- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Profiling and Optimizing Go Applications
Discover practical techniques for memory management, concurrency optimization, and performance tuning.
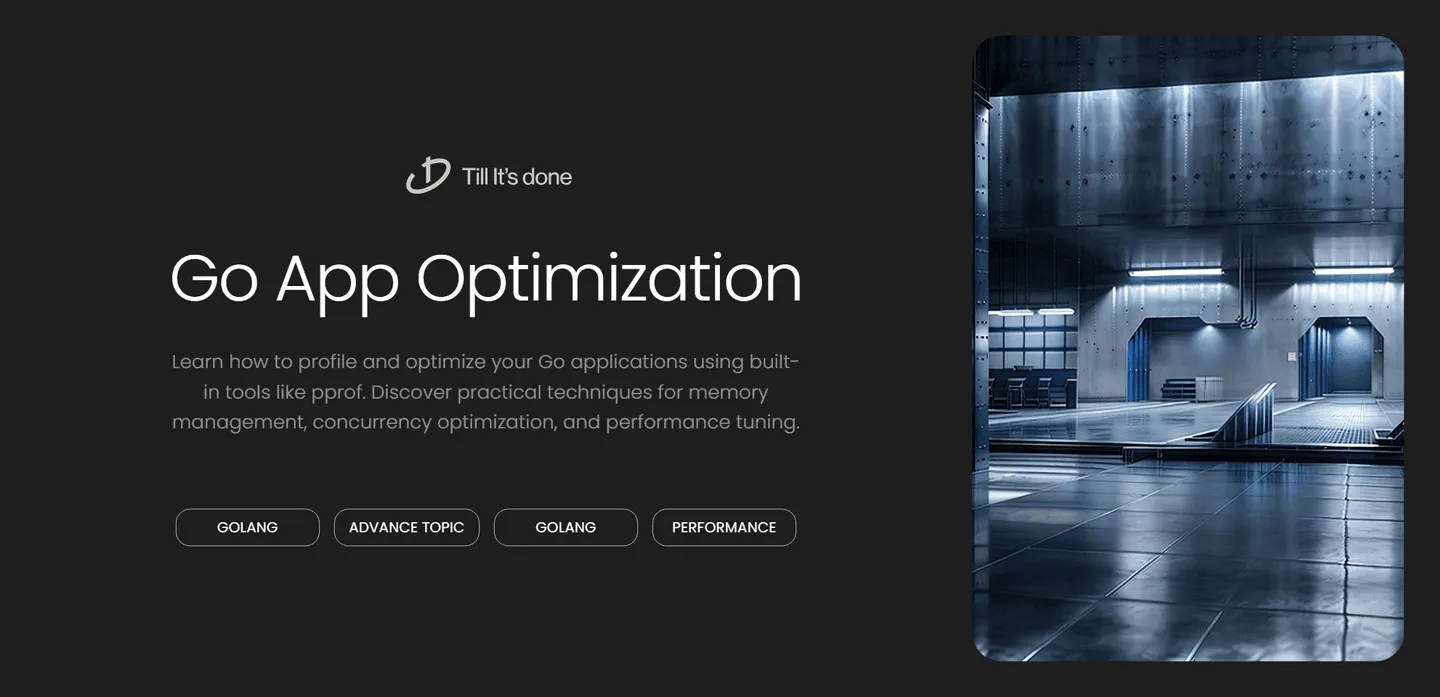
Mastering Performance: A Deep Dive into Profiling and Optimizing Go Applications
When building applications in Go, performance isn’t just a nice-to-have feature – it’s often a critical requirement. Today, we’ll explore how to profile and optimize Go applications to squeeze out every bit of performance while maintaining code clarity and reliability.
Understanding Go Profiling
Before diving into optimization techniques, we need the right tools to identify bottlenecks. Go provides several built-in profiling tools that help us understand where our application spends its time and resources.
Types of Profiling in Go
- CPU Profiling: Shows which functions consume the most CPU time
- Memory Profiling: Helps identify memory allocations and potential leaks
- Goroutine Profiling: Analyzes concurrent operations and deadlocks
- Block Profiling: Identifies where goroutines block waiting for shared resources
Essential Profiling Tools
pprof: Your Performance Swiss Army Knife
The pprof
tool is Go’s primary profiling utility. Here’s how to get started:
import "runtime/pprof"
func main() { // CPU profiling f, _ := os.Create("cpu.prof") pprof.StartCPUProfile(f) defer pprof.StopCPUProfile()
// Your application code here}
Benchmarking: Measure First, Optimize Later
Always establish baseline performance through benchmarks:
func BenchmarkMyFunction(b *testing.B) { for i := 0; i < b.N; i++ { MyFunction() }}
Optimization Techniques
1. Memory Management
- Use sync.Pool for frequently allocated objects
- Consider using arrays instead of slices for fixed-size collections
- Preallocate slices when the size is known
- Be mindful of string concatenation
2. Concurrency Optimization
- Use buffered channels appropriately
- Consider worker pools for concurrent tasks
- Avoid goroutine leaks
- Use sync.Once for one-time initializations
3. Algorithm Optimization
- Choose appropriate data structures
- Minimize allocations in hot paths
- Use efficient sorting algorithms
- Implement caching where appropriate
Real-world Optimization Example
Let’s look at a practical example of optimizing a data processing function:
// Before optimizationfunc processData(data []int) int { result := 0 for _, v := range data { result += v } return result}
// After optimizationfunc processDataOptimized(data []int) int { if len(data) < 2 { if len(data) == 0 { return 0 } return data[0] }
result := 0 for i := 0; i < len(data); i += 2 { if i == len(data)-1 { result += data[i] break } result += data[i] + data[i+1] } return result}
Best Practices and Common Pitfalls
- Profile before optimizing
- Focus on hot paths
- Benchmark after each optimization
- Don’t sacrifice code readability for minimal gains
- Consider the trade-offs between memory and CPU usage
Remember, premature optimization is the root of all evil. Always profile first, identify real bottlenecks, and optimize with clear metrics in mind. The goal is to make your Go applications not just faster, but more efficient and maintainable in the long run.
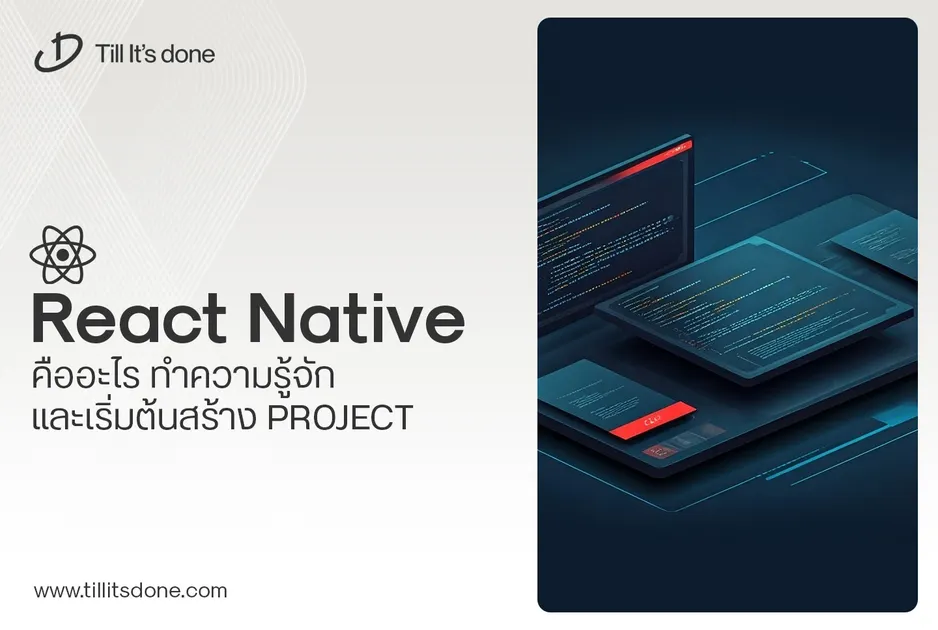
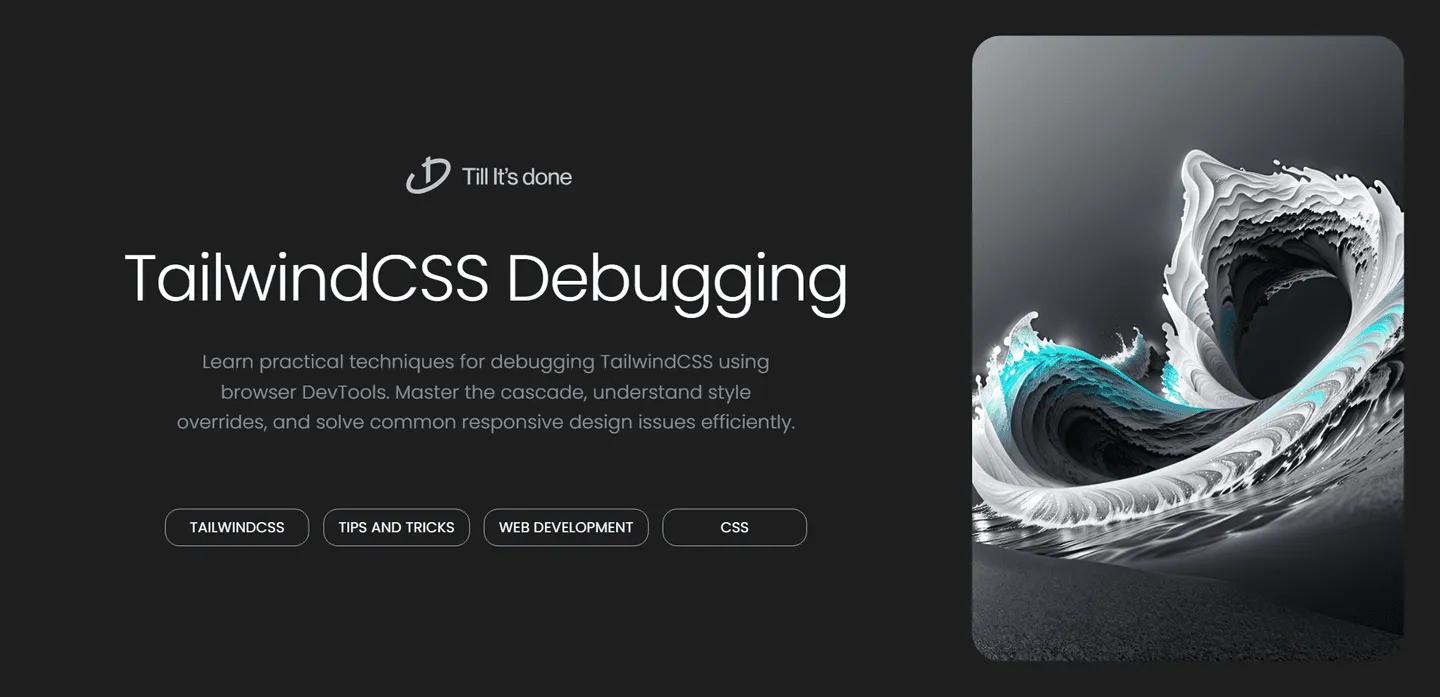
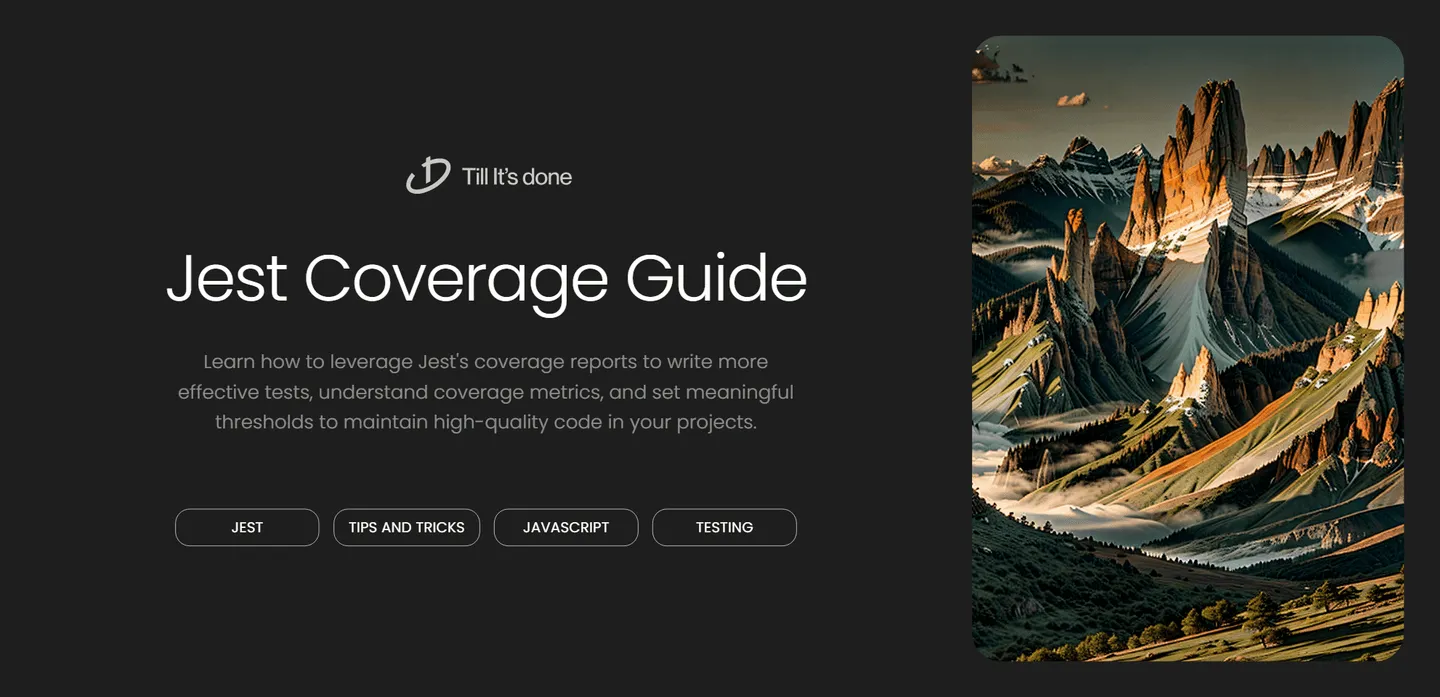
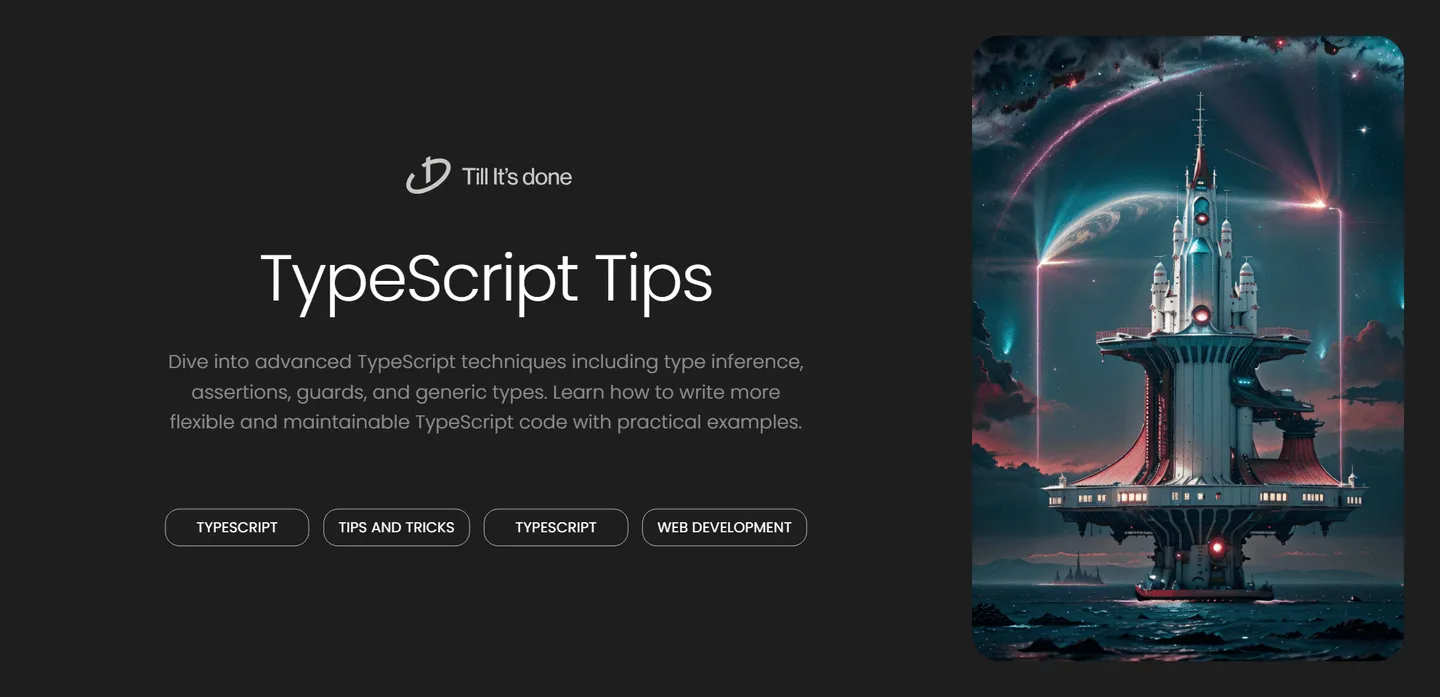
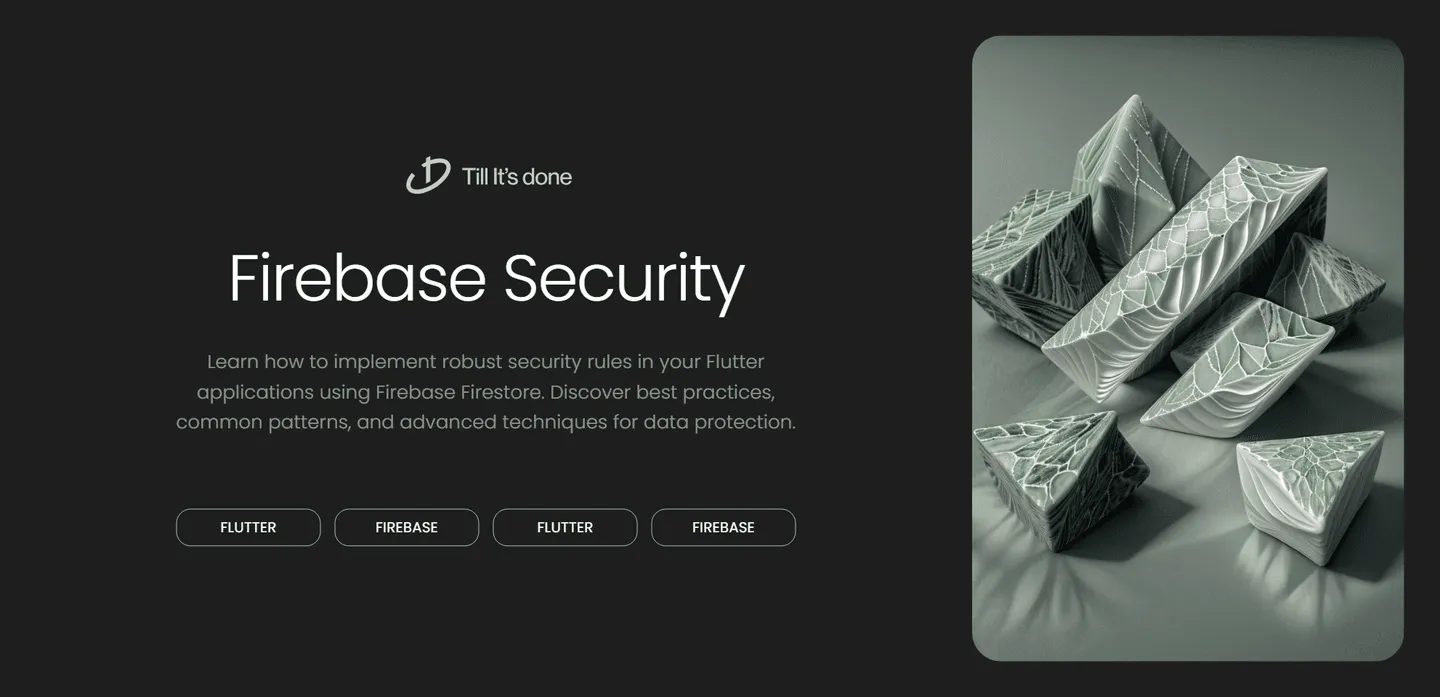
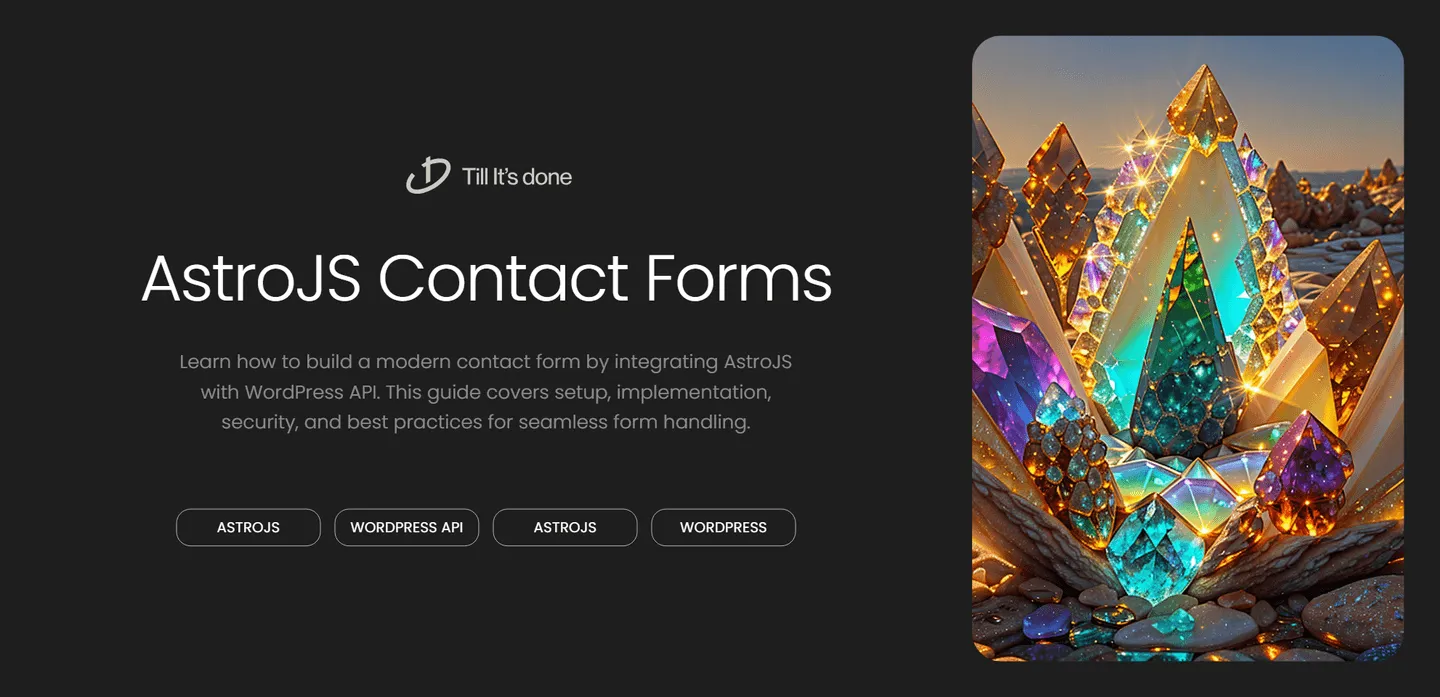
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.