- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Mock Dependencies in Flutter with Mockito
Master the art of creating maintainable tests with practical examples and best practices.
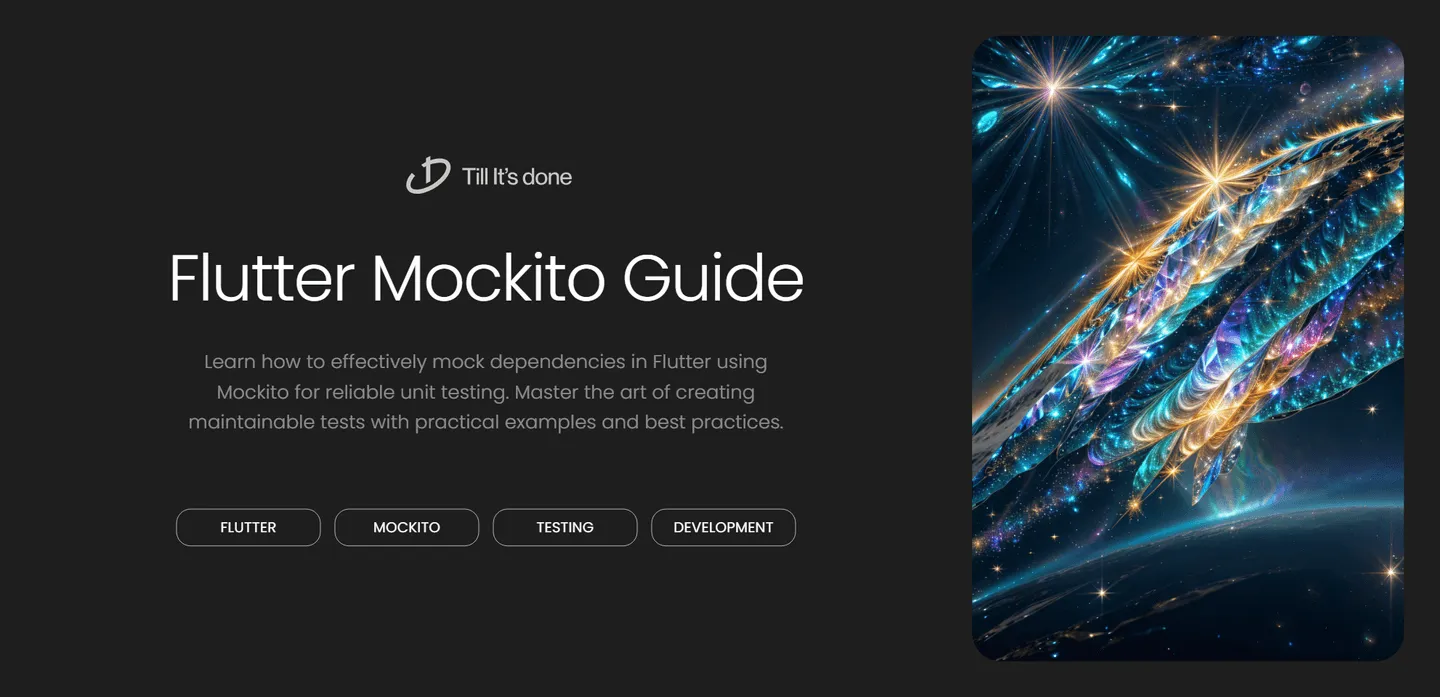
Testing is a crucial aspect of developing robust Flutter applications, and when it comes to unit testing, mocking dependencies is essential. In this guide, we’ll explore how to effectively use Mockito in Flutter to create reliable and maintainable tests.
Understanding Mocking and Mockito
Think of mocking as creating a stunt double for your real dependencies. Just like how movies use stunt doubles to perform dangerous scenes, we use mocks to simulate complex dependencies in our tests. Mockito is our talented casting director that helps us create and manage these stunt doubles.
Setting Up Your Testing Environment
First, let’s add the necessary dependencies to your pubspec.yaml
:
dev_dependencies: mockito: ^5.4.4 build_runner: ^2.4.8
Creating Your First Mock
Let’s say we have a weather service that fetches weather data:
class WeatherService { Future<String> getWeatherForecast() async { // API call implementation return 'Sunny'; }}
To mock this service, we create a mock class:
@GenerateMocks([WeatherService])void main() {}
Run the build runner to generate the mock:
flutter pub run build_runner build
Writing Tests with Mocks
Here’s how to use your mock in a test:
void main() { late MockWeatherService mockWeatherService; late WeatherBloc weatherBloc;
setUp(() { mockWeatherService = MockWeatherService(); weatherBloc = WeatherBloc(weatherService: mockWeatherService); });
test('should get weather forecast', () async { // Arrange when(mockWeatherService.getWeatherForecast()) .thenAnswer((_) async => 'Sunny');
// Act await weatherBloc.fetchWeather();
// Assert verify(mockWeatherService.getWeatherForecast()).called(1); expect(weatherBloc.state.weather, equals('Sunny')); });}
Best Practices and Tips
- Keep it Simple: Only mock what you need. Over-mocking can lead to brittle tests.
- Verify Interactions: Use
verify()
to ensure your code interacts with dependencies correctly. - Handle Errors: Test both success and failure scenarios using
thenAnswer()
andthenThrow()
. - Reset Mocks: Use
reset()
intearDown()
to ensure a clean slate between tests.
Common Pitfalls to Avoid
- Don’t mock value objects or simple structures
- Avoid mocking everything - sometimes real implementations are better
- Remember to handle async operations properly with
async/await
Mocking dependencies in Flutter using Mockito doesn’t have to be complicated. By following these patterns and practices, you can write cleaner, more maintainable tests that give you confidence in your code. Remember, the goal is to make your tests reliable and easy to understand, not to mock everything in sight.
Happy testing! 🚀
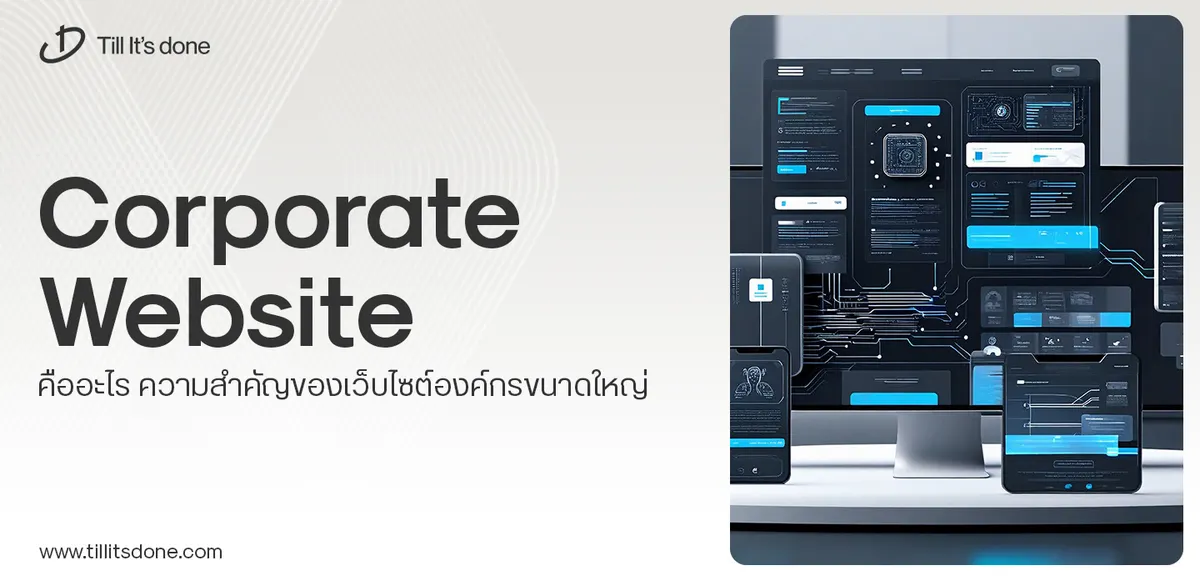
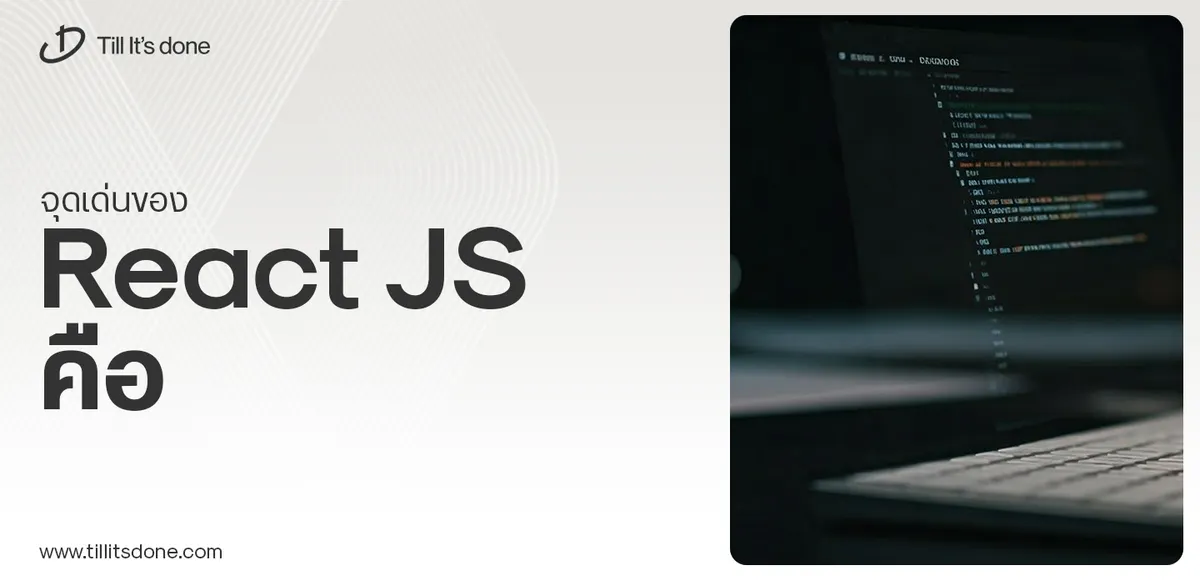
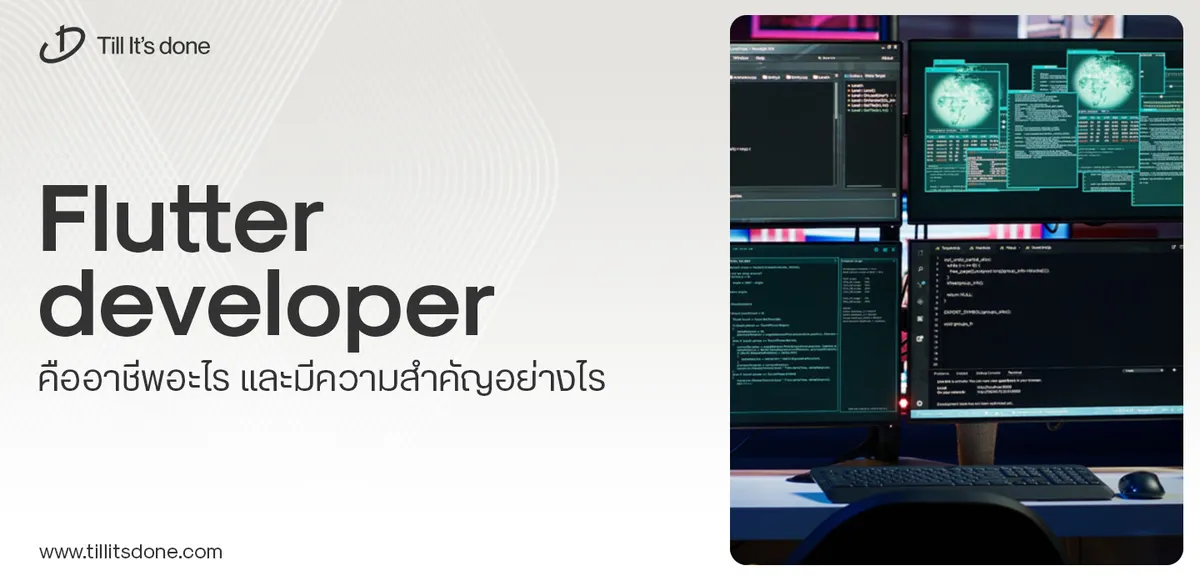
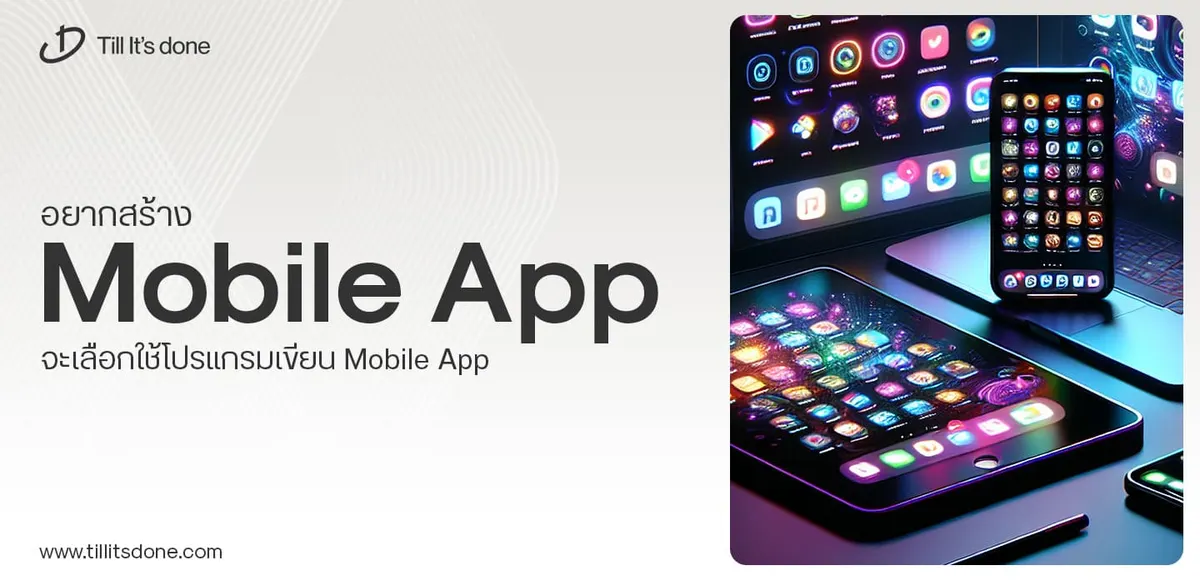
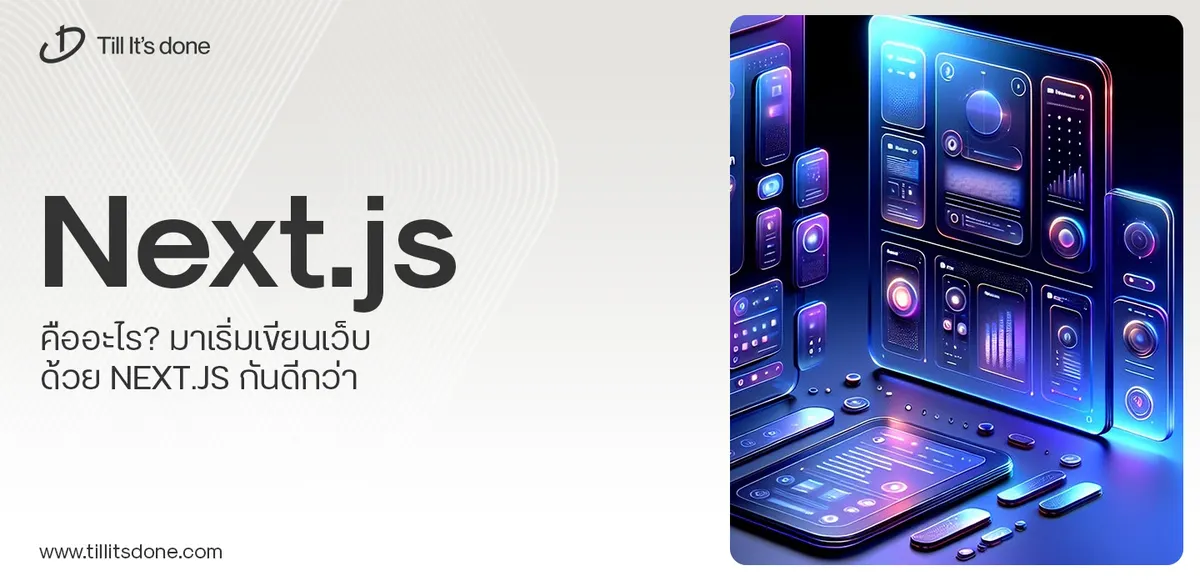
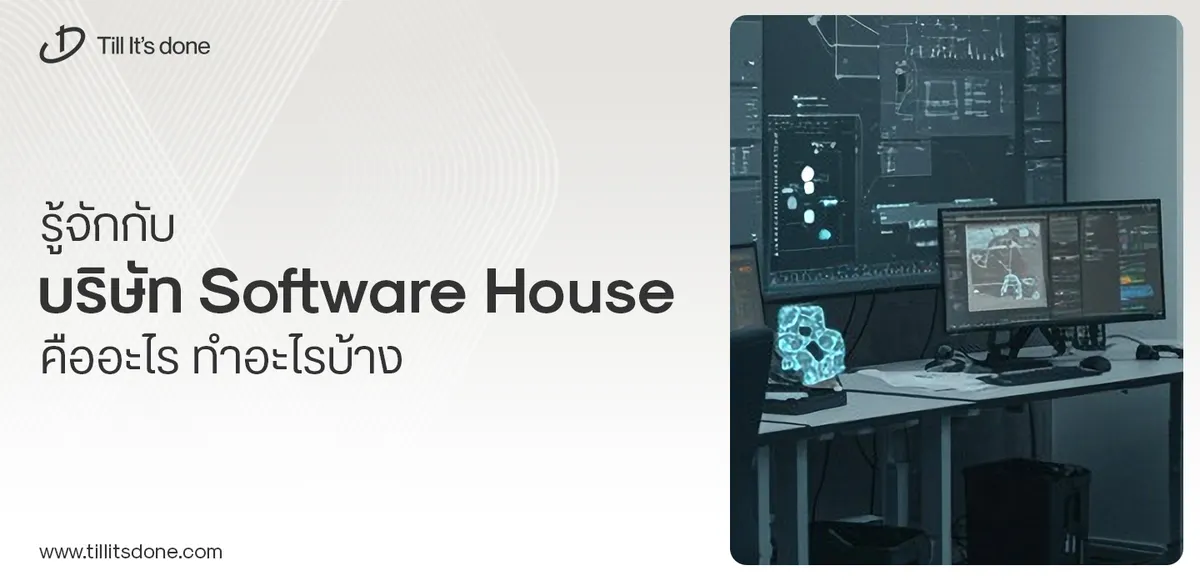
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.