- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master Mockito in Flutter Testing: A Guide
Discover practical examples, best practices, and tips for writing maintainable and reliable tests in your Flutter applications.
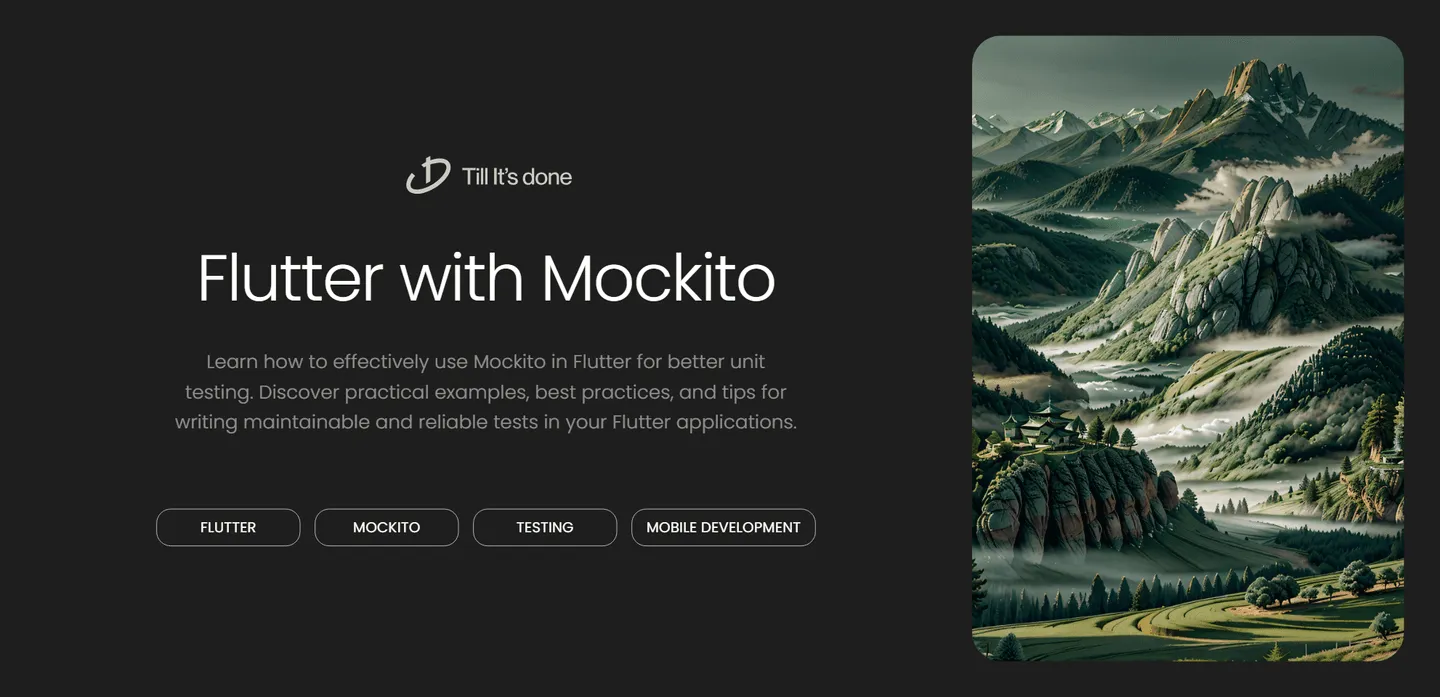
Introduction to Mockito in Flutter Testing
Testing is a crucial aspect of developing robust Flutter applications, and Mockito stands out as an invaluable tool in our testing arsenal. Today, let’s dive into how Mockito can transform your Flutter testing approach and make your tests more reliable and maintainable.
What is Mockito?
Think of Mockito as your personal stunt double for testing. Just as stunt doubles replace actors in challenging scenes, Mockito creates stand-ins for your complex dependencies. These “mock” objects simulate real object behavior, allowing you to test your code in isolation.
Getting Started with Mockito
First things first, add Mockito to your pubspec.yaml:
dev_dependencies: mockito: ^5.4.0 build_runner: ^2.4.6
Let’s look at a practical example. Imagine we have a weather app that fetches data from an API:
class WeatherService { Future<String> getWeatherForCity(String city) async { // API call logic here return 'Sunny'; }}
class WeatherBloc { final WeatherService weatherService;
WeatherBloc(this.weatherService);
Future<String> getWeather(String city) async { return await weatherService.getWeatherForCity(city); }}
Here’s how we can test this using Mockito:
@GenerateMocks([WeatherService])void main() { test('should return weather for city', () async { // Arrange final weatherService = MockWeatherService(); final weatherBloc = WeatherBloc(weatherService);
when(weatherService.getWeatherForCity('London')) .thenAnswer((_) async => 'Rainy');
// Act final result = await weatherBloc.getWeather('London');
// Assert expect(result, 'Rainy'); verify(weatherService.getWeatherForCity('London')).called(1); });}
Best Practices for Using Mockito
- Keep Mocks Simple: Mock only what you need. Over-mocking can lead to brittle tests.
- Use Verify Wisely: Verify important interactions, but don’t verify everything.
- Test Edge Cases: Use Mockito to simulate error conditions and edge cases easily.
- Maintain Readability: Structure your tests with Arrange-Act-Assert pattern.
Conclusion
Mockito is more than just a testing library – it’s a powerful ally in creating maintainable and reliable Flutter applications. By isolating components and simulating dependencies, we can write tests that are both thorough and efficient.
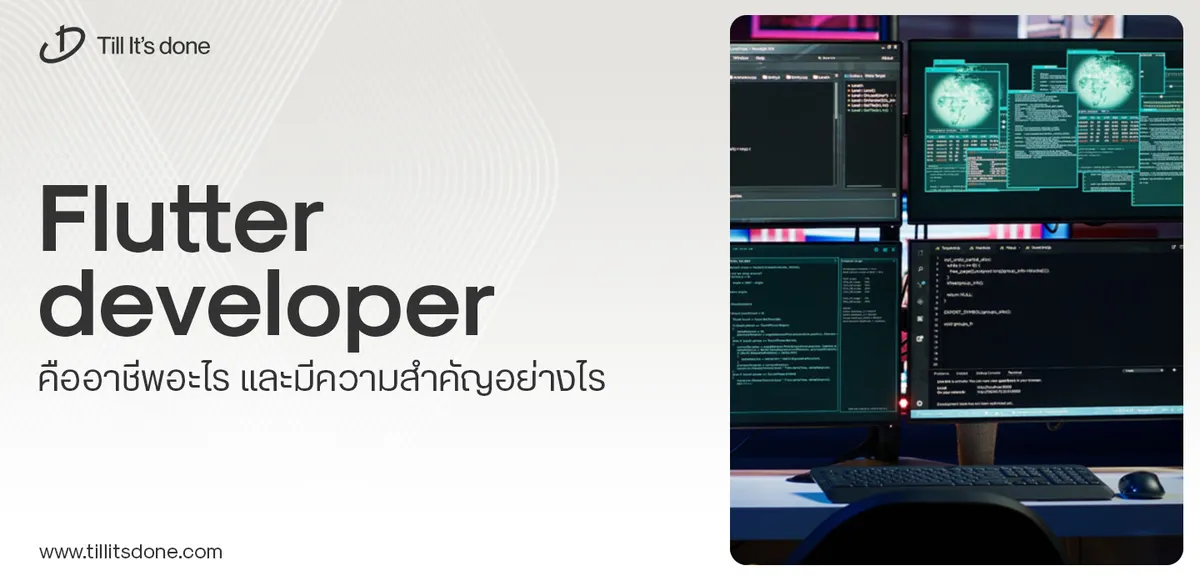
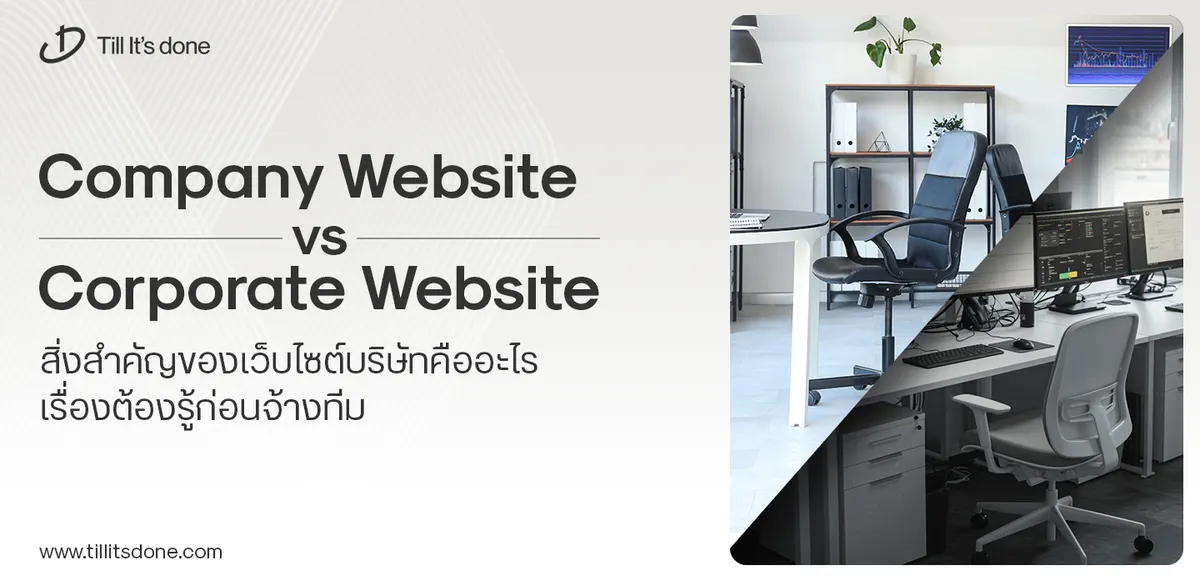
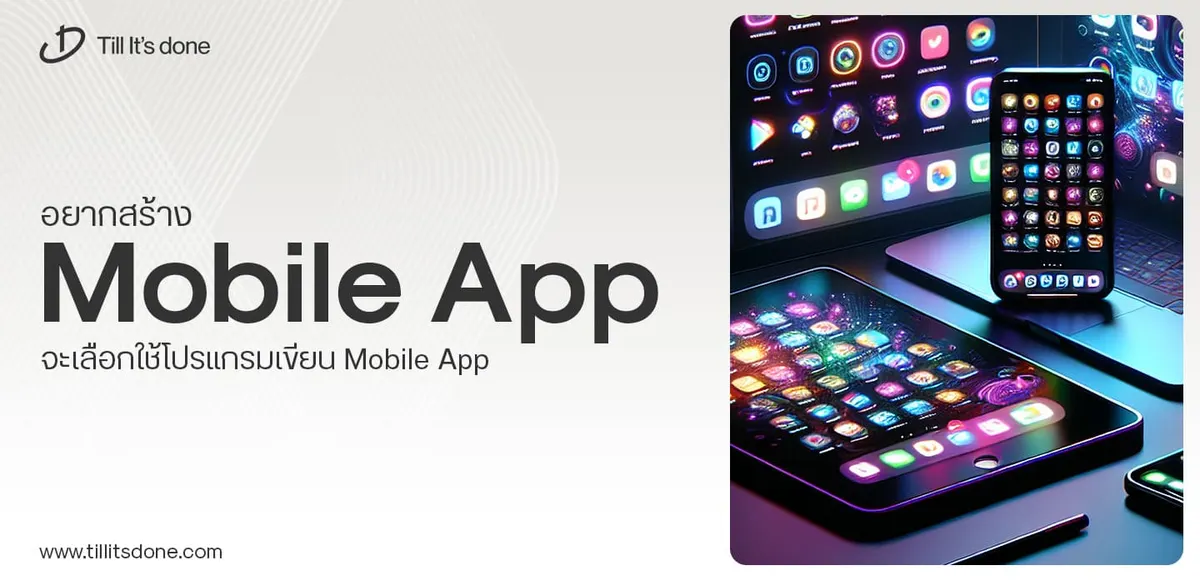
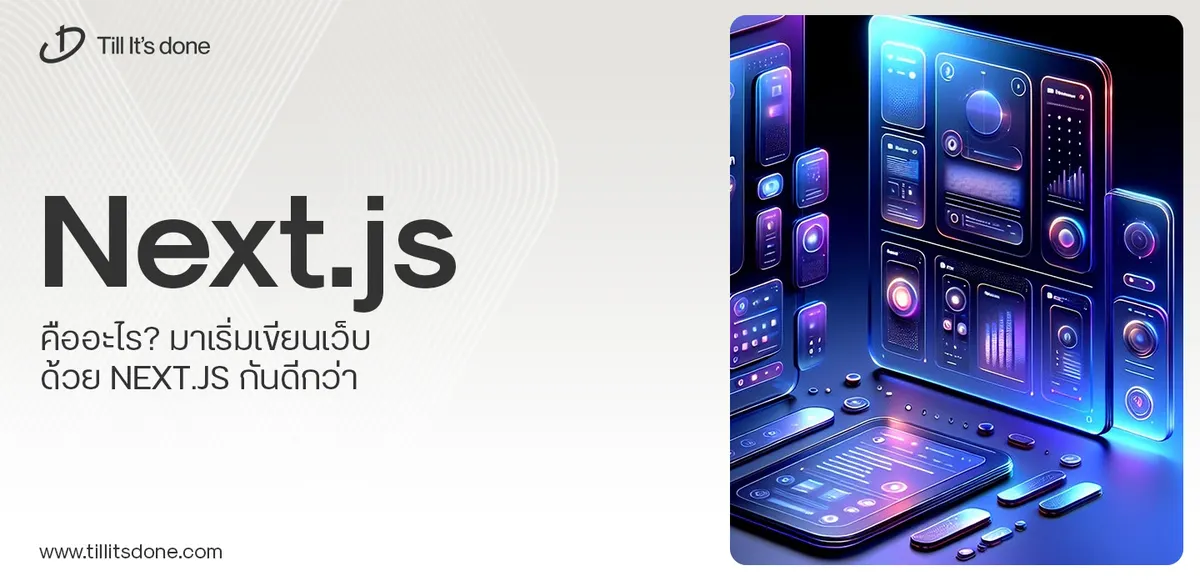
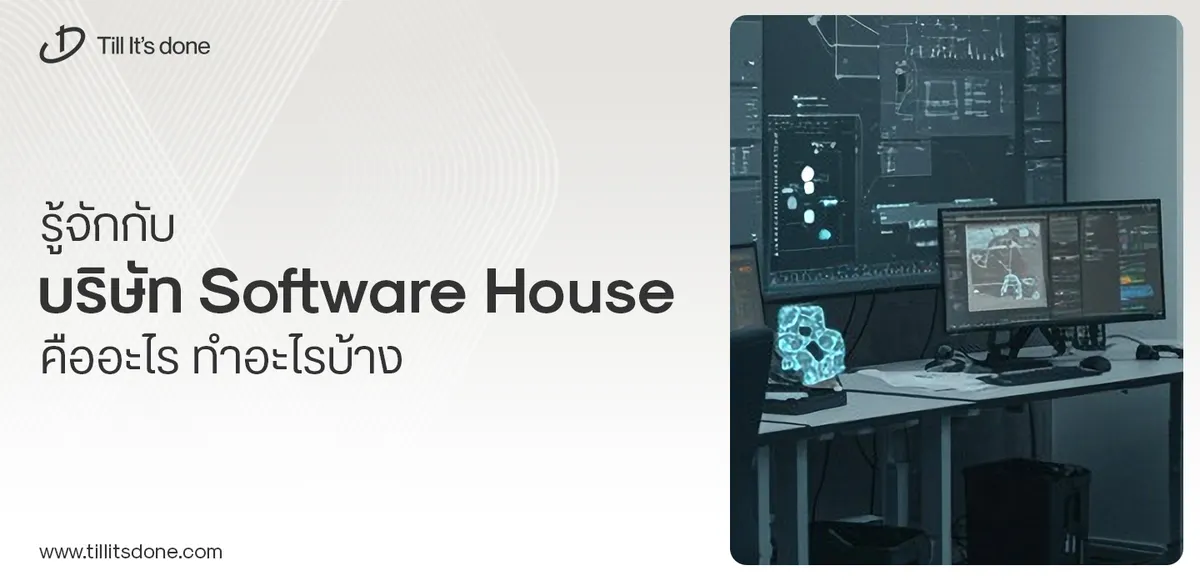
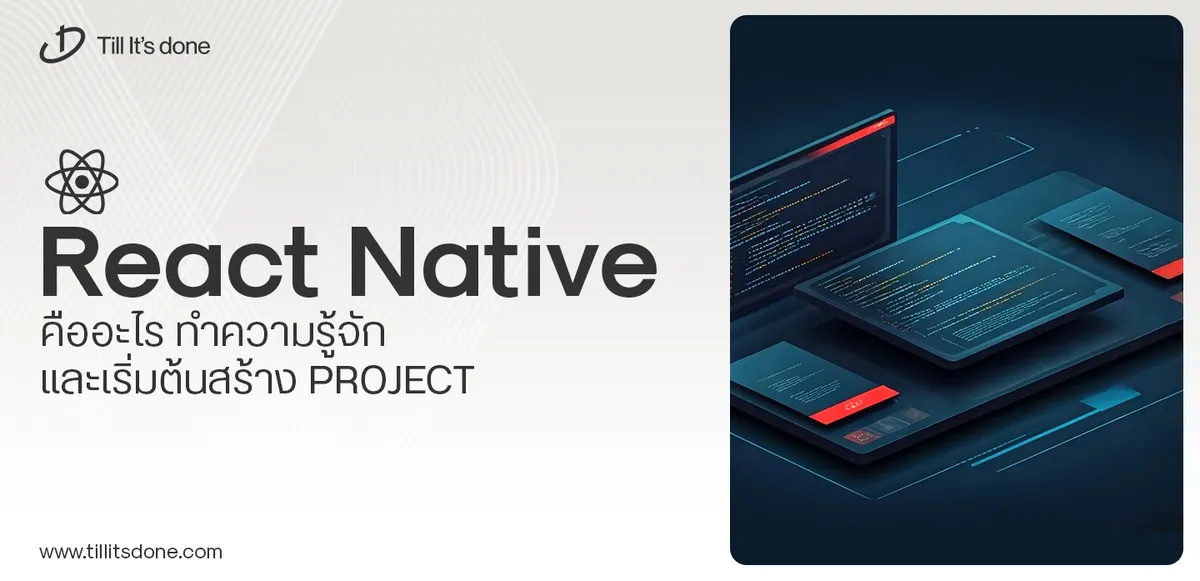
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.