- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Go Reflection: How It Works and When to Use It
Learn when to use reflection effectively in your Go projects.

Go Reflection: How It Works and When to Use It
Have you ever wondered how certain frameworks and libraries in Go can work with any type of data structure? The secret lies in a powerful feature called reflection. While it might seem like magic at first, understanding reflection can open up new possibilities in your Go programming journey.
Understanding Go Reflection
At its core, reflection is the ability of a program to examine and modify its structure and behavior at runtime. Think of it as holding up a mirror to your code - you can see and interact with things that are usually hidden during compile time.
The Three Laws of Reflection
- Types to Reflection: Any variable can tell us its type through reflection
- Reflection to Types: We can create a type from its reflection representation
- Modifiability: To modify a reflection object, it must be settable
Let’s explore each of these with practical examples:
type Person struct { Name string Age int}
func main() { p := Person{"Alice", 30}
// First law: Getting type information t := reflect.TypeOf(p) fmt.Printf("Type: %v\n", t)
// Second law: Examining values v := reflect.ValueOf(p) for i := 0; i < t.NumField(); i++ { fmt.Printf("Field: %s\n", t.Field(i).Name) fmt.Printf("Value: %v\n", v.Field(i).Interface()) }}
When to Use Reflection
While reflection is powerful, it’s important to use it judiciously. Here are some appropriate use cases:
- Generic Data Handling: When building functions that need to work with different types
- Framework Development: Creating ORMs, serialization libraries, or dependency injection containers
- Testing and Debugging: Inspecting private fields during testing
- Configuration Systems: Mapping configuration files to structs
However, remember that reflection comes with trade-offs:
- Slower performance compared to direct code
- Less type safety at compile-time
- More complex and harder to maintain code
- Potential runtime panics if not handled carefully
Best Practices
- Validate Before Reflecting: Always check if your reflection operations are valid
- Cache Type Information: Store reflected types if you’ll use them repeatedly
- Document Extensively: Make it clear when and why you’re using reflection
- Consider Alternatives: Often, interfaces or generics might be better solutions
Here’s a practical example of proper reflection usage:
func setPropSafely(obj interface{}, prop string, value interface{}) error { // Get the value we're working with v := reflect.ValueOf(obj)
// Check if we have a pointer if v.Kind() != reflect.Ptr { return fmt.Errorf("object must be a pointer") }
// Get the field we want f := v.Elem().FieldByName(prop)
if !f.IsValid() { return fmt.Errorf("invalid property: %s", prop) }
if !f.CanSet() { return fmt.Errorf("cannot set property: %s", prop) }
// Set the value safely fv := reflect.ValueOf(value) if f.Type() != fv.Type() { return fmt.Errorf("invalid value type") }
f.Set(fv) return nil}
Conclusion
Reflection in Go is like having a Swiss Army knife in your programming toolkit. While powerful, it should be used thoughtfully and only when simpler solutions won’t suffice. Understanding its capabilities and limitations will help you make better decisions about when to employ this powerful feature.
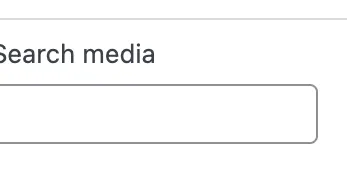





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.