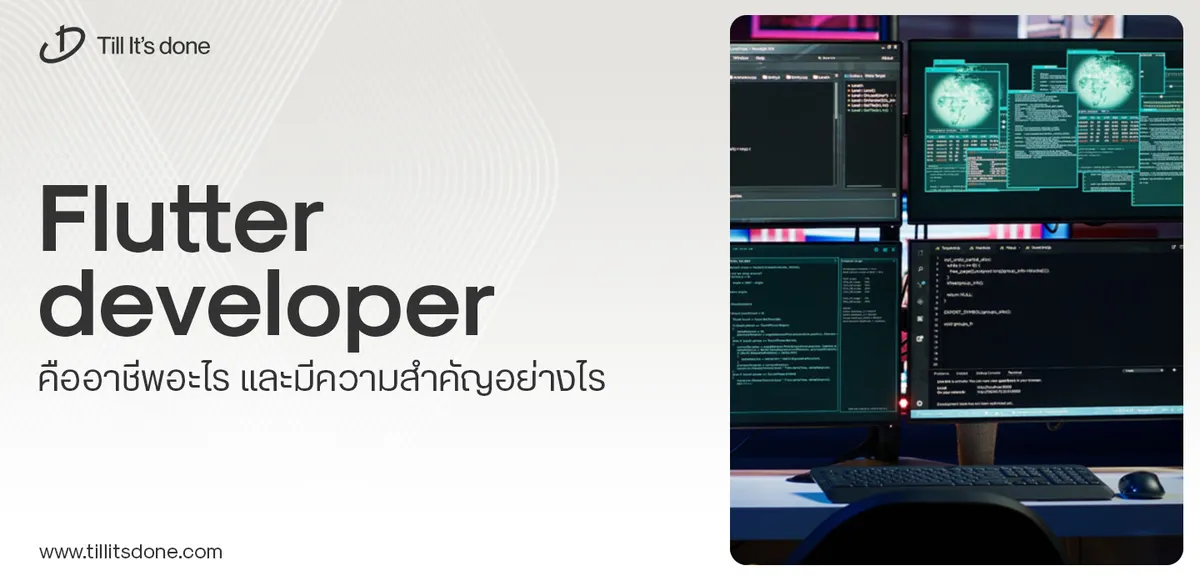
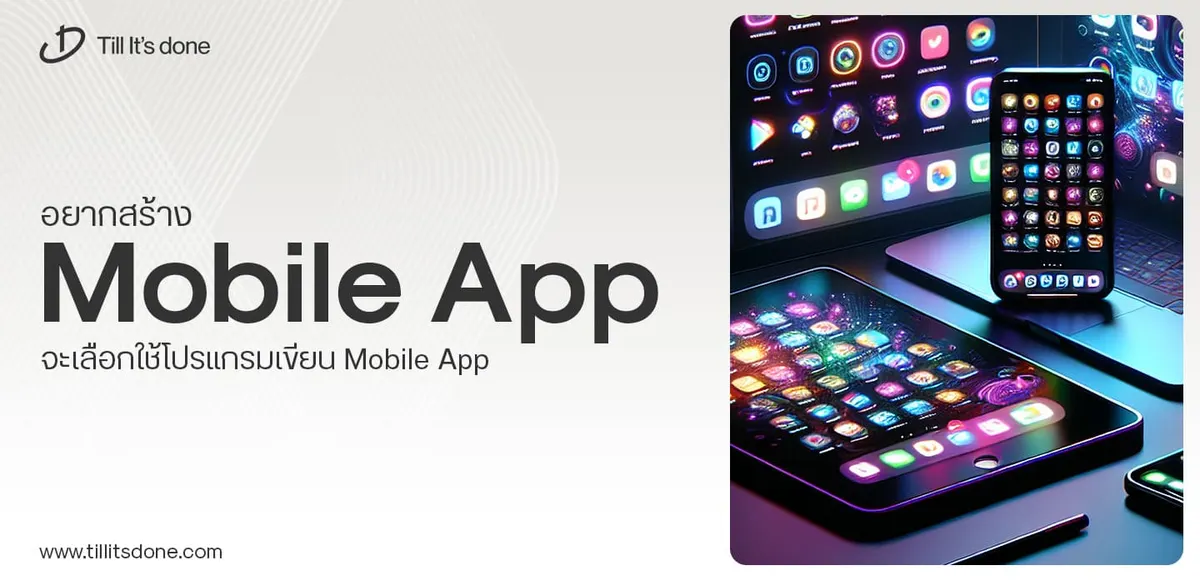
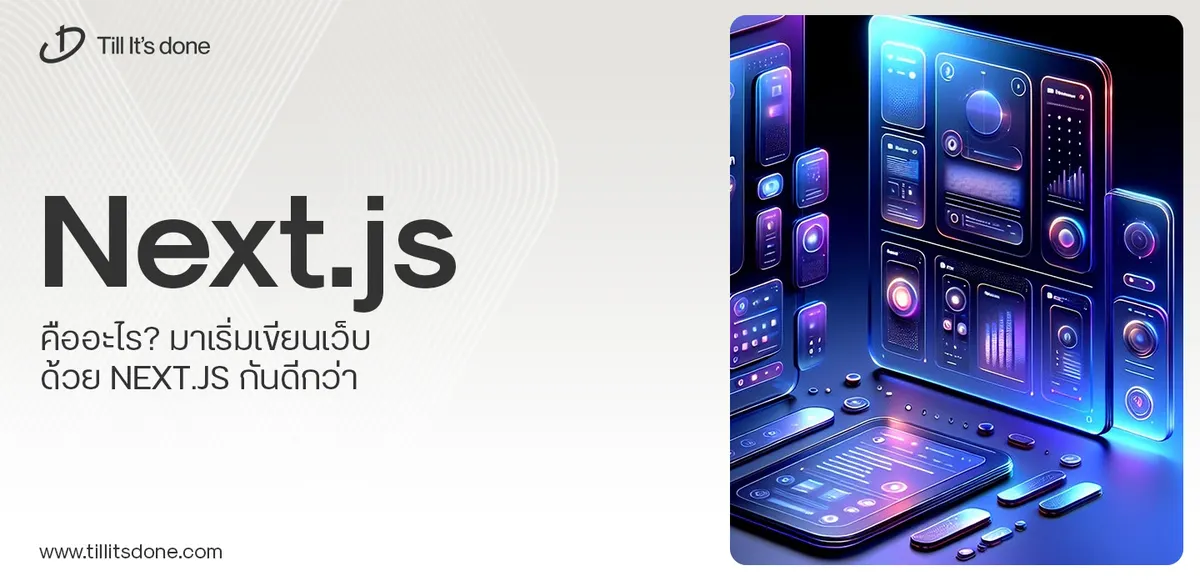
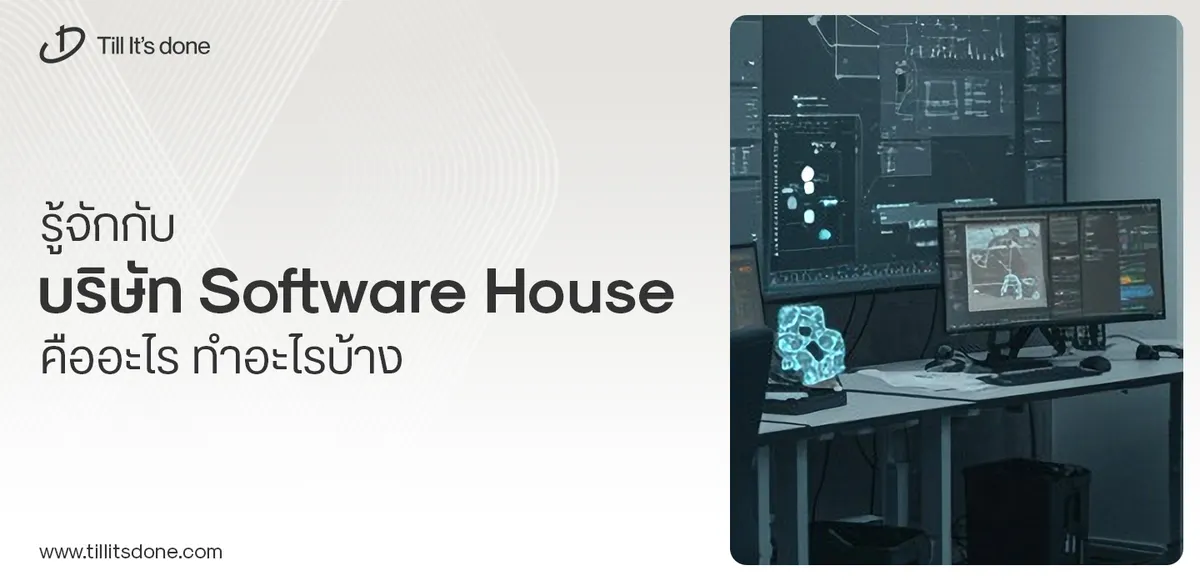
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.