- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Integrating Mockito with Flutter Widget Testing
Discover best practices, implementation tips, and practical examples for creating reliable test suites.

Integrating Mockito with Flutter’s Widget Testing: A Practical Guide
Testing is a crucial part of any software development process, and Flutter’s widget testing framework combined with Mockito gives us a powerful toolkit for ensuring our apps work as expected. Today, let’s dive into how we can effectively use Mockito in our Flutter widget tests to create more reliable and maintainable test suites.
Getting Started with Mockito
First things first, we need to add the necessary dependencies to our pubspec.yaml
file. We’ll need both the mockito
package and build_runner
for code generation:
dev_dependencies: mockito: ^5.4.0 build_runner: ^2.4.0 flutter_test: sdk: flutter
Understanding the Basics
When writing widget tests with Mockito, we’re essentially creating fake objects that mimic the behavior of real dependencies. This is particularly useful when testing widgets that depend on services, repositories, or other external dependencies.
Let’s say we have a simple weather app that displays the current temperature. We’ll need to mock our weather service to test our widget without making actual API calls.
// Define our mock classclass MockWeatherService extends Mock implements WeatherService {}
// Our widget testtestWidgets('WeatherWidget displays temperature correctly', (WidgetTester tester) async { // Create the mock final mockWeatherService = MockWeatherService();
// Define mock behavior when(mockWeatherService.getTemperature()) .thenAnswer((_) async => 25);
// Build widget with mock await tester.pumpWidget( MaterialApp( home: WeatherWidget(weatherService: mockWeatherService), ), );
// Verify the temperature is displayed expect(find.text('25°C'), findsOneWidget);});
Best Practices
- Reset Mocks Between Tests: Always reset your mocks between tests to ensure clean state:
setUp(() { mockWeatherService = MockWeatherService();});
- Verify Interactions: Use Mockito’s verify function to ensure your widget is interacting with dependencies correctly:
verify(mockWeatherService.getTemperature()).called(1);
- Handle Async Operations: Remember to use
pump()
orpumpAndSettle()
when testing widgets with async operations:
await tester.pump(Duration(milliseconds: 500));
This approach to testing helps us catch issues early and ensures our widgets behave correctly under different scenarios. Remember, good tests act as documentation for your code and make refactoring much safer.
Conclusion
Integrating Mockito with Flutter’s widget testing framework provides a robust solution for testing complex widgets with external dependencies. By following these patterns and best practices, you can create maintainable and reliable test suites that give you confidence in your code.



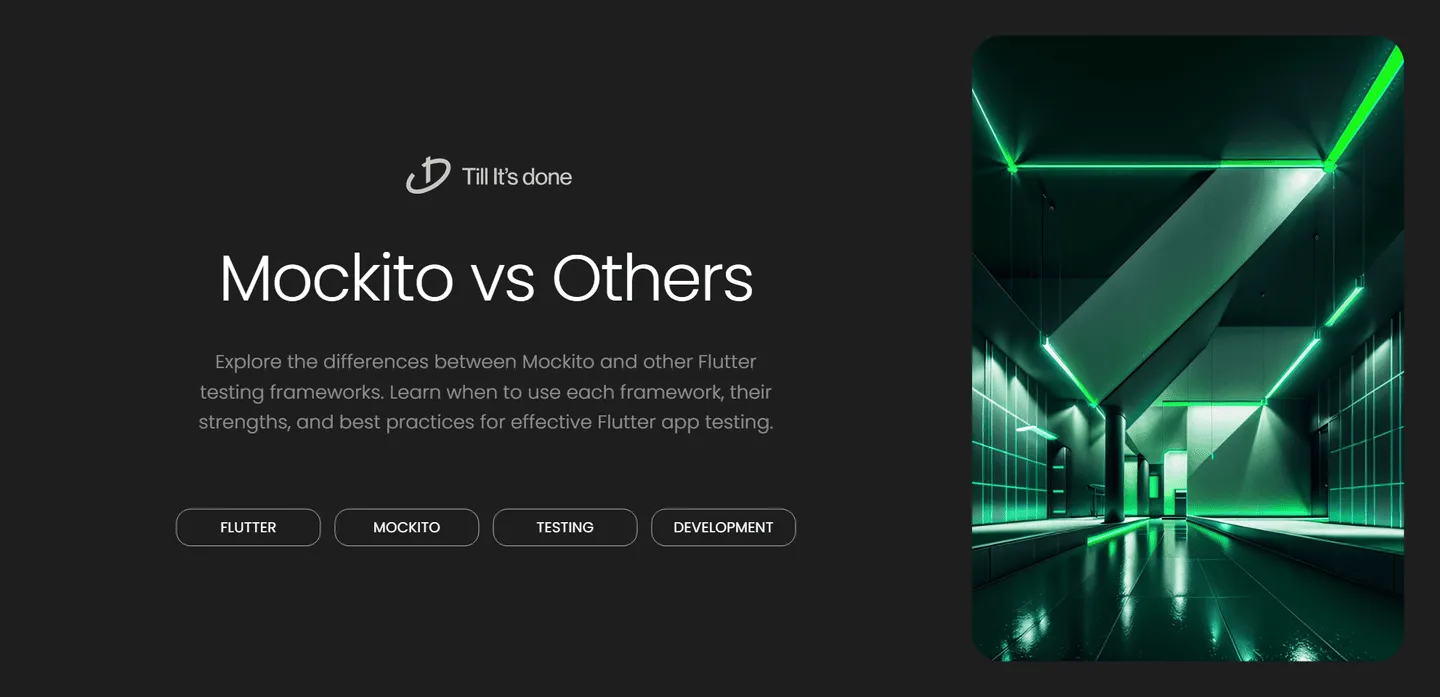


Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.