- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Understanding Goroutines and Channels in Go
Learn how these lightweight threads and communication channels enable efficient concurrent programming.
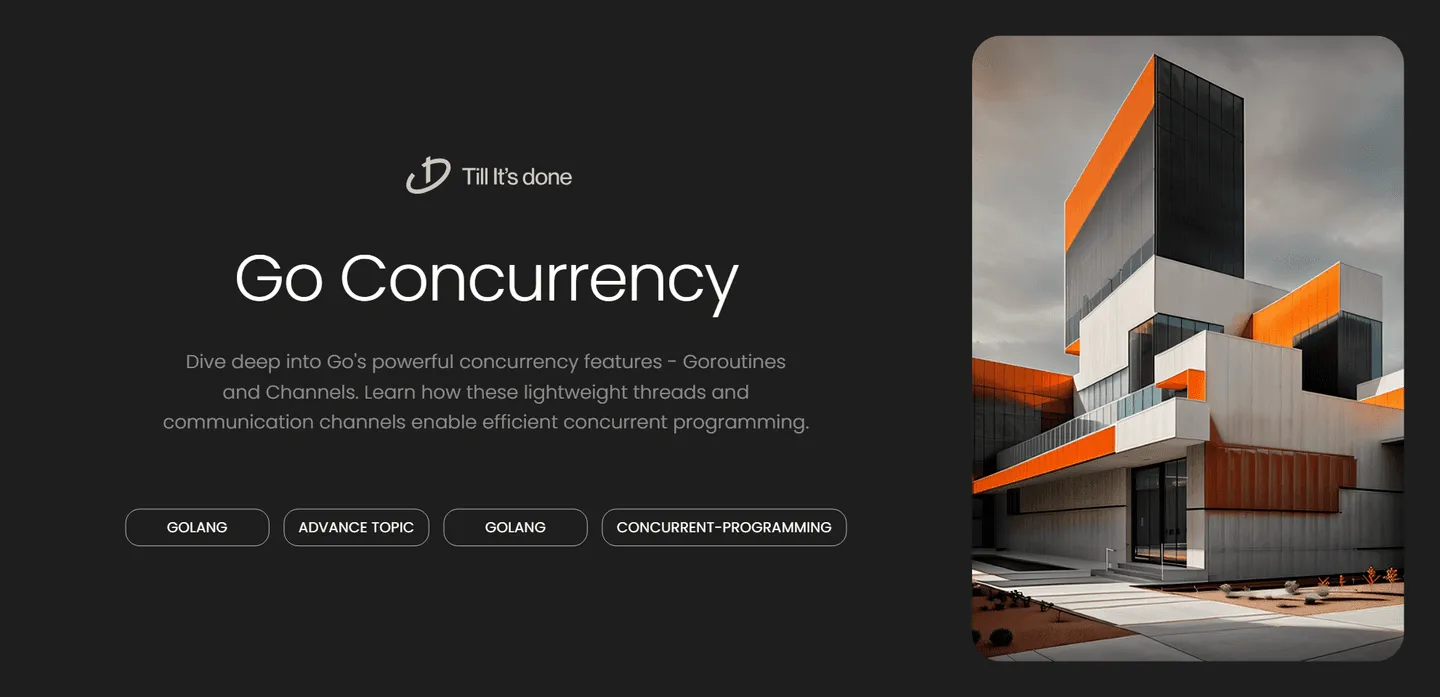
Understanding Goroutines and Channels in Depth
Go’s concurrency model is one of its most powerful features, setting it apart from many other programming languages. Today, let’s dive deep into two fundamental concepts that make Go’s concurrency so elegant: Goroutines and Channels.
What Are Goroutines?
Think of goroutines as lightweight threads on steroids. Unlike traditional threads that might consume megabytes of memory, a goroutine starts with just a few kilobytes. They’re so lightweight that you can spawn thousands of them without breaking a sweat.
The Magic Behind Goroutines
What makes goroutines special isn’t just their size - it’s how Go manages them. The Go runtime employs a clever scheduler that multiplexes goroutines onto OS threads. When one goroutine blocks (like waiting for I/O), the scheduler smoothly switches to another, ensuring optimal resource utilization.
Understanding Channels
If goroutines are the actors in Go’s concurrency play, channels are the stage where they perform. Channels provide a type-safe way for goroutines to communicate and synchronize their execution.
Types of Channels
-
Unbuffered Channels: These channels force synchronization. When a goroutine sends a value, it blocks until another goroutine receives it. It’s like a synchronized handshake between two goroutines.
-
Buffered Channels: Think of these as mailboxes with limited capacity. Sending goroutines can drop off multiple values without waiting for receivers, up to the buffer’s capacity.
Best Practices and Common Pitfalls
Do’s:
- Always use
defer close(ch)
for channels you own - Consider buffered channels for performance-critical sections
- Use select statements for handling multiple channels
- Remember: channels are first-class values
Don’ts:
- Don’t forget to close channels (leads to memory leaks)
- Avoid closing a channel from the receiver’s side
- Never close a channel more than once
- Don’t assume the order of operations in different goroutines
Real-World Applications
Goroutines and channels shine in scenarios like:
- Building web scrapers that can handle multiple URLs simultaneously
- Creating responsive web servers that can handle thousands of concurrent connections
- Processing large datasets in parallel
- Implementing pub/sub systems
- Building real-time data processing pipelines
Conclusion
Goroutines and channels aren’t just language features - they’re a different way of thinking about concurrent programming. They make it possible to write concurrent code that’s both powerful and maintainable. As you build more complex applications in Go, you’ll find these tools becoming an indispensable part of your development arsenal.
Remember: the power of Go’s concurrency isn’t just in its performance, but in how it helps us write code that’s both concurrent and comprehensible. That’s a rare combination in the programming world, and it’s what makes Go special.
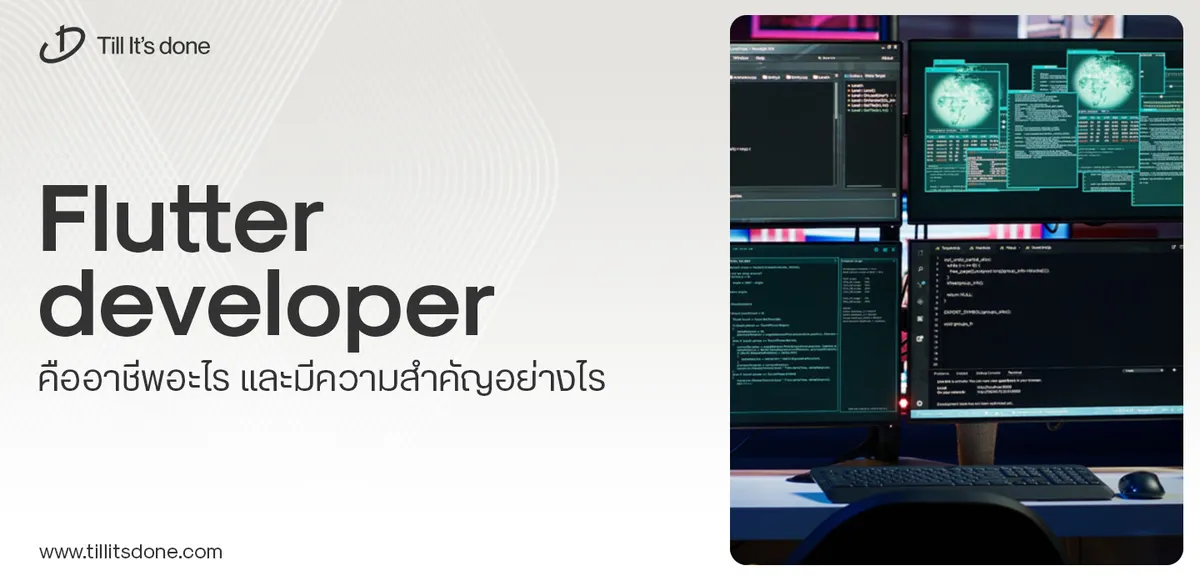
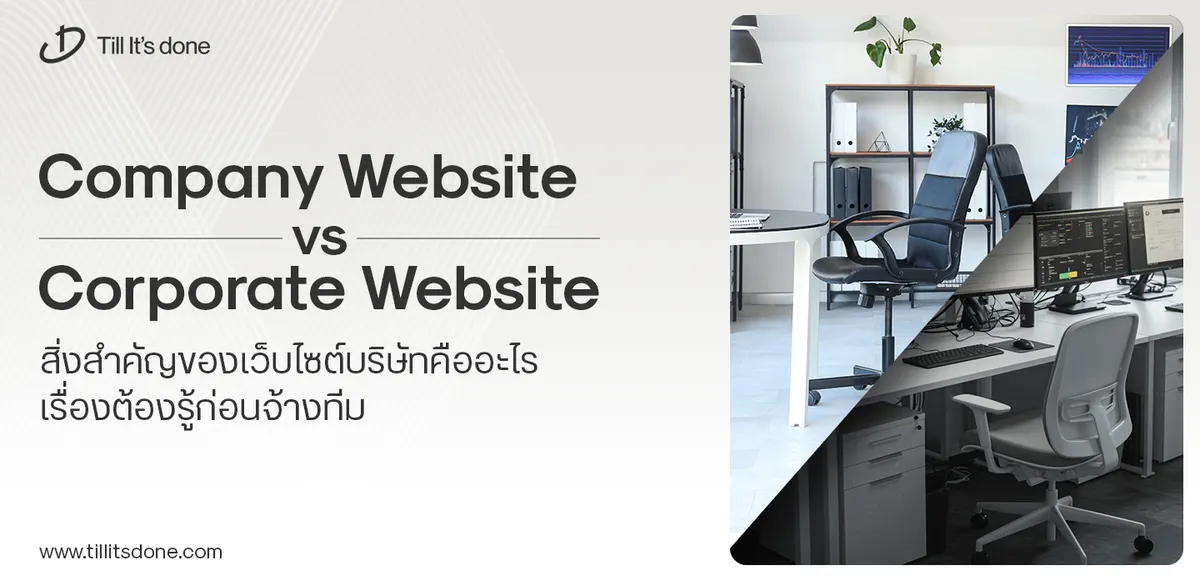
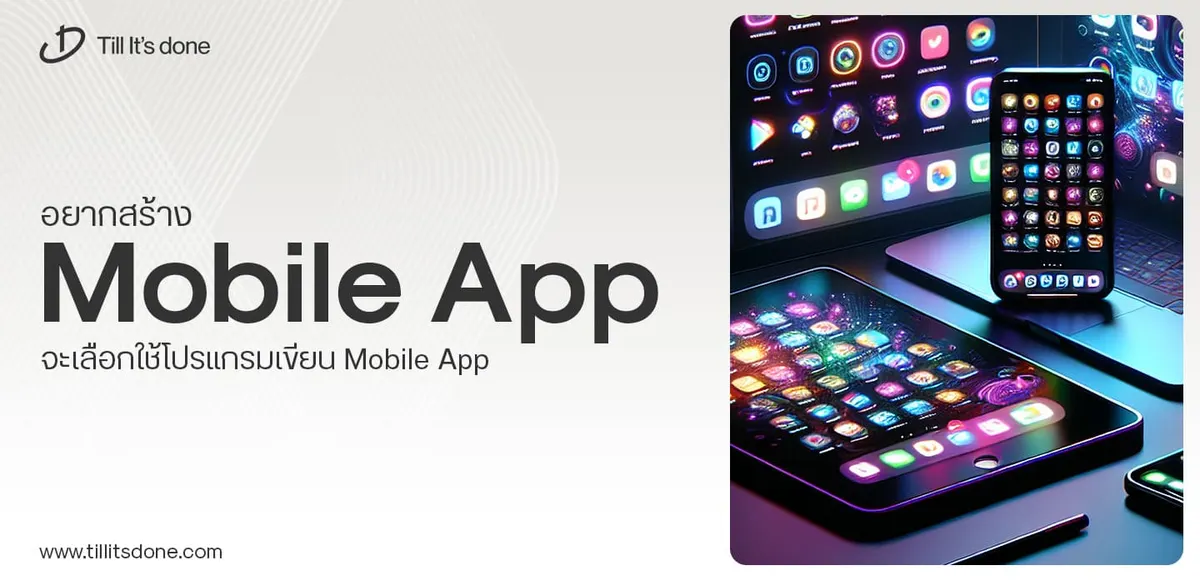
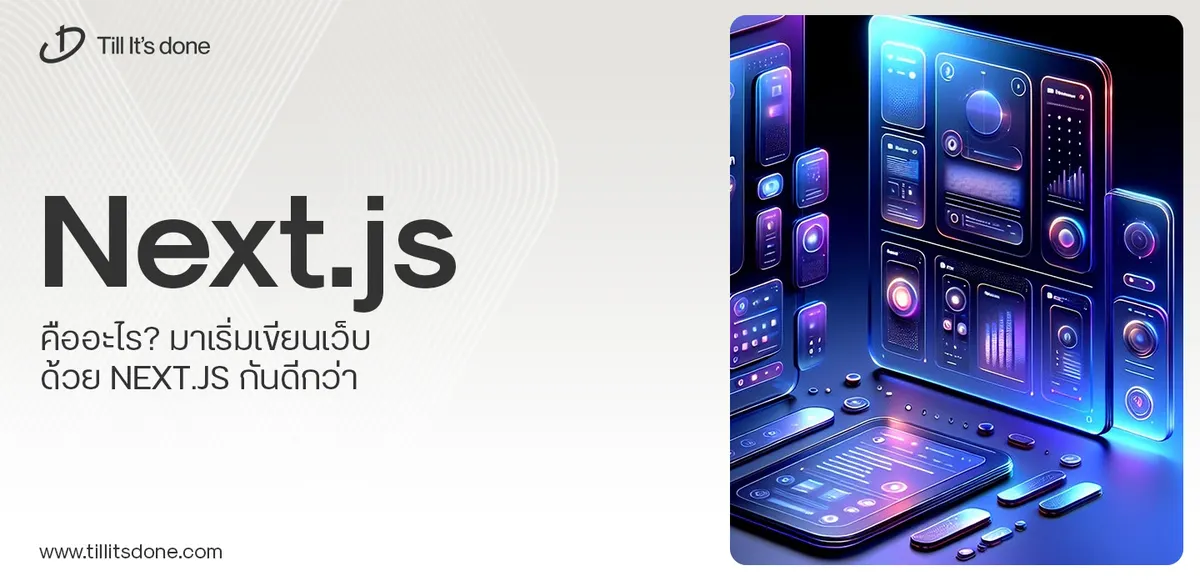
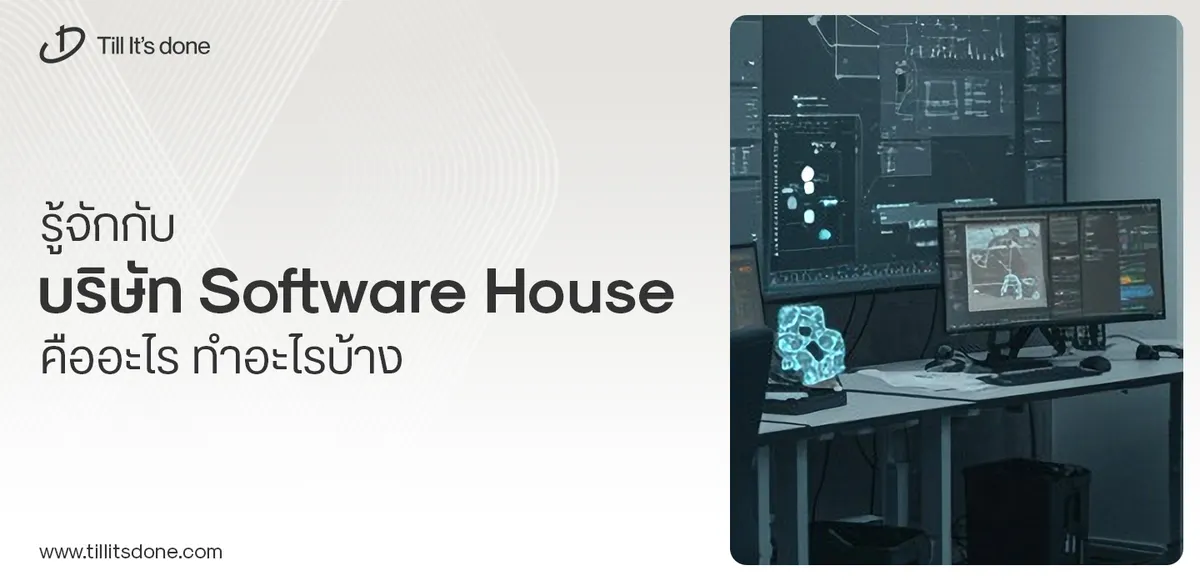
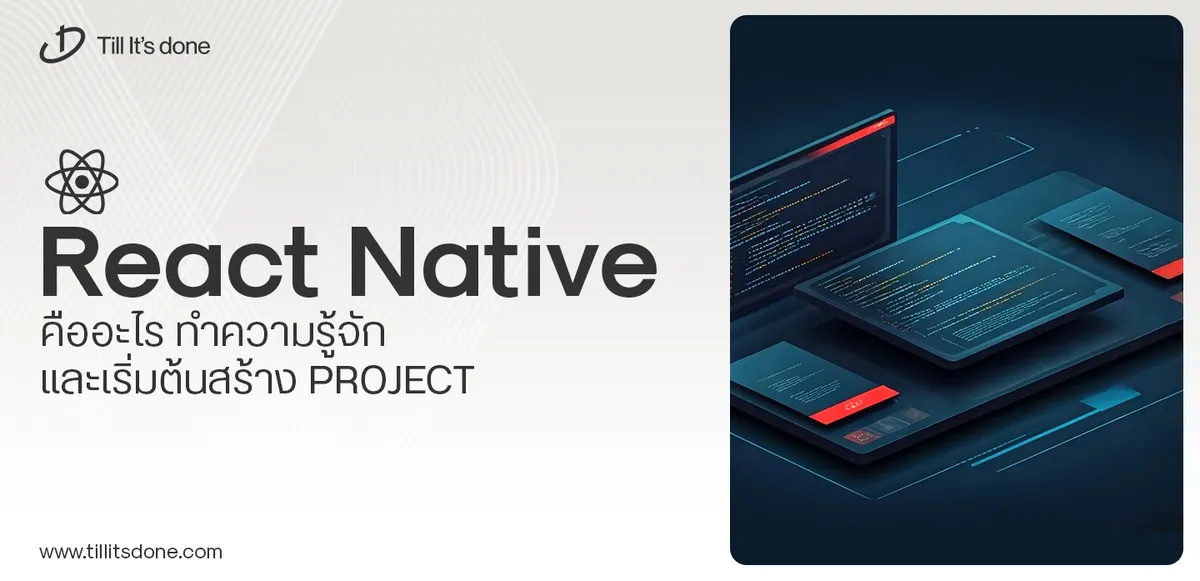
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.