- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Understanding Mockito Package for Flutter Testing

Understanding the Mockito Package for Flutter Testing
Testing is a crucial aspect of app development that helps ensure your code works as intended. When it comes to testing Flutter applications, the Mockito package is your best friend. Let’s dive into how this powerful testing tool can make your life easier.
What is Mockito?
Think of Mockito as your personal stunt double for testing. Just like how movies use stunt doubles to perform complex scenes, Mockito creates “doubles” of your classes and objects. These doubles mimic the behavior of real objects, but in a controlled way that’s perfect for testing.
Why Do We Need Mocking?
Imagine you’re testing a feature that relies on data from an API. You wouldn’t want to make actual API calls during testing – they’re slow, unreliable, and might cost money! This is where mocking shines. By creating mock objects, you can simulate API responses and test your code’s behavior under different scenarios.
Getting Started with Mockito
To use Mockito in your Flutter project, you’ll need two packages:
dev_dependencies: mockito: ^5.4.0 build_runner: ^2.4.0
The build_runner package is necessary because Mockito uses code generation to create its magic. After adding these dependencies, you’re ready to start mocking!
Creating and Using Mocks
Let’s say you have a simple weather service class:
class WeatherService { Future<String> getWeatherForecast(String city) async { // API call logic here return 'Sunny'; }}
To mock this service, you’ll first create a mock class:
@GenerateMocks([WeatherService])void main() {}
Run the build_runner to generate the mock:
flutter pub run build_runner build
Now you can use the mock in your tests:
void main() { late MockWeatherService mockWeatherService;
setUp(() { mockWeatherService = MockWeatherService(); });
test('should return weather forecast', () async { when(mockWeatherService.getWeatherForecast('London')) .thenAnswer((_) async => 'Rainy');
final forecast = await mockWeatherService.getWeatherForecast('London'); expect(forecast, 'Rainy'); });}
Best Practices and Tips
- Always verify important interactions using
verify()
:
verify(mockWeatherService.getWeatherForecast('London')).called(1);
- Use
thenThrow()
to test error scenarios:
when(mockWeatherService.getWeatherForecast(any)) .thenThrow(Exception('Network error'));
- Reset mocks between tests using
reset()
in thesetUp()
method - Be specific with your mocks – mock only what you need to test
Conclusion
Mockito is an invaluable tool in your Flutter testing arsenal. It helps you write reliable, fast, and maintainable tests by providing a way to isolate the code you’re testing. Remember, good tests make for good apps, and Mockito makes writing good tests a whole lot easier.



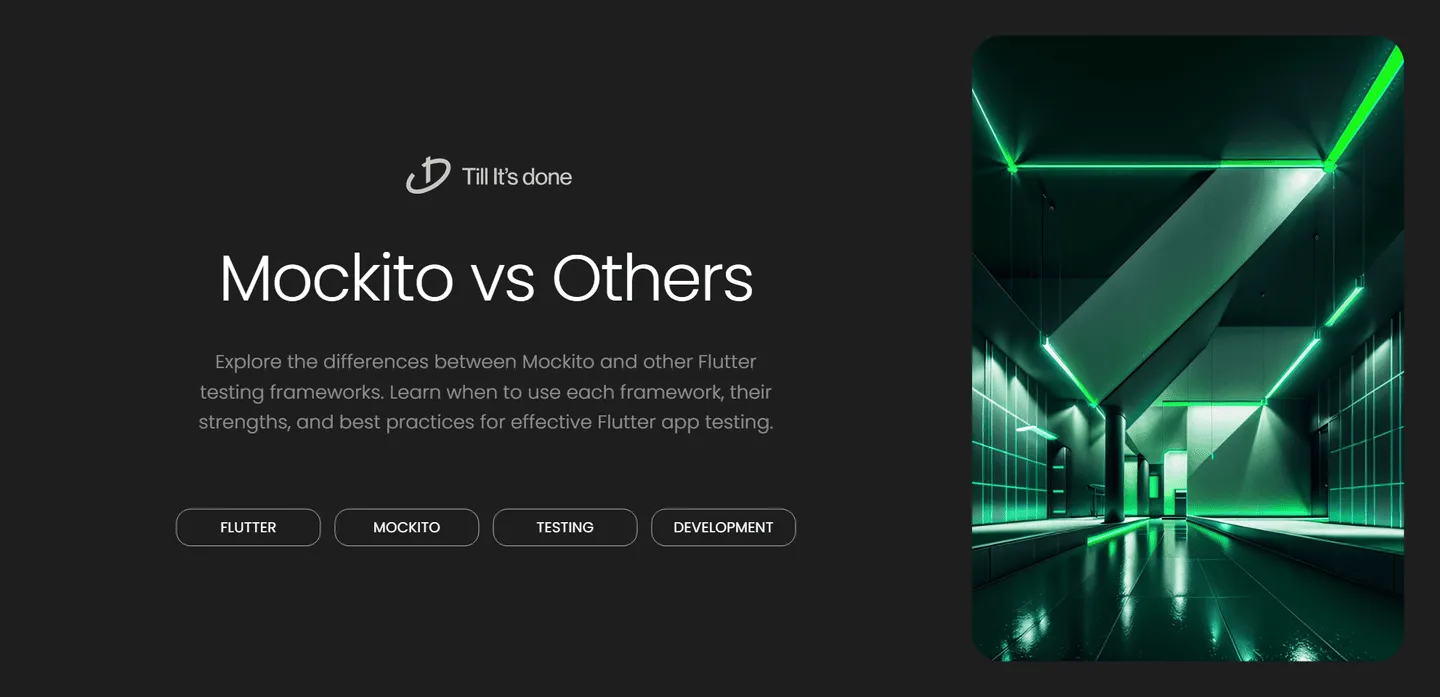


Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.