- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Go Memory Management: GC & Optimizations
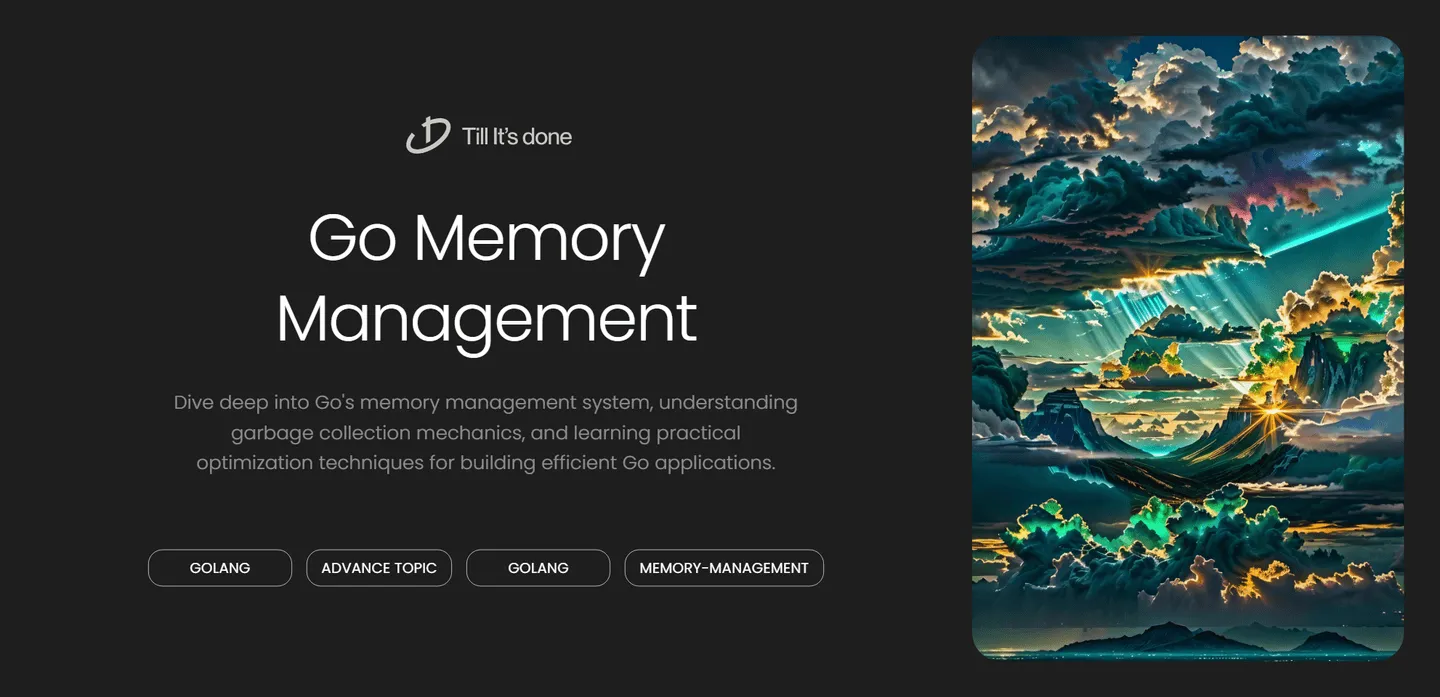
Understanding Go Memory Management: Garbage Collection and Optimizations
Memory management is one of the most crucial aspects of any programming language, and Go takes a unique approach that balances performance with developer convenience. In this deep dive, we’ll explore how Go handles memory management and discover ways to optimize your applications.
The Fundamentals of Go’s Memory Management
Go utilizes a tricolor mark-and-sweep garbage collector, which runs concurrently with your application code. Unlike traditional garbage collectors that require stopping the entire program (known as “stop-the-world” pauses), Go’s garbage collector is designed to minimize these pauses while maintaining high performance.
Memory Allocation in Go
Go’s memory allocator works on two levels:
- Stack Allocation: For small, fixed-size objects with a clear lifetime
- Heap Allocation: For larger or variable-sized objects, or when the compiler can’t determine an object’s lifetime
Let’s look at a practical example of how Go decides where to allocate memory:
func example() string { x := "local variable" // Stack allocated y := make([]int, 1000) // Heap allocated return process(x, y)}
Understanding Go’s Garbage Collector
The garbage collector in Go uses a concurrent mark-and-sweep algorithm with three main phases:
- Mark Setup (STW)
- Marking (Concurrent)
- Sweep (Concurrent)
Optimization Techniques
To help your applications perform better, consider these optimization strategies:
- Object Pooling:
var pool = sync.Pool{ New: func() interface{} { return make([]byte, 1024) },}
- Preallocating Slices:
data := make([]int, 0, expectedSize)
- Minimize Allocations:
// Preferreds := strings.Builder{}s.WriteString("Hello")s.WriteString(" World")
// Instead ofs := "Hello" + " World"
Performance Monitoring and Debugging
Go provides excellent tools for monitoring garbage collection and memory usage:
import "runtime/debug"
func init() { debug.SetGCPercent(100) // Adjust GC frequency}
The GOGC environment variable can also be used to tune garbage collection frequency:
GOGC=50 ./myapp # More frequent GCGOGC=200 ./myapp # Less frequent GC
Best Practices for Memory Optimization
- Use stack allocation when possible
- Avoid unnecessary memory allocation in hot paths
- Consider using sync.Pool for frequently allocated objects
- Profile your application using Go’s built-in tools
- Monitor GC patterns in production
Remember that premature optimization is the root of all evil. Always profile first to identify real bottlenecks before implementing optimizations. Go’s memory management system is highly sophisticated and works well for most applications out of the box.
By understanding these concepts and applying them thoughtfully, you can create efficient and performant Go applications while letting the garbage collector handle most of the heavy lifting for you.
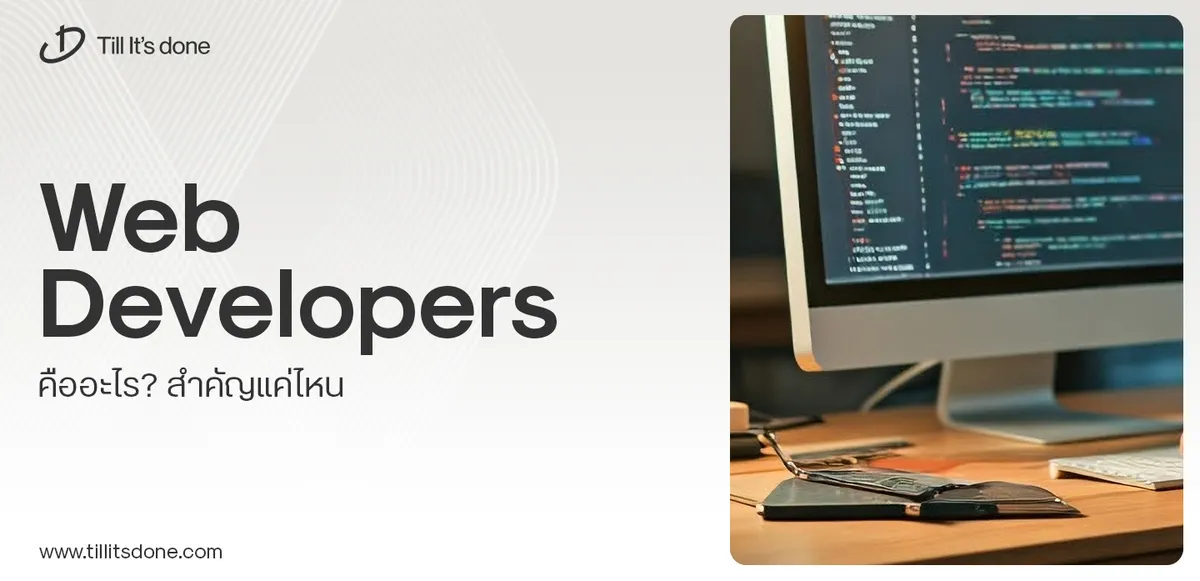
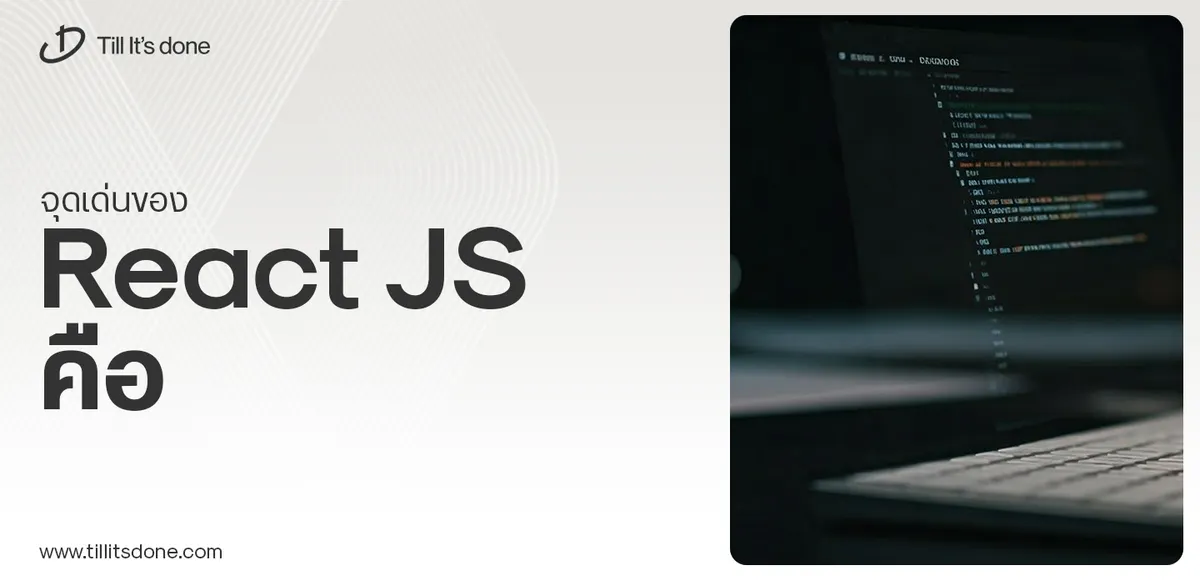
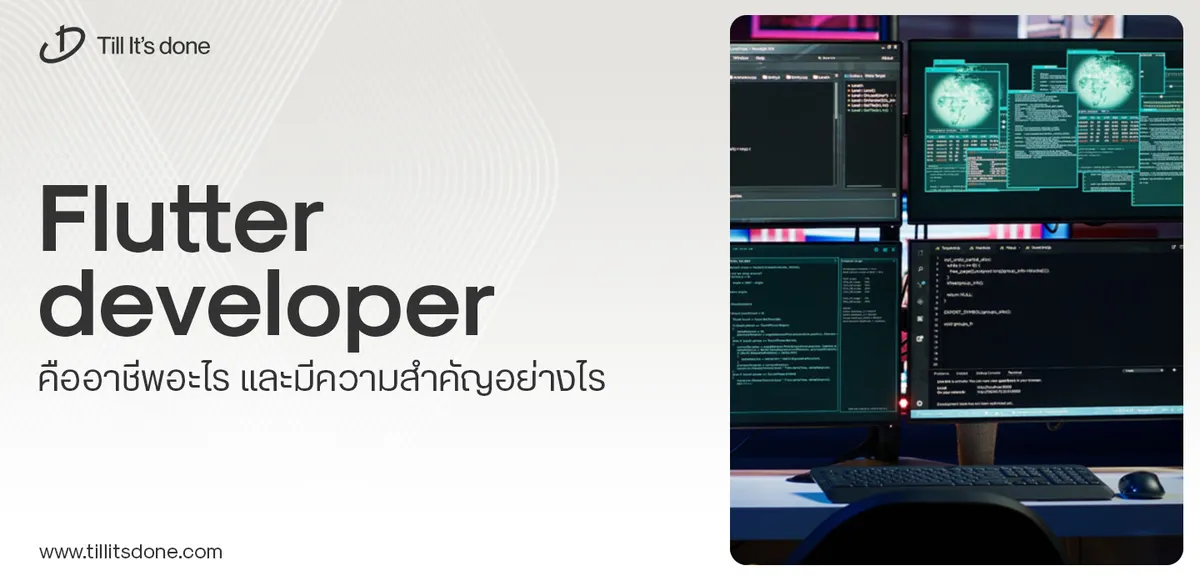
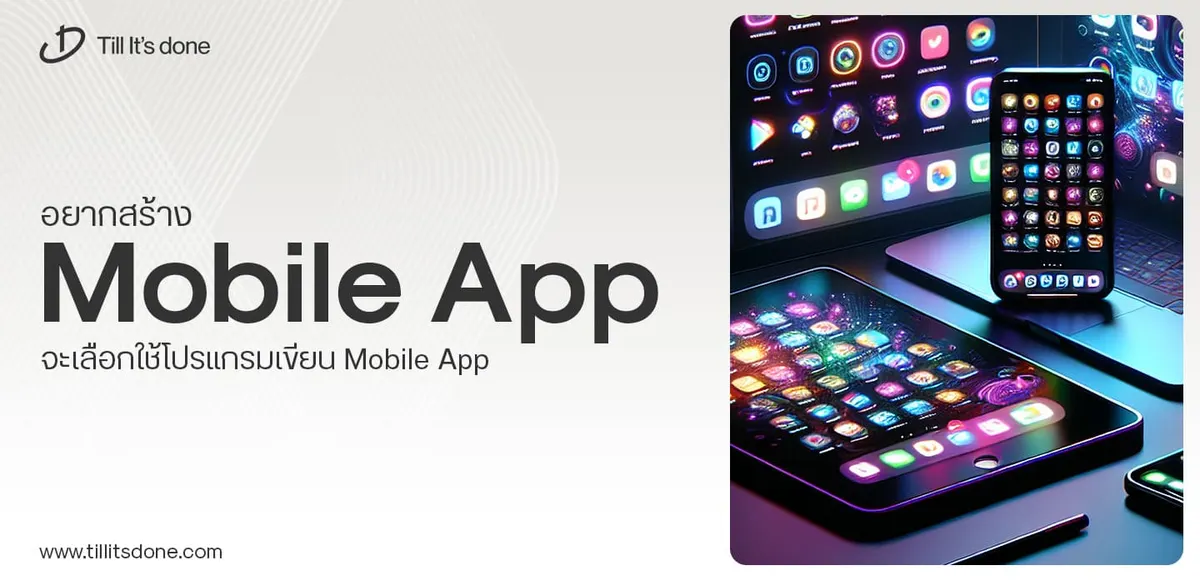
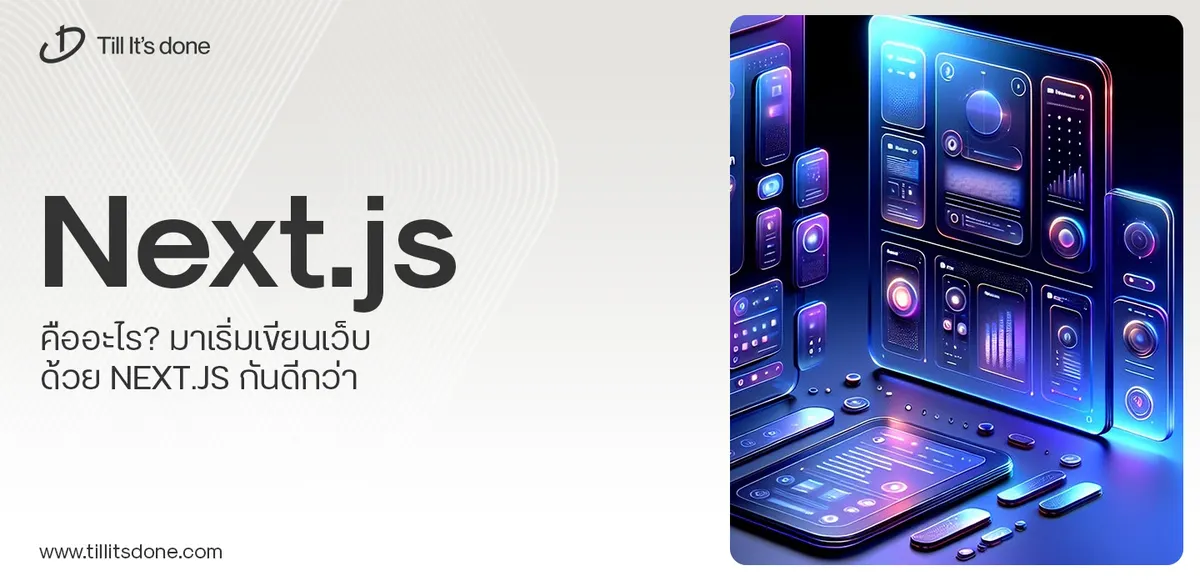
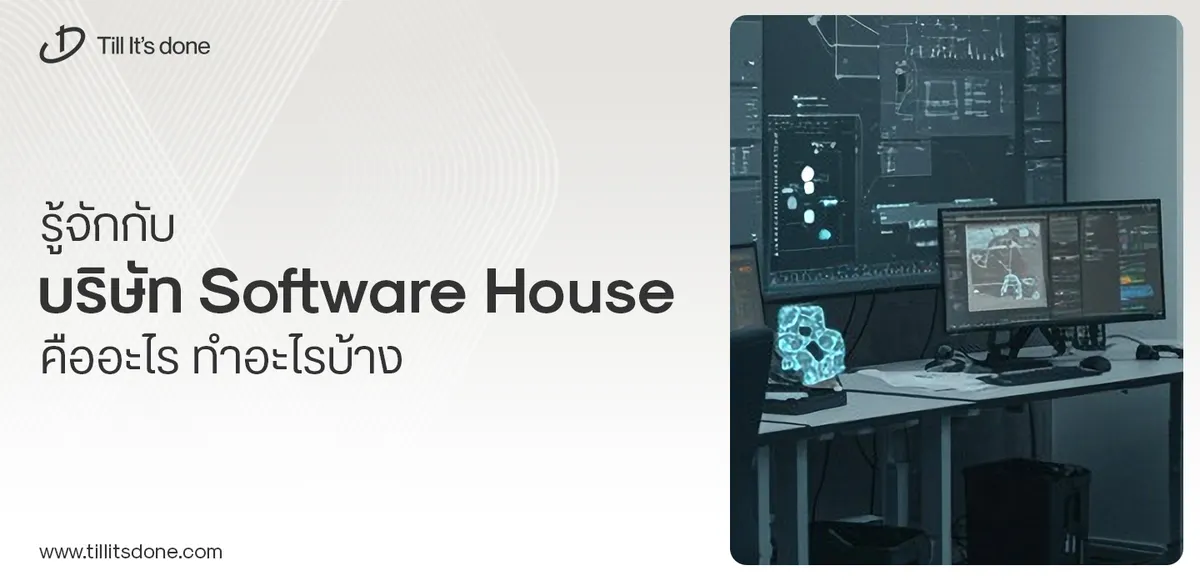
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.